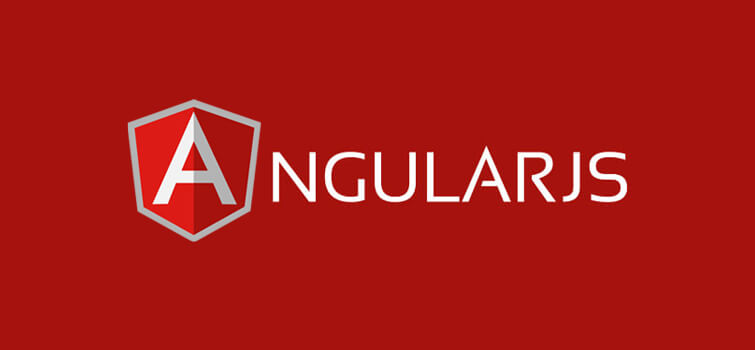
What is AngularJS ?
AngularJS provides an excellent framework for building interactive websites. It is an open-source framework developed by Google and used by most of the developers across the globe. Since its inception in 2009, it has been one of the most popular and efficient Java framework. AngularJS is a structural framework designed to simplify the development of website’s front-end.
One of the main reasons for the popularity of Angular JS is that it lets you use HTML as a template language. While HTML is a great declarative language for documents, it is not very efficient for applications. AngularJS extends the capability of HTML by allowing you to modify different elements of your web-page.
What Makes AngularJS Different ?
Static documents and dynamic applications require different approaches. The mismatch between HTML and different application development frameworks can be frustrating for the programmers. AngularJS solves this issue by data binding and code reuse. In simpler words, AngularJS teaches your browser a new syntax through directives.
Another main reason for the popularity of AngularJS is the fact that it presents a higher level of abstraction to the developers. It is, therefore, no surprise that AngularJS continues to be in the list of most popular JavaScript frameworks by many Magento 2 web development firms.
Example:
<!DOCTYPE html>
<html>
<script src= “http://ajax.googleapis.com/ajax/libs/angularjs/1.3.14/angular.min.js”></script>
<body>
<p>Try to change the names.</p>
<div ng-app=”myApp” ng-controller=”myCtrl”>
First Name: <input type=”text” ng-model=”firstName”><br>
Last Name: <input type=”text” ng-model=”lastName”><br>
<br>
Full Name: {{firstName + ” ” + lastName}}
</div>
<script>
var app = angular.module(‘myApp’, []);
app.controller(‘myCtrl’, function($scope) {
$scope.firstName= “John”;
$scope.lastName= “Doe”;
});
</script>
</body>
</html>
Why AngularJS?
AngularJS is backed by Google. This is a huge relief for developers to operate on a stable code base that will offer the application maximum support. it’s a stable platform to focus on. Many frameworks are nowadays a pure bundling of existing resources. They are an integrated group of tools but they are not very elegant. AngularJS is the next generation platform where each tool was built to function in an integrated manner with any other tool. Please find below some reasons why AngularJS is considered as best among its competitors:
MVC inbuilt support
Most frameworks enforce MVC by asking you to break your app into MVC components and then allow you to write code to re-string them. This is a lot of work. As a developer we have to just split the framework into MVC components and leave everything to AngularJS for taking care.
Declarative User Interface
AngularJS uses HTML to describe the user interface within the program. HTML is a more concise and less complex declarative language than the procedural interpretation of the JavaScript interface HTML is also less brittle to reorganize than a JavaScript-written GUI, so things are less likely to break. And when the view is written in HTML, you can put in a lot more UI developers.
Data models are POJO
The POJO acronym for plain old java objects are being used as data models in AngularJS and it works without superfluous functions getter and setter. You can include and change properties straightforwardly and circle over objects and exhibits voluntarily. Your code will look a lot of cleaner and progressively instinctive, the way mother nature expected.
Behaviors with Directive
Directives are the way AngularJS brings added functionality to HTML. Imagine a world in which HTML has so many rich elements ( e.g. < accordion>, < grid>, < lightbox>, etc.) that we never have to manipulate a DOM to simulate them. To get some functionality out of the box, all that our app needs to do is assign attributes to items.
Flexibility with filter
Before displaying data on UI, filters filter the data and may include anything as simple as formatting decimal places on a list, reversing the order of an array, filtering a parameter-based array, or implementing pagination. Filters are designed to be stand-alone features, similar to instructions, independent from your device, but concerned only with data transformations.
Less code
All the points you have received up to now mean you ‘re getting to write less code. No need to write your own MVC pipeline. The view is described using more succinct, HTML. Without getters / setters, data models are easier to write. Data-binding means you don’t have to manually insert data into the view. Also the directives are different from the software code, they can be written in parallel with limited integration issues by another team. Filters let you manipulate view level data without changing controllers.
Unit testing ready
The definition of AngularJS would be incomplete without speaking of its readiness for unit test. Dependency Injection (DI) links the whole of AngularJS together. Agular’s unit tests will use dependency injection for unit testing .It mocks the data and injects into server. In reality, AngularJS already has a mock HTTP provider for controllers to insert fake server responses. It beats the more conventional way web apps are checked by creating individual test pages that activate a single feature and then communicate with it to see if it is working.
- Google assisted, and a great community of growth.
- Supported by IntelliJ IDEA and Visual Studio .NET IDEs.
Limitations
AngularJS is not a magic bullet. Some of its drawbacks are the backsides of its positive points, some of which are intrinsic to JavaScript inefficiency that could not be solved even with the strongest derivatives of its limitations are listed below:
- AngularJS is broad and complicated. With multiple ways to do the same thing, it’s hard to tell which way is best for a particular task. Mastering AngularJS at the “Hello World” level takes significant effort. Different developers’ coding styles and habits can complicate the integration of different components into a complete solution.
- When the project expands over time, you will most likely need to throw away current implementations and build new ones using different approaches. AngularJS implementations are poor in scale.
- More than 2,000 watchers will seriously lag behind the UI. This limits the complexity of your AngularJS forms , especially large data grids and lists.
TOP 5 APPS AND WEBSITES DEVELOPED USING ANGULARJS
Apps Made with Angularjs now known as Angular
- Gmail App
- YouTube for PS3
- Weather.com
- Upwork
- PayPal
Apps Made with Angularjs now known as Angular
The Google product keeps getting better attracting technical minds and turns out to be one of the hottest discussions. Further below are mentioned some of the top Angular websites
Websites built with AngularJS
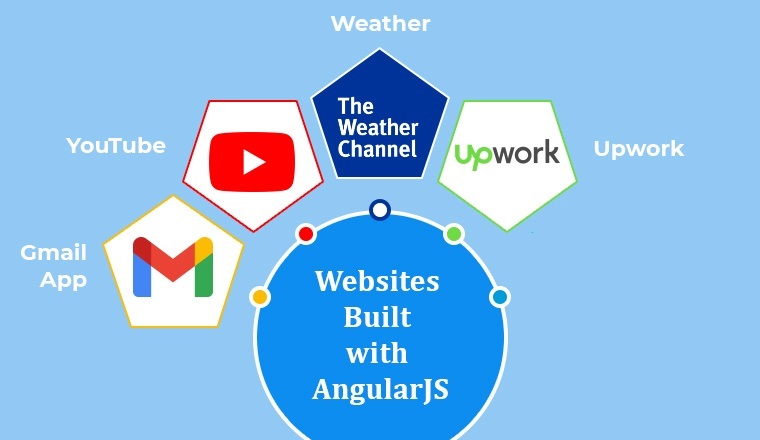
1. Gmail App
This app is one of the best examples of services offered by Angular. Now, what makes Gmail app a cut above is its simple and intuitive user interface. More than 1.5 billion users vouch for the fact that the app leaves no stone unturned when it comes to uninterrupted experience. This is where Angular comes in.
The angular platform was introduced by Google itself so do we have to mention that all Google products make the most of the tech. Now what’s so fascinating about these Gmail apps is they can render data right there on the front-end. Apart from this, it comprises several single-page application advantages such as enabling developers to cache data even when offline.
2. YouTube for PS3
Of course, Youtube doesn’t need any recognition! Available on Sony PlayStation 3, this one is developed using the Javascript framework. As we all know this platform was created keeping the watching and sharing experience of the millions of users in mind. Most of the time, individuals try to upload a video that is liked and shared across the globe. This platform is created using Angular frameworks.
And to achieve that the best thing to do is help people (engineers, companies) to build good framework-based websites and apps easily. And Angular does work wonders here.
3. Weather.com
This website has more than 126 million visitors on a monthly basis, yes you heard it right! This Angular platform is worth considering here! Here you get to see Weather forecasts online, related data and news, interesting facts, entertainment content, and such relatable content that it collects from around the world.
What happens is different teams are assigned to work on specific elements of the website. And fortunately, angular modules are stored in separate directives making it way easier for them to deal with. Other attention-grabbing features offered by Angular frameworks include maps, real-time broadcasting, HD videos with the ariel view, and more.
4. Upwork
Upwork and freelancer.com are another example of websites developed using Angular. After the COVID effect, the IT industry seems to be booming like a fire. This includes the rise in freelancing as well. This angular-based platform serves its best where individuals can work hard and get exactly what they deserve in return. What makes upwork more attractive is its brilliant functionality, best designs, data encryption, seamless navigation within the site.
5. PayPal
Now we have PayPal. Millions and billions of money are being transferred each day with the help of this app. It doesn’t matter whether you are an individual or a business, seamless transfer of money is highly possible with PayPal. At present, you may come across approx 305 million active users or more of the app who are using Paypal for online money transfers.
- What is Mobile Virtual Network Operator? - April 18, 2024
- What is Solr? - April 17, 2024
- Difference between UBUNTU and UBUNTU PRO - April 17, 2024
AngularJS is a popular JavaScript framework developed by Google, designed for building dynamic and responsive web applications. It follows the Model-View-Controller (MVC) architectural pattern, allowing developers to split their application logic, presentation, and data into separate components.
AngularJS extends HTML with its own attributes called directives, which enables the creation of powerful and reusable UI components. It also provides two-way data binding, meaning any changes in the user interface are automatically reflected in the underlying data model and vice versa, simplifying the development process.
To harness the full potential of AngularJS for your projects, you can hire dedicated AngularJS developers. They are skilled professionals who specialize in using AngularJS to create efficient and robust web applications, ensuring a smooth user experience and optimized performance.
Really good blog. Explained very simply and clearly.
Thanks for simple explain the angular js and also providing in-depth knowledge about angular js
angular js is very demanded front end that support mvc with friendly environment