Step:-1 first of all you have to install xampp server use this url for download xampp: https://www.apachefriends.org/index.html
Step:-2 Download gitbash and install the gitbash use this url: https://git-scm.com/downloads
Step:-3 After install xampp serve see the pic how to start xampp server
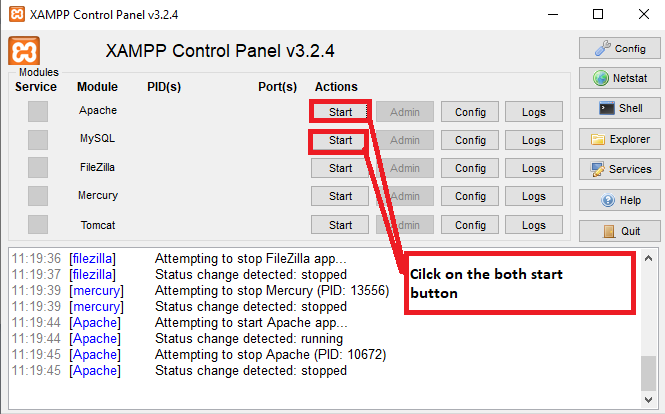
Step:-4 follow this path for gitbash start. C:\xampp\htdocs or see this pic.
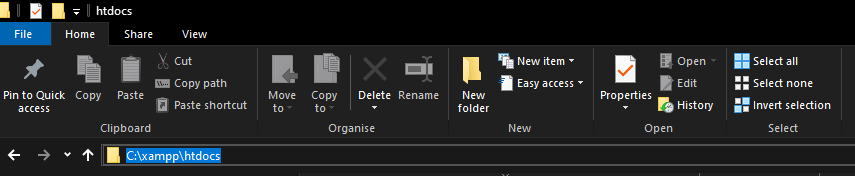
Step:-4 In htdocs area click the right-click and start git bash here or see the pic.
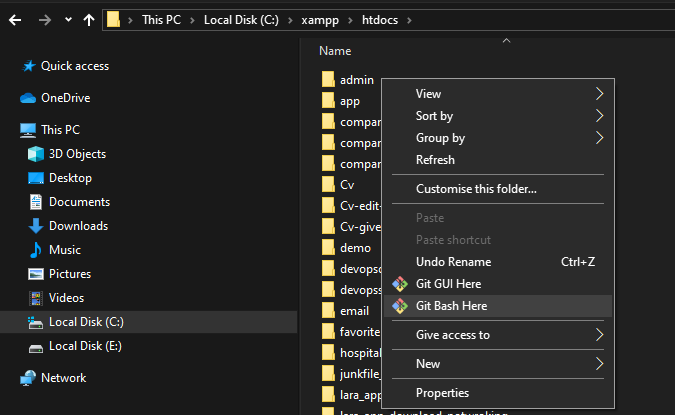
Step:-5 After Click on this Git Bash Here or see the pic
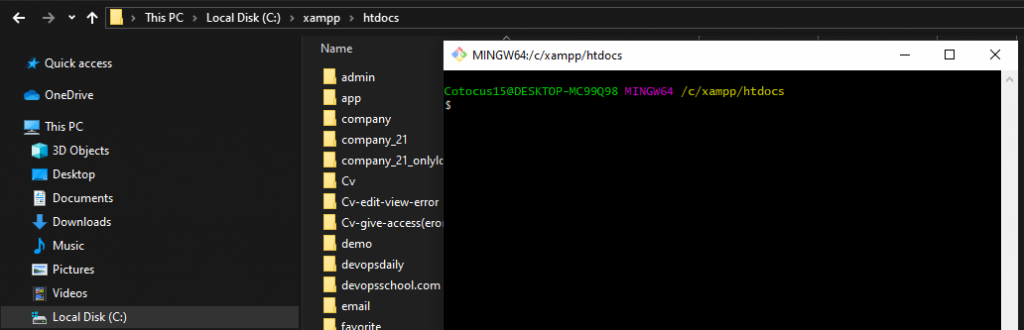
Step:6 Now you to have create one project whatever your project name (example:-hospital)
Step:-7
composer create –project –prefer-dist laravel/laravel hospital “5.5.*” after press Enter than it will take time for intalling your project. after install the project it will look like this.
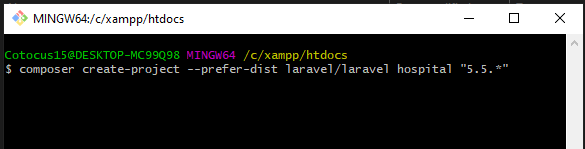
Step:-8 After install the project than you have to go inside project place(example: C:\xampp\htdocs\hospital) or see the pic
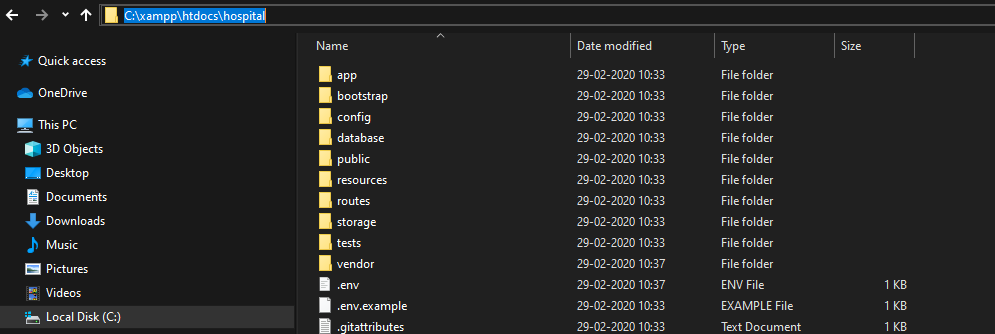
Step:-9 . Open your project in visual code or notepad++ or subline.
Step:-10 Go to .env file and put database name see the pic
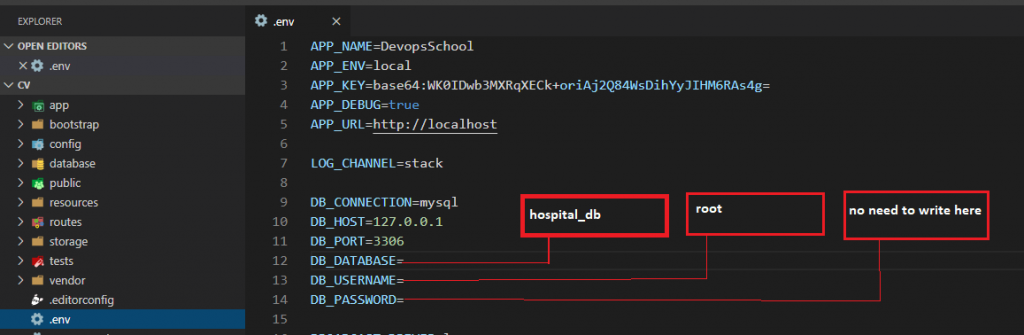
Step:-10 .Than go to this url: http://localhost/phpmyadmin/ and write your database name. or see the pic
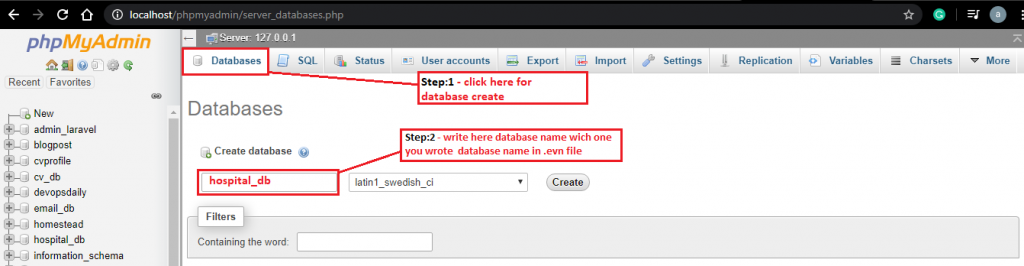
Step:11– open your git bash command and write php artisan make:auth and press enter .or see the pic


Step:12- Now you have to make controller php artisan make:controller Admin/AdminController than press enter or see the pic.
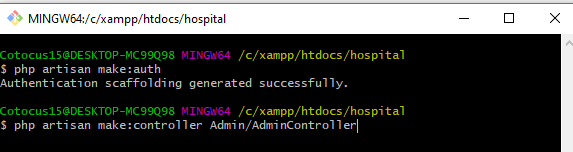
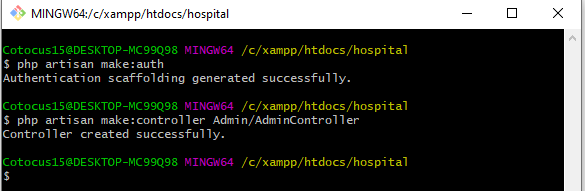
Step:-13– Make DashboardController for Admin Dashboard work (1) php artisan make:controller Admin/DashboardController (2) php artisan make:controller User/DashboardController
Step:-15 Now you have to go this path : Customise users table(database/migration/create_users_table.php) or see the pic
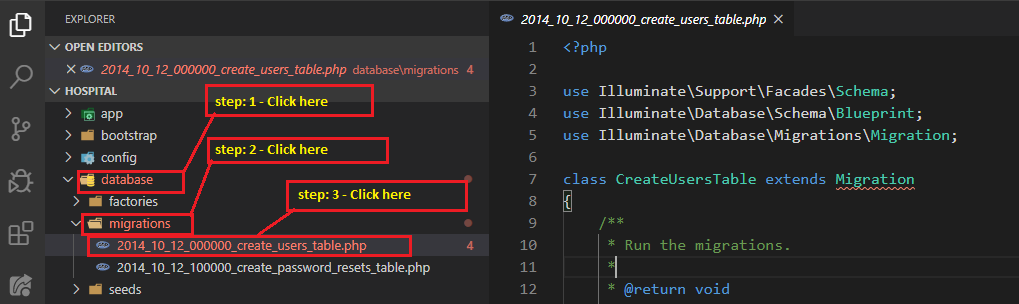
Step:16– Now you have to go : database/migration/create_users_table.php than write this code:
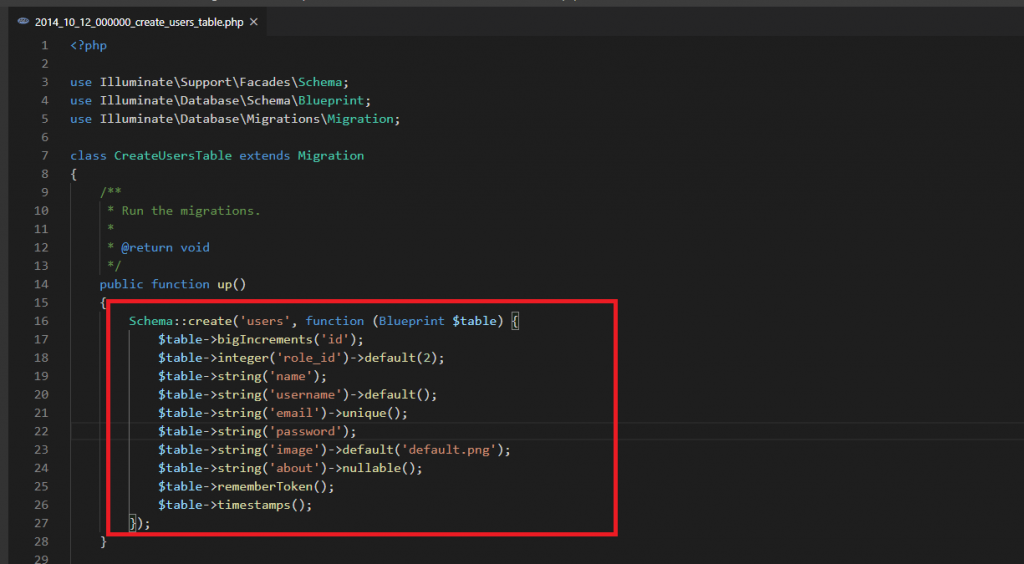
Step:-17 Make Model and Migration for Role Table. Run this code in git bash cammand. and press Enter. php artisan make:model Role -m
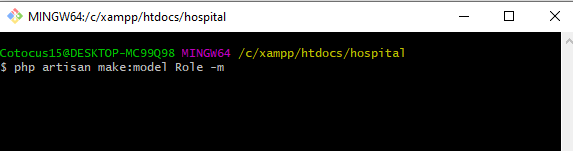
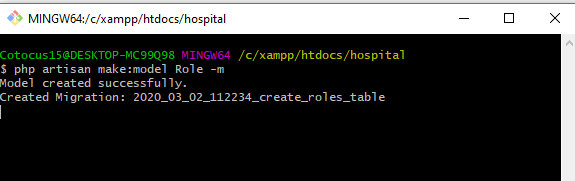
Step:18 Customise roles table(database/migration/create_roles_table.php)
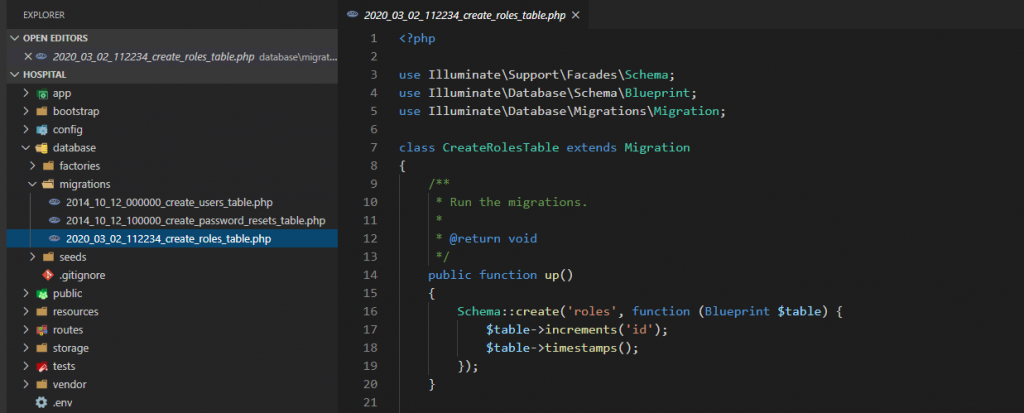
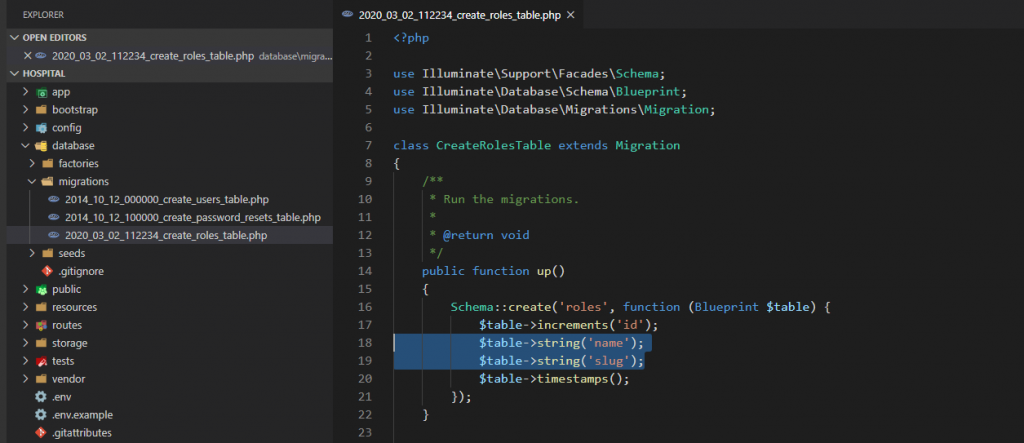
Step:-19 In Role Model create users() Function put this code in Role model
public function users()
{
return $this->hasMany(‘App\User’);
}
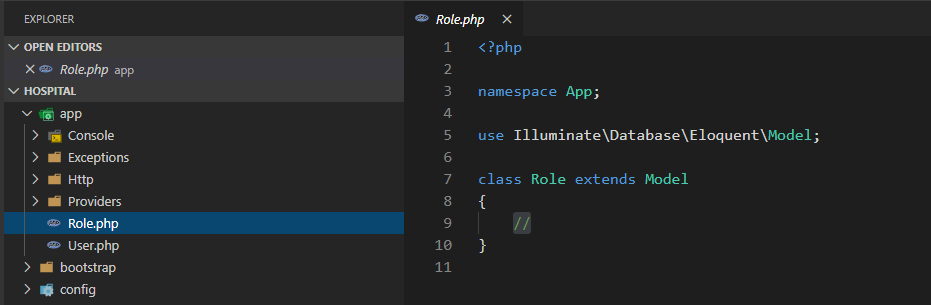
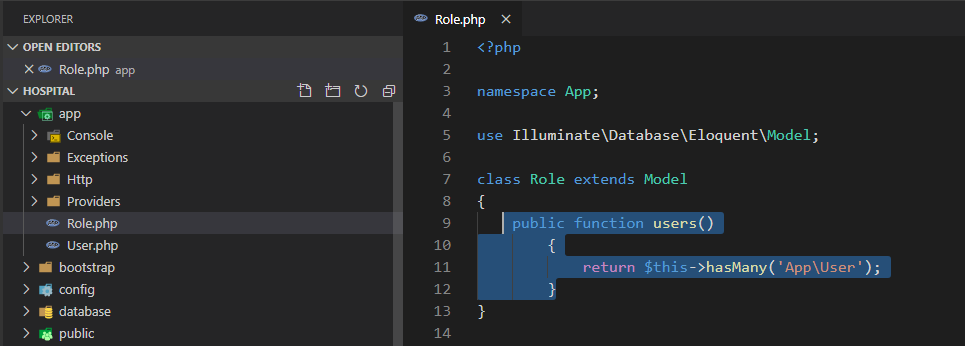
Step:-20 In User Model create role() Function for relationship and put this code in User model
public function roles()
{
return $this->belongsTo(‘App\Role’);
}
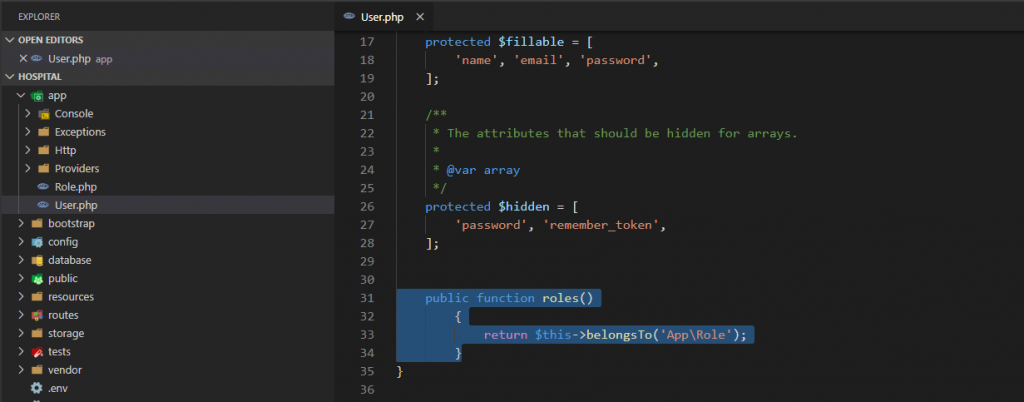
Step:21 Make UsersTableSeeder file for insert data in users table through migration and run this code in your git bash cammand. php artisan make:seed UsersTableSeeder
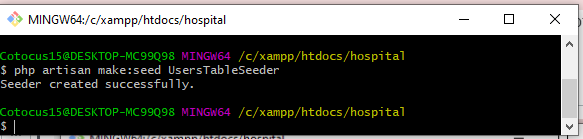
Step:22– Make RolesTableSeeder file for insert data in roles table through migration and run this code in your git bash cammand. php artisan make:seed RoleTableSeeder
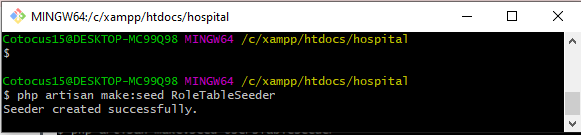
Step:23 Edit RoleTableSeeder.php(database/seeds/RoleTableSeeder.php) file for insert data in roles table put this code in the top use Illuminate\Support\Facades\DB;
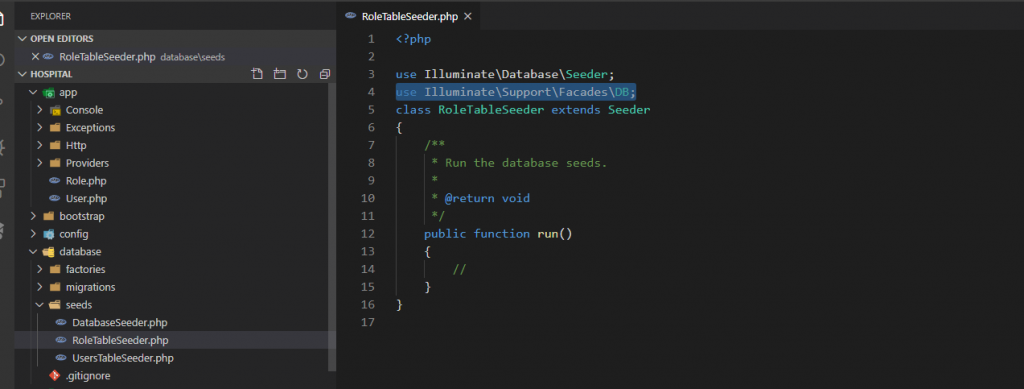
Step:24 put this code RoleTableSeeder.php(database/seeds/RoleTableSeeder.php
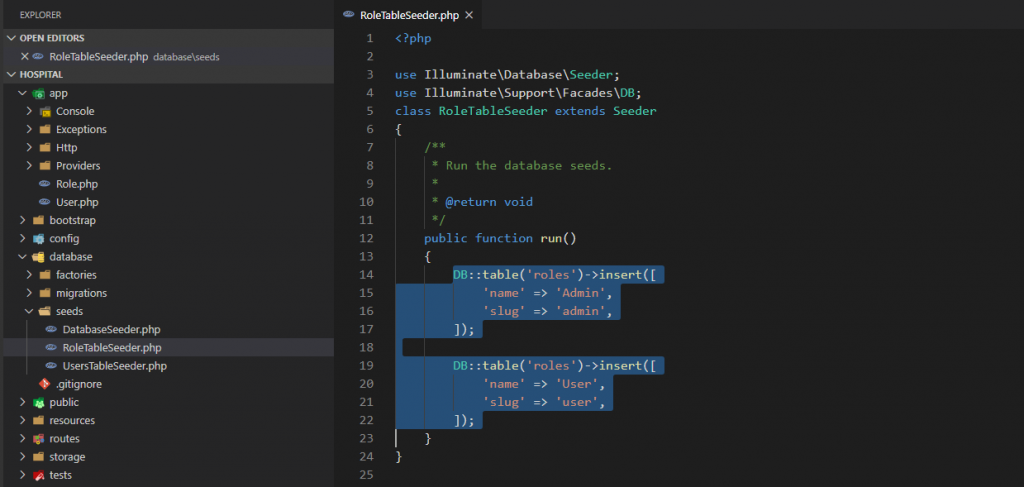
Step:25 Edit UserTableSeeder.php(database/seeds/UserTableSeeder.php) file for insert data in users table and put this code in top. use Illuminate\Support\Facades\DB;
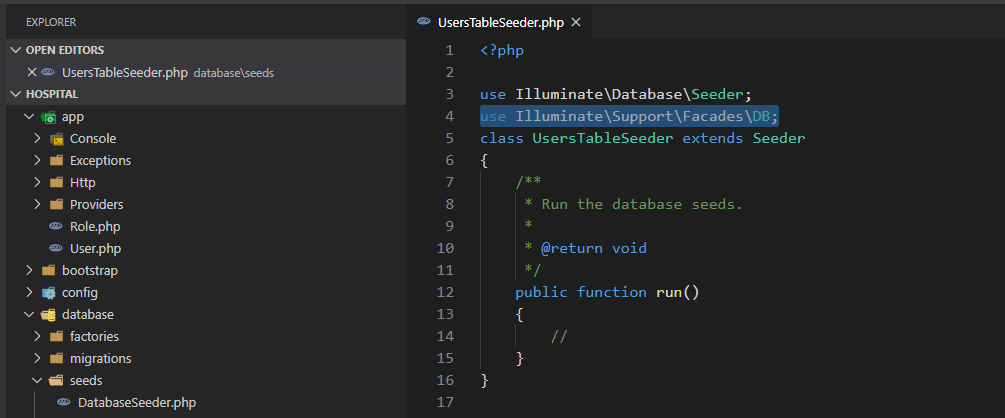
Step:26 Insert data in users table
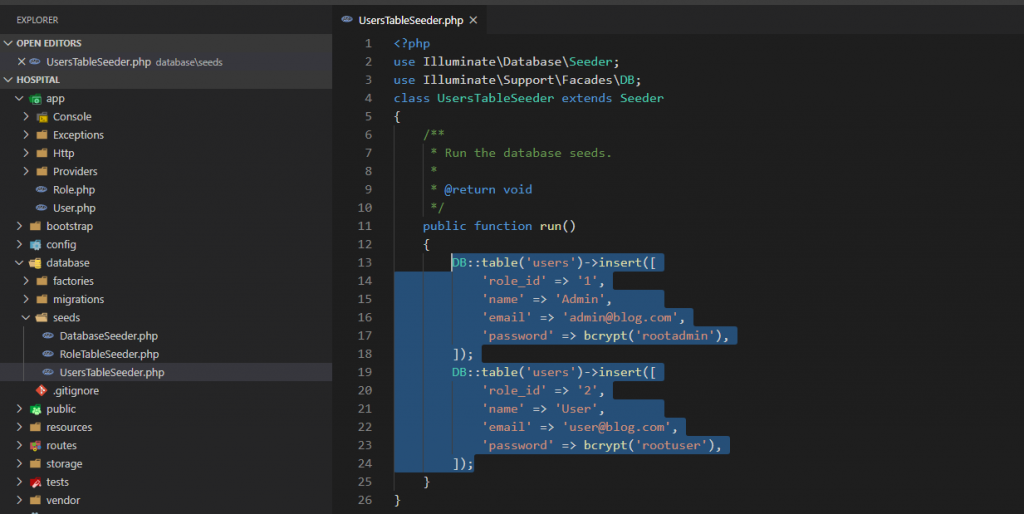
Step:26 Migration of tables in database php artisan migrate
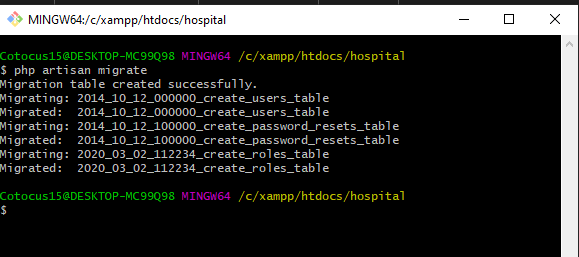
Step:27 Define UsersTableSeeder and RoleTableSeeder Class on DatabaseSeeder.php file
database/seeds/DatabaseSeeder.php and put this code
public function run()
{
$this->call(UsersTableSeeder::class);
$this->call(RoleTableSeeder::class);
}
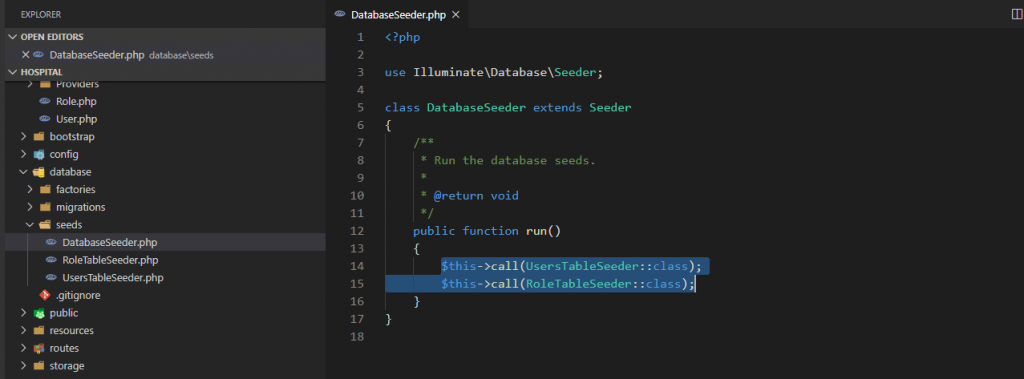
Step:28 php artisan db:seed (Insert data in tables)
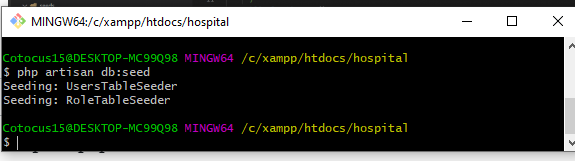
Step:29 Make AdminMiddleware for Admin Authentication Work. php artisan make:middleware AdminMiddleware
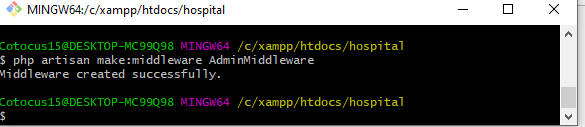
Step:30 Make AuthorMiddleware for User Authentication Work. php artisan make:middleware UserMiddleware
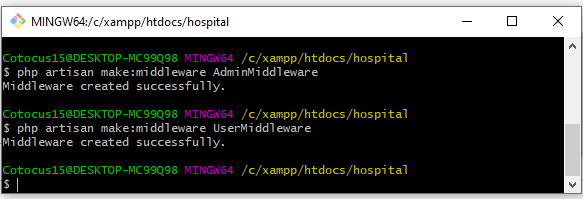
Step:31 Implement Condition for Admin Login in AdminMiddleware (app\Http\Middleware\AdminMiddleware.php) put this code in the top Use Auth;
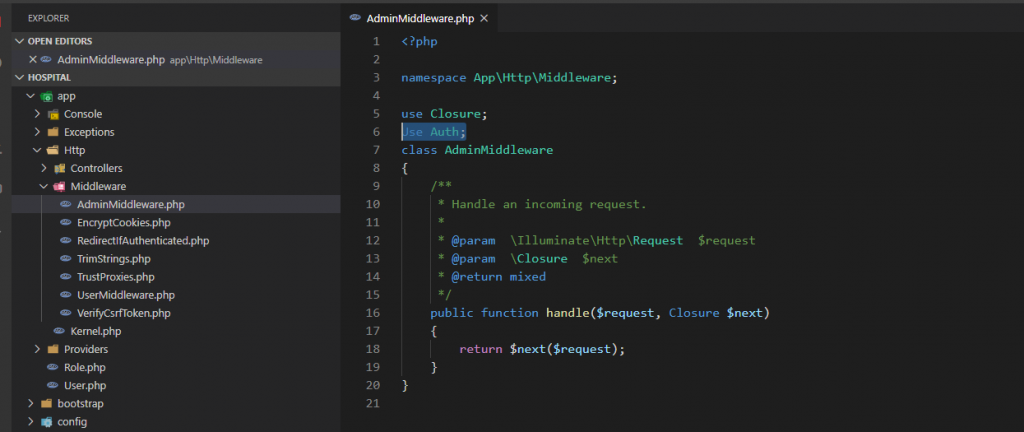
Step:32
public function handle($request, Closure $next)
{
if(Auth::check() && Auth::user()->role->id == 1){
return $next($request);
}
else {
return redirect()->route('login');
}
}
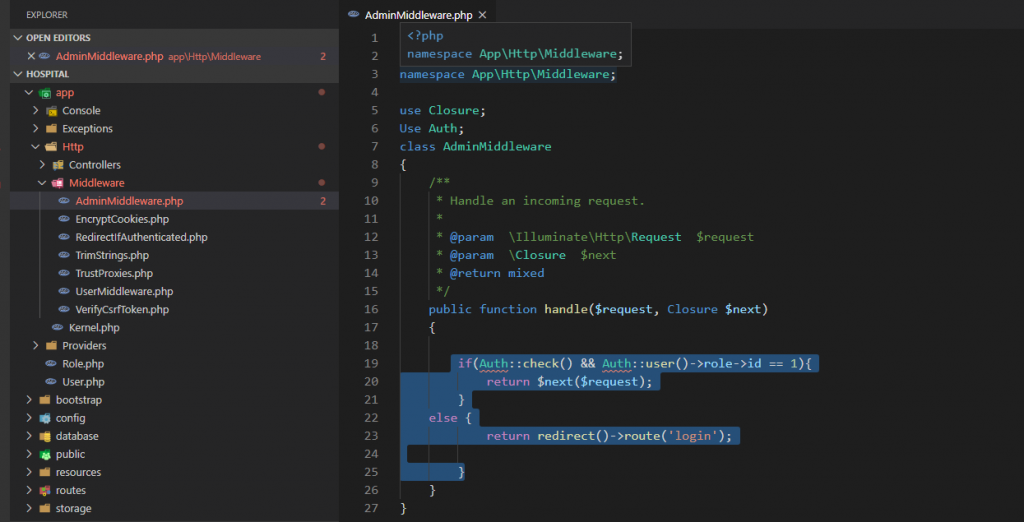
Step:33 Implement Condition for User Login in UserMiddleware (app\Http\Middleware\UserMiddleware.php) put this code in the top Use Auth;
public function handle($request, Closure $next)
{
if(Auth::check() && Auth::user()->role->id == 2){
return $next($request);
}
else {
return redirect()->route('login');
}
}
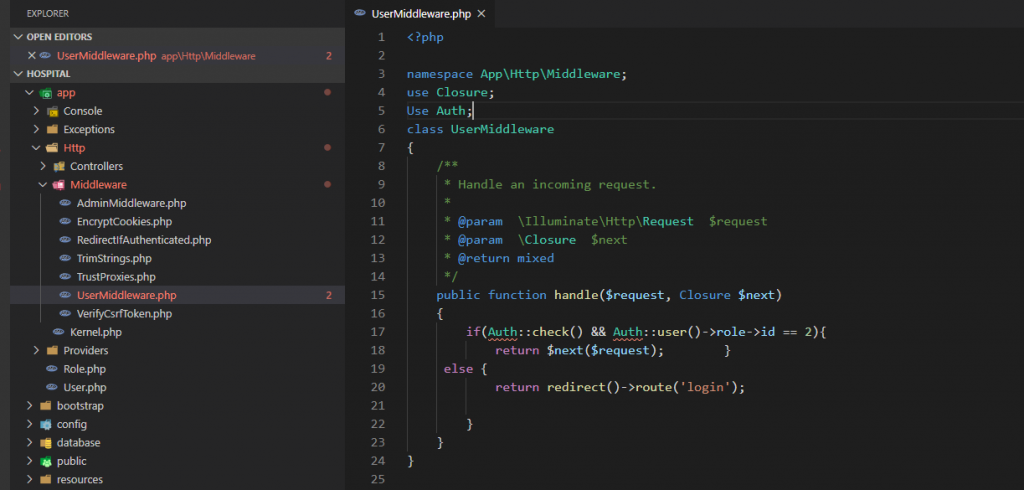
Step:34 Implement Condition for User Login in RedirectIfAuthenticated (app\Http\Middleware\RedirectIfAuthenticated.php
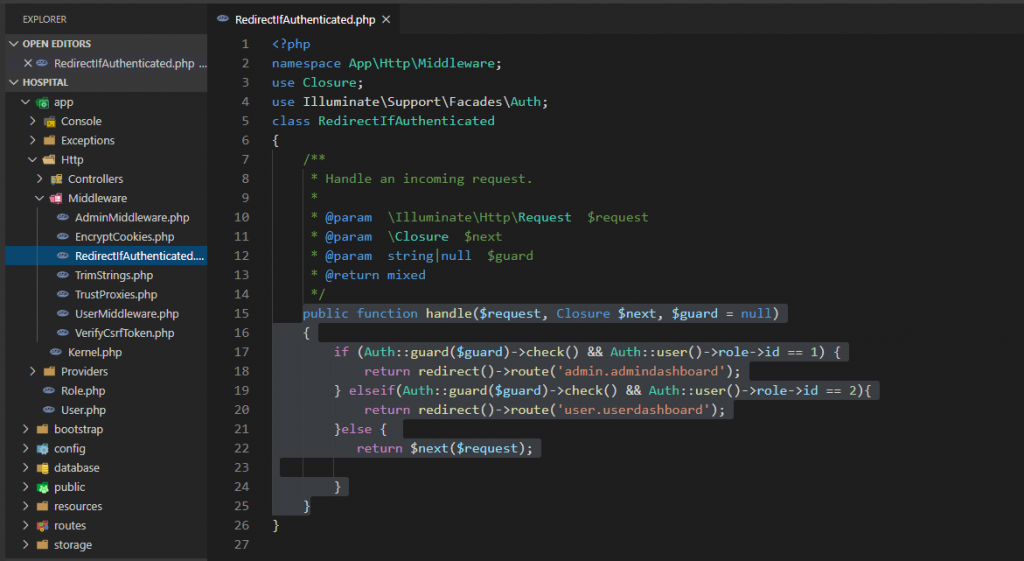
Step:35 Implement Condition for Login in LoginController (app\Http\Controllers\Auth\LoginController.php)
protected $redirectTo;
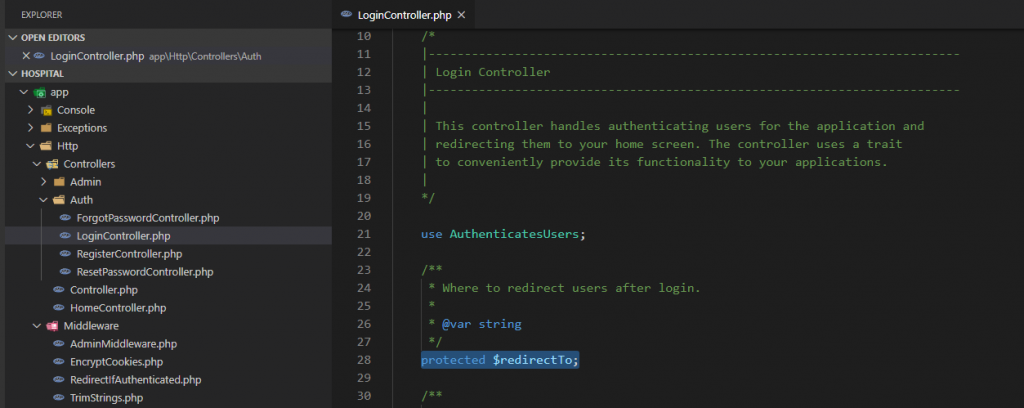
Step:36 Put this code in LoginController.php
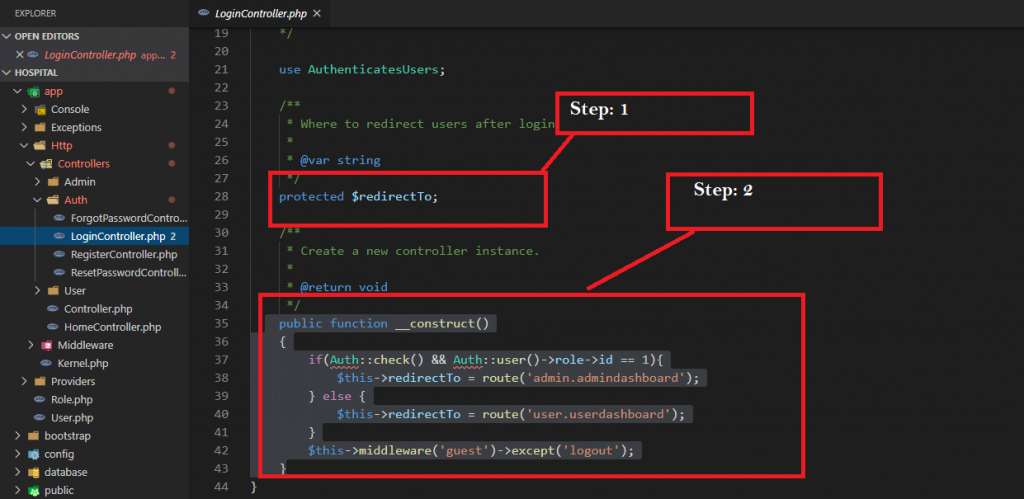
Step:37 Implement Condition for Login in ResetPasswordController (app\Http\Controllers\Auth\ResetPasswordController.php)
protected $redirectTo;
Step:38
public function __construct()
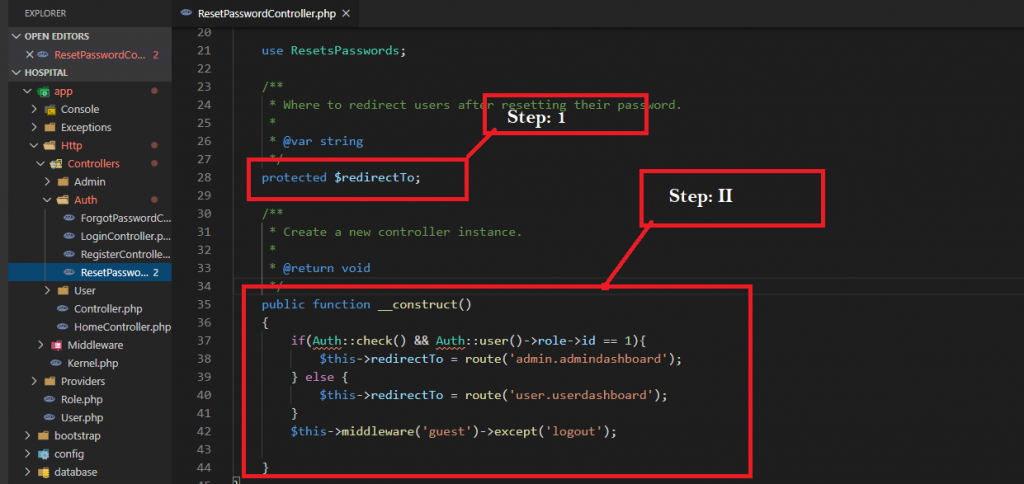
Step:39 Define AdminMiddleware on routeMiddleware(path- app/Http/Kernal.php) use this code in the top use App\Http\Middleware\AdminMiddleware; App\Http\Middleware\UserMiddleware;
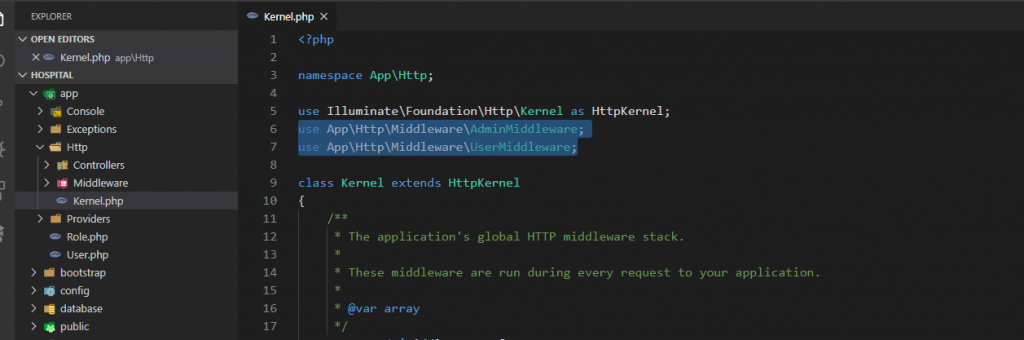
Step:40 protected $routeMiddleware = [
‘admin’ => AdminMiddleware::class,
];
protected $routeMiddleware = [
‘user’ => UserMiddleware::class,
];
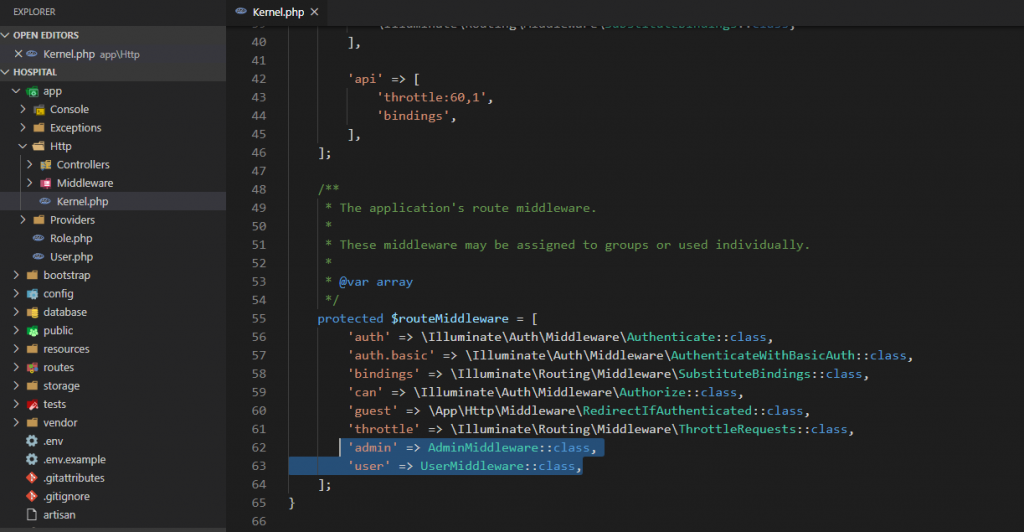
Step:41 Set middleware and route on web.php(Path routes/web.php)
a.Set Admin middleware and route
Step:42 .b.Set User middleware and route
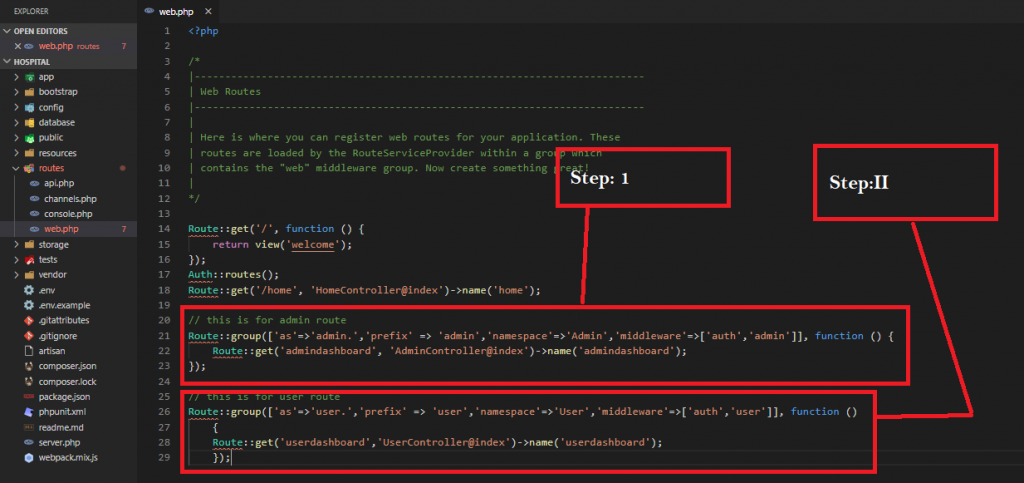
Step:43 you have create admin dashboard and user dashboard Make dashboard.blade.php file on resources/views/admin/admindashboard.blade.php Make dashboard.blade.php file on resources/views/user/userdashboard.blade.php
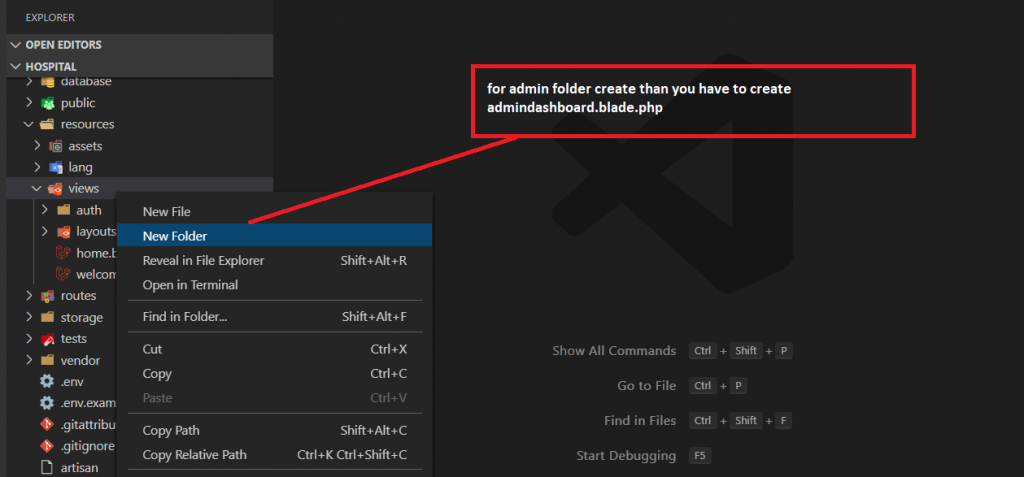
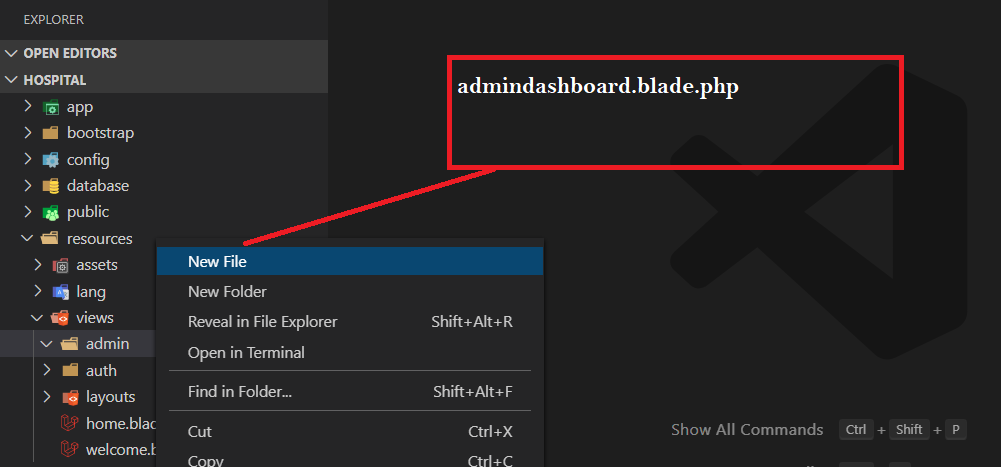
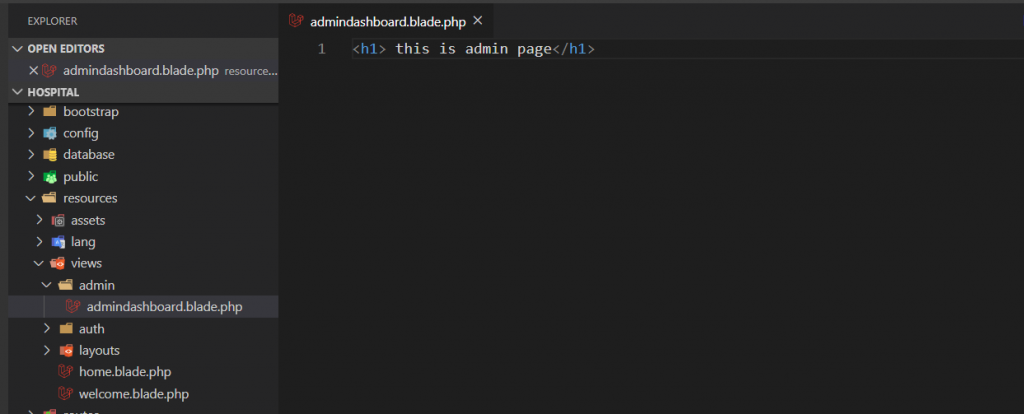
Step:44 . same you have to make for user
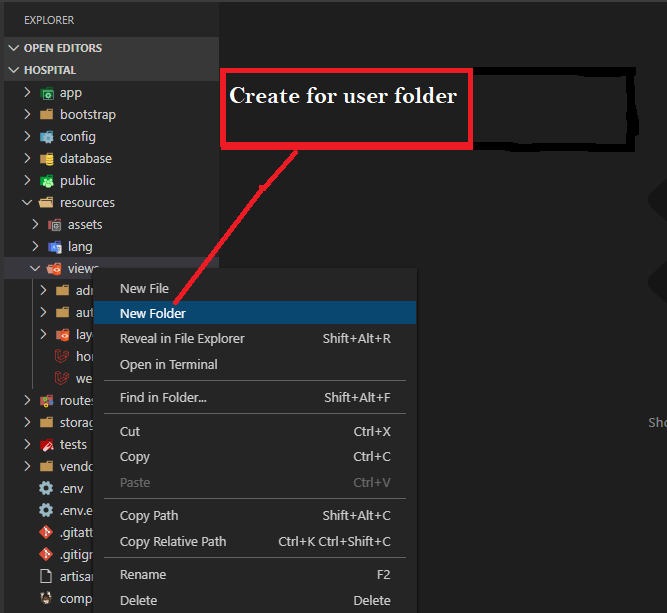
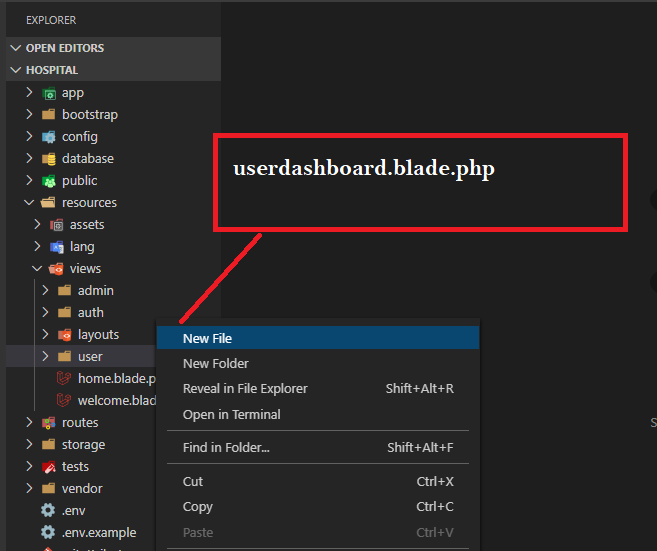
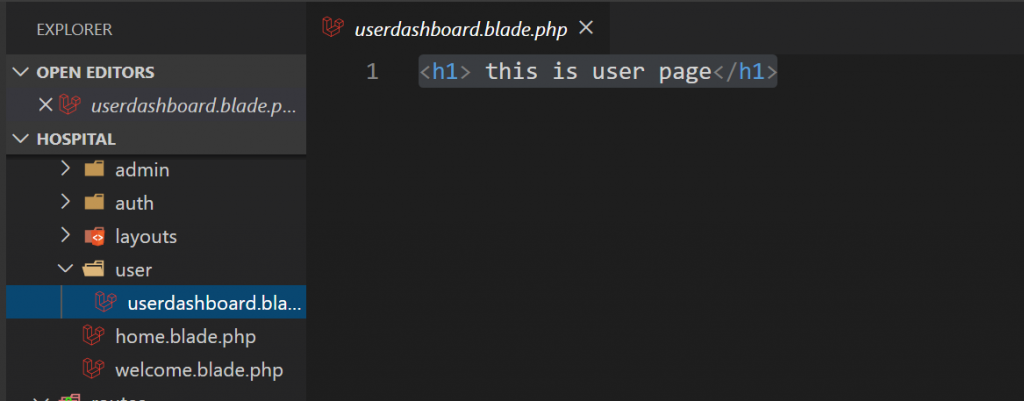
And finally you have run this code on your git base here cammand php artisan serve
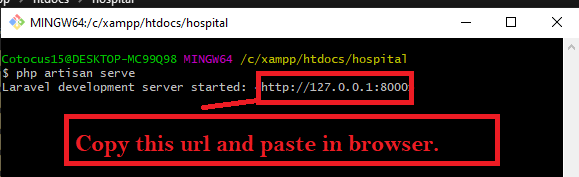