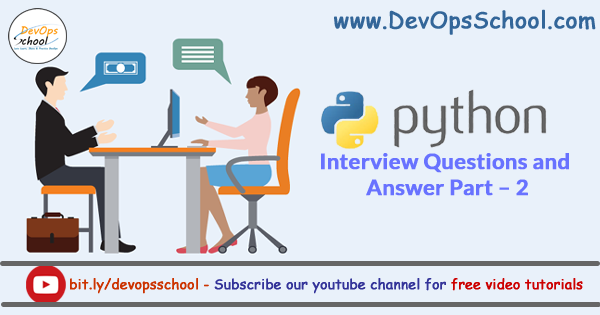
Which argument would you use in a Python function to obtain a dictionary of all the named arguments passed?
- Keyword Arguments (**kwargs) (Ans)
- *args
- The NoneType argument
- Regular function arguments
To create a virtual environment, you use the following command:
- virtualenv-create
- virtualenvironment
- virtualenv (Ans)
- virtual-env
A nested function can access its parent’s function variable and use that data within it.
- True (Ans)
- False
To install a Python package using pip, you use:
- pip install (Ans)
- pip get
- pip add
- pip uninstall
Flask is used:
- as a Python utility library.
- as a Web microframework. (Ans)
- as a general purpose Python library.
- Similarly to how pip is used.
One of the Python packages used for creating executable files out of Python code is called:
- PyInstaller (Ans)
- PyMaker
- Flask
- Django
Python requires us to declare types such as int, string, and char[] before declaring a variable
- True
- False (Ans)
In order to group multiple objects in one variable, you use:
- array
- list (Ans)
- collection
- dictionary
When creating two instances of the same class, those two instances will share all the data automatically
- True
- False (Ans)
In Python, a class can inherit from exactly this number of parent classes
- 1
- Many (Ans)
- None
- 3
To help debug your application, you can set something called a:
- Stop point
- Pause
- Resume point
- Breakpoint (Ans)
The constructor method in Python classes looks similar to this
- def initialize(self):
- def constructor():
- def init(self): (Ans)
- There is no constructor method in Python classes
What is the result of the following code?
names = [“Jonathan”, “Bo”, “Layla”, “Kristin”]
for name in names:
if name == “Bo”:
print(“Found the instructor!”)
break
print(“{0} is not the instructor”.format(name))
- It will print “Jonathan is not the instructor”, after which it will print “Found the instructor” and then stop the for loop. (Ans)
- It will print “Jonathan is not the instructor”, after which it will print “Found the instructor” and continue to print the rest of the list.
- It will print “Kristin is not the instructor”, after which it will print “Found the instructor” and stop the for loop.
- It will print all the names from the list.
Which one of the following is a valid dictionary?
- All of them (Ans)
- { “name”: “Mark” }
- { “name”: “Mark”, “student_Id”: 16135 }
- { “name”: “Bo”, “student_id”: 42671, instructor: True }
What keyword do we use in a function when we want to return the data to the function caller?
- respond
- give_back
- print()
- reply
- return (Ans)
Class attributes do share their value across different instances of that class.
- True, but only if the class attribute name has a dash.
- False
- False, except when the class attribute name starts with a capital letter.
- True (Ans)
Which function in unittest will run all of your tests?
- unittest.execute()
- unittest.run()
- unittest.main() (Ans)
- unittest.generate_report()
What is one way to create a list from another sequence?
- a with-statement
- the list constructor (Ans)
- the split() method
- the make_string() function
What does PDB’s where command do?
- Prints the current call stack (Ans)
- Shows where an attribute was initially defined
- Prints directory containing the current source file
- Prints the current timezone
When converting a float to an integer with the int() constructor in which direction is rounding?
- Towards zero (Ans)
- Away from zero
- Towards the nearest integer
- Towards positive infinity
E. Towards negative infinity
What is one way in which exceptions are superior to error codes?
- They are faster.
- They cannot be easily ignored. (Ans)
- They aren’t part of a function’s API.
- Python doesn’t support error codes.
What does Python’s id() function return?
- A unique identifier for an object (Ans)
- The version of the Python runtime
- The order in which an object was created
- The name of an object
Are functions also objects in Python?
- Yes (Ans)
- Only if you declare them so explicitly
- Module-level functions are, but not instance methods
- No

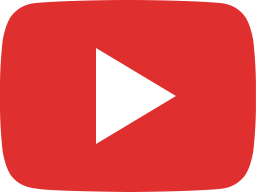
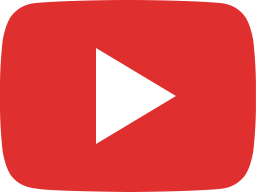

- Apache Lucene Query Example - April 8, 2024
- Google Cloud: Step by Step Tutorials for setting up Multi-cluster Ingress (MCI) - April 7, 2024
- What is Multi-cluster Ingress (MCI) - April 7, 2024