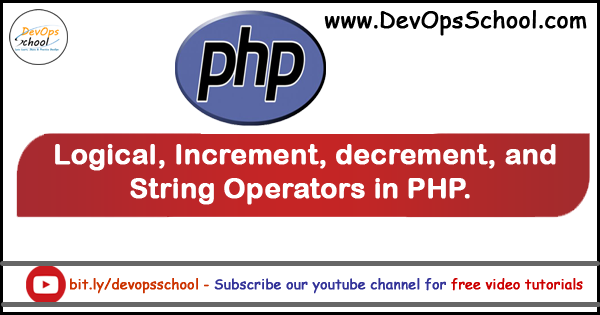
Logical Operators
1. Logical AND(&&/and).
There are two Logical AND Operators, First && and 2nd and where First Operator has higher precedence(means higher priority) than 2nd. It Compares two expressions or operators and gives output in 4 cases. See the Below image.
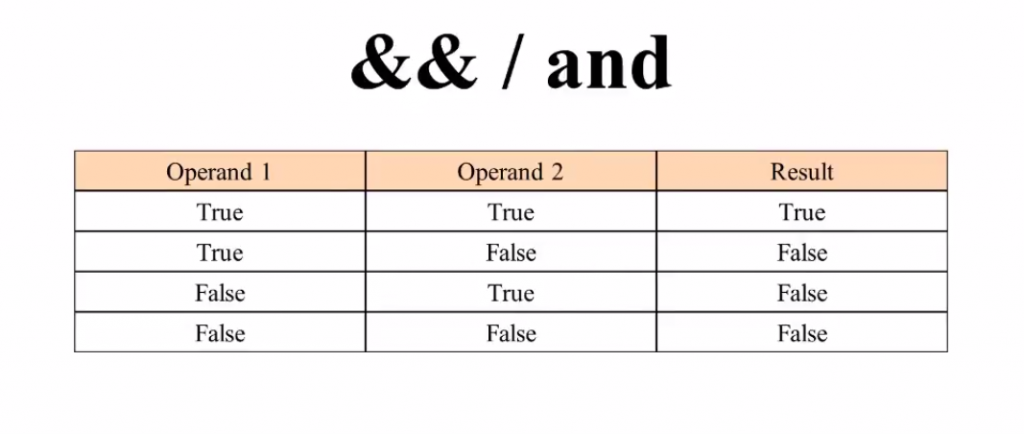
Case 1. When Both the expressions are true then it returns True.
For Example :- (5>2)&&(5<8)
In the above Example, It returns True because both the expressions are true.
Case 2. When 1st expression is true and 2nd is false then it returns False.
For Example :- (3<5)&&(5<2)
In the above Example, 1st expression is true but 2nd expression is false then it returns False.
Case 3. When 1st expression is false and 2nd is true then it returns False.
For Example :- (5<2)&&(3<5)
In the above Example, 1st expression is false but 2nd expression is true then it returns False.
Case 4. When Both the Expression is false then it also returns False.
For Example :- (6<2)&&(3>5)
In the above Example, Both expressions are false then it returns False.
2. Logical OR(||/or).
There are Two Logical OR, First || and 2nd or where First Operator has higher precedence(means higher priority) than 2nd. It Compares two expressions or operators and gives output in 4 cases. See the Below image.
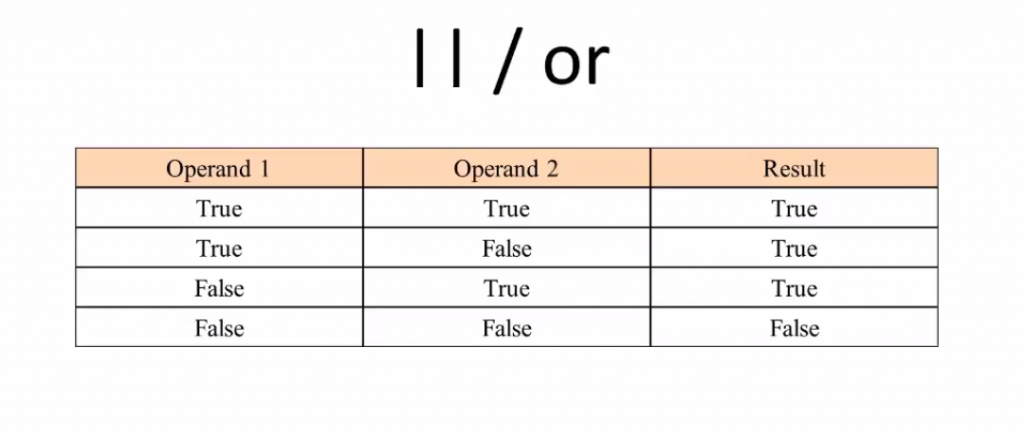
Case 1. When Both the expressions are true then it returns True.
For Example :- (5>2)||(5<8)
In the above Example, It returns True because both the expressions are true.
Case 2. When 1st expression is true and 2nd is false then it returns True.
For Example :- (3<5)||(5<2)
In the above Example, 1st expression is true but 2nd expression is false then it returns True.
Case 3. When 1st expression is false and 2nd is true then it returns True.
For Example :- (5<2)||(3<5)
In the above Example, 1st expression is false but 2nd expression is true then it returns True.
Case 4. When Both the Expression is false then it also returns False. For
Example :- (6<2)||(3>5)
In the above Example, Both expressions are false then it returns False.
3. Logical NOT(!).
It returns the reverse value of expression means when the expression is true then it returns False, and when the expression is false then it returns True.
For Example :- !(3<2)
In the above example, the expression is false but it returns True.
4. Exclusive OR(xor).
When both the expression are Identical (means both are true or false) then it returns False and when any one of them is true then it returns True. See the below image.
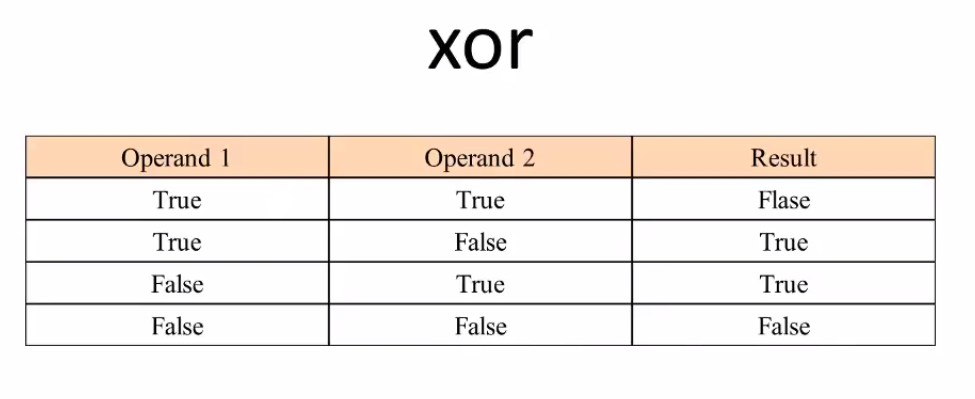
Case 1. When Both the expressions are true or false then it returns False.
For Example :- (5>2)xor(5<8) or (6<2)||(3>5)
In the above example, both the expressions in the first example is true and both the expressions in the second example is false so both the examples return False.
Case 2. When 1st expression is true and 2nd is false then it returns True.
For Example :- (3<5)xor(5<2)
In the above Example, 1st expression is true but 2nd expression is false then it returns True.
Case 3. When 1st expression is false and 2nd is true then it returns True.
For Example :- (5<2)xor(3<5)
In the above Example, 1st expression is false but 2nd expression is true then it returns True.
Increment and Decrement Operators.
Increment Operator:- It increases the value of the variable by 1. It is of two types:
1. Pre-increment:- It first increases the value of variable, then uses the new variable value.
For Example:- ++$a
<?php
$a=40;
echo ++$a;
?>
Output :- 41
2. Post-increment:- First uses the current value of the variable, then increases the value of variable by 1.
For Example:- $a++
<?php
$a=25;
echo $a++.”<br>”;
echo $a;
?>
Output:- 25
26
Decrement Operator:- It decreases the value of the variable by 1. It is of two types:
1. Pre-decrement:- It first decreases the value of the variable, then uses the new variable value.
For Example:- – – $a
<?php
$a=40;
echo – – $a;
?>
Output :- 39
2. Post-decrement:- First uses the current value of the variable, then decreases the value of the variable by 1.
For Example:- $a- –
<?php
$a=25;
echo $a – -.”<br>”;
echo $a;
?>
Output:- 25
24

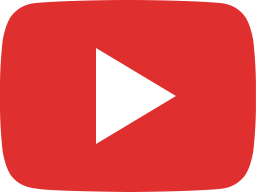
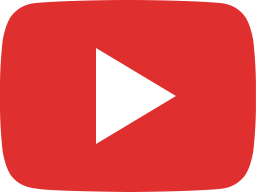

- What is On-Page Optimization and Off-page Optimization - March 14, 2024
- [SOLVED] Flutter : PlatformException(sign_in_failed, com.google.android.gms.common.api.ApiException: 10: , null, null) - December 7, 2021
- [Solved] Flutter : Error: The getter ‘subhead’ isn’t defined for the class ‘TextTheme’ from package:flutter/src/material/text_theme.dart’ – searchable_dropdown - December 6, 2021