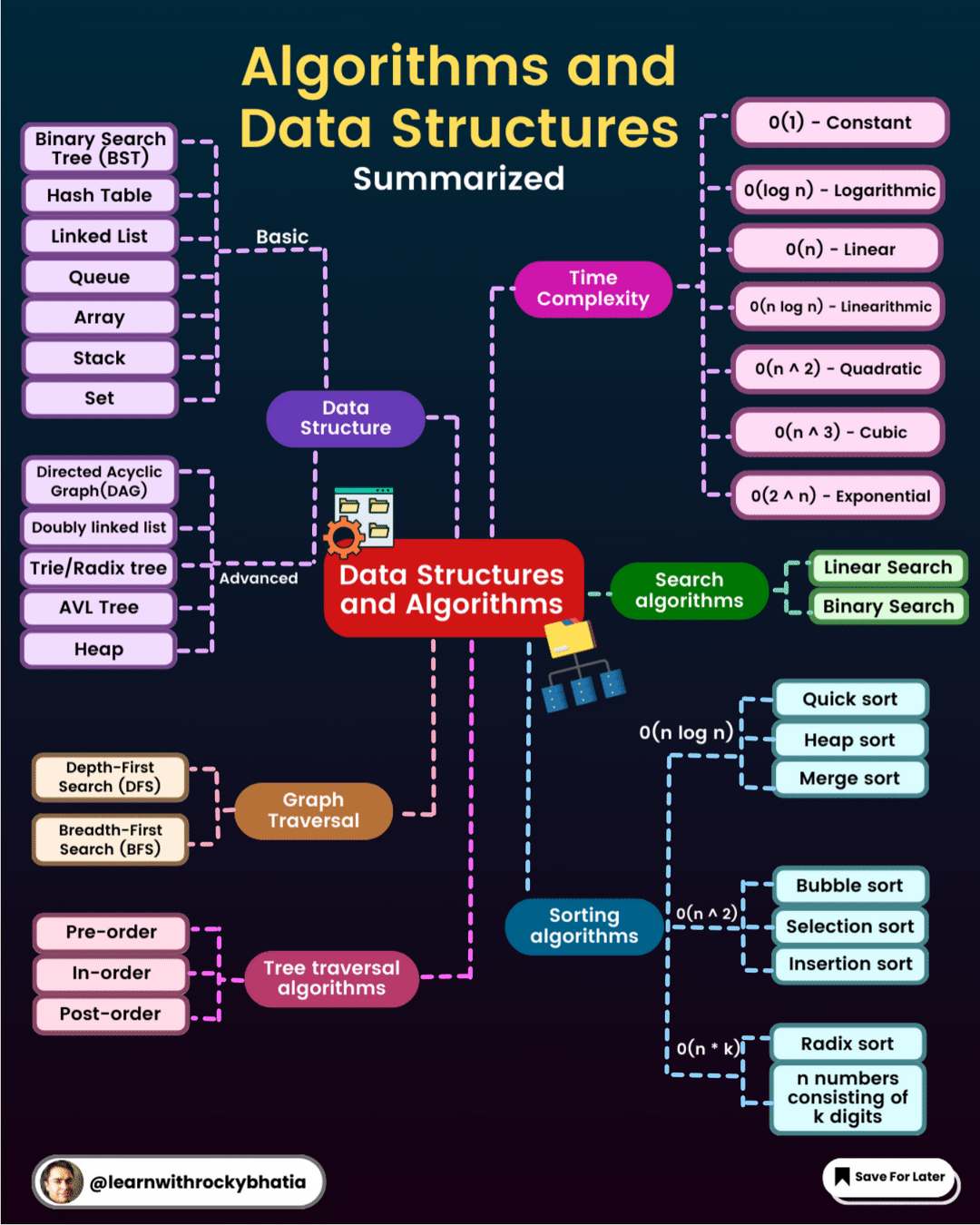
Algorithms and data structures are two of the most important concepts in computer science. Algorithms are step-by-step procedures for solving problems, while data structures are ways of organizing data so that it can be used efficiently.
Algorithms
Algorithms are used in all sorts of computer applications, from simple tasks like searching for a word in a text file to complex tasks like rendering 3D graphics. Some common examples of algorithms include:
- Sorting algorithms: These algorithms are used to sort a list of items in a specific order, such as alphabetical order or numerical order.
- Searching algorithms: These algorithms are used to find a specific item in a list or database.
- Graph algorithms: These algorithms are used to traverse and analyze graphs, which are data structures that represent networks of nodes and edges.
- String algorithms: These algorithms are used to manipulate strings of text, such as searching for patterns or finding the longest common subsequence.
Data structures
Data structures are used to store and organize data in a way that makes it easy to access and manipulate. Some common examples of data structures include:
- Arrays: Arrays are fixed-size lists of items that can be accessed by their index.
- Linked lists: Linked lists are dynamic lists of items that are connected by pointers.
- Stacks: Stacks are data structures that follow a last-in-first-out (LIFO) principle.
- Queues: Queues are data structures that follow a first-in-first-out (FIFO) principle.
- Trees: Trees are hierarchical data structures that can be used to represent data such as file systems or family trees.
- Graphs: Graphs are data structures that represent networks of nodes and edges.
Relationship between algorithms and data structures
Algorithms and data structures are closely related. The choice of data structure can have a significant impact on the performance of an algorithm. For example, if you are searching for an item in a sorted array, you can use a binary search algorithm, which is much more efficient than a linear search algorithm.
Conversely, the choice of algorithm can also affect the design of a data structure. For example, if you need to implement a stack, you can use a linked list or an array. However, if you need to implement a queue, you cannot use an array because it is difficult to implement efficient enqueue and dequeue operations on an array.
Importance of algorithms and data structures
Algorithms and data structures are essential for writing efficient and effective computer programs. By understanding how algorithms and data structures work, you can write programs that are faster, use less memory, and are easier to maintain.
Algorithms and data structures are also important for interviews for software engineering positions. Many companies ask questions about algorithms and data structures in their interviews to assess the candidate’s programming skills and problem-solving ability.
Algorithms and data structures are fundamental concepts in computer science that provide solutions to common computational problems and efficient ways to store and manipulate data.
Data Structures: Data structures are a way of organizing and storing data so that it can be accessed and modified efficiently. Common data structures include:
- Arrays: A contiguous memory allocation that stores elements of the same type. Access is O(1), but insertions and deletions can be O(n) if done in the middle.
- Linked Lists: Elements (nodes) are connected using pointers. There are variations like singly linked lists, doubly linked lists, and circular linked lists.
- Stacks: A last-in, first-out (LIFO) data structure. Common operations: push, pop, peek.
- Queues: A first-in, first-out (FIFO) data structure. Variations include priority queues and circular queues.
- Trees: Hierarchical data structure with a root and nodes. Types include binary trees, binary search trees, AVL trees, and more.
- Graphs: Nodes connected by edges. Can be directed, undirected, weighted, or unweighted.
- Hash Tables: Uses a hash function to map keys to indices in an array. Good for quick lookups.
- Heaps: A specialized tree-based data structure that satisfies the heap property. Commonly used in algorithms like heapsort.
Algorithms: Algorithms are a set of steps to accomplish a task. Some fundamental algorithms are:
- Sorting Algorithms: Bubble sort, insertion sort, selection sort, quicksort, mergesort, heapsort, etc.
- Searching Algorithms: Linear search, binary search.
- Graph Algorithms: Depth-first search (DFS), breadth-first search (BFS), Dijkstra’s shortest path, Floyd-Warshall, minimum spanning tree (Prim’s, Kruskal’s), etc.
- Tree Algorithms: Tree traversal (in-order, pre-order, post-order), tree balancing, etc.
- Dynamic Programming: Techniques to solve problems by breaking them down into smaller subproblems. Examples: Fibonacci series, longest common subsequence, coin change, etc.
- Divide and Conquer: Breaking down a problem into smaller, solvable subproblems. Mergesort and quicksort are examples.
- Greedy Algorithms: Making the locally optimal choice at each step in the hope of finding the global optimum. Examples: Huffman coding, activity selection.
- Backtracking: A trial and error-based method. Used for problems like the N-queens problem, the knapsack problem, etc.
Importance: Understanding data structures and algorithms is essential because they:
- Provide efficient solutions to common problems.
- Are frequently used in software development and system design.
- Form the basis for many advanced topics and specialized areas in computer science.
- What is Mobile Virtual Network Operator? - April 18, 2024
- What is Solr? - April 17, 2024
- Difference between UBUNTU and UBUNTU PRO - April 17, 2024