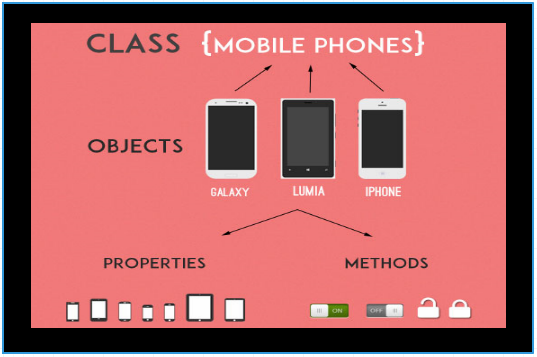
The points of object-oriented programming in PHP are discussed in this article. We will begin with a class and object introduction and examine a few advanced concepts of such How, Why, What and some examples of Objects and Classes. But first let me give you a brief explanation of what is Object-oriented programming (OOP)?
What is Object-oriented programming (OOP)
Object-oriented programming consists of combining a set of variables (properties) and functions (methods), which are referred to as an object. These things are arranged into classes in which individual items can be combined. OOP can enable you to consider the objects and the many activities in connection with the objects in a program’s code.
for more details you can go on my previous article Read More. [Object-oriented programming (OOP) Concept Simplified!]
What Is a PHP Class?
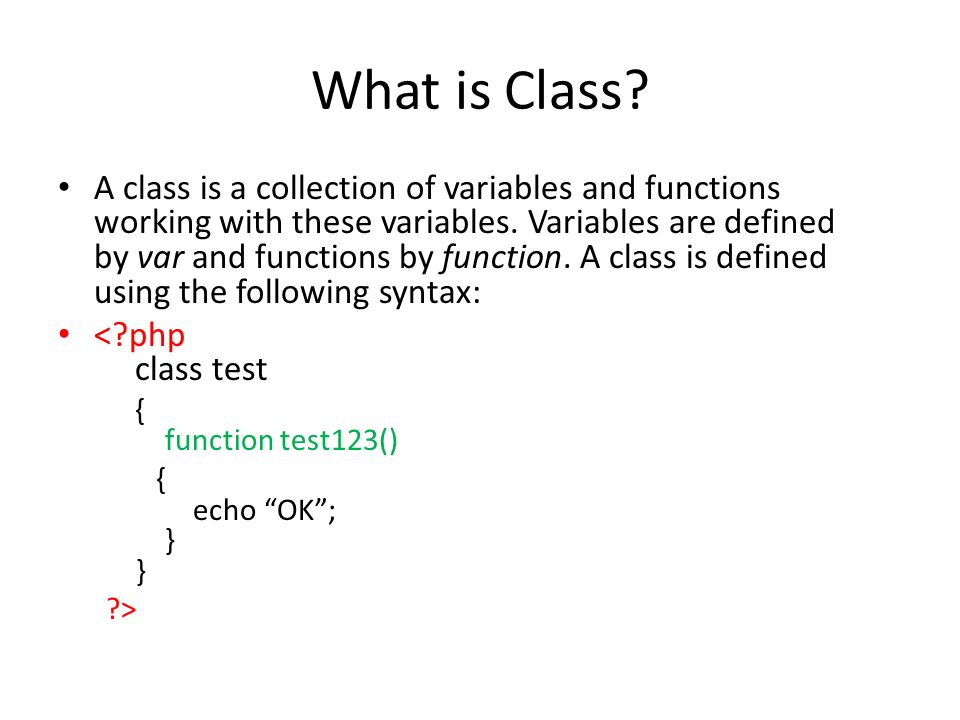
A class is an abstract plan for creating more particular, specialised items. Classes often include large categories, such as (Car
),(Fruits
) and (Dog
) with properties in common. These classes specify what characteristics an instance like (colour
) has for a certain object, but not the value of the attributes. The data type is determined by the programmer and contains both local and local data functions. A class may be a template for several examples of the same object type (or class).
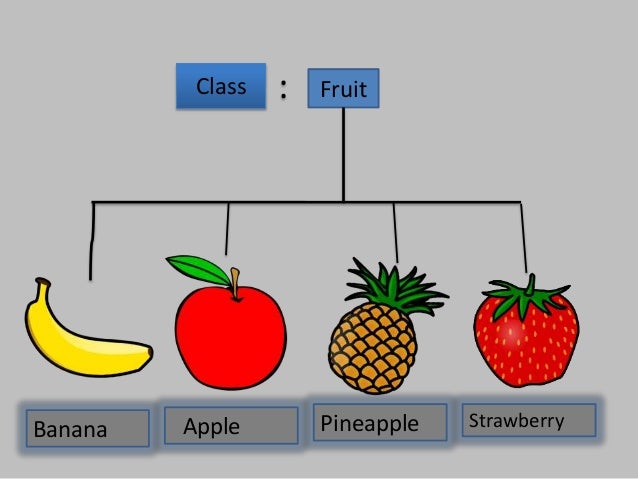
Here is the explanation of the code are in following points:-
– The specific form class, then the name of the class you are interested in defining.
– A collection of braces with a number of variable statements and function definitions.
– Variable declarations begin with the special (var
) form which is followed by the standard $ variable name;
they may also be given a constant value for first assignment.
– The function definitions are similar to standalone PHP features but are local to the class and are used to set and access data on the object.
Here are some of the Examples:-
Example 1:-
Example 2:-
Example 3:-
Some Explanation about the Examples:-
- The keyword {
Class Employee
} in the first line defines the class {Employee
}. We then report the properties, the builder and the other methods of the classes. - The Keyword
public
indicates that this property or method is accessible from anywhere. - The Keyword
Private
indicates that property or method is accessible only from within the class that defines it.
Why to write a PHP Class?
I found that as a Beginner, PHP Developers have to write one or more PHP files/scripts functions. Indeed, I have also found several (beneficial) projects developed using a few extremely large PHP files that include all the code in the form of several features. It’s OK to do this when learning PHP. But, This may not be the preferred technique for web applications to be produced. Below are some of the downsides of PHP scripts that contain only the functionalities are explained in following points:-
- Low Maintainability: These functional files are hard to keep/manage (change). It’s almost difficult to think about developing unit tests. There are other functions that may be reused in addition to low testing. Because of the way they are written, however, the files are also less reusable. The code replication spreads, which further affects the maintenance of the code since updating the functionality in numerous locations requires changes.
- Low Usability: The Functions and files are very hard to learn and Understand. Think when you doesn’t understand the things so how you can implement on you projects. This is the main reason of the downsides of PHP scripts.
To deal with some of the aforementioned issues you should study how to write PHP Through object-oriented methods, e.g. write code in one or more classes. Writing PHP code using classes allow you to isolate similar look functions in a class and utilize the class elsewhere in the code (different PHP scripts). In truth, the SOLID concept might simply be followed by PHP and the code organized in a well manner. This technique promotes high maintainability and makes code accessible and comprehensible (high testability, high cohesion, high reusability etc.).
How to create a Class in PHP?
To construct a class, we gather in one location the code which covers a certain issue. For instance, all the code handling blog users may be grouped into a single class, all of the code involved in publishing articles in a second class blog and all of the code that is used for commenting in a third class. We may see class methods as functions that perform certain object-related tasks. In the majority of the situations, the object’s attributes and related activities are accessed and manipulated.
The keyword for class definition is used in PHP. Below are the guidelines for PHP class creation.
- The class name should start with a letter
- The class name cannot be a PHP reserved word
- The class name cannot contain spaces
Creating a class for representing Animals.
Let’s start with discovering the features that are common to all animals.
- All animals belong to a family such as a herbivore, carnival, etc.
- All animals eat food
The diagram below shows the diagram for the animal
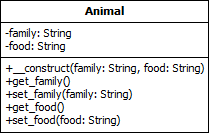
Following are the Explanation of the Code:-
- “private $family, $food” signifies that variables cannot be accessed outside the class directly (Encapsulation).
- The php function method is “public function __construct($family…).” Whenever a class instance is created, that function is invoked. We set up the family and meals in this scenario.
- ‘public function get..()’ represents the mechanism used to retrieve family or food value (Encapsulation)
- “public function set..()” represents the technique used to set family value or food value is (Encapsulation)
What Is an Object in PHP?
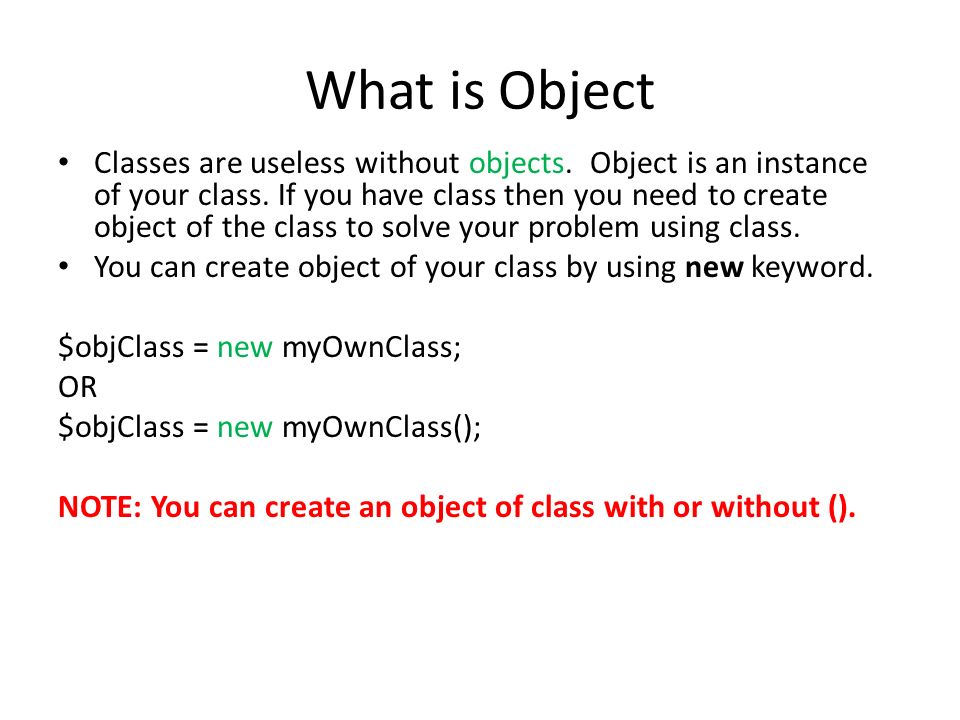
The fundamental structure of a class in PHP was explained in the previous section. Now, you need to use it, and the final result is an object, when you wish to utilize a class. We may consider of a class as a blueprint, whereas an object is something you can really deal with.
A separate instance of a class-defined data structure. One time, you define a class and then produce a lot of objects. Also known as instance are objects. Without objects, classes are nothing! Multiple objects can be created in one class. Each object contains all the class declared attributes and methods, however they have different values for properties.
Following are the Explanation of Given Code:-
- If you wish to create any class object along with its class name, you need to use (
new
) the keyword, and a new object instance of that class will return. - In our situation, one parameters are needed in the (
Fruit
) class categories, and so we supplied the parameters when we built the object ($apple
) and ($banana
) - Next, we called (
$apple
) and ($banana
) class methods for printing information populated during the construction of objects. Naturally, many objects of the same class can be created.
Why we Use Objects in PHP?
While all the functions and variables in the proceeding style are combined in the global scene in such a manner as to make it possible to use only by naming their name, the usage of classes is something that is concealed within the classes. This is because the code within the classes is isolated beyond the global reach within the class scope. Therefore, we need to utilise the code from a global point of view, and we accomplish this by constructing instances from a class.
I am saying objects and not objects, since we may construct as many things from the same class as we like, and all of them will share the methods and attributes of the class. See the following picture:
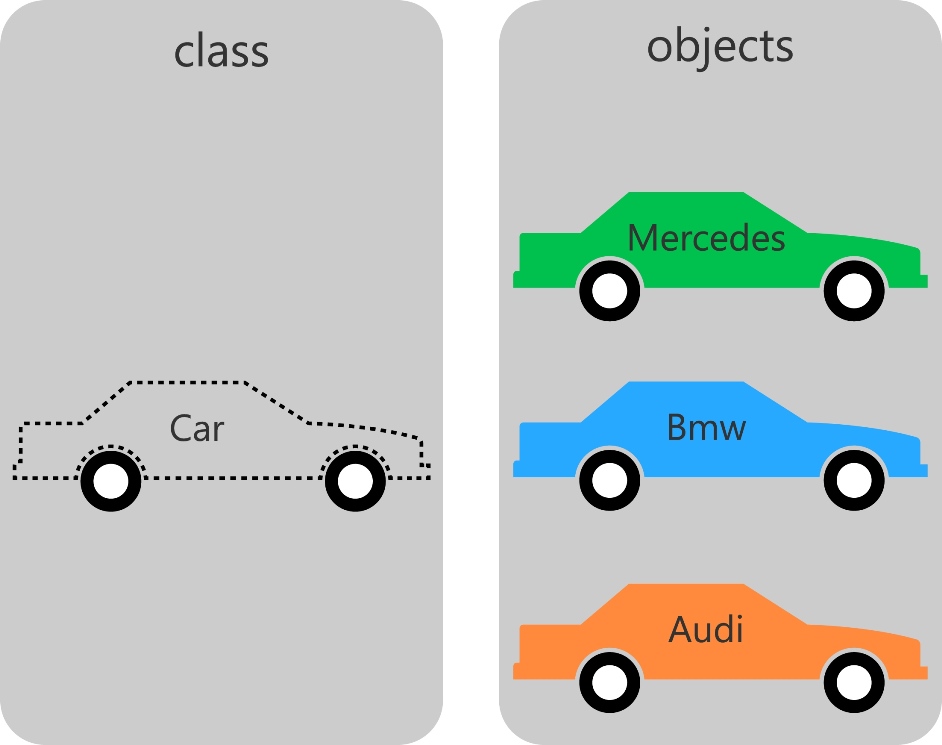
Three separate things named: Mercedes, Bmw and Audi were constructed from the same (Car Class).
Even if all objects were created in the same class and so have the methods and characteristics of the class, they are different. This is because not only can they have various names, but they might also have different values allocated to their attributes. for example, In the above image, the colour characteristics are differ – The Mercedes are green while the Bmw is blue, while the Audi is orange.
How to create Object of the class?
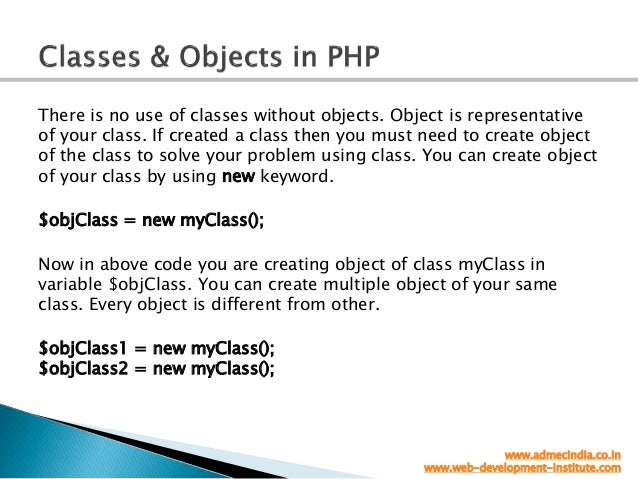
You can construct as many objects as you like for that class type once you have determined your class. This is an example of how objects may be created by using (new
) operator.
$bmw = new Car ();
We created the object $bmw
from the class Car
with the new
keyword.
We can create more than one object from the same class.
$bmw = new Car ();
$mercedes = new Car ();
In fact, we may build as many objects as we wish from the same Class and then add a set of characteristics for each object.
Let’s now create the application that uses our classes.
The Animal, Cow, and Lion classes should all be in the same directory for simplicity’s sake.
Some Working Examples:-
Example 1:-
Output:-

Example 2:-
Output:-

Conclusion:-
You have taken your most important step in object-oriented programming with this lesson through learning about classes and what the objects may be produced from them. There are many ways to learn about Class and Object in PHP like Video Tutorials on YouTube, Notes, Blogs, Articles, etc. I have share this Tutorials for you all guys with a great explanation and useful examples. Hope this will help you in a great way. Thanks for being with us.

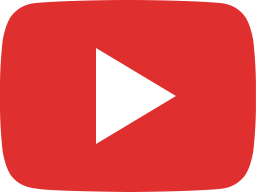
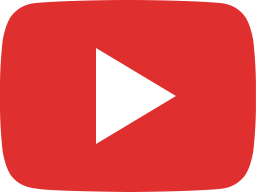

- Building a DevOps Culture: Tips and Strategies - April 4, 2024
- Why Understanding DevOps Could Be Your Key To Success In The Music Industry - April 4, 2024
- Top Picks: The Best Laptops for Graphic Designers in 2024 - March 31, 2024