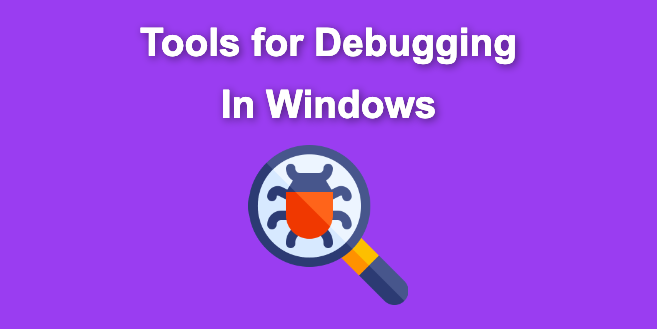
Are you tired of spending hours trying to track down elusive bugs in your code? Do you wish there was an easier way to debug your programs? Look no further! In this article, we’ll explore a variety of debugging tools and techniques that can help you find and fix bugs more quickly and efficiently.
Introduction to Debugging
Before we dive into the specifics of debugging tools, let’s first define what we mean by “debugging.” In the world of programming, debugging refers to the process of finding and fixing errors (or “bugs”) in your code. These bugs can cause your program to crash, produce incorrect results, or behave in unexpected ways.
Debugging is an essential skill for any programmer, but it can also be one of the most time-consuming and frustrating parts of the development process. That’s why it’s important to have the right tools and techniques at your disposal to make the process as smooth and painless as possible.
Types of Bugs
Before we can talk about how to debug your code, it’s important to understand the different types of bugs you might encounter. Here are a few common types of bugs:
- Syntax errors: These are errors that occur when your code violates the rules of the programming language. For example, forgetting a closing parenthesis or semicolon can cause a syntax error.
- Logic errors: These are errors that occur when your code doesn’t do what you intended it to do. For example, if you’re trying to calculate the average of a set of numbers, but you accidentally divide by the wrong number, you’ll get the wrong result.
- Runtime errors: These are errors that occur while your program is running. For example, if you try to access an array element that doesn’t exist, you’ll get a runtime error.
Debugging Techniques
Now that we’ve covered the basics of debugging, let’s talk about some techniques you can use to find and fix bugs in your code.
Print statements
One of the simplest and most effective debugging techniques is to use print statements. By inserting print statements throughout your code, you can see the values of variables and the flow of your program as it runs. This can help you identify where your code is going wrong and what values are causing problems.
For example, if you’re trying to calculate the area of a rectangle but getting the wrong result, you could add a print statement to see what values your program is using to calculate the area:
width = 5
height = 10
area = width * height
print("width:", width)
print("height:", height)
print("area:", area)
This would output:
width: 5
height: 10
area: 50
From this output, you can see that the width and height values are correct, but the area value is wrong. This tells you that there’s an issue with your calculation.
Debuggers
Another powerful debugging tool is a debugger. Debuggers allow you to step through your code line by line, inspect variables, and see the state of your program at any given point. This can be especially useful for tracking down elusive bugs that are difficult to find with print statements alone.
Most programming languages come with a built-in debugger, or you can use a third-party debugger like PyCharm or Visual Studio Code.
Code Profilers
In addition to print statements and debuggers, code profilers can also be helpful for identifying performance issues and bottlenecks in your code. A code profiler is a tool that measures the time and resources used by different parts of your program. This can help you identify areas of your code that are slow or inefficient and optimize them for better performance.
Some popular code profiling tools include cProfile and PyCharm’s built-in profiler.
Conclusion
Debugging is an essential part of the programming process, but it doesn’t have to be a frustrating and time-consuming task. By using the right tools and techniques, you can find and fix bugs more quickly and efficiently, leaving you with more time to focus on writing great code.
So the next time you encounter a bug in your code, try out some of the techniques we’ve covered in this article and see how they can help you debug like a pro!
- Tips on How to Become a DevOps Engineer - April 28, 2024
- Computer Programming Education Requirements – What You Need to Know - April 28, 2024
- The Role of Big Data in Higher Education - April 28, 2024