History of php
father of php rasmus lerdorf in 1994 version 1.0 in(june).
(that time he was created (CGI) Comment Gateway Interface
and written in C )
second version in appril 1996 was released 2.0
login Acces Password Protection Create/Display Forms.
form interpetred.
and
(1)conditional satements [to hidding the blocks ]
(2) Access to datbases and terms scripting language
6th june 1998 php version 3.0
(1) personal Home Page
(2) php Hepertext Preprocessor.
PHP 4.0
22th may 2000 new zend Engine version 4.0
PHP 5.0
july 2004 Zend engine (2.0) and version 5.0
php 5.5 suported 64-bits builds
Posibility of PHP:-
PHP is a server-side scripting language designed for web development
PHP powers everything from blogs to the most popular websites in the world
Where is PHP used
The world’s largest social network, Facebook uses PHP
The world’s largest content management system, WordPress runs on PHP
Many developers use PHP as a server-side language
PHP is simple. Hence, many beginners use it.
Why PHP
PHP is secure
PHP runs on many operating systems like Windows, Mac OS, Linux, etc.
PHP is supported by many servers like Apache, IIS, Lighttpd, etc.
PHP supports many database systems like MYSQL, Mango DB, etc.
PHP is free
The power of PHP
PHP can serve dynamic web pages
PHP can collect, validate, save form data
PHP can add, modify, delete data in the database
PHP can handle sessions and cookies
PHP can read, write, delete files on the server
PHP can serve Images, PDFs, Flash Movies and more file types
PHP can resize, compress, edit images
About the PHP Language
PHP Language is a human-readable programming language which can easily be learned. The below code will output “Hello World”
<?php
echo “helloworld”;
?>
Why Should I Learn PHP?
PHP is beginner-friendly. If you are a beginner, you can understand PHP without much effort.
PHP is one of the highest paid programming languages.
lets go for creating simple programs in php
how to display the content using php tag:-
how to give comment in php [ double back salesh // example: //echo “hello word”;
<?php
$message=”hello world”;
echo $message;
?
example 2
<?php
$meesage=”hello world”;
echo $message;
//echo “hello world”; ?>
Function
PHP functions are similar to other programming languages. A function is a piece of code
which takes one more input in the form of parameter and does some processing and
returns a value.
<?php
/* Defining a PHP Function */
function writeMessage() {
echo “You are really a nice person, Have a nice time!”;
}
/* Calling a PHP Function */
writeMessage();
?>
<?php
/* Defining a PHP Function */
function display($name1,$name2)
}
$name=”welcome to america”;
echo “$name1 to $name2”;
} ?>
<?php
function simplemsg() {
echo “Hello world!”;
}
simplemsg(); // call the function
?>
<?php
function familyName($fname, $year) {
echo “$fname Refsnes. Born in $year <br>”;
}
familyName(“Hege”, “1975”);
familyName(“Stale”, “1978”);
familyName(“Kai Jim”, “1983”);
?>
Strings
What if you needed to add a single quote inside a single quoted string?
Just escape that character with a back slash. \’
A string is collection of charactors .string is one of the data types supported by php.
Escape Sequence Meaning
\n Line Break
\r Carriage Return
\t Tab Space
\\ Backslash
\$ Dollar Sign
\” Double Quotes
example 1
<?php
echo “hello world”;
echo”<br>”;
echo”\”hello world\ “”;
echo”\n\t hello world\n”;
?>
example 2
<?php
$display = “sam”;
echo “My name is $display”;
?>
example 3
<?php
$veg = ‘Apple’;
// echo “$veg”; # there’s no variable $fruits
echo “{$veg}s”;
?>
Note: Out put data will be show with content with s
as a Apples.
example 4
<?php
// both works
echo “Hello <br>”;
echo ‘Hello <br>’;
// escape sequences are not parsed in single quotes
echo “World” .”<br>”; // outputs “World”
echo ‘”World” <br>’; // outputs “World”
// variables are not parsed in single quotes
$hello = “Hello”;
echo “$hello World <br>”; // outputs Hello World
echo ‘$hello World <br>’; // outputs $hello World ?>
Array
Note:-
An array stores multiple values in one single variable:
What is an Array?
An array is a special variable, which can hold more than one value at a time.
A list of items (a list of car names, for example), storing the cars in single variables could look like this:
An array can be declared using the array() function.
An array can be declared wrapped with [ and ].
array are colection of data items store under a single name.
Indexed or Numeric Arrays:
An array with a numeric index where values are stored linearly.
Associative Arrays:
An array with a string index where instead of linear storage, each value can be assigned a specific key.
Multidimensional Arrays:
An array which contains single or multiple array within it and can be accessed via multiple indices.
example 1
<?php
$fee [“ramu”]= 500;
echo $fee[“ramu”];
?>
example 2
<?php
$fee [“ramu”]= 900;
echo “rahul fee:”,$fee[“ramu”];
?>
example 3
<?php
$fees = array(“ali”=>500, “sonu”=>300 );
echo $fees [“ali”];
?>
example 4
<?php
$vegetable = array(“pumking”, “chilli”, “redish”);
echo “I like ” . $vegetable[0] . “, ” . $vegetable[1] . ” and ” . $vegetable[2] . “.”;
?>
example 5
<?php
// Defining a multidimensional array
$favorites = array(
array(
“name” => “ali”,
“mob” => “0000000000”,
“email” => “ali@gmail.com”,
),
array(
“name” => “Monty”,
“mob” => “00000000000”,
“email” => “monty@gmail.com”,
),
array(
“name” => “sammu “,
“mob” => “000000000000”,
“email” => “sammu@gmail.com”,
)
);
// Accessing elements
echo ” email-id is: ” . $favorites[0][“email”], “\n”;
echo ” mobile number is: ” . $favorites[1][“mob”];
?>
Numeric Array
Arrays can store numbers, strings and any object but their index will be represented by numbers. By default array index starts from zero.
<?php /* First method to create array. */ $numbers = array( 1, 2, 3, 4, 5); foreach( $numbers as $value ) { echo “Value is $value <br />”; } /* Second method to create array. */ $numbers[0] = “one”; $numbers[1] = “two”; $numbers[2] = “three”; $numbers[3] = “four”; $numbers[4] = “five”; foreach( $numbers as $value )
{ echo “Value is $value <br />”; } ?>
Class and objects
paper-source:0;} div.WordSection1 {page:WordSection1;} –>
Object is class type variable. the collection of object is called class.
class:- This is a programmer-defined data type, which includes local functions as well as local data.
Object – An individual instance of the data structure defined by a class. Member Variable – These are the variables defined inside a class. Member function – These are the function defined inside a class and are used to access object data.
<?php
class Myclass
{
// Add property statements here
// Add the methods here
}
$myobj = new MyClass;
var_dump($myobj);
?>
Notes:- class —–class is keyword of php
Myclass—–is class name
$myobjet– Here $myobj represents an object of the class Myclass.
new :——–object of Myclass
var_dum : ————here var_dum is a ——-function
example 2
<?php
class Myclass
{
public $font_size =10;
}
$f = new MyClass;
echo $f->font_size;
?>
example 3
<?php
class Myclass
{
public $font =”18px”;
public $font_color = “blue”;
public $string_name = “w3resource”;
public function customize_print()
{
echo “<p style=font-size:”.$this->font_size.”;color:”.$this->font_color.”;>”.$this->string_name.”</p>”;
}
}
$f = new MyClass;
echo $f->customize_print();
?>
example 4
<?php
class Myclass
{
public $vegetable =””apple;
}
$dd =new Myclass;
echo $f->vegetable;
?>

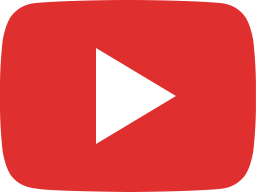
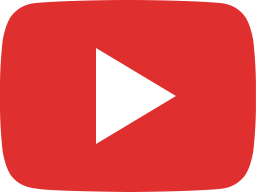

- AJAX Crud with laravel 5.8 - October 29, 2021
- Upload image in the database with local folder and image send mail. - October 23, 2020
- How to protect .env file in Laravel. - September 10, 2020