Start some containers
In this section you will start up some containers that you can work with in the following sections. When all the containers are started it will look like something like this.
# Start a detached (-d) Redis container named redis with its /data volume
# mounted on directory ./data/redis
$ docker run -d --name redis -v $PWD/data/redis:/data redis
# Start a Mongo container
$ docker run -d --name mongo mongo
# Start a Postgres container
$ docker run -d --name postgres postgres
# Start an Nginx container with port published on 80 (-p) and the docker
# socket mounted as a read-only volume
$ docker run -d -p 80:80 \
-v /var/run/docker.sock:/tmp/docker.sock:ro \
--name nginx \
jwilder/nginx-proxy
# Browse to the container http://docker
$ open http://docker # open only works on mac, copy-paste in other browsers
# $ Start a counter web app with a VIRTUAL_HOST environment
# variable set to memory-counter.docker and port published on 8081
$ docker run -d \
-e VIRTUAL_HOST=memory-counter.docker \
-p 8081:80 \
--name memory-counter \
andersjanmyr/counter
# Browse to the container, click the counter or the digit
$ open http://memory-counter.docker # or http://docker:8081
# $ Start a counter web app linked to redis with a VIRTUAL_HOST environment
# variable set to redis-counter.docker and port published on 8082
$ docker run -d --link redis -e REDIS_URL=redis:6379 \
-e VIRTUAL_HOST=redis-counter.docker \
-p 8082:80 \
--name redis-counter \
andersjanmyr/counter
# Browse to the container, click the counter or the digit
$ open http://redis-counter.docker # or http://docker:8082
# $ Start a counter web app linked to postgres with a VIRTUAL_HOST environment
# variable set to postgres-counter.docker and port published on 8083
$ docker run -d --link postgres \
-e POSTGRES_URL=postgres://postgres@postgres \
-e VIRTUAL_HOST=postgres-counter.docker \
-p 8083:80 \
andersjanmyr/counter
# Browse to the container, click the counter or the digit
$ open http://postgres-counter.docker # or http://docker:8083
# $ Start a counter web app linked to mongo with a VIRTUAL_HOST environment
# variable set to mongo-counter.docker and port published on 8084
$ docker run -d --link mongo \
-e MONGO_URL=mongo:27017 \
-e VIRTUAL_HOST=mongo-counter.docker \
-p 8084:80 \
andersjanmyr/counter
# Browse to the container, click the counter or the digit
$ open http://mongo-counter.docker # or http://docker:8084
# Check that the containers are running
$ docker ps
Check the logs
Most Docker images are configured to log to stdout. This is extremely useful since it makes it very easy to check the logs of different images with a simple command, docker logs
# Find out the name of the running containers
$ docker ps
# Check the logs of some of the running database containers
# List the logs of the Redis image
$ docker logs redis
# Follow (-f) the logs of the Mongo database
$ docker logs -f mongo
# List the logs of the Postgres images with docker timestamps (-t)
$ docker logs -t postgres
Inspect the containers
Containers, both running and stopped, can be inspected with docker inspect. docker inspect lists information about the container in a JSON format. Since the command outputs a lot of information it can be useful with some additional tools for finding the information.
# Inspect the Redis container
$ docker inspect redis
# Get the hostname of the Redis container
$ docker inspect --format '{{.Config.Hostname}}' redis
# Find everything with Host in the name with `grep`
# This is useful if you don't know exectly what you are looking for
$ docker inspect redis | grep Host
# Add a few lines of context to help figure out the path to it
$ docker inspect redis | grep Host -C2
# List the entire Config object with `json`
$ docker inspect --format '{{json .Config}}' redis
# Add `jq` to pretty-print the JSON
$ docker inspect --format '{{json .Config}}' redis |jq
# Or use `jq` without format
# Note that you have to start with .[] to get into the outer array
$ docker inspect redis |jq .[].Config
It is useful to learn more about the information provided by docker inspect. Here’s a small guide to the most interesting parts of the output.
- Name – the name of the container.
- Path – the command that started the container.
- Args – the arguments to the command.
- Config – the containers view of the configuration, a merge of data from the Dockerfile, and values that have been added on the command line, such as –env, –workdir, –tty, etc.
- HostConfig – the hosts view of the container. This maps well to the host options given at the command-line, such as –publish, –memory, –privileged, –cpu*, –volume, –link, etc.
- Networking – Networking settings, IPAddress, and Ports are particularly useful.
- Mounts – the mounted volumes
- State – the state of the container, running, stopped, etc.
…

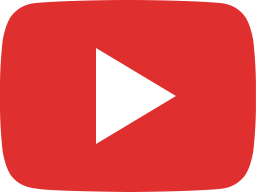
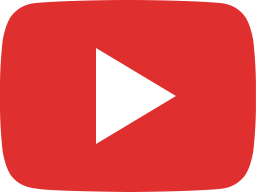
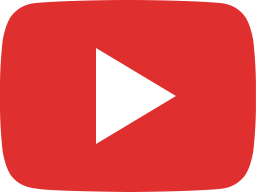
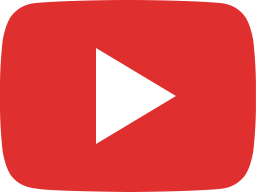
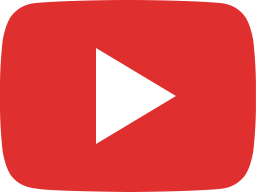
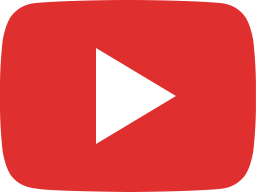
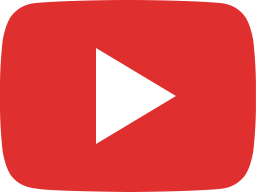
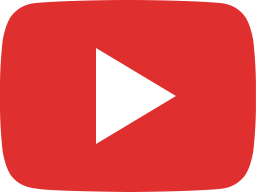
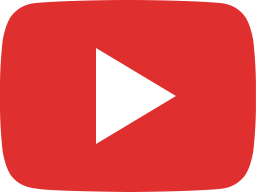
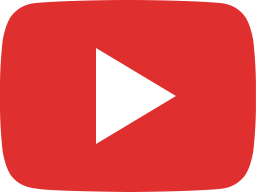
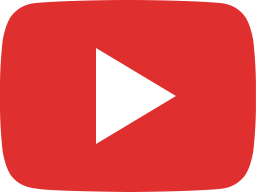
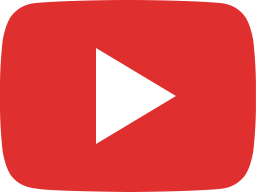
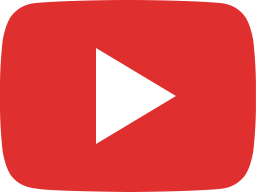
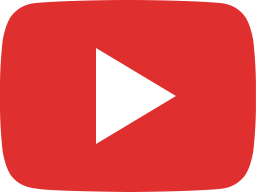
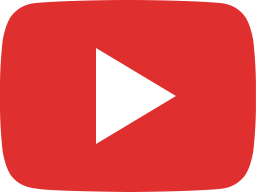

- Apache Lucene Query Example - April 8, 2024
- Google Cloud: Step by Step Tutorials for setting up Multi-cluster Ingress (MCI) - April 7, 2024
- What is Multi-cluster Ingress (MCI) - April 7, 2024