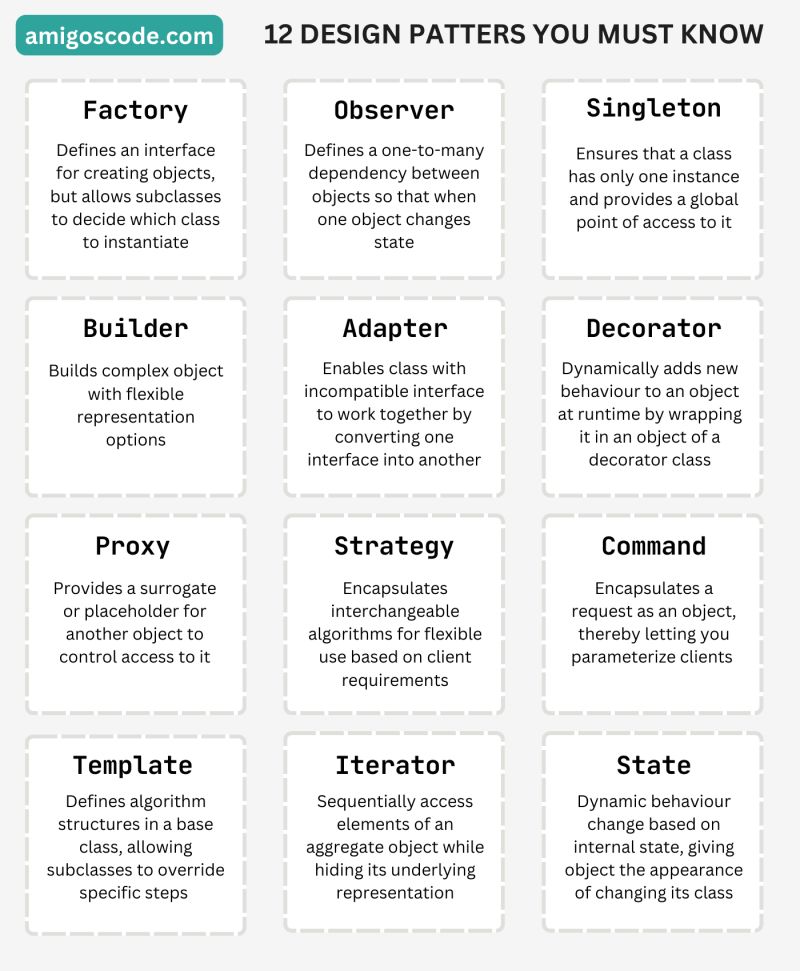
A design pattern is a general reusable solution to a commonly occurring problem in software design. It is not a finished design that can be transformed directly into source or machine code. Rather, it is a description or template for how to solve a problem that can be used in many different situations.
Design patterns are often used to solve problems related to object-oriented design, but they can also be used to solve problems in other areas of software development, such as functional programming and distributed systems.
There are many different design patterns, but some of the most common ones include:
- Singleton: This pattern ensures that there is only one instance of a class in existence.
- Factory: This pattern creates objects without exposing the underlying creation logic to the client code.
- Builder: This pattern separates the construction of a complex object from its representation.
- Observer: This pattern allows objects to be notified of changes to other objects.
- Adapter: This pattern allows objects with incompatible interfaces to work together.
- Decorator: This pattern allows objects to be dynamically extended with new functionality.
Design patterns are reusable solutions to common problems encountered in software design and development. They provide proven, structured approaches to solving specific design challenges. Here’s a list of some commonly used design patterns categorized into three main groups: creational, structural, and behavioral.
Creational Design Patterns:
- Singleton Pattern: Ensures a class has only one instance and provides a global point of access to it.
- Factory Method Pattern: Defines an interface for creating an object but lets subclasses alter the type of objects that will be created.
- Abstract Factory Pattern: Provides an interface for creating families of related or dependent objects without specifying their concrete classes.
- Builder Pattern: Separates the construction of a complex object from its representation, allowing the same construction process to create different representations.
- Prototype Pattern: Creates new objects by copying an existing object, known as the prototype.
Structural Design Patterns:
- Adapter Pattern: Allows the interface of an existing class to be used as another interface.
- Bridge Pattern: Separates an object’s abstraction from its implementation, allowing them to vary independently.
- Composite Pattern: Composes objects into tree structures to represent part-whole hierarchies.
- Decorator Pattern: Attaches additional responsibilities to an object dynamically, providing a flexible alternative to subclassing.
- Facade Pattern: Provides a simplified interface to a set of interfaces in a subsystem, making it easier to use.
- Flyweight Pattern: Reduces memory usage or computational expenses by sharing as much as possible with other related objects.
- Proxy Pattern: Provides a surrogate or placeholder for another object to control access to it.
Behavioral Design Patterns:
- Chain of Responsibility Pattern: Passes a request along a chain of handlers. Each handler decides whether to process the request or pass it to the next handler in the chain.
- Command Pattern: Encapsulates a request as an object, allowing for parameterization of clients with queuing, requests, and operations.
- Interpreter Pattern: Defines a grammar for interpreting a language and provides an interpreter to interpret sentences in the language.
- Iterator Pattern: Provides a way to access elements of an aggregate object sequentially without exposing its underlying representation.
- Mediator Pattern: Defines an object that centralizes communication between objects in a system, promoting loose coupling.
- Memento Pattern: Captures an object’s internal state so that it can be restored to this state later.
- Observer Pattern: Defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified and updated automatically.
- State Pattern: Allows an object to alter its behavior when its internal state changes. The object will appear to change its class.
- Strategy Pattern: Defines a family of algorithms, encapsulates each one, and makes them interchangeable. It allows the algorithm to vary independently from the context that uses it.
- Template Method Pattern: Defines the skeleton of an algorithm in the superclass but lets subclasses override specific steps of the algorithm without changing its structure.
- Visitor Pattern: Represents an operation to be performed on elements of an object structure. It lets you define a new operation without changing the classes of the elements on which it operates.
- What is Mobile Virtual Network Operator? - April 18, 2024
- What is Solr? - April 17, 2024
- Difference between UBUNTU and UBUNTU PRO - April 17, 2024