In Terraform, there are several types of variables that you can define, including:
- String: A sequence of characters. Example: “hello”.
- Number: A numeric value. Example: 42.
- Boolean: A logical value that is either true or false.
- List: A sequence of values of the same type. Example: [ “apples”, “oranges”, “bananas” ].
- Map: A collection of key-value pairs, where the keys and values can be of any type. Example: { “name” = “John”, “age” = 30 }.
- Object: A complex data type that can contain multiple attributes. Example: { name = “John”, age = 30, address = { street = “123 Main St”, city = “Anytown”, state = “CA” } }.
- Tuple: A sequence of values of different types. Example: [ “John”, 30, true ].
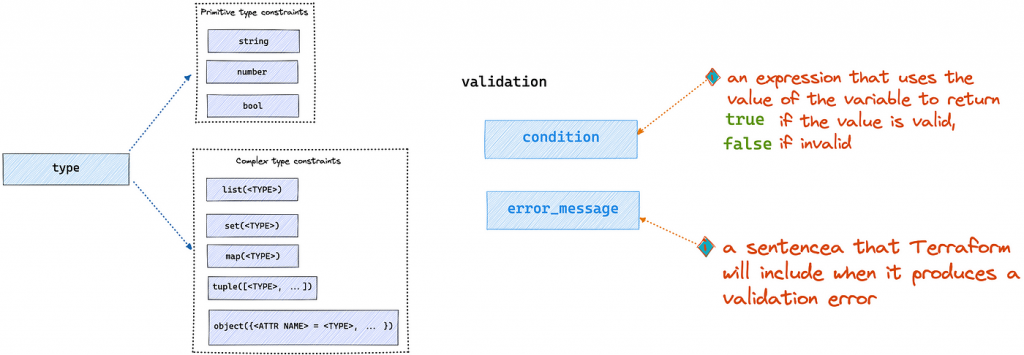
Quick Tutorials on Terraform Variable
Terraform Variables and Configuration explained – 5 mins reading!
Terraform Variable Naming convention
Types of Terraform variable
- Types of Terraform variable – Number
- Types of Terraform variable – String
- Types of Terraform variable – List
- Types of Terraform variable – Map
- Types of Terraform variable – Boolean
Location of Declaring Terraform Variable

- Environment Variables
- Local Values
- Command Line
- terraform.tfvars
- anyotherfile.tfvars
- Terrform Outout Variable
- Example of terraform terraform.tfvars
- Terraform Environment Variables Exaplained!
One Example Code
variable "instance_count" {
type = number
default = 1
}
variable "instance_name" {
type = string
default = "Rajesh"
}
resource "aws_instance" "example-number" {
count = var.instance_count
ami = "ami-053b0d53c279acc90"
instance_type = "t2.micro"
tags = {
Name = var.instance_name
}
}
variable "security_groups" {
type = list(string)
default = ["sg-0541801a7a059ba17"]
}
resource "aws_instance" "example-list" {
ami = "ami-053b0d53c279acc90"
instance_type = "t2.micro"
vpc_security_group_ids = var.security_groups
}
variable "instance_tags" {
type = map(string)
default = {
Name = "my-instance4mMap-Rajesh"
}
}
resource "aws_instance" "example-map" {
ami = "ami-053b0d53c279acc90"
instance_type = "t2.micro"
tags = var.instance_tags
}
variable "create_vm" {
description = "If set to true, it will create vm"
type = bool
}
resource "aws_instance" "example-bool" {
count = var.create_vm ? 1 : 0
ami = "ami-053b0d53c279acc90"
instance_type = "t2.micro"
tags = var.instance_tags
}

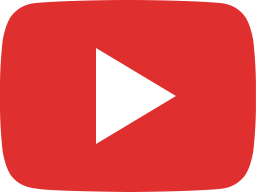
Terraform Basic Tutorial with Demo by Piyush 2020
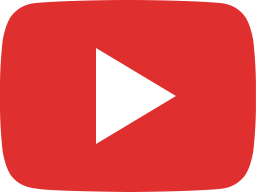
Terraform Fundamental Tutorials by Harish in 2020
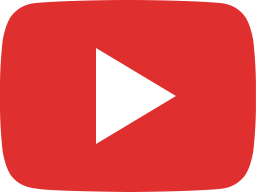
Terraform Fundamental Tutorial By Guru in 2020 Part-1
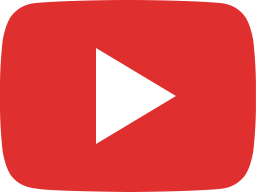
Terraform Fundamental Tutorial By Guru in 2020 Part-2
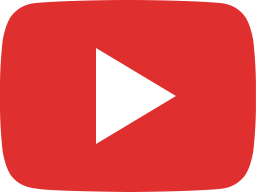
Terraform Fundamental Tutorial By Guru in 2020 Part-3
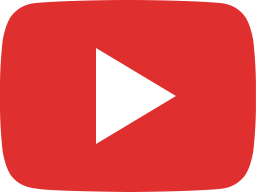
Terraform Fundamental Tutorial By Guru in 2020 Part-4
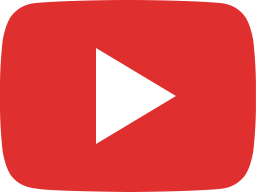
Terraform Fundamental Tutorial By Guru in 2020 Part-5
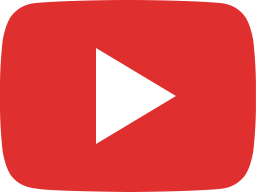
Terraform Advance Tutorial for Beginners with Demo 2020 — By DevOpsSchool

Latest posts by Rajesh Kumar (see all)
- How to remove sensitive warning from ms office powerpoint - July 14, 2024
- AIOps and DevOps: A Powerful Duo for Modern IT Operations - July 14, 2024
- Leveraging DevOps and AI Together: Benefits and Synergies - July 14, 2024