- What is C language?
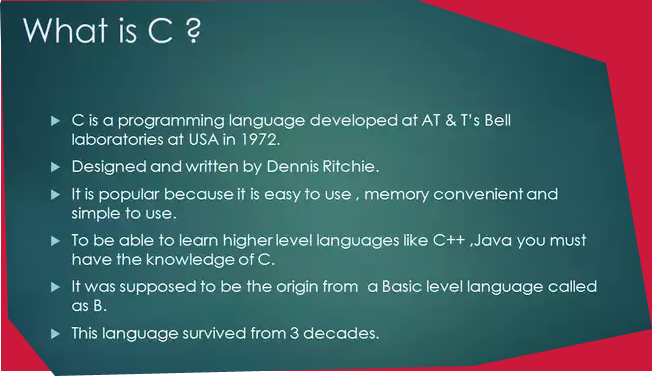
C is a mid-level and procedural programming language. The Procedural programming language is also known as the structured programming language is a technique in which large programs are broken down into smaller modules, and each module uses structured code. This technique minimizes error and misinterpretation.
2. Why is C called a mid-level programming language?
C is called a mid-level programming language because it binds the low level and high -level programming language. We can use C language as a System programming to develop the operating system as well as an Application programming to generate menu driven customer driven billing system.
3. Why is C known as a mother language?
C is known as a mother language because most of the compilers and JVMs are written in C language. Most of the languages which are developed after C language has borrowed heavily from it like C++, Python, Rust, javascript, etc. It introduces new core concepts like arrays, functions, file handling which are used in these languages.
4. Who is the founder of C language?
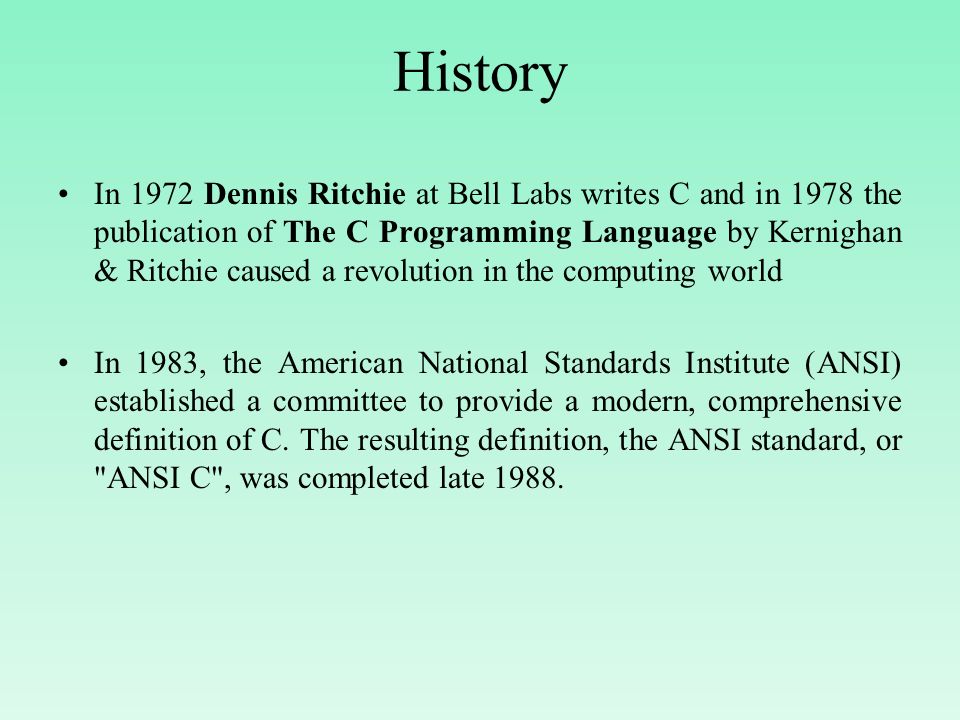
Dennis Ritchie
5. When was C language developed?
C language was developed in 1972 at bell laboratories of AT&T.
6. What is the use of printf() and scanf() functions?
printf(): The printf() function is used to print the integer, character, float and string values on to the screen.
Following are the format specifier:
- %d: It is a format specifier used to print an integer value.
- %s: It is a format specifier used to print a string.
- %c: It is a format specifier used to display a character value.
- %f: It is a format specifier used to display a floating point value.
scanf(): The scanf() function is used to take input from the user.
7. What are the features of the C language?
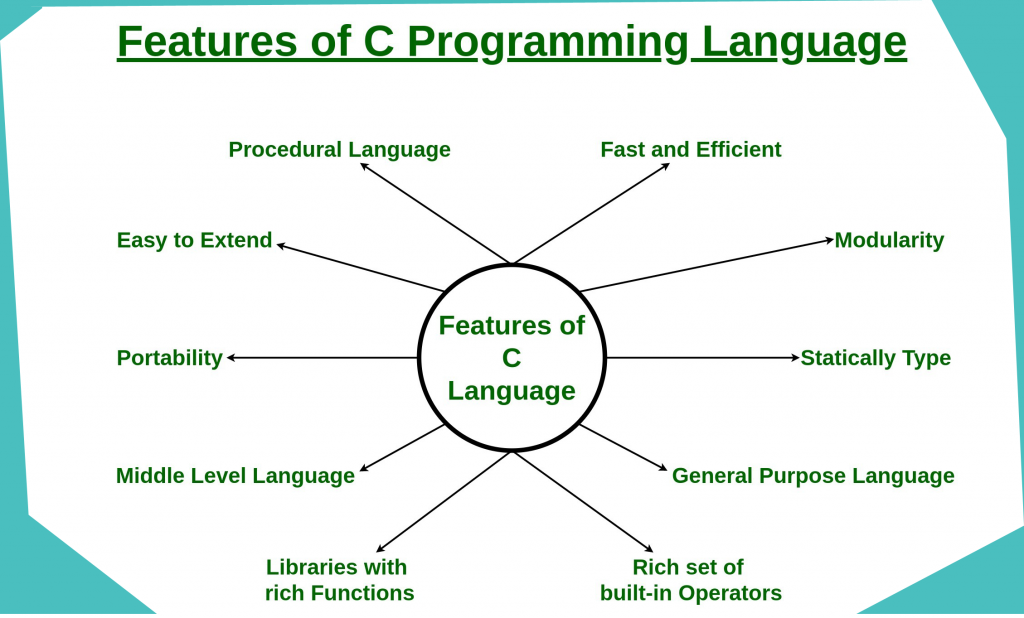
The main features of C language are given below:
- Simple: C is a simple language because it follows the structured approach, i.e., a program is broken into parts
- Portable: C is highly portable means that once the program is written can be run on any machine with little or no modifications.
- Mid Level: C is a mid-level programming language as it combines the low- level language with the features of the high-level language.
- Structured: C is a structured language as the C program is broken into parts.
- Fast Speed: C language is very fast as it uses a powerful set of data types and operators.
- Memory Management: C provides an inbuilt memory function that saves the memory and improves the efficiency of our program.
- Extensible: C is an extensible language as it can adopt new features in the future.
8. What is the difference between the local variable and global variable in C?
Following are the differences between a local variable and global variable:
Basis for comparison | Local variable | Global variable |
Declaration | A variable which is declared inside function or block is known as a local variable. | A variable which is declared outside function or block is known as a global variable. |
Scope | The scope of a variable is available within a function in which they are declared. | The scope of a variable is available throughout the program. |
Access | Variables can be accessed only by those statements inside a function in which they are declared. | Any statement in the entire program can access variables. |
Life | Life of a variable is created when the function block is entered and destroyed on its exit. | Life of a variable is created when the function block is entered and destroyed on its exit. |
Storage | Variables are stored in a stack unless specified. | The compiler decides the storage location of a variable. |
9. What is the use of the function in C?

Uses of C function are:
- C functions are used to avoid the rewriting the same code again and again in our program.
- C functions can be called any number of times from any place of our program.
- When a program is divided into functions, then any part of our program can easily be tracked.
- C functions provide the reusability concept, i.e., it breaks the big task into smaller tasks so that it makes the C program more understandable.
10. What is the use of a static variable in C?
Following are the uses of a static variable:
- A variable which is declared as static is known as a static variable. The static variable retains its value between multiple function calls.
- Static variables are used because the scope of the static variable is available in the entire program. So, we can access a static variable anywhere in the program.
- The static variable is initially initialized to zero. If we update the value of a variable, then the updated value is assigned.
- The static variable is used as a common value which is shared by all the methods.
- The static variable is initialized only once in the memory heap to reduce the memory usage.
11. What is pointer to pointer in C?
In case of a pointer to pointer concept, one pointer refers to the address of another pointer. The pointer to pointer is a chain of pointers. Generally, the pointer contains the address of a variable. The pointer to pointer contains the address of a first pointer. Let’s understand this concept through an example:
________________________________________
#include <stdio.h>
int main()
{
int a=10;
int *ptr,**pptr; // *ptr is a pointer and **pptr is a double pointer.
ptr=&a;
pptr=&ptr;
printf("value of a is:%d",a);
printf("\n");
printf("value of *ptr is : %d",*ptr);
printf("\n");
printf("value of **pptr is : %d",**pptr);
return 0;
}
________________________________
In the above example, pptr is a double pointer pointing to the address of the ptr variable and ptr points to the address of ‘a’ variable.
12. What is static memory allocation?
- In case of static memory allocation, memory is allocated at compile time, and memory can’t be increased while executing the program. It is used in the array.
- The lifetime of a variable in static memory is the lifetime of a program.
- The static memory is allocated using static keyword.
- The static memory is implemented using stacks or heap.
- The pointer is required to access the variable present in the static memory.
- The static memory is faster than dynamic memory.
- In static memory, more memory space is required to store the variable.
___________________________
For example:
int a[10];
_________________
The above example creates an array of integer type, and the size of an array is fixed, i.e., 10
13. What is dynamic memory allocation?
- In case of dynamic memory allocation, memory is allocated at runtime and memory can be increased while executing the program. It is used in the linked list.
- The malloc() or calloc() function is required to allocate the memory at the runtime.
- An allocation or deallocation of memory is done at the execution time of a program.
- No dynamic pointers are required to access the memory.
- The dynamic memory is implemented using data segments.
- Less memory space is required to store the variable.
___________________________________
For example
int *p= malloc(sizeof(int)*10);
___________________________
14. What is the difference between malloc() and calloc()?
calloc() | malloc() | |
Description | The malloc() function allocates a single block of requested memory. | The calloc() function allocates multiple blocks of requested memory. |
Initialization | It initializes the content of the memory to zero. | It does not initialize the content of memory, so it carries the garbage value. |
It does not initialize the content of memory, so it carries the garbage value. | It consists of two arguments. | It consists of only one argument. |
Return value |
15. What is an auto keyword in C?
In C, every local variable of a function is known as an automatic (auto) variable. Variables which are declared inside the function block are known as a local variable. The local variables are also known as an auto variable. It is optional to use an auto keyword before the data type of a variable. If no value is stored in the local variable, then it consists of a garbage value.
16. What is a token?
The Token is an identifier. It can be constant, keyword, string literal, etc. A token is the smallest individual unit in a program. C has the following tokens:
- Identifiers: Identifiers refer to the name of the variables.
- Keywords: Keywords are the predefined words that are explained by the compiler.
- Constants: Constants are the fixed values that cannot be changed during the execution of a program.
- Operators: An operator is a symbol that performs the particular operation.
- Special characters: All the characters except alphabets and digits are treated as special characters.
17. What is command line argument?
The argument passed to the main() function while executing the program is known as command line argument. For example:
_____________________________________
main(int count, char *args[]){
//code to be executed
}
____________________________
18. What is the acronym for ANSI?
The ANSI stands for ” American National Standard Institute.” It is an organization that maintains the broad range of disciplines including photographic film, computer languages, data encoding, mechanical parts, safety and more.
19. What are the functions to open and close the file in C language?
The fopen() function is used to open file whereas fclose() is used to close file.
20. What are the basic Datatypes supported in C Programming Language?
The Datatypes in C Language are broadly classified into 4 categories. They are as follows:
Basic Datatypes
Derived Datatypes
Enumerated Datatypes
Void Datatypes
The Basic Datatypes supported in C Language are as follows:
Datatype Name | Datatype Size | Datatype Range |
short | 1 byte | -128 to 127 |
unsigned short | 1 byte | 0 to 255 |
char | 1 byte | -128 to 127 |
unsigned char | 1 byte | 0 to 255 |
int | 2 bytes | -32,768 to 32,767 |
unsigned int | 2 bytes | 0 to 65,535 |
long | 4 bytes | -2,147,483,648 to 2,147,483,647 |
unsigned long | 4 bytes | 0 to 4,294,967,295 |
float | 4 bytes | 3.4E-38 to 3.4E+38 |
double | 8 bytes | 1.7E-308 to 1.7E+308 |
long double | 10 bytes | 3.4E-4932 to 1.1E+4932 |
21. What do you mean by Dangling Pointer Variable in C Programming?
Ans: A Pointer in C Programming is used to point the memory location of an existing variable. In case if that particular variable is deleted and the Pointer is still pointing to the same memory location, then that particular pointer variable is called as a Dangling Pointer Variable.
22. What are static variables and functions?

Ans: The variables and functions that are declared using the keyword Static are considered as Static Variable and Static Functions. The variables declared using Static keyword will have their scope restricted to the function in which they are declared.
23. What are the valid places where the programmer can apply Break Control Statement?
Break Control statement is valid to be used inside a loop and Switch control statements.
24. To store a negative integer, we need to follow the following steps. Calculate the two’s complement of the same positive integer?
Eg: 1011 (-5)
- Step-1 − One’s complement of 5: 1010
- Step-2 − Add 1 to above, giving 1011, which is -5
25. Differentiate between Actual Parameters and Formal Parameters?
The Parameters which are sent from main function to the subdivided function are called as Actual Parameters and the parameters which are declared a the Subdivided function end are called as Formal Parameters.
26. Can a C program be compiled or executed in the absence of a main()?
The program will be compiled but will not be executed. To execute any C program, main() is required.
27. What do you mean by a Nested Structure?
When a data member of one structure is referred by the data member of another function, then the structure is called a Nested Structure.
28. What is a C Token?
Keywords, Constants, Special Symbols, Strings, Operators, Identifiers used in C program are referred to as C Tokens.
29. What is Preprocessor?
A Preprocessor Directive is considered as a built-in predefined function or macro that acts as a directive to the compiler and it gets executed before the actual C Program is executed.
In case you are facing any challenges with these C Programming Interview Questions, please write your problems in the comment section below.
30. Why is C called the Mother of all Languages?
C introduced many core concepts and data structures like arrays, lists, functions, strings, etc. Many languages designed after C are designed on the basis of C Language. Hence, it is considered as the mother of all languages.
31. What is the purpose of printf() and scanf() in C Program?
printf() is used to print the values on the screen. To print certain values, and on the other hand, scanf() is used to scan the values. We need an appropriate datatype format specifier for both printing and scanning purposes. For example,
- %d: It is a datatype format specifier used to print and scan an integer value.
- %s: It is a datatype format specifier used to print and scan a string.
- %c: It is a datatype format specifier used to display and scan a character value.
- %f: It is a datatype format specifier used to display and scan a float value.
32. Write a simple example of a structure in C Language?
Structure is defined as a user-defined data type that is designed to store multiple data members of the different data types as a single unit. A structure will consume the memory equal to the summation of all the data members.
_________________________________
struct employee
{
char name[10];
int age;
}e1;
int main()
{
printf("Enter the name");
scanf("%s",e1.name);
printf("n");
printf("Enter the age");
scanf("%d",&amp;e1.age);
printf("n");
printf("Name and age of the employee: %s,%d",e1.name,e1.age);
return 0;
}
________________________
33. Differentiate between getch() and getche()?
Ans: Both the functions are designed to read characters from the keyboard and the only difference is that
getch(): reads characters from the keyboard but it does not use any buffers. Hence, data is not displayed on the screen.
getche(): reads characters from the keyboard and it uses a buffer. Hence, data is displayed on the screen.
//Example
__________________________
#include&lt;stdio.h&gt;
#include&lt;conio.h&gt;
int main()
{
char ch;
printf("Please enter a character ");
ch=getch();
printf("nYour entered character is %c",ch);
printf("nPlease enter another character ");
ch=getche();
printf("nYour new character is %c",ch);
return 0;
}
____________________
//Output
Please enter a character
Your entered character is x
Please enter another character z
Your new character is z
34. Can I create a customized Head File in C language?
Ans: It is possible to create a new header file. Create a file with function prototypes that need to be used in the program. Include the file in the ‘#include’ section in its name.
35. What is the difference between declaring a header file with < > and ” “?
If the Header File is declared using < > then the compiler searches for the header file within the Built-in Path. If the Header File is declared using ” ” then the compiler will search for the Header File in the current working directory and if not found then it searches for the file in other locations.
36. When should we use the register storage specifier?
Ans: We use Register Storage Specifier if a certain variable is used very frequently. This helps the compiler to locate the variable as the variable will be declared in one of the CPU registers.
37. What are the different storage class specifiers in C?
Ans: The different storage specifiers available in C Language are as follows:
- auto
- register
- static
- extern
38. What is a built-in function in C?
The most commonly used built-in functions in C are sacnf(), printf(), strcpy, strlwr, strcmp, strlen, strcat, and many more.
Built-function is also known as library functions that are provided by the system to make the life of a developer easy by assisting them to do certain commonly used predefined tasks. For example, if you need to print output or your program into the terminal, we use printf() in C.
39. Why doesn’t C support function overloading?
After you compile the C source, the symbol names need to be intact in the object code. If we introduce function overloading in our source, we should also provide name mangling as a preventive measure to avoid function name clashes. Also, as C is not a strictly typed language many things(ex: data types) are convertible to each other in C. Therefore, the complexity of overload resolution can introduce confusion in a language such as C.
When you compile a C source, symbol names will remain intact. If you introduce function overloading, you should provide a name mangling technique to prevent name clashes. Consequently, like C++, you’ll have machine-generated symbol names in the compiled binary.
Additionally, C does not feature strict typing. Many things are implicitly convertible to each other in C. The complexity of overload resolution rules could introduce confusion in such kind of language.
40. What is the difference between struct and union in C?
A struct is a group of complex data structures stored in a block of memory where each member on the block gets a separate memory location to make them accessible at once
Whereas in the union, all the member variables are stored at the same location on the memory as a result to which while assigning a value to a member variable will change the value of all other members.
______________________________
/* struct & union definations*/
struct bar {
int a; // we can use a & b both simultaneously
char b;
} bar;
union foo {
int a; // we can't use both a and b simultaneously
char b;
} foo;
/* using struc and union variables*/
struct bar y;
y.a = 3; // OK to use
y.b = 'c'; // OK to use
union foo x;
x.a = 3; // OK
x.b = 'c'; // NOl this affects the value of x.a!
__________________________
41. What is the difference between ‘g’ and “g” in C?
In C double-quotes variables are identified as a string whereas single-quoted variables are identified as the character. Another major difference being the string (double-quoted) variables end with a null terminator that makes it a 2 character array.
42. What are header files and what are its uses in C programming?
In C header files must have the extension as .h, which contains function definitions, data type definitions, macro, etc. The header is useful to import the above definitions to the source code using the #include directive. For example, if your source code needs to take input from the user do some manipulation and print the output on the terminal, it should have stdio.h file included as #include , with which we can take input using scanf() do some manipulation and print using printf().
43. How many types of data type in C Language?
C language supports 2 type of data types:
a). Primary data types:
Primary data types are known as fundamental data types.Some primary data types are integer(int), floating point(float), character(char) and void.
b).Derived data types:
Derived data types are derived from primary datatypes. Some derived data types are array, structure, union and pointer.
44. Write format specifiers in C?
- %d: It is used to print an integer value.
- %s: It is used to print a string.
- %c: It is used to display a character value.
- %f: It is used to display a floating point value.
45. What is C Token?
Ans: In c, Keywords, Constants, Special Symbols, Strings, Operators, Identifiers are referred as Tokens.
46. Difference b/w Entry controlled and Exit controlled loop?
In Entry controlled loop first the loop condition is checked then after the body of the loop is executed whereas in Exit controlled loop first the body of the loop is executed then after condition is checked.
Entry Controlled Loops are : for, while
Exit Controlled Loop is : do while
47. What is a far pointer in C?
A pointer which can access all the 16 segments (whole residence memory) of RAM is known as far pointer. A far pointer is a 32-bit pointer that obtains information outside the memory in a given section.
48. What is the maximum length of an identifier?
It is 32 characters ideally but implementation specific.
49. What do you mean by the Scope of the variable? What is the scope of the variables in C?
Scope of the variable can be defined as the part of the code area where the variables declared in the program can be accessed directly. In C, all identifiers are lexically (or statically) scoped.
50. What is /0 character?
Ans: The Symbol mentioned is called a Null Character. It is considered as the terminating character used in strings to notify the end of the string to the compiler.
- Mastering Qualitative Research: The Role of Focus Groups in Data Collection - July 11, 2024
- What is robots ops? - July 10, 2024
- 5 Effective Online Learning Strategies for DevOps Professionals - July 4, 2024