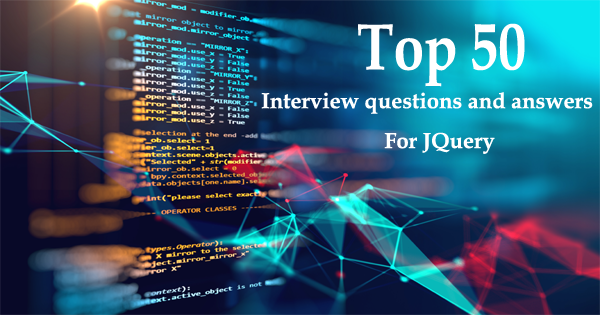
The JQuery is a wild, minor, and feature-rich JavaScript library. It brands things like HTML document traversal and operation, event handling, animation, and Ajax much humbler with an easy-to-use API that works across a crowd of browsers. With a mixture of adaptability and extensibility, jQuery has altered the way that millions of people write JavaScript.
Interview Questions and answers for JQuery
- What is jQuery?
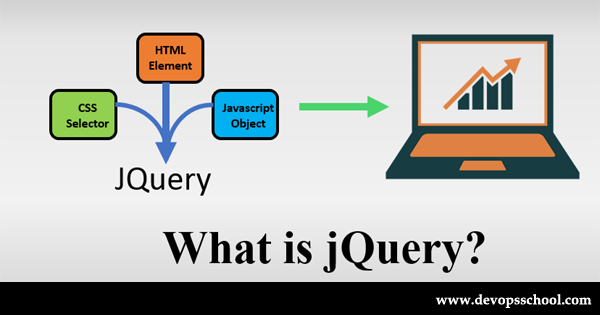
jQuery is a fast, lightweight, feature-rich client-side JavaScript library. It is cross-platform and supports different types of browsers. It has provided a much-needed boost to JavaScript. Before jQuery, JavaScript codes were lengthy and bigger, even for smaller functions. It makes a website more interactive and attractive.
2. Is jQuery a programming language?
JQuery is not a programming language but a well-written JavaScript code. It is used to traverse documents, event handling, Ajax interaction, and Animation.
3. What is the difference between JavaScript and jQuery?
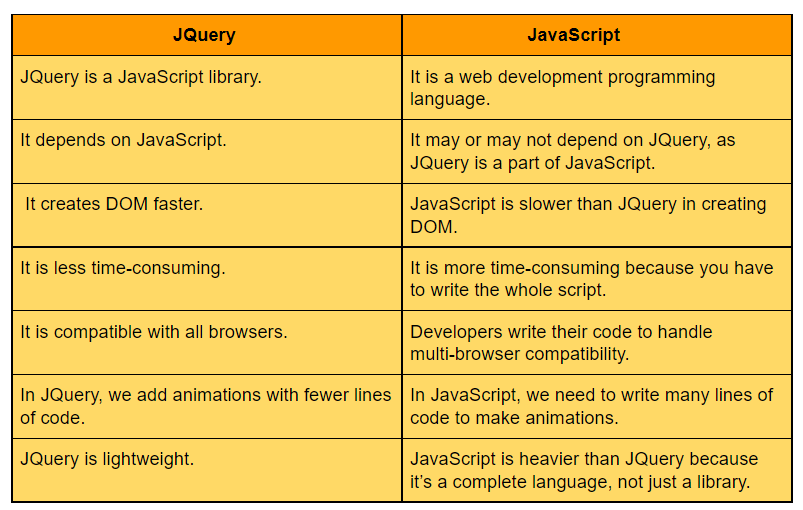
The simple difference is that JavaScript is a language while jQuery is a built-in library built for JavaScript. JQuery simplifies the use of JavaScript language.
4. Is jQuery replacement of JavaScript?
No, jQuery is not the replacement of JavaScript. JQuery is written on the top of JavaScript, and it is a different library. JQuery is a lightweight JavaScript library which is used to interact with JavaScript and HTML.
5. Why do we use jQuery?
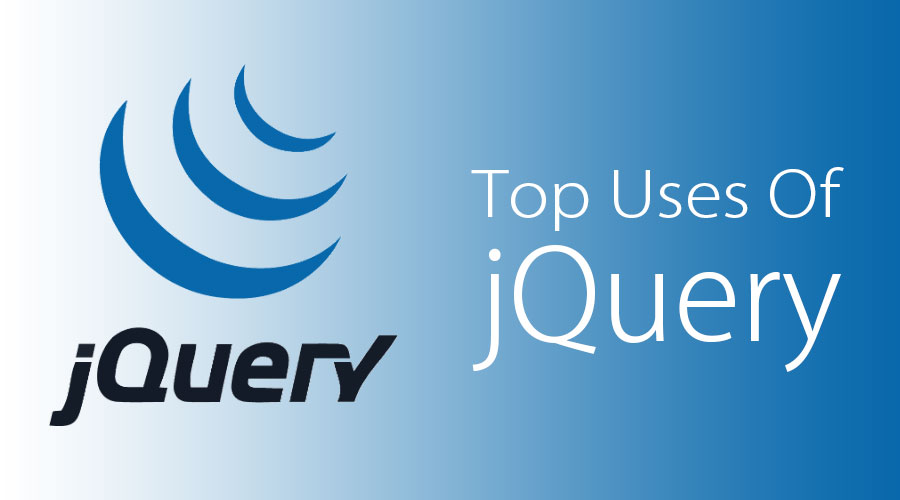
- It is very easy to learn and use.
- It is used to develop browser compatible web applications.
- It improves the performance of an application.
- It is very fast and extensible.
- It facilitates you to write minimal lines of codes for UI related functions.
- It provides cross-browser support.
6. What is $() in jQuery library?
The $() function is an alias of jQuery() function. It is used to wrap any object into jQuery object which later facilitates you to call the various method defined jQuery object. You can pass a selector string to $() function, and it returns a jQuery object which contains an array of all matched DOM elements.
Syntax:
$(document).ready(function() {
$("p").css("background-color", "pink");
});
7. What are the effects methods used in jQuery?
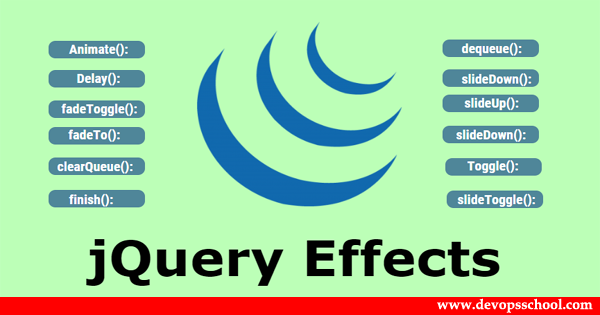
These are some effects methods used in jQuery:
show() – It displays or shows the selected elements.
hide() – It hides the matched or selected elements.
toggle() – It shows or hides the matched elements. In other words, it toggles between the hide() and shows() methods.
fadeIn() – It shows the matched elements by fading it to opaque. In other words, it fades in the selected elements.
fadeOut() – It shows the matched elements by fading it to transparent. In other words, it fades out the selected elements.
Jquery effects
8. What is the use of toggle() method in JQuery?
The jQuery toggle() is a particular type of method which is used to toggle between the hide() and show() method. It shows the hidden elements and hides the shown element.
Syntax:
$(selector).toggle();
$(selector).toggle(speed, callback);
$(selector).toggle(speed, easing, callback);
$(selector).toggle(display);
- speed: It is an optional parameter. It specifies the speed of the delay. Its possible values are slow, fast and milliseconds.
- easing: It specifies the easing function to be used for transition.
- callback: It is also an optional parameter. It specifies the function to be called after completion of toggle() effect.
- display: If true, it displays an element. If false, it hides the element.
9. What is the purpose of fadeToggle() method in JQuery?
The jQuery fadeToggle() method is used to toggle between the fadeIn() and fadeOut() methods. If the elements are faded in, it makes them faded out, and if they are faded out, it makes them faded in.
Syntax:
$(selector).fadeToggle();
$(selector).fadeToggle(speed,callback);
$(selector).fadeToggle(speed, easing, callback);
- speed: It is an optional parameter. It specifies the speed of the delay. Its possible values are slow, fast and milliseconds.
- easing: It specifies the easing function to be used for transition.
- callback: It is also an optional parameter. It specifies the function to be called after completion of fadeToggle() effect.
10. What is the use of delay() method in JQuery?
The jQuery delay() method is used to delay the execution of functions in the queue. It is the best method to make a delay between the queued jQuery effects. The jQUery delay () method sets a timer to delay the execution of the next item in the queue.
Syntax:
$(selector).delay (speed, queueName)
- speed: It is an optional parameter. It specifies the speed of the delay. Its possible values are slow, fast and milliseconds.
- queueName: It is also an optional parameter. It specifies the name of the queue. Its default value is “fx” the standard queue effect.
11. Is it possible that jQuery HTML work for both HTML and XML document?
No, jQuery HTML only works for HTML document. It doesn’t work for XML documents.
12. What is the use of html() method in JQuery?
The jQuery html() method is used to change the entire content of the selected elements. It replaces the selected element content with new contents.
Syntax:
$(document).ready(function(){
$("button").click(function(){
$("p").html("Hello <b>Javatpoint.com</b>");
});
});
13. What is the use of css() method in JQuery?
The jQuery CSS() method is used to get (return)or set style properties or values for selected elements. It facilitates you to get one or more style properties. The jQuery CSS() provides two ways:
Return a CSS property
It is used to get the value of a specified CSS property.
$(document).ready(function(){
$("button").click(function(){
alert("Background color = " + $("p").css("background-color"));
});
});
Set a CSS property
This property is used to set a specific value for all matched element.
$(document).ready(function(){
$("button").click(function(){
$("p").css("background-color", "violet");
});
});
14. Is jQuery library used for server scripting or client scripting?
It is a library for client-side Scripting.
15. Is jQuery a W3C standard?
No, jQuery is not a W3C standard.
16. What is the starting point of code execution in jQuery?
$(document).ready() function is the starting point of jQuery code. It is executed when DOM is loaded.
17. What is the basic requirement to start with the jQuery?
You need refer to its library to start with jQuery. You can download the latest version of jQuery from jQuery.com.
18. Can you use any other name in place of $ (dollar sign) in jQuery?
Yes, instead of $ (dollar sign) we can use jQuery as a function name. For example:
jQuery(document).ready(function() {
jQuery("p").css("background-color", "pink");
});
19. Can you use multiple document.ready() function on the same page?
Yes. You can use any number of document.ready() function on the same page. For example:
$(document).ready(function() {
$("h1").css("background-color", "red");
});
$(document).ready(function() {
$("p").css("background-color", "pink");
});
20. What is the difference between find and children methods?
Find method is used to find all levels down the DOM tree while children method is used to find single level down the DOM tree.
21. What is a CDN?
CDN stands for Content Delivery Network or Content Distribution Network. It is a large distributed system of servers deployed in multiple data centers across the internet. It provides the files from servers at a higher bandwidth that leads to faster loading time. These are several companies that provide free public CDNs:
- Microsoft
- Yahoo
22. What is the goal of CDN and what are the advantages of using CDN?
The primary goal of the CDN is to provide content to the end-users with high availability and high performance.
Advantages of using CDN:
- It reduces the load from the server.
- It saves bandwidth. jQuery framework is loaded faster from these CDN.
- If a user regularly visits a site which is using jQuery framework from any of these CDN, it will be cached.
23. How can you use a jQuery library in your project?
You can use a jQuery library in the ASP.Net project from downloading the latest jQuery library from jQuery.com and include the references to the jQuery library file in your HTML/PHP/JSP/Aspx page.
<script src="_scripts/jQuery-1.2.6.js" type="text/javascript"></script>
<script language="javascript">
$(document).ready(function() {
alert('test');
});
</script>
24. What are the selectors in jQuery? How many types of selectors in jQuery?
If you want to work with an element on the web page, first you need to find it. Selectors find the HTML elements in jQuery. There are many types of selectors. Some basic selectors are:
Name: It is used to select all elements which match with the given element Name.
#ID: It is used to select a single element which matches with the given ID
.Class: It is used to select all elements which match with the given Class.
Universal (*): It is used to select all elements available in a DOM.
Multiple Elements E, F, G: It is used to selects the combined results of all the specified selectors E, F or G.
Attribute Selector: It is used to select elements based on its attribute value.
25. What is a use of jQuery filter?
: jQuery filter is used to filter the specific values from the object. It filters the result of your original query into specific elements.
26. What are the different types of selectors in jQuery?
There are three types of selectors in jQuery:
CSS Selector
Custom Selector
XPath Selector
27. What is the difference between the ID selector and class selector in jQuery?
ID selector and class selector are the same as they are in CSS. ID selector uses ID while class selector uses a class to select elements.
You use an ID selector to select just one element. If you want to select a group of elements, having the same CSS class, use class selector.
28. What is the use of serialize() method in JQuery?
The jQuery serialize() method is used to create a text string in standard URL-encoded notation. It serializes the form values so that its serialized values can be used in the URL query string while making an AJAX request.
Syntax:
$(document).ready(function(){
$("button").click(function(){
$("div").text($("form").serialize());
});
});
29. What is the use of val() method in JQuery?
The jQuery val() method is used:
To get the current value of the first element in the set of matched elements.
To set the value of every matched element.
Syntax:
$(selector).val()
30. How to add and remove CSS classes to an element using jQuery?
You can use addclass() jQuery method to add CSS class to an element and removeclass() jQuery method to remove CSS class from an element.
CSS addClass() Example
<!DOCTYPE html>
<html>
<head>
<title>jQuery Example</title>
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.3/jquery.min.js">
</script>
<script type="text/javascript" language="javascript">
$(document).ready(function()
{
$("#btn").click(function()
{
$("#para").addClass("change");
});
});
</script>
<style>
.change
{
color:blue;
}
</style>
</head>
<body>
<p id="para">This method adds CSS class from an element</p>
<input type="button" id="btn" value="Click me">
</body>
</html>
CSS removeClass() Example
<!DOCTYPE html>
<html>
<head>
<title>jQuery Example</title>
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.3/jquery.min.js">
</script>
<script type="text/javascript" language="javascript">
$(document).ready(function()
{
$("#btn").click(function()
{
$("p").removeClass("change");
});
});
</script>
<style>
.change
{
color:blue;
}
</style>
</head>
<body>
<p class="change">This method removes CSS class to an element</p>
<input type="button" id="btn" value="Click me">
</body>
</html>
31. Can you write a jQuery code to select all links inside the paragraph?
Yes. You can use <a> tag nested inside paragraph <p> tag to select all links. For example:
<!DOCTYPE html>
<html>
<head>
<title>jQuery Example</title>
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.3/jquery.min.js">
</script>
<script type="text/javascript" language="javascript">
$(document).ready(function()
{
$("p a").attr("href", "https://www.javatpoint.com");
});
</script>
</head>
<body>
<p><a>Learn JavaScript</a></p>
<p><a>Learn jQuery</a></p>
</body>
</html>
32. What is the difference between prop and attr?
attr(): It gets the value of an attribute for the first element in the set of matched element.
prop(): it gets the value of a property for the first element in the set of matched elements. It is introduced in jQuery 1.6.
33. What are the two types of CDNs?
There are two types of CDN:
- Microsoft: It loads jQuery from AJAX CDN.
- Google: It loads jQuery from Google libraries API.
34. What is the use of the animate() method in jQuery?
The animate function is used to apply the custom animation effect to elements. Syntax:
$(selector).animate({params}, [duration], [easing], [callback])
Here,
“param” defines the CSS properties on which you want to apply the animation.
“duration” specify how long the animation run. It can be one of the following values: “slow,” “fast,” “normal” or milliseconds
“easing” is the string which specifies the function for the transition.
“callback” is the function which we want to run once the animation effect is complete.
35. Question: Explain the difference between the .detach() and remove() methods in jQuery.
Answer: The detach() and remove() methods are the same, except that .detach() retains all jQuery data associated with the removed elements and .remove() does not. detach() is therefore useful when removed elements may need to be reinserted into the DOM later.
36. Question: Can a jQuery library be used for server scripting?
Answer: jQuery is designed with the functionality for client-side scripting. jQuery is not compatible with server-side scripting.
37. Question: What is jQuery.noConflict?
Answer: Usually, JS functions and variables use $ as a name. In jQuery, $ is just an alias for jQuery, so we don’t need to use $. If we have to use a JS library along with jQuery, the control of $ is given to the JS library. To give this control, we use jQuery.noConflict(). It is also used to assign a new name to a variable.
38. Question: Explain the various Ajax functions available in jQuery?
Answer: There are many methods like:
.ajaxStart() – register the handler to be called when the first Ajax request begins.
.ajaxStop() – register the handler to be called when all requests are complete.
.ajaxSuccess() – register the handler to be called when an Ajax request is successfully completed.
Check all the methods on the official jQuery documentation page, which explains each method with an example.
39. Question: What is the difference between width() vs css(‘width’) in jQuery?
Answer: CSS(‘width’) returns the width value in pixels, whereas width() returns the integer (without the unit values). For example:
div{
width: 20cm;
}
If you print the values:
$(this).width();
$(this).css(‘width’);
you will get the values like 756 and 756px, respectively. Note that though we specified width in cm, it is converted to pixel (px) for output purposes.
40. Question: What is the difference between bind() vs live() vs delegate() methods in jQuery?
Answer:
bind(): this method registers the event handler directly to the required DOM element. E.g.:
$(“#members a”).bind(“click”, function(f){….});
This means any matching anchors will have this event handler attached!
- live(): this method attaches the event handler to the root of the document. This means one handler can be used for all events that propagated to the root. The handler is thus attached only once.
- delegate(): in this method, you can choose where to attach the handler. This is the most efficient and robust method for delegation.
E.g.:
$(“#members”).delegate(“ul li a”, “click”, function(f){….});
41. Question: Describe the use of the param() method in jQuery?
Answer: The param() method outputs a serialized representation of an object or array.
For example:
student = new Object();
student.name = “Mary”;
student.marks = 67;
$("div").text($.param(student);
When an event occurs that calls this code, the method will give the following output:
name=Mary&marks=67
42. Question: Explain the difference between $(this) and this in jQuery?
Answer: $() is the jQuery constructor function, whereas this is a reference to the DOM element. To use jQuery methods, we use $(this).
43. Question: Explain the difference between jquery.size() and jquery.length?
Answer: Both return the number of elements. But length is faster. As of jQuery 1.8, size() has been deprecated.
44. Question: What are the four parameters used for the jQuery Ajax method?
Answer: The four parameters are:
URL: URL for sending the request
Type: GET/POST request
Success: the callback function when the request is successful
DataType: return data type – HTML, XML, text etc.
45. Question: What are all the ways to include jQuery on a page?
Answer:
You can use <script> to add the library in the HTML <head> or <body> tag: <script src=’jquery-3.2.1.min.js’></script>
Write the code within the HTML document inside the <script> tag, here we have used cdn link.
<script src='http://ajax.aspnetcdn.com/ajax/jQuery/jquery-3.2.1.js'></script>
<script type = “text/javascript”>
$(document)……… <jQuery code>
</script>
Include the .js file, which has the jQuery code into the HTML document.
<script src='script.js' type="text/javascript"></script>
46. Question: What is the use of the css() method in JQuery?
Answer: css() sets style properties for all the selected elements. It also returns the first matched element of the specified CSS property.
<p>Welcome to styling</p>
<p>I will be styled just as the previous paragraph</p>
<button>click me to change the style</button>
<script>
$(document).ready(function(){
$(“button”).click(function(){
$(“p”).css(“color”, “blue”);
});
});
</script>
47. Question: What is jQuery Datepicker in jQuery?
Answer: It is a plugin/widget that adds datepicker functionality in HTML pages. It is highly configurable and can be customized for date format, language, restricting date selection, etc. Refer to this jQuery documentation for datepicker options.
48. Question: Define slideToggle() effect?
Answer: It is used to toggle between sliding up and sliding down for the selected elements.
<h2>This is a paragraph.</h2>
<button>show me toggle</button>
<script>
$(document).ready(function(){
$("button").click(function(){
$("h2").slideToggle();
});
});
</script>
49. Question: How can you use an array with jQuery?
Answer: To create an array use $.makeArray(<object>)
var myObj = [“John”, “Jake”, “Jack”, “King”];
var myArr = $.makeArray(myobj);
You can search for specific element in array using $.inArray()
$.inArray(“Jack”, myArr);
To merge two arrays, use $.merge() method
var arr1 = [“John”, “Jake”, “Jack”, “King”];
var arr2 = [“Mary”, “Katy”, “Jill”, “Queen”];
var mergeArr = $.merge(arr1, arr2);
50. Question: What are jQuery plugins?
Answer: Plugins are simply methods that enable developers to extend jQuery’s prototype objects. Plugins are written in a standard javascript file. jQuery provides a lot of plugins that you can download from their repository link. You can include plugins in the code using <script src = “jquery.plugin.js” type = “text/javascript”></script>
51. Question: Difference between Map and Grep function in jQuery?
Answer: Map function translates a set of elements into another set of values in a jQuery array that may or may not have the elements. The map is called:
$(“<element>”).map(<function to execute for elements in the object>)
Grep, on the other hand, finds an element in an array.
jQuery.grep(myArr, function(){}
52. Question: What is the method chaining in jQuery, and what are the advantages?
Answer: With chaining, multiple jQuery commands on a particular element can be executed in one go. It helps in implementing various actions on an element at once rather than executing them one after the other.
$("#h2").css("color","blue").animate((left: '100px'}).slideDown(1000);
53. Question: Difference between jQuery.get() and jQuery.ajax()?
Answer: In the get() method, we have to pass individual arguments, whereas the ajax() methods get all those arguments as an object.
jQuery.ajax({
url: 'mydoc.txt',
dataType: 'text',
type: “GET”,
success: function(data) {
console.log(data);
}
});
get() method accepts arguments. The three main arguments passed are explained below:
jQuery.get('mydoc.txt',function(data){
console.log(data)
},'text');
In this, the first argument is the url, the second is the callback function, and the third (‘text’) is the return type.
- Mastering Qualitative Research: The Role of Focus Groups in Data Collection - July 11, 2024
- What is robots ops? - July 10, 2024
- 5 Effective Online Learning Strategies for DevOps Professionals - July 4, 2024