- What is Kotlin?
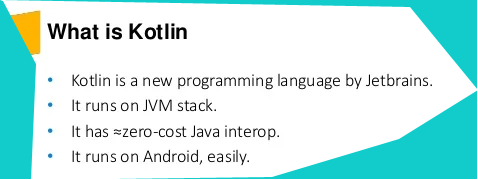
Kotlin is a statically-typed programming language which runs on the JVM. It can be compiled either using Java source code and LLVM compiler.
2. Why you should switch to Kotlin from Java?
Kotlin language is quite simple compared to Java. It reduces may redundancies in code as compared to Java. Kotlin can offer some useful features which are not supported by Java.
3. Who is the developer of Kotlin?
Kotlin was developed by JetBrains.
4. Explain the use of extension functions?
Extension functions are beneficial for extending class without the need to inherit from the class.
5. Tell three most important benefits of using Kotlin?
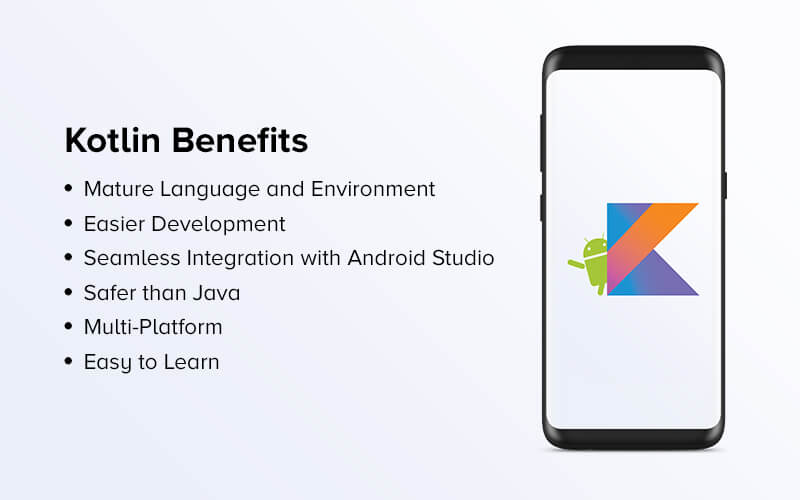
- Kotlin language is easy to learn as its syntax is similar to Java.
- Kotlin is a functional language and based on JVM. So, it removes lots of boiler plate
- It is an expressive language which makes code readable and understandable.
6. What does ‘Null Safety’ mean in Kotlin?
Null Safety feature allows removing the risk of occurrence of NullPointerException in real time. It is also possible to differentiate between nullable references and non-nullable references.
7. Is there any Ternary Conditional Operator in Kotlin like in Java?
No there is no ternary conditional operator in Kotlin language.
8. Why is Kotlin interoperable with Java?
Kotlin is interoperable with Java because it uses JVM bytecode. Compiling it directly to bytecode helps to achieve faster compile time and makes no difference between Java and Kotlin for JVM.
9. How can you declare a variable in Kotlin?
_______________________
value my_var: Char
_________________
10. How many constructors are available in Kotlin?
Two types of constructors available in Kotlin are:
- Primary constructor
- Secondary constructor
11. Give me name of the extension methods Kotlin provides to java.io.File?
- bufferedReader(): Use for reading contents of a file into BufferedReader
- readBytes() : Use for reading contents of file to ByteArray
- readText(): Use of reading contents of file to a single String
- forEachLine() : Use for reading a file line by line in Kotlin
- readLines(): Use to reading lines in file to List
12. What are some of the features which are there in Kotlin but not In Java?
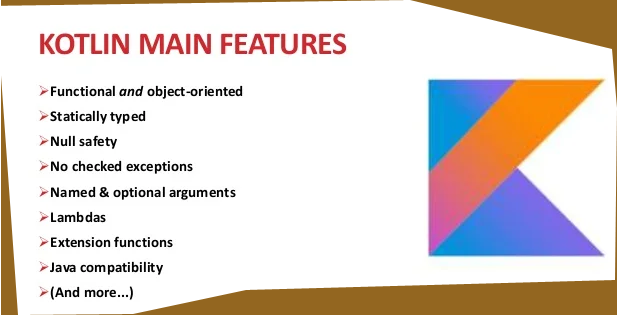
Here, are few important Kotlin features that Java doesn’t have:
- Null Safety
- Operator Overloading
- Coroutines
- Range expressions
- Smart casts
- Companion Objects
13. How can you handle null exceptions in Kotlin?
Elvis Operator is used for handling null expectations in Kotlin.
14. Does Kotlin allow macros?
No. Kotlin does not offer support for macros because the developers of Kotlin find it difficult to include it in the language.
15. Does Kotlin support primitive Datatypes?
No, Kotlin does not provide support for primitive Data types like in Java.
16. Tell me the default behavior of Kotlin classes?
In Kotlin all classes are final by default. That’s because Kotlin allows multiple inheritances for classes, and an open class is more expensive than a final class.
17. What is Ranges operator in Kotlin?
Ranges operator helps to iterate through a range. Its operator form is (..) For Example
__________________________
for (i in 1..15)
print(i)
____________________
It will print from 1 to 15 in output.
18. Give a syntax for declaring a variable as volatile in Kotlin?
____________________
Volatile var x: Long? = null
__________________
19. What is the use of abstraction in Kotlin?
Abstraction is the most important concept of Objected Oriented Programming. In Kotlin, abstraction class is used when you know what functionalities a class should have. But you are not aware of how the functionality is implemented or if the functionality can be implemented using different methods.
20. Differentiate between Kotlin and Java?
Following are the differences between Kotlin and Java:-
Basis | Kotlin | Java |
Null Safety | By default, all sorts of variables in Kotlin are non-nullable (that is, we can’t assign null values to any variables or objects). Kotlin code will fail to build if we try to assign or return null values. If we absolutely want a null value for a variable, we can declare it as follows: value num: Int? = null | NullPointerExceptions are a big source of annoyance for Java developers. Users can assign null to any variable, however, when accessing an object reference with a null value, a null pointer exception is thrown, which the user must manage. |
Coroutines Support | We can perform long-running expensive tasks in several threads in Kotlin, but we also have coroutines support, which halt execution at a given moment without blocking threads while doing long-running demanding operations. | The corresponding thread in Java will be blocked anytime we launch a long-running network I/0 or CPU-intensive task. Android is a single-threaded operating system by default. Java allows you to create and execute numerous threads in the background, but managing them is a difficult operation. |
Data Classes | If we need to have data-holding classes in Kotlin, we may define a class with the keyword “data” in the class declaration, and the compiler will take care of everything, including constructing constructors, getter, and setter methods for various fields. | Let’s say we need a class in Java that only holds data and nothing else. Constructors, variables to store data, getter and setter methods, hashcode(), function toString(), and equals() functions are all required to be written explicitly by the developer. |
Functional Programming | Kotlin is procedural and functional programming (a programming paradigm where we aim to bind everything in functional units) language that has numerous useful features such as lambda expressions, operator overloading, higher-order functions, and lazy evaluation, among others. | Java does not allow functional programming until Java 8, however it does support a subset of Java 8 features when developing Android apps. |
Extension Functions | Kotlin gives developers the ability to add new functionality to an existing class. By prefixing the name of a class to the name of the new function, we can build extended functions. | In Java, we must create a new class and inherit the parent class if we want to enhance the functionality of an existing class. As a result, Java does not have any extension functions. |
Data Type Inference | We don’t have to declare the type of each variable based on the assignment it will handle in Kotlin. We can specify explicitly if we want to. | When declaring variables in Java, we must declare the type of each variable explicitly. |
Smart Casting | Smart casts in Kotlin will take care of these casting checks with the keyword “is-checks,” which checks for immutable values and conducts implicit casting. | We must examine the type of variables in Java and cast them appropriately for our operation. |
Checked Exceptions | We don’t have checked exceptions in Kotlin. As a result, developers do not need to declare or catch exceptions, which has both benefits and drawbacks. | We have checked exceptions support in Java, which enables developers to declare and catch exceptions, resulting in more robust code with better error handling. |
21. What do you understand about function extension in the context of Kotlin? Explain.
In Kotlin, we can add or delete method functionality using extensions, even without inheriting or altering them. Extensions are statistically resolved. It provides a callable function that may be invoked with a dot operation, rather than altering the existing class.
Function Extension – Kotlin allows users to specify a method outside of the main class via function extension. We’ll see how the extension is implemented at the functional level in the following example:
______________________
// KOTLIN
class Sample {
var str : String = "null"
fun printStr() {
print(str)
}
}
fun main(args: Array<String>) {
var a = Sample()
a.str = "Interview"
var b = Sample()
b.str = "Bit"
var c = Sample()
c.str = a.add(b)
c.printStr()
}
// function extension
fun Sample.add(a : Sample):String{
var temp = Sample()
temp.str = this.str + " " +a.str
return temp.str
}
________________________
Output:-
___________________
Interview Bit
______________
Explanation:-
We don’t have a method named “addStr” inside the “Sample” class in the preceding example, but we are implementing the same method outside of the class. This is all because of function extension.
22. Differentiate between open and public keywords in Kotlin?
The keyword “open” refers to the term “open for expansion”. The open annotation on a class is the polar opposite of the final annotation in Java: it allows others to inherit from it. By default, a class cannot be inherited in Kotlin. In Kotlin, an open method signifies that it can be overridden, whereas it cannot be by default. Instead, any methods in Java can be overridden by default.
In Kotlin, all the classes are public by default. If no visibility modifier is specified, public is used by default, which means our declarations will be accessible everywhere inside the program.
23. Explain about the “when” keyword in the context of Kotlin?
The “when” keyword is used in Kotlin to substitute the switch operator in other languages such as Java. When a certain condition is met, a specific block of code must be run. Inside the when expression, it compares all of the branches one by one until a match is discovered.
After finding the first match, it proceeds to the conclusion of the when block and executes the code immediately following the when block. We do not need a break statement at the end of each case, unlike switch cases in Java or any other programming language.
For example,
__________________
// KOTLIN
fun main(args: Array<String>) {
var temp = "Interview"
when(temp) {
"Interview" -> println("Interview Bit is the solution.")
"Job" -> println("Interview is the solution.")
"Success" -> println("Hard Work is the solution.")
}
}
____________________
Output:-
____________________________
Interview Bit is the solution
_____________________
Explanation:- In the above code, the variable temp has the value “Interview”. The when condition matches for the exact value as that of temp’s and executes the corresponding code statements. Thus, “Interview Bit is the solution” is printed.
24. What are the advantages of Kotlin over Java?
Following are the advantages of Kotlin over Java:-
- Data class: In Java, you must create getters and setters for each object, as well as properly write hashCode (or allow the IDE to build it for you, which you must do every time you update the class), toString, and equals. Alternatively, you could utilize lombok, but that has its own set of issues. In Kotlin, data classes take care of everything.
- Patterns of getter and setter: In Java, for each variable, you use it for, rewrite the getter and setter methods. You don’t have to write getter and setter in kotlin, and if you must, custom getter and setter take a lot less typing. There are additional delegates for identical getters and setters.
- Extension Functions: In Java, there is no support for extension functions. Kotlin on the other hand provides support for extension functions which makes the code more clear and cleaner.
- Support for one common codebase: You may extract one common codebase that will target all of them at the same time using the Kotlin Multi-Platform framework.
- Support for Null Safety: Kotlin has built-in null safety support, which is a lifesaver, especially on Android, which is full of old Java-style APIs.
- Less prone to errors: There is less space for error because it is more concise and expressive than Java.
25. Differentiate between lateinit and lazy initialisation. Explain the cases when you should use lateinit and when you should use lazy initialisation?
Following are the differences between lateinit and lazy initialisation:-
lateinit | lazy initialisation |
The main purpose is to delay the initialisation to a later point in time. | The main purpose is to initialise an object only when it is used at a later point in time. Also, a single copy of the object is maintained throughout the program |
It’s possible to initialise the object from anywhere in the program. | Only the initializer lambda can be used to initialise it. |
Multiple initializations are possible in this case. | Only a single initialisation is possible in this case. |
It’s not thread-safe. In a multi-threaded system, it is up to the user to correctly initialise. | Thread-safety is enabled by default, ensuring that the initializer is only called once. |
It works only with var. | It works only with val. |
The isInitialized method is added to verify if the value has previously been initialised. | It is impossible to uninitialize a property. |
Properties of primitive types are not allowed | Allowable on primitive type properties. |
There are a few easy principles to follow when deciding whether to use lateinit or lazy initialisation for property initialization:
- Use lateInit if properties are mutable (i.e., they may change later).
- Use lateinit if properties are set externally (for example, if you need to pass in an external variable to set it). There is still a way to use lazy, but it isn’t as obvious.
- If they’re only meant to be initialised once and shared by everybody, and they’re more internally set (depending on a class variable), then lazy is the way to go. We could still use lateinit in a tactical sense, but utilising lazy initialisation would better encapsulate our initialization code.
26. What do you understand about coroutines in the context of Kotlin?
Unlike many other languages with equivalent capabilities, async and await are neither keywords nor part of Kotlin’s standard library. JetBrains’ kotlinx.coroutines library is a comprehensive library for coroutines. It includes a number of high-level coroutine-enabled primitives, such as launch and async. Kotlin Coroutines provide an API for writing asynchronous code in a sequential manner.
Coroutines are similar to thin threads. Coroutines are lightweight since they don’t allocate new threads when they’re created. Instead, they employ pre-defined thread pools as well as intelligent scheduling. The process of deciding which piece of work you will do next is known as scheduling. Coroutines can also be paused and resumed in the middle of their execution. This means you can have a long-term project that you can work on incrementally. You can pause it as many times as you want and continue it whenever you’re ready.
27. Explain scope functions in the context of Kotlin. What are the different types of Scope functions available in Kotlin?
The Kotlin standard library includes numerous functions that aid in the execution of a block of code within the context of an object. When you use a lambda expression to call these functions on an object, temporary scope is created. These functions are referred to as Scope functions. The object of these functions can be accessed without knowing its name. Scope functions make code more clear, legible, and succinct, which are key qualities of the Kotlin programming language.
Following are the different types of Scope functions available in Kotlin:-
- let:-
- Context object: it
Return value: lambda result
The let function is frequently used for null safety calls. For null safety, use the safe call operator(?.) with ‘let’. It only runs the block with a non-null value. - apply:-
- Context object: this
Return value: context object
“Apply these to the object,” as the name suggests. It can be used to operate on receiver object members, primarily to initialise them. - with:-
- Context object: this
Return value: lambda result
When calling functions on context objects without supplying the lambda result, ‘with’ is recommended. - run:-
- Context object: this
Return value: lambda result
The ‘run’ function is a combination of the ‘let’ and ‘with’ functions. When the object lambda involves both initialization and computation of the return value, this is the method to use. We can use run to make null safety calls as well as other calculations. - also:-
- Context object: it
Return value: context object
It’s used when we need to do additional operations after the object members have been initialised
28. What do you understand about sealed classes in Kotlin?
Kotlin introduces a crucial new form of class that isn’t seen in Java. These are referred to as “sealed classes.” Sealed classes, as the name implies, adhere to constrained or bounded class hierarchies. A sealed class is one that has a set of subclasses. When it is known ahead of time that a type will conform to one of the subclass types, it is employed. Type safety (that is, the compiler will validate types during compilation and throw an exception if a wrong type has been assigned to a variable) is ensured through sealed classes, which limit the types that can be matched at compile time rather than runtime.
The syntax is as follows:-
_________________
sealed class className
___________________
Another distinguishing aspect of sealed classes is that their constructors are by default private. Due to the fact that a sealed class is automatically abstract, it cannot be instantiated.
For example,
_____________________
// KOTLIN
sealed class Sample {
class A : Sample() {
fun print()
{
println(“This is the subclass A of sealed class Sample”)
}
}
class B : Sample() {
fun print()
{
println(“This is the subclass B of sealed class Sample”)
}
}
}
fun main()
{
val obj1 = Sample.B()
obj1.print()
val obj2 = Sample.A()
obj2.print()
}
___________________________
Output:-
________________________________
This is the subclass B of sealed class Sample
This is the subclass A of sealed class Sample
_________________________
Explanation:– In the above code, we have created a sealed class named “Sample” and we have created two sub classes within it named “A” and “B”. In the main function, we create an instance of both the sub classes and call their “print” method.
29. Differentiate between launch / join and async / await in Kotlin?
- launch / join:-
The launch command is used to start and stop a coroutine. It’s as though a new thread has been started. If the code inside the launch throws an exception, it’s considered as an uncaught exception in a thread, which is typically written to stderr in backend JVM programs and crashes Android applications. Join is used to wait for the launched coroutine to complete before propagating its exception. A crashed child coroutine, on the other hand, cancels its parent with the matching exception.
- async / await:-
The async keyword is used to initiate a coroutine that computes a result. You must use await on the result, which is represented by an instance of Deferred. Uncaught exceptions in async code are held in the resultant Deferred and are not transmitted anywhere else. They are not executed until processed.
30. What are some of the disadvantages of Kotlin?
Following are some of the disadvantages of Kotlin:
- In Kotlin, there are a few keywords that have non-obvious meanings: internal, crossinline, expect, reified, sealed, inner, open. Java has none of these.
- Checked exceptions are likewise absent in Kotlin. Although checked exceptions have become less prominent, many programmers believe them to be an effective technique to ensure that their code is stable.
- A lot of what happens in Kotlin is hidden. You can almost always trace the logic of a program in Java. When it comes to bug hunting, this can be really useful. If you define a data class in Kotlin, getters, setters, equality testing, tostring, and hashcode are automatically added for you.
- Learning resources are limited. The number of developers who are moving to Kotlin is growing, yet there is a small developer community accessible to help them understand the language or address problems during development.
- Kotlin has variable compilation speed. In some situations, Kotlin outperforms Java, particularly when executing incremental builds. However, we must remember that when it comes to clean builds, Java is the clear winner.
31. How to ensure null safety in Kotlin?
One of the major advantages of using Kotlin is null safety. In Java, if you access some null variable then you will get a NullPointerException. So, the following code in Kotlin will produce a compile-time error:
___________________________
var name: String = "MindOrks"
name = null //error
____________________
So, to assign null values to a variable, you need to declare the name variable as a nullable string and then during the access of this variable, you need to use a safe call operator i.e. ?.
_________________________
var name: String? = "MindOrks"
print(name?.length) // ok
name = null // ok
____________________
32. Do we have a ternary operator in Kotlin just like java?
No, we don’t have a ternary operator in Kotlin but you can use the functionality of ternary operator by using if-else or Elvis operator.
33. What is Elvis operator in Kotlin?
In Kotlin, you can assign null values to a variable by using the null safety property. To check if a value is having null value then you can use if-else or can use the Elvis operator i.e. ?:
For example:
_______________________
var name:String? = “Mindorks”
val nameLength = name?.length ?: -1
println(nameLength)
_________________________
The Elvis operator(?:) used above will return the length of name if the value is not null otherwise if the value is null, then it will return -1.
34. How to convert a Kotlin source file to a Java source file?
Steps to convert your Kotlin source file to Java source file:
- Open your Kotlin project in the IntelliJ IDEA / Android Studio.
- Then navigate to Tools > Kotlin > Show Kotlin Bytecode.
- Now click on the Decompile button to get your Java code from the bytecode.
35. What is the use of @JvmStatic, @JvmOverloads, and @JvmFiled in Kotlin?
- @JvmStatic: This annotation is used to tell the compiler that the method is a static method and can be used in Java code.
- @JvmOverloads: To use the default values passed as an argument in Kotlin code from the Java code, we need to use the @JvmOverloads annotation.
- @JvmField: To access the fields of a Kotlin class from Java code without using any getters and setters, we need to use the @JvmField in the Kotlin code.
36. Can we use primitive types such as int, double, float in Kotlin?
In Kotlin, we can’t use primitive types directly. We can use classes like Int, Double, etc. as an object wrapper for primitives. But the compiled bytecode has these primitive types.
37. What is String Interpolation in Kotlin?
If you want to use some variable or perform some operation inside a string then String Interpolation can be used. You can use the $ sign to use some variable in the string or can perform some operation in between {} sign.
_______________________
var name = "MindOrks"
print("Hello! I am learning from $name")
__________________
38. What do you mean by destructuring in Kotlin?
Destructuring is a convenient way of extracting multiple values from data stored in(possibly nested) objects and Arrays. It can be used in locations that receive data (such as the left-hand side of an assignment). Sometimes it is convenient to destructure an object into a number of variables, for example:
________________________
val (name, age) = developer
________________
Now, we can use name and age independently like below:
______________________
println(name)
println(age)
________________
39. When to use the lateinit keyword in Kotlin?
lateinit is late initialization.
Normally, properties declared as having a non-null type must be initialized in the constructor. However, fairly often this is not convenient.
For example, properties can be initialized through dependency injection, or in the setup method of a unit test. In this case, you cannot supply a non-null initializer in the constructor, but you still want to avoid null checks when referencing the property inside the body of a class. To handle this case, you can mark the property with the lateinit modifier.
40. What is the difference between lateinit and lazy in Kotlin?
lazy can only be used for val properties, whereas lateinit can only be applied to var because it can’t be compiled to a final field, thus no immutability can be guaranteed.
If you want your property to be initialized from outside in a way probably unknown beforehand, use lateinit.
41. What is the forEach in Kotlin?
In Kotlin, to use the functionality of a for-each loop just like in Java, we use a forEach function. The following is an example of the same:
________________________________
var listOfMindOrks = listOf("mindorks.com", "blog.mindorks.com", "afteracademy.com")
listOfMindOrks.forEach {
Log.d(TAG,it)
}
________________________
42. What is the equivalent of Java static methods in Kotlin?
To achieve the functionality similar to Java static methods in Kotlin, we can use:
- companion object
- package-level function
- object
43. What is the difference between FlatMap and Map in Kotlin?
- FlatMap is used to combine all the items of lists into one list.
- Map is used to transform a list based on certain conditions.
44. What is the difference between List and Array types in Kotlin?
If you have a list of data that is having a fixed size, then you can use an Array. But if the size of the list can vary, then we have to use a mutable list.
45. What are visibility modifiers in Kotlin?
A visibility modifier or access specifier or access modifier is a concept that is used to define the scope of something in a programming language. In Kotlin, we have four visibility modifiers:
private: visible inside that particular class or file containing the declaration.
protected: visible inside that particular class or file and also in the subclass of that particular class where it is declared.
internal: visible everywhere in that particular module.
public: visible to everyone.
Note: By default, the visibility modifier in Kotlin is public.
46. What do you know about the history of Kotlin?
Kotlin was developed by the JetBrains team. This project was started in 2010 to develop a language for Android apps development, and officially its first version was released in February 2016. Kotlin was developed under the Apache 2.0 license.
47. What is the default behavior of Kotlin classes?
By default, all classes are final in Kotlin. That’s because Kotlin allows multiple inheritances for classes, and an open class is more expensive than a final class.
48. Does Kotlin provide support for macros?
No. Kotlin does not provide support for macros because the developers of Kotlin find it difficult to include them in the language.
49. What is the use of the open keyword in Kotlin?
In Kotlin, the classes and functions are final by default. So, it is not possible to inherit the class or override the functions. To achieve this, we need to use the open keyword before the class and function.
50. What do you understand by lazy initialization in Kotlin?
Kotlin provides the facility of lazy initialization, which specifies that your variable will not be initialized unless you use that variable in your code. It will be initialized only once. After that, you use the same value.
In lazy initialization, the lazy() function is used that takes a lambda and returns an instance of lazy, which can serve as a delegate for implementing a lazy property: the first call to get() executes the lambda passed to lazy() and remembers the result, subsequent calls to get() simply return the remembered result.
- Mastering Qualitative Research: The Role of Focus Groups in Data Collection - July 11, 2024
- What is robots ops? - July 10, 2024
- 5 Effective Online Learning Strategies for DevOps Professionals - July 4, 2024