- What is MATLAB?
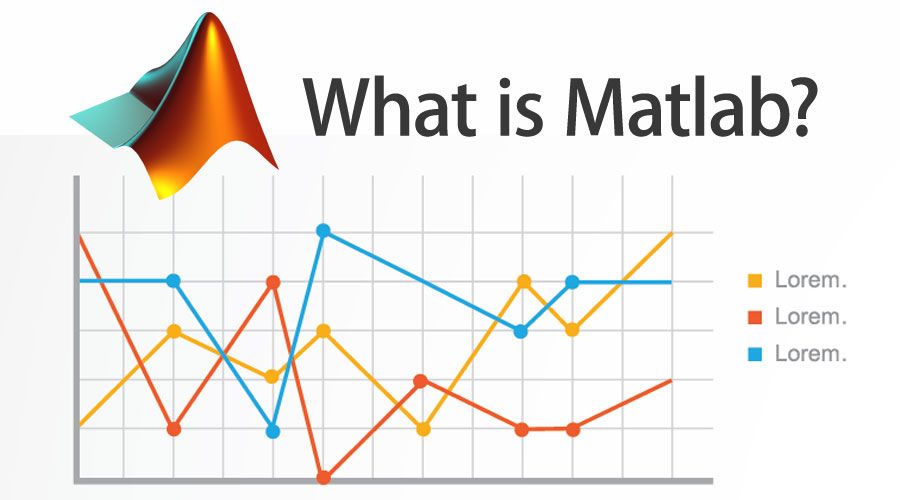
MATLAB is an acronym for MATrix LABoratory. It is an open-source software/API which was initially developed for the mathematical calculations including matrix operations. The latest version of the software can perform on various types of complex calculations such as data analysis and visualization, scientific and engineering graphics etc. It’s prime features also include simulation and modelling.
2. How to plot a graph in MATLAB?
For any two points x and y with some values given, a function called plot (x, y) is used to plot a graph in MATLAB.
_________________________________
Syntax : x : [value of array];
y : [value of array];
plot(x, y)
__________________________
3. How to call a function in MATLAB?
Ans. A function in MATLAB can be called using the name you give to the function, but first it needs to be written in the New Script tab under the File Tab. You can simply call the function by writing the function’s name in the code area.
__________________________
>> function_name (in the work area)
____________________
4. How to run MATLAB code?
NOTE:- Before running any code, make sure you save the respective code, so that your progress doesn’t fly away. Any code can run in MATLAB, once it’s saved, by using the Save and Run button in the home tab of MATLAB, or you can simply use the F5 key on your keyboard.
5. What is MATLAB used for?
MATLAB language is a high-level matrix language. It has control structures, functions, data structures, input/ output and OOP features. MATLAB API allows to author C and FORTRAN programs to interact with MATLAB. It is used for various industry level designing processes and for running the control systems, automations of different types of machines, by defining a particular set of codes for an object.
6. How to read csv file in MATLAB ?
CSV stands for Comma-Separated Values. A command called csvread is generally used to read the csv files, but is not actually preferred.
________________________
Syntax : M = csvread(filename)
__________________
The newer version of MATLAB instead recommends readmatrix to read such type of files.
______________________________
Syntax : A = readmatrix(filename)
_______________________
7. Tell me about some tools of MATLAB?
- LMI control
- Neural Networks
- Robust Control
- System Identification
- Control System
- Fuzzy Logic
- Image Processing
- LMI control
8. How to comment in MATLAB ?
Comments in MATLAB can be inserted in between the codes. The syntax for comment goes like this:-
________________________
“ % your comment goes here. ”
__________________
9. How to implement neural network in MATLAB ?
A neural network is an adaptive system that learns by using interconnected nodes or neurons in a layered structure that resembles a human brain. A neural network can learn from data, so it can be trained to recognize patterns, classify data, and forecast future events.
It breaks down the input into layers of abstraction. It can be trained using many examples to recognize patterns in speech or images, for example, just as the human brain does. Its behaviour is defined by the way its individual elements are connected and by the strength, or weights, of those connections. With just a few lines of code, MATLAB lets you develop neural networks.
The work flow for the general neural network design process has seven primary steps :-
- Collect data
- Create the network
- Configure the network
- Initialize the weights and biases
- Train the network
- Validate the network (post-training analysis)
- Use the network
MATLAB and Deep Learning Toolbox provide command-line functions and apps for creating, training, and simulating shallow neural networks. The apps make it easy to develop neural networks for tasks such as classification, regression (including time-series regression), and clustering. After creating your networks in these tools, you can automatically generate MATLAB code to capture your work and automate tasks.
10. How to open SIMULINK in MATLAB ?
SIMULINK can be easily accessed in MATLAB by the use of Home tab. Simply go on the Home tab and click Simulink.
11. How to create GUI in MATLAB ?
Steps to create a GUI :-
- Start GUIDE by typing guide at the MATLAB prompt.
- In the GUIDE Quick Start dialog box, select the Blank GUI (Default) template, and then click OK.
- Display the names of the components in the component palette:
- Select File > Preferences > GUIDE.
- Select Show names in component palette.
- Click OK.
Following the steps, you can start to create a GUI in MATLAB.
12. How to stop a program in MATLAB ?
You can simply use the quit command to stop a program in MATLAB or you can use the desktop shortcut such as Ctrl + C.
13. How to import data from EXCEL in MATLAB ?
You can do this by clicking the Import Data icon under the Home tab and navigating to the Excel file you that want to import. Its just a simple step.
14. How to add toolbox in MATLAB ?
Ans. To create a toolbox installation file :-
- In the Environment section of the Home tab, select Package Toolbox from the Add-Ons menu.
- In the Package a Toolbox dialog box, click the ‘plus’ button and select your toolbox folder. It is good practice to create the toolbox package from the folder level above your toolbox folder. The .mltbx toolbox file contains information about the path settings for your toolbox files and folders. By default, any of the included folders and files that are on your path when you create the toolbox appear on their paths after the end users install the toolbox.
- In the dialog box, add the information about your toolbox such as, ToolBox name, version, Author name, email and Company, ToolBox image, its summary and description.
- To save your toolbox, click Package at the top of the Package a Toolbox dialog box. Packaging your toolbox generates a .mltbx file in your current MATLAB folder.
15. How to declare array in MATLAB ?
An array can be declared in MATLAB using the following syntax :
________________________________
A = [1 2 3 4 5]
____________________
It creates an array of 1X5.
Or it can be declared in the following syntax :
____________________________
N=[1,2,3,4,5]
___________________
It also creates an array of same 1X5 dimension.
16. How to calculate classification accuracy in MATLAB ?
Here’s one approach we may try:
__________________________________
% output= evalfis( fis, input);
pred = round(output);
acc_count = nnz( pred==input);
acc = acc_count/length(input);
__________________________
Here we are considering round values of the fuzzy system as the predictions obtained and then counting the number of correct predictions over the total number of inputs.
17. How to generate sine wave in MATLAB ?
A program to generate sine wave in MATLAB is given below :-
____________________
t = 0:0.01:2;
w = 5;
a = 4;
st = a*sin(w*t);
plot(t, st);
__________________
Using this program and altering the values of ‘t’, ‘w’ and ‘a’, we can further generate longer sine waves.
18. How to read audio file in MATLAB ?
Here is the syntax to read audio files in MATLAB :-
_____________________________
[y, Fs] = audioread(filename)
_______________________
x Here it reads data from the file named filename, and returns sampled data, y, and a sample rate for that data, Fs.
19. What is the MATLAB working environment ?
MATLAB working environment has various tools to work with MATLAB. It has facilities to manage variables. MATLAB supports export and import data across applications. Certain tools are available to develop and manage MATLAB files. Debugging and profiling of MATLAB applications are more flexible with MATLAB. It is the blank space in between the functions and command history box where we can write the codes.
20. Explain how polynomials can be expressed in MATLAB ?
There are a number of ways in which a polynomial function can be expressed in MATLAB. Polynomials are equations of a single variable with nonnegative integer exponents. MATLAB represents polynomials with numeric vectors containing the polynomial coefficients ordered by descending power. For example, [1 -4 4] corresponds to x2 – 4x + 4. Some of the functions are :-
Poly, polyeig, polyfit, residue, roots, polyval etc.
21. Explain handle graphics in MATLAB ?
Handle Graphics is a subsystem of MATLAB that handles graphics. It has high level commands for 2D and 3D data visualization. Image processing, animation and presentation graphics can be generated using Handle Graphics. Low level commands allow customizing the graphics appearances. Handle Graphics allows to build customized Graphics User Interfaces.
22. What are the types of loops that MATLAB provides ?
MATLAB provides three types of loops, just like any other programming language, which are :-
- For loop
- While loop
- Nested loops (if else, elif etc) .
23. What are 3D-Visualization elements in MATLAB ?
3D-visualization elements lets MATLAB deal with the 3D graphics. These are some of the 3D-visualization elements in MATLAB :-
- Surface and Mesh plots – Includes plot matrices and colour maps.
- Lightening – Used for adding and controlling scene lightening.
- Transparency – Used to specify object transparency.
- Volume visualization – Used for volume data grid.
24. What are memory management functions in MATLAB ?
There are basically five types of memory management functions in MATLAB, which are:-
- clear – Removes variables from memory.
- pack – Saves the existing variables to disk, and then reloads them contiguously.
- save – Selectively persists variables to disk.
- load – Reloads a data file saved with the save function.
- quit – Exits MATLAB and returns all allocated memory to the system.
25. What do you mean by M-file in MATLAB ?
An M file is a text file used by MATLAB .It can store a script, class, or an individual function in the MATLAB language. M files are used for executing algorithms, plotting graphs, and performing other mathematical operations. It is the basic type of files that MATLAB have. The extension for m file is .m . Any file with the extension .m is a m-file.
26. What are MEX files ?
A MEX file is a function, created in MATLAB, that calls a C/C++ program or a Fortran subroutine. A MEX function behaves just like a MATLAB script or function.
The MEX file contains only one function or subroutine. The calling syntax depends on the input and output arguments defined by the MEX function. The MEX file must be on your MATLAB path.
27. What are standard toolboxes present in MATLAB? How can they be accessed?
There are a variety of toolboxes present in MATLAB, some of which are:-
- Optimization
- Neural Networks
- Partial Differential Equations
- Image processing
- Statistics
- Wavelets
- Control systems
- And many more…
To access these toolboxes, simply go to the MATLAB start menu, after which choose the Toolboxes sub menu, then choose the Toolbox which we want to use.
28. Can we run MATLAB without graphics ?
The answer is YES. We can run MATLAB without graphics too, since it is a GUI. Also, at times, we can run the script codes without displaying the graphs.
29. What is Stress Analysis in MATLAB ?
Stress Analysis or Finite Element Analysis is a computational method for predicting how any object will react to the real-world forces, heat, vibrations etc. We are well aware of the fact that MATLAB is a multidimensional software which finds its application in various disciples of engineering and for example, mechanical engineering makes use of stress analysis to design automotive et al.
30. What is the full form for MATLAB?
The full form of MATLAB is MATrix LABoratory.
31. Explain MATLAB API (Application Program Interface)?
MATLAB API is a library that allows us to write Fortran and C programs that interact with MATLAB. It includes the facilities for calling routines from MATLAB, for reading and writing Mat files and calling MATLAB as a computational engine.
32. What is The MATLAB working environment?
This is the set of tools and facilities that we work with as the MATLAB client or programmer. It contains facilities for managing the variables in our workspace and importing and exporting data. It also contains tools for developing, managing, debugging, and profiling M-files, MATLAB?s applications.
33. What is Get and Set in MATLAB?
Get and Set are indicated to as getter and setter functions. For assigning properties, setter functions are used while for accessing features, getter functions are used.
34. What are the types of Loops does MATLAB provides?
MATLAB provides loops like
- While Loop
- For Loop
- Nested Loops
35. What are the basic Plots and Graphs of MATLAB?
Following table defines basic plots and graphs:
- Box: Axis Border
- Errorbar: Plots error bars along the curve.
- Hold: Retains the current graph while adding a new graph.
- Line: Creates a line object.
- LineSpec (Line Specification): Syntax of Line Specification String.
- Loglog: Log to the log-scale plot.
- Plot: 2-D Line Plot.
- Plot3: 3-D Line Plot.
- Plotyy: 2-D Line plots with y-axis on both the left and right side
- Polar: Polar coordinate plot.
- Semilogx: Semilogarithmic plot.
- Semilogy: Semilogarithmic plot.
- Subplot: It creates an axis in tiled positions.
- Xlim: Sets or queries x-axis limits.
- Ylim:: Sets or queries y-axis limits.
- Zlim: Sets or queries z-axis limits.
36. What is latex in MATLAB?
MATLAB already deals naturally with simple latex encoding that enables introducing Greek letters or changing the font size and displays in plots.
37. What do you mean by M-file in MATLAB?
M-files are nothing but just a plain ASCII script that is interpreted at run time. We can say these are the subprograms stored in text files with .m extensions and are known as M-files. M-files are used for most of the MATLAB development, and platform independence and maintainability. It is parsed once and “just-in-time” compiled, but it is also transparent to the customer.
38. What does MatLab consist of?
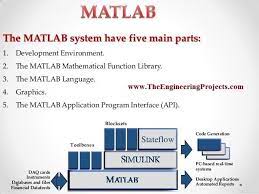
MatLab consists of five main parts
• MatLab Language
• MatLab working environment
• Handle Graphics
• MatLab function library
• MatLab Application Program Interface (API)
39. List out the operators that MatLab allows?
Matlab allows following Operators
• Arithmetic Operators
• Relational Operators
• Logical Operators
• Bitwise Operations
• Set Operations
40. Explain how to modify the MatLab Path?
To modify the MatLab Path use the PathTool GUI. Also, you can use add path directories from the command line and add the path to rc to write the current path back to ‘pathdef.m.’ In the case if you don’t have permission to write for ‘pathdef.m’ then pathrc can be written into a different file, you can execute from your ‘startup.m.
41. What is Xmath-Matlab? Mention the Xmath features?
For Xwindow workstations, Xmath is an interactive scripting and graphics environment.
Following are the X-math features
• Scripting language with OOP features
• Libraries that are LNX and C language compatible
• A debugging tools with GUI features
• Color graphics can be pointed and clickable
42. List out some of the common toolboxes present in Matlab?
Some of the common toolboxes in Matlab are
• Control System
• Fuzzy Logic
• Image Processing
• LMI control
• Neural Networks
• Robust Control
• System Identification
43. What is the disadvantage of MATLAB?
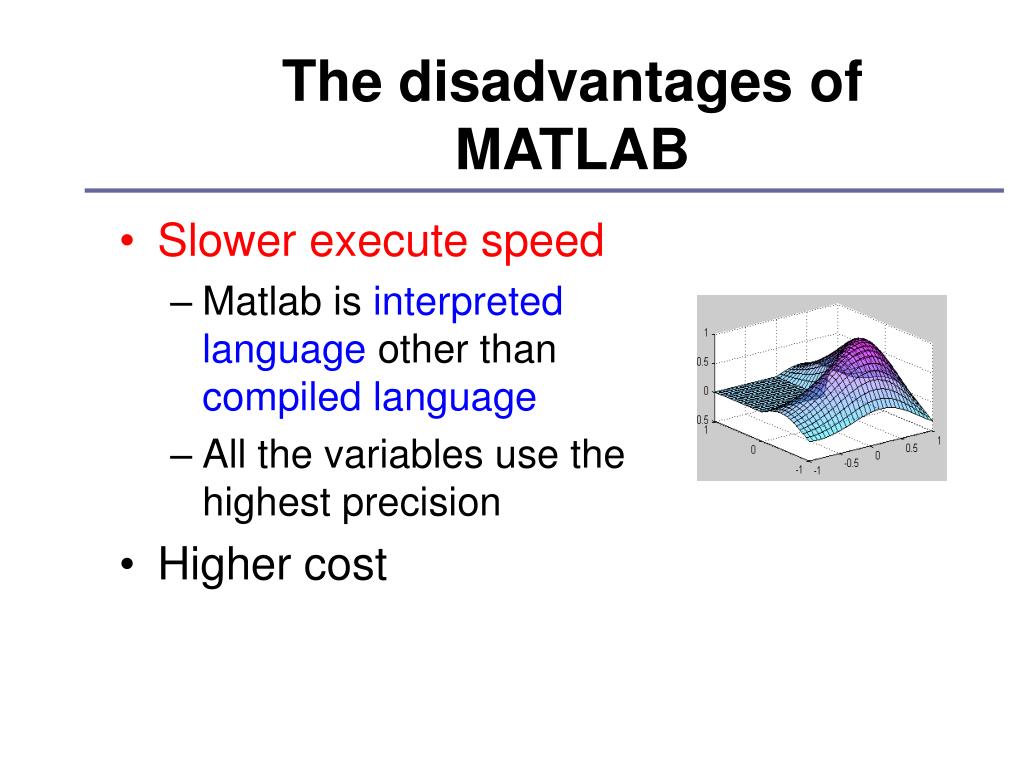
One of the disadvantages of MATLAB is that it is an interpreted language, so it may perform slower as compared to a compiled language.
44. Who uses MATLAB?
MATLAB is used by several engineers as well as scientists across the world for various applications. They use it in academia and industry, which include image and video processing, deep learning and machine learning, signal processing and communications, control systems, computational finance, test and measurement, and computational biology.
45. Is MATLAB harder than Python?
MATLAB is the easiest and highly productive computing environment for engineers as well as scientists. It uses the MATLAB language. This is the only top programming language which is dedicated to mathematical and technical computing. On the other hand, Python is called a general-purpose programming language.
46. Is MATLAB worth learning in 2022?
MATLAB is important for those who want to build a career in mathematics (abstract or applied), science, engineering, computational biology, physics, or data-oriented finance. The answer is yes, it is worth learning.
Hope these questions have helped you to understand the core concepts of Matlab better and prepare for the interview. For more learning content on Data Science and Machine Learning visit Great Learning Academy where you will find various courses for professionals for free.
47. How vectorization is helpful in MATLAB?
Firstly vectorization helps in the conversion of vector or matrix operations from “for” and while” loops, secondly its algo speeds up the code as it is really short.
For Example:
One way to compute the sine of 1001 values ranging from 0 to 10:
___________________________________
i = 0;
for t = 0:.01:10
i = i + 1;
y (i) = sin (t);
end
_______________________
A vectorized version of the same code is
______________________________
t = 0:.01:10;
y = sin(t);
____________________
The second example executes much faster than the first
48. What is a P-code?
Pcode is a prepared and encoded version of the M-file. It stores the load time of the function. This is most likely not an issue except for very high M-files since most are parsed only once anyway. Pcode also lets us hide the source code from others. There is no way to change Pcode back to the M-file source. Pcode is platform-independent.
49. How the source code can be protected in Matlab?
By default the code is saved in (.m) extension, which is secured but if the user wants it to be stored in a more secured way then he can try the following methods:
- Make it as P-code : Convert some or all of your source code files to a content-obscured form called a P-code file (from its .p file extension), and distribute your application code in this format.
- Compile into binary format : Compile your source code files using the MATLAB Compiler to produce a standalone application. Distribute the latter to end users of your application.
50. What is fminsearch?
General fits which are fitted by giving a decent initial guess for fitting parameters in it is done by fminsearch which is a multidimensional minimizer routine.
Suppose we have a set of data points (xj , yj) and a proposed fitting function of the form y = f(x, a1, a2, a3, …).
For example : we could try to fit to an exponential function
With two adjustable parameters a1 and a2 as is done in the example in leastsq.m below:
_____________________________________
f(x, a1, a2) = a1ea2x
__________________________
Or we could fit to a cubic polynomial in x2 with four adjustable parameters a1, a2, a3, a4 with this f:
___________________________________
f(x, a1, a2, a3, a4) = a1 + a2x2 + a3x4 + a4x6
__________________________
- Mastering Qualitative Research: The Role of Focus Groups in Data Collection - July 11, 2024
- What is robots ops? - July 10, 2024
- 5 Effective Online Learning Strategies for DevOps Professionals - July 4, 2024