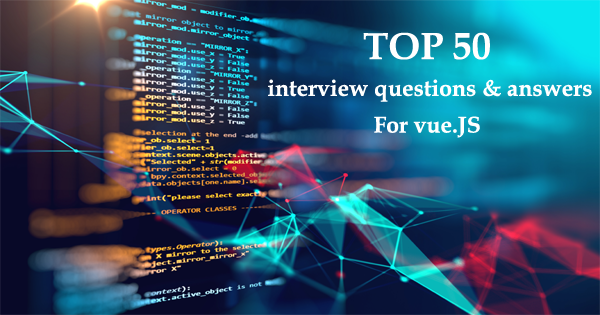
The Vue (pronounced /vjuː/, like view) is a liberal framework for building user borders. Dissimilar other huge frameworks, Vue is designed from the ground up to be incrementally adoptable. The core library is absorbed on the view layer only, and is easy to pick up and mix with other libraries or existing projects. On the other hand, Vue is also perfectly accomplished of powering urbane Single-Page Applications when used in combination with modern tooling and supporting libraries.
Interview Question & Answers:-
1. What is Vue.js?
Answer:
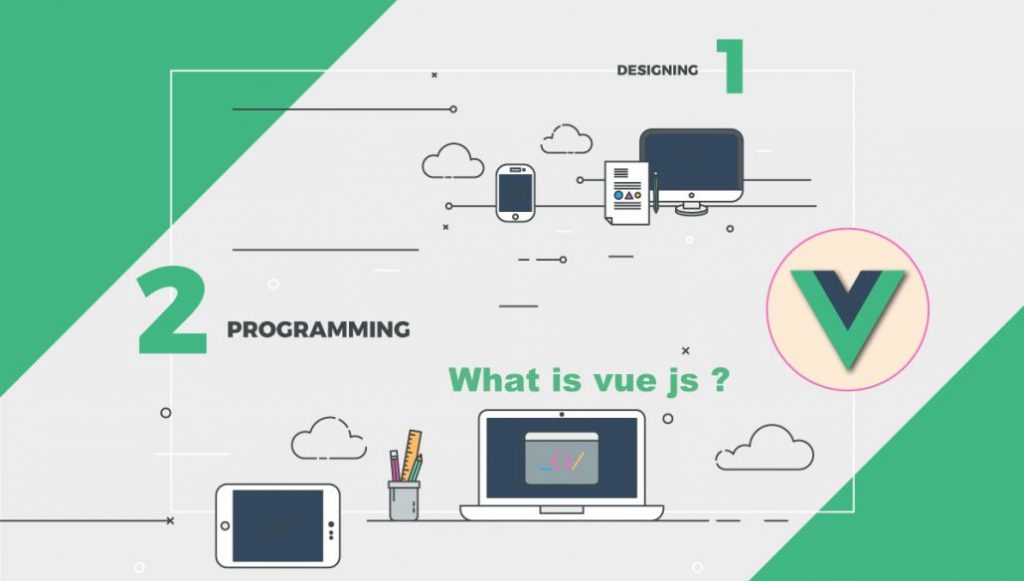
Vue js is progressive javascript script used to create dynamic user interfaces.Vue js is very easy to learn.In order to work with Vue js you just need to add few dynamic features to a website.You don’t need to install any thing to use Vue js just need add Vue js library in your project.
2. How to create an instance of Vue.js?
Answer:
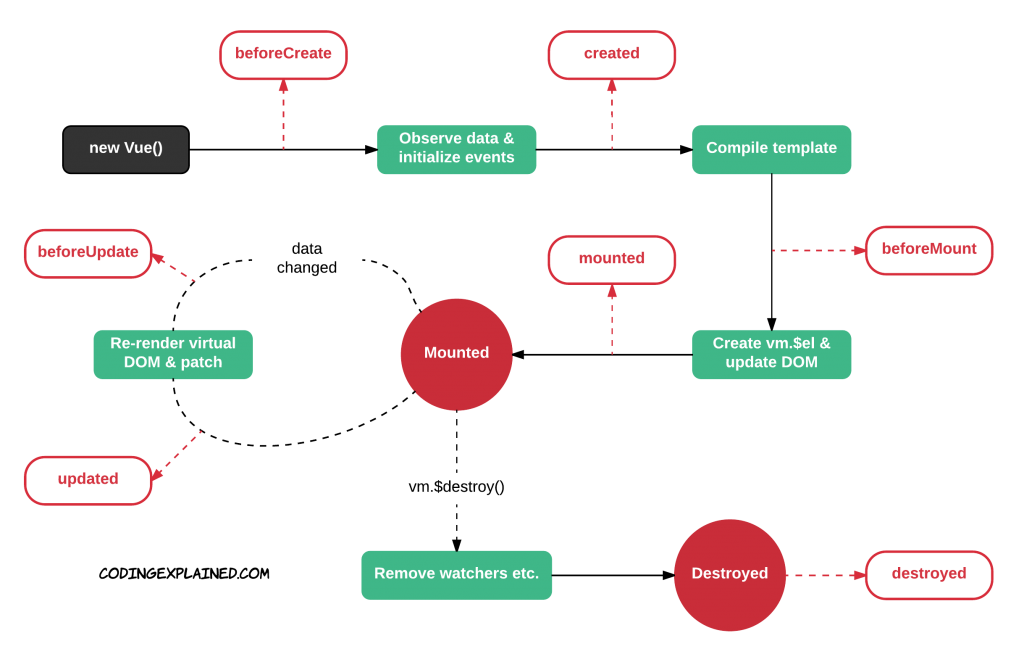
Every Vue application starts by creating a new Vue instance with the Vue function:
var vm = new Vue({
// options
})
3. What are Components in Vue.js?
Answer:
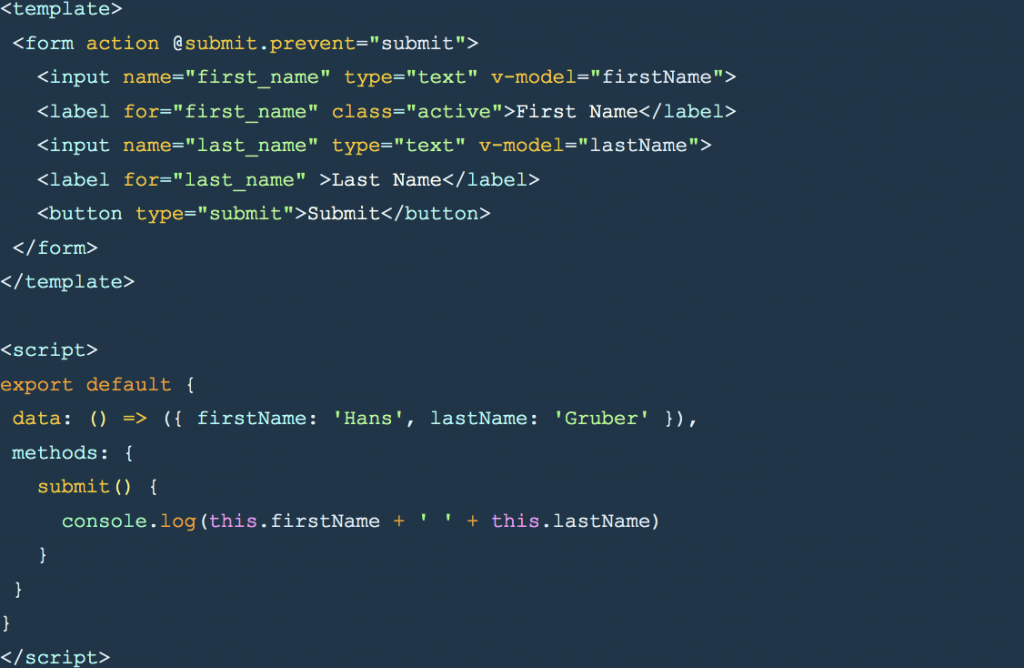
Components are one of most powerful features of Vue js.In Vue components are custom elements that help extend basic HTML elements to encapsulate reusable code.
Following is the way to register a Vue component inside another component:
export default {
el: '#your-element'
components: {
'your-component'
}
}
4. What are filters in Vue.js?
Answer:
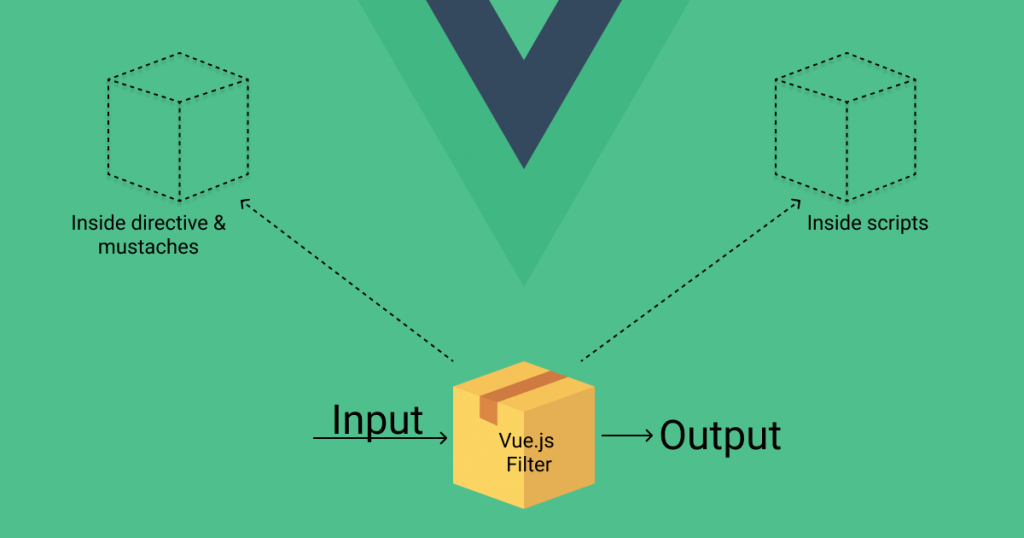
In Vue js filters are used to transform the output that are going to rendered on browser.
A Vue.js filter is essentially a function that takes a value, processes it, and then returns the processed value. In the markup it is denoted by a single pipe (|) and can be followed by one or more arguments:
<element directive="expression | filterId \[args...\]"></element>
5. What are Directives in Vue.js, List some of them you used?
Answer:
The concept of directive in Vue js is drastically simpler than that in Angular. Vue.js directives provides a way to extend HTML with new attributes and tags. Vue.js has a set of built-in directives which offers extended functionality to your applications.You can also write your custom directives in Vue.js .
Below are list of commonly used directives in Vue.js
v-show
v-if
v-model
v-else
v-on
6. How can I fetch query parameters in Vue.js?
Answer:
You have access to a $route object from your components, that expose what we need.
//from your component
console.log(this.$route.query.test)
7. Explain the basic logical Vue.js app organisation
Answer:
A Vue.js application consists of a root Vue instance created with new Vue, optionally organized into a tree of nested, reusable components. For example, a todo app’s component tree might look like this:
Root Instance
└─ TodoList
├─ TodoItem
│ ├─ DeleteTodoButton
│ └─ EditTodoButton
└─ TodoListFooter
├─ ClearTodosButton
└─ TodoListStatistics
All Vue components are also Vue instances.
8. Explain the differences between one-way data flow and two-way data binding?
Answer:
In one-way data flow the view(UI) part of application does not updates automatically when data Model is change we need to write some custom code to make it updated every time a data model is changed. In Vue js v-bind is used for one-way data flow or binding.
In two-way data binding the view(UI) part of application automatically updates when data Model is changed. In Vue.js v-model directive is used for two way data binding.
9. List some features of Vue.js
Answer:
Vue js comes with following features
Templates
Reactivity
Components
Transitions
Routing
10. How to create Two-Way Bindings in Vue.js?
Answer:
v-model directive is used to create Two-Way Bindings in Vue js.In Two-Way Bindings data or model is bind with DOM and Dom is binded back to model.
In below example you can see how Two-Way Bindings is implemented.
<div id="app">
{{message}}
<input v-model="message">
</div>
<script type="text/javascript">
var message = 'Vue.js is rad';
new Vue({ el: '#app', data: { message } });
</script>
11. What are the Advantages/Disadvantages of Vuejs?
Answer:
Vue.js Advantages
Easy for applications and interfaces development
Support Two-way communication as like AngularJs
Ability to control the states
Natural thought process
Vue.js Disadvantages
Limited scope
Single creator
Small developer community
12. Can I pass parameters in computer properties in Vue.js?
Answer:
You can use a method or computed function.
The method way:
<span>{{ fullName('Hi') }}</span>
methods: {
fullName(salut) {
return </span><span class="token interpolation"><span class="token interpolation-punctuation cBase">${</span>salut<span class="token interpolation-punctuation cBase">}</span></span><span class="token cString"> </span><span class="token interpolation"><span class="token interpolation-punctuation cBase">${</span><span class="token cVar">this</span><span class="token cBase">.</span>firstName<span class="token interpolation-punctuation cBase">}</span></span><span class="token cString"> </span><span class="token interpolation"><span class="token interpolation-punctuation cBase">${</span><span class="token cVar">this</span><span class="token cBase">.</span>lastName<span class="token interpolation-punctuation cBase">}</span></span><span class="token template-punctuation cString">
}
}
Computed property with a parameter way:
computed: {
fullName() {
return salut => </span><span class="token interpolation"><span class="token interpolation-punctuation cBase">${</span>salut<span class="token interpolation-punctuation cBase">}</span></span><span class="token cString"> </span><span class="token interpolation"><span class="token interpolation-punctuation cBase">${</span><span class="token cVar">this</span><span class="token cBase">.</span>firstName<span class="token interpolation-punctuation cBase">}</span></span><span class="token cString"> </span><span class="token interpolation"><span class="token interpolation-punctuation cBase">${</span><span class="token cVar">this</span><span class="token cBase">.</span>lastName<span class="token interpolation-punctuation cBase">}</span></span><span class="token template-punctuation cString">
}
}
13. What are components props?
Answer:
Every component instance has its own isolated scope. This means you cannot (and should not) directly reference parent data in a child component’s template. Data can be passed down to child components using props. Props are custom attributes you can register on a component. When a value is passed to a prop attribute, it becomes a property on that component instance.
Vue.component('blog-post', {
// camelCase in JavaScript
props: ['postTitle'],
template: '<h3>{{ postTitle }}</h3>'
})
14. How to deploy Vue.js app?
Answer:
If you’ve created your project like this:
vue init webpack myproject
Now you can run
npm run build
Then copy index.html and /dist/ folder into your website root directory. Done.
15. How to use local storage with Vue.js?
Answer:
You can just do following:
localStorage.setItem(‘YourItem’, response.data)
localStorage.getItem(‘YourItem’)
localStorage.removeItem(‘YourItem’)
16. Describe the Vuex?
Vuex acts as a centralized store for all the components in an application and ensures that the state can be mutated in a predictable way. It’s a State Management Pattern plus Library for the applications developed in Vue.js.
18. Explain the single file component.
Single File components or SFCs are like any other component except that they are self-contained in their own files. SFCs come with the following advantages.
Global Definitions – means all SFCs are registered globally so they have unique names.
Strong Templates – you can write the template code easily in SFCs instead of a single template property like in other components.
CSS Support – SFCs provide support to add CSS style to the component itself.
Preprocessors Support – can easily use preprocessors like babel, SAAS etc in SFCs.
19. What is Reactivity in Vue.js?
Reactivity – Whenever you make any changes in data value then it triggers page update to reflect data changes. Vue data property, computed property are reactive. Services in Vue.js are not reactive. For more you can visit Reactivity in Vue.js
20. Explain the Vue.js lifecycle hooks.
Every Vue instance passes through certain functions called lifecycle hooks. There are 8 lifecycle hooks for each vue.js instance.
Before Create – First lifecycle hook that’s called immediately after a vue instance has been initialized.
Created – It’s called just after the ‘Before create’ hook but the vue instance has set initial properties, data etc.
Before mount – It’s called just before the instance is mounted on DOM. At this moment the template has been compiled.
Mounted – It’s called once the template is ready with data and functional.
Before update – It’s called when any changes are made to data that requires DOM to be updated.
Updated – It’s called just after DOM has updated.
Before destroy It’s a place where you can perform resource clean up before destroying the vue instance.
destroyed – It’s get called when all vue instances have been destroyed. It will be triggered when run the destroy method on an object in code.
21. What is the Key in Vue.js?
Key is a special attribute in Vue that is used as a hint for the virtual DOM algorithm of Vue to differentiate the VNodes when defining a new node list against an old list.
If the ‘key’ attribute is not used then Vue uses an algorithm that minimizes element movement and tries to reuse the elements of the same type. But with ‘key’ attribute elements will be reordered and the elements without key are destroyed. It’s similar to $index in AngularJS. It’s mostly used with the ‘v-for’ directive.
<ul>
<li v-for="item in items" :key="item.id">...</li>
</ul>
22. Explain the difference between v-show and v-if.
v-show and v-if both are used to show or hide the elements. But there are some differences.
v-if directive is used to render a block conditionally. It has lazy behavior meaning if the initial condition is false then it will not render the block until the condition becomes true. v-if completely destroy and recreate the elements during condition change.
It has less initial render cost but high toggle cost so when your condition is not changing frequently at runtime then use v-if directive.
v-show directive is also used to render a block conditionally. v-show always renders the element. It just sets the CSS display property instead of destroying the element or block from DOM.
It has high cost of initial rendering but less cost of toggle, so when you need frequent toggle then use v-show directive.
23. What is $root in Vue.js?
$root property is used to access the root instance (new Vue()) in every subcomponent of this instance. All subcomponents can access this root instance and can use it as a global storage.
// foo will be available globally
this.$root.foo = 2
24. Explain the usage of $parent.
$parent is used to access the parent component instance in the child component. It makes the application harder to test and debug and you can not find out where mutation comes from. Similar to $parent Vue also provides a $child that gives a child component instance.
25. Describe the ref in Vue.js.
Vue.js provides the concept of props and events to communicate from parent to child and vice versa. Sometimes you need to directly access child components in JavaScript. To achieve this behavior you can use ref attribute to assign a reference ID to the child component as below.
<base-input ref=”usernameInput”></base-input>
Now you can access the ‘base-input’ instance in the component where you have defined ‘ref’.
this.$refs.usernameInput
26) What are the array detection mutation methods in Vue.js?
As the name suggests, the array detection mutation methods in Vue.js are used to modify the original array. Following is a list of array mutation methods which trigger view updates:
push()
pop()
shift()
unshift()
splice()
sort()
reverse()
When you perform any of the above mutation methods on the list, then it triggers view update.
27) What are the array detection non-mutation methods in Vue.js?
The array detection non-mutation methods in Vue.js are the methods that do not mutate the original array but always return a new array.
Following is a list of the non-mutation methods:
filter()
concat()
slice()
For example, let's take a todo list where it replaces the old array with new one based on status filter:
vmvm.todos = vm.todos.filter(function (todo) {
return todo.status.match(/Completed/)
})
28) What are the different event modifiers Vue.js provides?
Normally, JavaScript provides event.preventDefault() or event.stopPropagation() inside event handlers. We can use Vue.js methods, but these methods are meant for data logic instead of dealing with DOM events.
Vue.js provides the following event modifiers for v-on and these modifiers are directive postfixes denoted by a dot symbol.
.stop
.prevent
.capture
.self
.once
.passive
See the following example of stop modifier:
<!-- the click event's propagation will be stopped -->
<a v-on:click.stop="methodCall"></a>
Example of chain modifiers as follows:
<!-- modifiers can be chained -->
<a v-on:click.stop.prevent="doThat"></a>
29) Give an example to demonstrate how can you use event handlers?
Event handlers are used in Vue.js are similar to plain JavaScript. The method calls also support the special $event variable.
Example:
<button v-on:click="show('Welcome to VueJS world', $event)">
Submit
</button>
methods: {
show: function (message, event) {
// now we have access to the native event
if (event) event.preventDefault()
console.log(message);
}
}
30) Give an example to demonstrate how do you define custom key modifier aliases in Vue.js?
We can define custom key modifier aliases via the global config.keyCodes. Following are some guidelines for the properties:
We can’t use camelCase. Instead, we should use a kebab-case with double quotation marks.
We can define multiple values in an array format.
See the example:
Vue.config.keyCodes = {
f1: 112,
"media-play-pause": 179,
down: [40, 87]
}
Note: The use of keyCode events is deprecated, and the new browsers don't support it.
31) What are the different supported System Modifier Keys in Vue.js?
Vue.js supports the following modifiers to trigger mouse or keyboard event listeners when we press the corresponding keys. The list of supported System Modifier Keys is:
.ctrl
.alt
.shift
.meta
See the following example of a control modifier with the click event.
Example:
<!-- Ctrl + Click -->
<div @click.ctrl="doAction">Do some action here</div>
32) What is the requirement of local registration in Vue.js?
In Vue.js, local registration is required when the global registration seems not ideal. For example, suppose you are using a build system like Webpack and globally registering all components. In that case, even if we stop using a component, it could still be included in your final build. This unnecessarily increases the amount of JavaScript your users have to download. In these cases, it is better to define your components as plain JavaScript objects as follows:
var ComponentA = {/*.......*/}
var ComponentB = {/*.......*/}
var ComponentC = {/*.......*/}
After that define the components you would like to use in a components option as follows:
new Vue({
el: '#app',
components: {
'component-a': ComponentA,
'component-b': ComponentA
}
})
33) What are the different supported Mouse Button Modifiers in Vue.js?
Vue.js supports the following mouse button modifiers:
.left
.right
.middle
Example:
The usage of .right modifier as follows:
<button
v-if="button === 'right'"
v-on:mousedown.right="increment"
v-on:mousedown.left="decrement"
/>
34) What are the supported modifiers on the v-model directive in Vue.js?
Following are the three modifiers supported for the v-model directive in Vue.js:
lazy: By default, the v-model directive syncs the input with the data after each input event. We can add the lazy modifier to instead sync after change events.
<!-- synced after "change" instead of "input" -->
<input v-model.lazy="msg" >
number: The number modifier is used to our v-model when we want user input to be automatically typecast as a number. With the type="number", the value of HTML input elements always returns a string. That's why this typecast modifier is required.
<input v-model.number="age" type="number">
trim: We should add the trim modifier to our v-model when we want whitespace from user input to be trimmed automatically.
<input v-model.trim="msg">
35) When the components need a single root element?
In Vue.js 2.x version, every component must have a single root element when template has more than one element. In this case, you need to wrap the elements with a parent element.
<template>
<div class="todo-item">
<h2>{{ title }}</h2>
<div v-html="content"></div>
</div>
</template>
Otherwise, it will show an error, saying that "Component template should contain exactly one root element,"
The Vue.js 3.x version facilitates that the components now can have multiple root nodes. This way of adding multiple root nodes is called as fragments.
<template>
<h2>{{ title }}</h2>
<div v-html="content"></div>
</template>
36) What do you understand by global registration in components in Vue.js?
The global registration in components in Vue.js facilitates us to use it in the template of any root Vue instance (new Vue) created after registration.
In the global registration, the components created using Vue.component as follows:
Vue.component('my-component-name', {
// ... options ...
})
We can take multiple components which are globally registered in the vue instance,
Vue.component('component-a', { /* ... */ })
Vue.component('component-b', { /* ... */ })
Vue.component('component-c', { /* ... */ })
new Vue({ el: '#app' })
The above components can be used in the vue instance as follows:
<div id="app">
<component-a></component-a>
<component-b></component-b>
<component-c></component-c>
</div>
Note: The components can be used in subcomponents as well.
37) What is the purpose of using v-for directive in Vue.js?
In Vue.js, the v-for directive is used because it allows us to loop through items in an array or object. By using this directive, we can iterate on each element in the array or object.
Example of v-for directive usage in Array:
<ul id="list">
<li v-for="(item, index) in items">
{{ index }} - {{ item.message }}
</li>
</ul>
var vm = new Vue({
el: '#list',
data: {
items: [
{ message: 'Alex' },
{ message: 'Muler' }
]
}
})
We can also use the delimiter instead of in, similar to JavaScript iterators.
Example of v-for directive usage in Object:
<div id="object">
<div v-for="(value, key, index) of user">
{{ index }}. {{ key }}: {{ value }}
</div>
</div>
var vm = new Vue({
el: '#object',
data: {
user: {
firstName: 'Alex',
lastName: 'Muller',
age: 30
}
}
})
38) Give an example to demonstrate how do you reuse elements with key attribute?
Vue.js always facilitates us to render elements as efficiently as possible. So, it is possible to reuse the elements instead of building them from scratch. But this can create problems in few scenarios. For example, if you try to render the same input element in both v-if and v-else blocks then it holds the previous value as follows:
<template v-if="loginType === 'Admin'">
<label>Admin</label>
<input placeholder="Enter your ID">
</template>
<template v-else>
<label>Guest</label>
<input placeholder="Enter your name">
</template>
In the above case, we should not reuse it. It is better to make both input elements as separate by applying key attribute as follows:
<template v-if="loginType === 'Admin'">
<label>Admin</label>
<input placeholder="Enter your ID" key="admin-id">
</template>
<template v-else>
<label>Guest</label>
<input placeholder="Enter your name" key="user-name">
</template>
In the above example, both inputs are independent and do not impact each other.
39) Why is it recommended to use a key attribute for directive?
It is recommended to use a key attribute for a directive to track each node’s identity and thus reuse and reorder existing elements. We have to provide a unique key attribute for each item with in v-for iteration. An ideal value for the key would be the unique id of each item. Example:
<div v-for="item in items" :key="item.id">
{{item.name}}
</div>
That's why it is always recommended to provide a key with v-for whenever possible unless the iterated DOM content is simple.
Note: We should use string or numeric values instead of non-primitive values like objects and arrays.
40) What do you understand by the array detection non-mutation methods?
The methods that do not mutate the original array but always return a new array are known as non-mutation methods.
Following is a list of the non-mutation methods:
filter() method
concat() method
slice() method
Let's take an example to understand it better. We have a todo list replacing the old array with a new one based on the status filter.
Example:
vmvm.todos = vm.todos.filter(function (todo) {
return todo.status.match(/Completed/)
})
This approach would not re-render the entire list due to Vue.js implementation.
41) How can you redirect to another page in Vue.js?
In Vue.js, if you are using vue-router, you should use router.go(path) to navigate to any particular route. You can access the router from within a component using this.$router. router.go() changed in Vue.js 2.0. You can use router.push({ name: “yourroutename”}) or just router.push(“yourroutename”) now to redirect.
42) What do you understand by slots in Vue.js?
In Vue.js, the <slot> element is used to serve as distribution outlets for content.
Let’s take an example to create an alert component with slots for content insertion.
Example:
In Vue.js, the <slot> element is used to serve as distribution outlets for content.
Let’s take an example to create an alert component with slots for content insertion.
Example:
Vue.component('alert', {
template: `
<div class="alert-box">
<strong>Error!</strong>
<slot></slot>
</div>`
})
We can insert dynamic content as follows:
<alert>
There is an issue with in application.
</alert>
43) What are the problems solved by Single File Components in Vue.js?
In Vue.js, the Single File Components are used to solve the common problems in a JavaScript-driven application with a .vue extension.
Following is a list of issues solved by Single File Components in Vue.js:
Global definitions specify unique names for every component.
String templates lack syntax highlighting and require ugly slashes for multiline HTML.
No CSS support. It means while HTML and JavaScript are modularized into components, CSS is conspicuously left out.
No, build step restrictions to HTML and ES5 JavaScript, rather than preprocessors like Pug and Babel.
44) What are the different ways to create filters?
<
There are two ways to define filters:
Local filters: You can define local filters in a component’s options. In this case, filter is applicable to that specific component.
filters: {
capitalize: function (value) {
if (!value) return ''
valuevalue = value.toString()
return value.charAt(0).toUpperCase() + value.slice(1)
}
}
Global filters: You can also define a filter globally before creating the Vue instance. In this case, filter is applicable to all the components within the vue instance,
Vue.filter('capitalize', function (value) {
if (!value) return ''
valuevalue = value.toString()
return value.charAt(0).toUpperCase() + value.slice(1)
})
new Vue({
// ...
})
45) What do you understand by mapState helper?
In the Vuex application, creating a computed property every time whenever we want to access the store’s state property or getter is going to be repetitive, difficult, and boring, especially if a component needs more than one state property. In this situation, we can use the mapState helper of vuex, which generates computed getter functions for us.
In the following increment example, we have demonstrated the mapState helper:
import { mapState } from 'vuex'
export default {
// ...
computed: mapState({
// arrow functions can make the code very succinct!
username: state => state.username,
// passing the string value 'username' is same as `state => state.username`
usernameAlias: 'username',
// to access local state with `this`, a normal function must be used
greeting (state) {
return this.localTitle + state.username
}
})
}
You can also pass a string array to mapState when the name of a mapped computed property is the same as a state sub-tree name
computed: mapState([
// map this.username to store.state.username
'username'
])
46) What are the most prominent features of stylelint?
Following is a list of the most prominent features of stylelint:
- The stylelint has more than 160 built-in rules to catch errors, apply limits and enforce stylistic conventions.
- It understands the latest CSS syntax, including custom properties and level 4 selectors.
- It extracts the embedded styles from HTML, markdown, and CSS-in-JS object & template literals.
- It is also used to parse CSS-like syntaxes like SCSS, Sass, Less, and SugarSS.
- It supports for reusing community plugins and creating their plugins.
47) What is a single-file component in Vue.js?
In Vue.js, a single-file component is a file with a .vue extension that contains a Vue component. The single-file component consists of the component’s template, logic, and styles, all bundled together in one file. It also contains one <script> block, optional <template> and <style> blocks, and possible additional custom blocks.
48) How to use a single-file component in Vue.js?
To use a single-file component in Vue.js, we have to set up Vue Loader for parsing the file (It is done automatically as a part of a webpack building pipeline). It also supports non-default languages such as Sass or HTML templating languages with pluggable pre-processors.
49) What is $parent property in Vue.js?
In Vue.js, the $parent property is used to access the parent instance from a child. It is similar to the $root property. The $parent property provides direct access, but it makes the application hard to test and debug. In this property, it is very difficult to find out where the mutation comes from.
50) What is $child property in Vue.js?
In Vue.js, the $child property is just like $parent property, but it is used to access the child instance.
- Mastering Qualitative Research: The Role of Focus Groups in Data Collection - July 11, 2024
- What is robots ops? - July 10, 2024
- 5 Effective Online Learning Strategies for DevOps Professionals - July 4, 2024