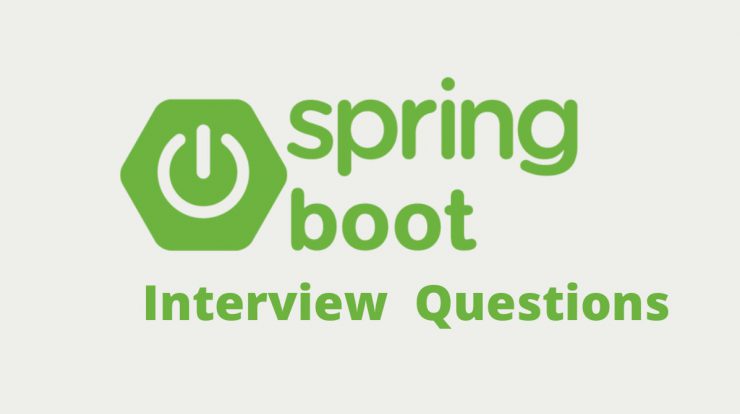
1. What is Spring Boot?
Spring Boot is a framework that makes it easy to create stand-alone, production-grade Spring applications. It provides a number of features that make it easier to develop, run, and deploy Spring applications, including:
- Auto-configuration: Spring Boot automatically configures many of the components that are needed to run a Spring application.
- Embedded servers: Spring Boot includes embedded servers that can be used to run Spring applications without the need for a separate web server.
- Starter dependencies: Spring Boot provides starter dependencies that make it easy to add common features to Spring applications.
2. What are the benefits of using Spring Boot?
There are many benefits to using Spring Boot, including:
- Ease of development: Spring Boot makes it easy to develop Spring applications by automatically configuring many of the components that are needed.
- Faster development: Spring Boot can help you to develop Spring applications faster by providing starter dependencies and embedded servers.
- Production-ready: Spring Boot applications are production-ready out of the box, so you can focus on developing your application instead of configuring the underlying infrastructure.
- Scalability: Spring Boot applications are scalable, so you can easily add new features or users without having to make major changes to your application.
3. What are the differences between Spring Boot and Spring?
Spring Boot is a framework that builds on top of Spring. It provides a number of features that make it easier to develop, run, and deploy Spring applications. Spring, on the other hand, is a more general-purpose framework that provides a wider range of features.
4. What are the different ways to create a Spring Boot project?
There are a few different ways to create a Spring Boot project, including:
- Using the Spring Boot CLI
- Using the Spring Initializr
- Using an IDE
5. What are the different ways to run a Spring Boot application?
There are a few different ways to run a Spring Boot application, including:
- Running the application from the command line
- Running the application from an IDE
- Deploying the application to a production environment
6. What are the different types of Spring Boot starters?
Spring Boot starters are dependencies that make it easy to add common features to Spring applications. There are a number of different types of Spring Boot starters, including:
- Web starters: Web starters make it easy to add web functionality to Spring applications.
- Data access starters: Data access starters make it easy to add data access functionality to Spring applications.
- Security starters: Security starters make it easy to add security functionality to Spring applications.
7. What are the different types of Spring Boot actuators?
Spring Boot actuators are endpoints that provide information about a running Spring Boot application. There are a number of different types of Spring Boot actuators, including:
- Health actuator: The health actuator provides information about the health of a running Spring Boot application.
- Metrics actuator: The metrics actuator provides metrics about a running Spring Boot application.
- Audit actuator: The audit actuator provides audit logs about a running Spring Boot application.
8. What are the different ways to configure a Spring Boot application?
There are a few different ways to configure a Spring Boot application, including:
- Using the application.properties file
- Using the application.yml file
- Using environment variables
9. What are the different ways to test a Spring Boot application?
There are a few different ways to test a Spring Boot application, including:
- Unit testing
- Integration testing
- System testing
10. What are the different ways to deploy a Spring Boot application?
There are a few different ways to deploy a Spring Boot application, including:
- Deploying to a local development environment
- Deploying to a staging environment
- Deploying to a production environment
11. What is the Spring Boot CLI?
The Spring Boot CLI is a command-line tool that can be used to create, run, and manage Spring Boot applications. It provides a number of features that make it easier to develop Spring Boot applications, including:
- Auto-completion: The Spring Boot CLI provides auto-completion for Spring Boot commands and properties.
- Command aliases: The Spring Boot CLI allows you to create command aliases to make it easier to run common commands.
- Documentation: The Spring Boot CLI provides documentation for Spring Boot commands and properties.
12. What is the Spring Initializr?
The Spring Initializr is a web-based tool that can be used to create Spring Boot projects. It provides a number of features that make it easier to create Spring Boot projects, including:
- A selection of starter dependencies: The Spring Initializr allows you to select a number of starter dependencies to add to your project.
- A configuration wizard: The Spring Initializr provides a configuration wizard that helps you to configure your project.
- A download link: The Spring Initializr provides a download link for your project.
13. What are the different types of Spring Boot profiles?
Spring Boot profiles are used to configure different environments for a Spring Boot application. There are a few different types of Spring Boot profiles, including:
- Development profiles: Development profiles are used to configure a Spring Boot application for development environments.
- Production profiles: Production profiles are used to configure a Spring Boot application for production environments.
- Staging profiles: Staging profiles are used to configure a Spring Boot application for staging environments.
14. What are the different ways to manage Spring Boot applications?
There are a few different ways to manage Spring Boot applications, including:
- Using the Spring Boot CLI
- Using a build automation tool
- Using a cloud-based service
15. What are some of the best practices for developing Spring Boot applications?
There are a few best practices that can be followed when developing Spring Boot applications, including:
- Use starter dependencies: Starter dependencies make it easy to add common features to Spring Boot applications.
- Use the Spring Boot CLI: The Spring Boot CLI can be used to create, run, and manage Spring Boot applications.
- Use the Spring Initializr: The Spring Initializr can be used to create Spring Boot projects.
- Use profiles: Profiles can be used to configure different environments for a Spring Boot application.
- Use a build automation tool: A build automation tool can be used to automate the build and deployment process for a Spring Boot application.
- Use a cloud-based service: A cloud-based service can be used to deploy and manage Spring Boot applications.
16. What are some of the challenges of developing Spring Boot applications?
There are a few challenges that can be encountered when developing Spring Boot applications, including:
- Understanding the Spring Boot ecosystem: The Spring Boot ecosystem is large and complex, so it can be difficult to understand all of the components that are available.
- Choosing the right starter dependencies: There are a large number of starter dependencies available, so it can be difficult to choose the right ones for your application.
- Configuring profiles: Profiles can be complex to configure, and it can be difficult to ensure that they are configured correctly.
- Deploying Spring Boot applications: Spring Boot applications can be deployed to a variety of environments, so it can be difficult to choose the right deployment strategy.
17. What are some of the best resources for learning more about Spring Boot?
There are a number of resources that can be used to learn more about Spring Boot, including:
- The Spring Boot website: The Spring Boot website provides documentation, tutorials, and other resources for learning about Spring Boot.
- The Spring Boot blog: The Spring Boot blog provides news and updates about Spring Boot.
- The Spring Boot community: The Spring Boot community is a forum where you can ask questions and get help from other Spring Boot developers.
- The Spring Boot GitHub repository: The Spring Boot GitHub repository contains the source code for Spring Boot.
18. What is the difference between Spring Boot and Spring MVC?
Spring Boot is a framework that makes it easy to create stand-alone, production-grade Spring applications. Spring MVC is a web framework that can be used to create web applications with Spring.
The main difference between Spring Boot and Spring MVC is that Spring Boot is a more opinionated framework. Spring Boot provides a number of default configurations that make it easy to create production-ready applications. Spring MVC, on the other hand, is a more flexible framework. Spring MVC allows you to configure the framework to your specific needs.
19. What are some of the advantages of using Spring Boot?
There are many advantages to using Spring Boot, including:
- Ease of development: Spring Boot makes it easy to develop Spring applications by automatically configuring many of the components that are needed.
- Faster development: Spring Boot can help you to develop Spring applications faster by providing starter dependencies and embedded servers.
- Production-ready: Spring Boot applications are production-ready out of the box, so you can focus on developing your application instead of configuring the underlying infrastructure.
- Scalability: Spring Boot applications are scalable, so you can easily add new features or users without having to make major changes to your application.
20. What are some of the challenges of using Spring Boot?
There are a few challenges that can be encountered when using Spring Boot, including:
- Understanding the Spring Boot ecosystem: The Spring Boot ecosystem is large and complex, so it can be difficult to understand all of the components that are available.
- Choosing the right starter dependencies: There are a large number of starter dependencies available, so it can be difficult to choose the right ones for your application.
- Configuring profiles: Profiles can be complex to configure, and it can be difficult to ensure that they are configured correctly.
- Deploying Spring Boot applications: Spring Boot applications can be deployed to a variety of environments, so it can be difficult to choose the right deployment strategy.
21. What are some of the best practices for using Spring Boot?
There are a few best practices that can be followed when using Spring Boot, including:
- Use starter dependencies: Starter dependencies make it easy to add common features to Spring Boot applications.
- Use the Spring Boot CLI: The Spring Boot CLI can be used to create, run, and manage Spring Boot applications.
- Use the Spring Initializr: The Spring Initializr can be used to create Spring Boot projects.
- Use profiles: Profiles can be used to configure different environments for a Spring Boot application.
- Use a build automation tool: A build automation tool can be used to automate the build and deployment process for a Spring Boot application.
- Use a cloud-based service: A cloud-based service can be used to deploy and manage Spring Boot applications.
22. What are some of the most popular Spring Boot projects?
Some of the most popular Spring Boot projects include:
- Spring Boot Admin: https://github.com/codecentric/spring-boot-admin: A monitoring and management tool for Spring Boot applications.
- Spring Cloud: https://spring.io/projects/spring-cloud: A set of tools for building microservices-based applications.
- Spring Data: https://spring.io/projects/spring-data: A set of libraries for accessing data with Spring Boot.
- Spring Security: https://spring.io/projects/spring-security: A framework for securing Spring Boot applications.
23. What are some of the most common Spring Boot mistakes?
Some of the most common Spring Boot mistakes include:
- Not using starter dependencies: Starter dependencies make it easy to add common features to Spring Boot applications. Not using them can make your application more difficult to develop and maintain.
- Not using profiles: Profiles can be used to configure different environments for a Spring Boot application. Not using them can make your application difficult to deploy to different environments.
- Not using a build automation tool: A build automation tool can be used to automate the build and deployment process for a Spring Boot application. Not using one can make your application more difficult to build and deploy.
- Not using a cloud-based service: A cloud-based service can be used to deploy and manage Spring Boot applications. Not using one can make your application more difficult to deploy and manage.
24. What are some of the best ways to learn more about Spring Boot?
There are a number of resources that can be used to learn more about Spring Boot, including:
- The Spring Boot website: The Spring Boot website provides documentation, tutorials, and other resources for learning about Spring Boot.
- The Spring Boot blog: The Spring Boot blog provides news and updates about Spring Boot.
- The Spring Boot community: The Spring Boot community is a forum where you can ask questions and get help from other Spring Boot developers.
- The Spring Boot GitHub repository: The Spring Boot GitHub repository contains the source code for Spring Boot.
25. What are the future trends for Spring Boot?
Spring Boot is a rapidly evolving framework, and there are a number of future trends that can be expected for Spring Boot. These trends include:
- The continued growth of the Spring Boot ecosystem: The Spring Boot ecosystem is constantly growing, and there are a number of new projects and components being added all the time.
- The increasing popularity of microservices: Spring Boot is a popular framework for building microservices, and this trend is expected to continue in the future.
- The growing adoption of cloud-based services: Spring Boot is a good fit for cloud-based deployments, and this trend is expected to continue in the future.
26. What are some of the benefits of using Spring Boot for microservices?
Spring Boot is a good fit for microservices because it makes it easy to:
- Develop microservices: Spring Boot provides a number of features that make it easy to develop microservices, such as starter dependencies and embedded servers.
- Deploy microservices: Spring Boot makes it easy to deploy microservices to a variety of environments, such as cloud-based services.
- Manage microservices: Spring Boot provides a number of features that make it easy to manage microservices, such as actuators and profiles.
27. What are some of the challenges of using Spring Boot for microservices?
Some of the challenges of using Spring Boot for microservices include:
- Complexity: Microservices can be complex to develop and manage, and Spring Boot can add to this complexity.
- Security: Microservices can be difficult to secure, and Spring Boot does not provide a lot of security features out of the box.
- Testing: Microservices can be difficult to test, and Spring Boot does not provide a lot of testing features out of the box.
28. What are some best practices for using Spring Boot for microservices?
Some best practices for using Spring Boot for microservices include:
- Use a service discovery mechanism: A service discovery mechanism can be used to dynamically discover microservices.
- Use a load balancer: A load balancer can be used to distribute traffic across microservices.
- Use a centralized logging mechanism: A centralized logging mechanism can be used to collect logs from microservices.
- Use a centralized configuration mechanism: A centralized configuration mechanism can be used to manage configuration for microservices.
29. What are some of the most popular Spring Boot microservices frameworks?
Some of the most popular Spring Boot microservices frameworks include:
- Spring Cloud: Spring Cloud is a set of tools for building microservices-based applications.
- Netflix OSS: Netflix OSS is a set of libraries for building microservices-based applications.
- Apache Camel: Apache Camel is a framework for routing and transforming messages.
- Apache Thrift: Apache Thrift is a framework for RPC.
30. What are the future trends for Spring Boot microservices?
Spring Boot microservices is a rapidly evolving field, and there are a number of future trends that can be expected. These trends include:
- The continued growth of the Spring Boot ecosystem: The Spring Boot ecosystem is constantly growing, and there are a number of new projects and components being added all the time.
- The increasing popularity of cloud-based services: Spring Boot microservices are a good fit for cloud-based deployments, and this trend is expected to continue in the future.
- The growing adoption of event-driven architectures: Event-driven architectures are becoming increasingly popular, and Spring Boot microservices are a good fit for this type of architecture.
31. What are some of the benefits of using Spring Boot for cloud-based deployments?
Spring Boot is a good fit for cloud-based deployments because it makes it easy to:
- Deploy microservices: Spring Boot makes it easy to deploy microservices to a variety of cloud-based services, such as Amazon Web Services (AWS) and Microsoft Azure.
- Manage microservices: Spring Boot provides a number of features that make it easy to manage microservices in the cloud, such as actuators and profiles.
- Scale microservices: Spring Boot makes it easy to scale microservices in the cloud, as needed.
32. What are some of the challenges of using Spring Boot for cloud-based deployments?
Some of the challenges of using Spring Boot for cloud-based deployments include:
- Cost: Cloud-based deployments can be expensive, and Spring Boot can add to this cost.
- Security: Cloud-based deployments can be more difficult to secure, and Spring Boot does not provide a lot of security features out of the box.
- Performance: Cloud-based deployments can be more demanding on performance, and Spring Boot can add to this demand.
33. What are some best practices for using Spring Boot for cloud-based deployments?
Some best practices for using Spring Boot for cloud-based deployments include:
- Use a cloud-native architecture: A cloud-native architecture can help you to take advantage of the benefits of cloud-based deployments.
- Use a cloud-based service discovery mechanism: A cloud-based service discovery mechanism can help you to dynamically discover microservices in the cloud.
- Use a cloud-based load balancer: A cloud-based load balancer can help you to distribute traffic across microservices in the cloud.
- Use a cloud-based logging mechanism: A cloud-based logging mechanism can help you to collect logs from microservices in the cloud.
- Use a cloud-based configuration mechanism: A cloud-based configuration mechanism can help you to manage configuration for microservices in the cloud.
34. What are some of the most popular Spring Boot cloud-based deployment frameworks?
Some of the most popular Spring Boot cloud-based deployment frameworks include:
- Spring Cloud: Spring Cloud is a set of tools for building microservices-based applications.
- Netflix OSS: Netflix OSS is a set of libraries for building microservices-based applications.
- Amazon Web Services (AWS): AWS is a cloud-based platform that provides a variety of services, including Elastic Beanstalk and Amazon Relational Database Service (RDS).
- Microsoft Azure: Azure is a cloud-based platform that provides a variety of services, including App Service and SQL Database.
35. What are the future trends for Spring Boot cloud-based deployments?
Spring Boot cloud-based deployments is a rapidly evolving field, and there are a number of future trends that can be expected. These trends include:
- The continued growth of the Spring Boot ecosystem: The Spring Boot ecosystem is constantly growing, and there are a number of new projects and components being added all the time.
- The increasing popularity of serverless computing: Serverless computing is a new paradigm for cloud-based deployments, and Spring Boot is a good fit for this type of deployment.
- The growing adoption of containers: Containers are becoming increasingly popular for cloud-based deployments, and Spring Boot is a good fit for this type of deployment.
36. What is the difference between Spring Boot and Spring Cloud?
Spring Boot and Spring Cloud are both frameworks that can be used to build microservices-based applications. However, there are a few key differences between the two frameworks.
- Spring Boot: Spring Boot is a framework that makes it easy to create stand-alone, production-grade Spring applications. Spring Boot provides a number of default configurations that make it easy to create production-ready applications.
- Spring Cloud: Spring Cloud is a set of tools that can be used to build microservices-based applications. Spring Cloud provides a number of features that make it easier to build and manage microservices, such as service discovery, load balancing, and configuration management.
In general, Spring Boot is a good choice for developers who want to create simple, stand-alone Spring applications. Spring Cloud is a good choice for developers who want to build more complex microservices-based applications.
37. What are some of the most popular Spring Cloud components?
Some of the most popular Spring Cloud components include:
- Spring Cloud Config: Spring Cloud Config is a component that provides a centralized configuration management system for microservices.
- Spring Cloud Netflix: Spring Cloud Netflix is a component that provides a number of features for building microservices-based applications, such as service discovery, load balancing, and circuit breakers.
- Spring Cloud Sleuth: Spring Cloud Sleuth is a component that provides tracing for microservices-based applications.
- Spring Cloud Bus: Spring Cloud Bus is a component that provides a messaging system for microservices-based applications.
38. What are the future trends for Spring Cloud?
Spring Cloud is a rapidly evolving framework, and there are a number of future trends that can be expected. These trends include:
- The continued growth of the Spring Cloud ecosystem: The Spring Cloud ecosystem is constantly growing, and there are a number of new projects and components being added all the time.
- The increasing popularity of microservices: Microservices are becoming increasingly popular, and Spring Cloud is a good fit for this type of architecture.
- The growing adoption of cloud-based deployments: Spring Cloud is a good fit for cloud-based deployments, and this trend is expected to continue in the future.
39. What are some best practices for using Spring Cloud?
Some best practices for using Spring Cloud include:
- Use a cloud-native architecture: A cloud-native architecture can help you to take advantage of the benefits of Spring Cloud.
- Use a service discovery mechanism: A service discovery mechanism can help you to dynamically discover microservices in a cloud-based environment.
- Use a load balancer: A load balancer can help you to distribute traffic across microservices in a cloud-based environment.
- Use a logging mechanism: A logging mechanism can help you to collect logs from microservices in a cloud-based environment.
- Use a configuration management system: A configuration management system can help you to manage configuration for microservices in a cloud-based environment.
40. What are some of the challenges of using Spring Cloud?
Some of the challenges of using Spring Cloud include:
- Complexity: Spring Cloud can be complex to configure and use.
- Security: Spring Cloud can be difficult to secure.
- Testing:Â Spring Cloud can be difficult to test.
41. What are some of the best resources for learning more about Spring Cloud?
Here are some of the best resources for learning more about Spring Cloud:
- Spring Cloud website: The Spring Cloud website provides documentation, tutorials, and other resources for learning about Spring Cloud.Opens in a new windowspring.ioSpring Cloud website
- Spring Cloud blog: The Spring Cloud blog provides news and updates about Spring Cloud.Opens in a new windowsysdig.comSpring Cloud blog
- Spring Cloud community: The Spring Cloud community is a forum where you can ask questions and get help from other Spring Cloud developers.Opens in a new windowdataflow.spring.ioSpring Cloud community forum
- Spring Cloud GitHub repository: The Spring Cloud GitHub repository contains the source code for Spring Cloud.Opens in a new windowwww.thetechnojournals.comSpring Cloud GitHub repository
42. What are some of the most popular Spring Boot projects?
Here are some of the most popular Spring Boot projects:
- Spring Boot Admin: Spring Boot Admin is a monitoring and management tool for Spring Boot applications.Opens in a new windowwww.tutorialspoint.comSpring Boot Admin website
- Spring Cloud: Spring Cloud is a set of tools for building microservices-based applications.Opens in a new windowspring.ioSpring Cloud website
- Spring Data: Spring Data is a set of libraries for accessing data with Spring Boot.Opens in a new windowtechnicalsand.comSpring Data website
- Spring Security: Spring Security is a framework for securing Spring Boot applications.Opens in a new windowwww.kindsonthegenius.comSpring Security website
43. What are some of the most common Spring Boot mistakes?
Here are some of the most common Spring Boot mistakes:
- Not using starter dependencies: Starter dependencies make it easy to add common features to Spring Boot applications. Not using them can make your application more difficult to develop and maintain.
- Not using profiles: Profiles can be used to configure different environments for a Spring Boot application. Not using them can make your application difficult to deploy to different environments.
- Not using a build automation tool: A build automation tool can be used to automate the build and deployment process for a Spring Boot application. Not using one can make your application more difficult to build and deploy.
- Not using a cloud-based service: A cloud-based service can be used to deploy and manage Spring Boot applications. Not using one can make your application more difficult to deploy and manage.
44. What are some of the best ways to learn more about Spring Boot?
Here are some of the best ways to learn more about Spring Boot:
- Read the Spring Boot documentation: The Spring Boot documentation provides a comprehensive overview of Spring Boot.
- Take a Spring Boot tutorial: There are a number of Spring Boot tutorials available online.
- Join the Spring Boot community: The Spring Boot community is a great resource for getting help and support with Spring Boot.
- Contribute to the Spring Boot project: If you are interested in contributing to the Spring Boot project, you can do so by forking the GitHub repository and submitting pull requests.
45. What are some of the future trends for Spring Boot?
Spring Boot is a rapidly evolving framework, and there are a number of future trends that can be expected. These trends include:
- The continued growth of the Spring Boot ecosystem: The Spring Boot ecosystem is constantly growing, and there are a number of new projects and components being added all the time.
- The increasing popularity of microservices: Microservices are becoming increasingly popular, and Spring Boot is a good fit for this type of architecture.
- The growing adoption of cloud-based deployments: Spring Boot is a good fit for cloud-based deployments, and this trend is expected to continue in the future.
- The increasing use of Kotlin: Kotlin is a modern programming language that is gaining popularity, and it is well-suited for use with Spring Boot.
- The increasing use of artificial intelligence (AI) and machine learning (ML): AI and ML are becoming increasingly important, and Spring Boot is well-suited for use with these technologies.
46. What are some of the challenges of using Spring Boot?
Some of the challenges of using Spring Boot include:
- Complexity: Spring Boot can be complex to configure and use.
- Security: Spring Boot can be difficult to secure.
- Testing: Spring Boot can be difficult to test.
- Community: The Spring Boot community is not as large as some other Java communities.
47. What are some of the best practices for using Spring Boot?
Some best practices for using Spring Boot include:
- Use starter dependencies: Starter dependencies make it easy to add common features to Spring Boot applications.
- Use profiles: Profiles can be used to configure different environments for a Spring Boot application.
- Use a build automation tool: A build automation tool can be used to automate the build and deployment process for a Spring Boot application.
- Use a cloud-based service: A cloud-based service can be used to deploy and manage Spring Boot applications.
- Use a version control system: A version control system can be used to track changes to your Spring Boot application.
- Test your application thoroughly: It is important to test your Spring Boot application thoroughly before deploying it to production.
48. What are some of the most common Spring Boot interview questions?
Here are some of the most common Spring Boot interview questions:
- What is Spring Boot?
- What are the benefits of using Spring Boot?
- What are the challenges of using Spring Boot?
- What are some of the most popular Spring Boot projects?
- What are some of the best resources for learning more about Spring Boot?
- What are some of the most common Spring Boot mistakes?
- What are some of the best ways to learn more about Spring Boot?
- What are some of the future trends for Spring Boot?
- What is Spring Boot? Spring Boot is an opinionated framework built on top of the Spring framework that simplifies the process of developing and deploying production-ready applications with minimal configuration.
- What are the key features of Spring Boot? Key features of Spring Boot include auto-configuration, embedded server support, starter dependencies, production-ready metrics, and monitoring.
- What is the difference between Spring and Spring Boot? Spring is a larger framework that provides various modules like Spring MVC, Spring Data, etc., while Spring Boot is specifically designed to simplify Spring application development by providing default configurations and reducing boilerplate code.
- Explain the concept of Auto-configuration in Spring Boot. Auto-configuration automatically configures beans based on classpath dependencies and other configurations. It allows developers to focus on writing business logic rather than worrying about setting up infrastructure.
- What are Spring Boot Starters? Spring Boot Starters are a set of convenient dependency descriptors that simplify the inclusion of various libraries and frameworks in your application.
- How can you create a Spring Boot application? You can create a Spring Boot application either using Spring Initializr (web-based) or by configuring dependencies manually in your build configuration (e.g., Maven or Gradle).
- How do you define configuration properties in Spring Boot? Configuration properties can be defined in the application.properties or application.yml file, and they can be accessed in the application using the
@Value
annotation or@ConfigurationProperties
annotation. - Explain the difference between @Component, @Service, @Repository, and @Controller annotations in Spring Boot. These annotations are used for component scanning and bean registration. @Component is a generic stereotype for any Spring-managed component, @Service is used for service layer classes, @Repository is used for DAO classes, and @Controller is used for Spring MVC controller classes.
- How does Spring Boot support database connectivity? Spring Boot provides easy database connectivity through Spring Data JPA, which simplifies database access and reduces boilerplate code using its repositories.
- What is the significance of the @SpringBootApplication annotation? @SpringBootApplication is a composite annotation that includes @SpringBootConfiguration, @EnableAutoConfiguration, and @ComponentScan. It is used to enable Spring Boot features in the application.
- Explain the use of the @RestController annotation. The @RestController annotation is a combination of @Controller and @ResponseBody. It is used to indicate that the response of a request mapping method should be directly written to the HTTP response body.
- How can you enable cross-origin requests (CORS) in Spring Boot? You can enable CORS in Spring Boot by adding the @CrossOrigin annotation at the controller level or by configuring it globally using WebMvcConfigurer.
- What is the difference between @RequestParam and @PathVariable annotations? @RequestParam is used to extract query parameters from the request URL, while @PathVariable is used to extract values from the URI path.
- How can you handle exceptions in Spring Boot? You can handle exceptions in Spring Boot using the
@ExceptionHandler
annotation, global exception handling with@ControllerAdvice
, or by defining custom exception classes. - Explain the role of the @Autowired annotation. The @Autowired annotation is used to automatically wire dependencies (beans) into the Spring-managed components.
- What is Spring Boot Actuator? Spring Boot Actuator provides production-ready features like health checks, metrics, and monitoring to monitor and manage your application.
- How can you enable Spring Boot Actuator? Spring Boot Actuator is enabled by adding the
spring-boot-starter-actuator
dependency in the project’s build configuration. - What are the various ways to configure data sources in Spring Boot? You can configure data sources in Spring Boot through the application.properties or application.yml file or by programmatically creating the data source bean.
- Explain the Spring Boot bean lifecycle. Spring Boot follows the standard Spring bean lifecycle, which involves bean instantiation, initialization, and destruction. Spring Boot manages the lifecycle automatically.
- What is the purpose of the SpringApplication class? The SpringApplication class is used to bootstrap and launch a Spring Boot application.
- How can you profile a Spring Boot application? You can profile a Spring Boot application by using different application properties files, such as application-dev.properties, application-prod.properties, etc.
- How do you externalize configuration in Spring Boot? Configuration can be externalized in Spring Boot by using external properties files or by using environment variables.
- Explain the concept of Spring Boot Actuator endpoints. Actuator endpoints are HTTP endpoints provided by Spring Boot Actuator, which expose useful information about the application, such as health, metrics, and environment details.
- What is the purpose of the @SpringBootTest annotation? The @SpringBootTest annotation is used to test Spring Boot applications and provide the application context for testing.
- How do you implement caching in Spring Boot? Caching can be implemented in Spring Boot by using the
@Cacheable
and@CacheEvict
annotations along with the caching configuration. - What is the use of the @Value annotation? The @Value annotation is used to inject values from properties files into Spring beans.
- How can you enable asynchronous processing in Spring Boot? Asynchronous processing can be enabled in Spring Boot by using the
@Async
annotation and configuring the ThreadPoolTaskExecutor. - What is the role of the CommandLineRunner interface? The CommandLineRunner interface allows you to execute code after the Spring Boot application has started.
- How do you implement security in Spring Boot? Security in Spring Boot can be implemented using Spring Security, which provides authentication and authorization features.
- Explain the concept of Spring Boot DevTools. Spring Boot DevTools provide development-time features like automatic restarts and live reload to enhance developer productivity.
- What is the use of the @RequestMapping annotation? The @RequestMapping annotation is used to map HTTP requests to controller methods in Spring Boot.
- How can you handle file uploads in Spring Boot? File uploads can be handled in Spring Boot using the
@RequestParam
annotation and theMultipartFile
class. - What is the purpose of the @Configuration annotation? The @Configuration annotation is used to indicate that a class contains bean definition methods.
- Explain the concept of Spring Boot TestSlice. Test slices in Spring Boot allow you to test specific layers of your application, such as the web layer or the JPA repository layer.
- How do you create a RESTful API in Spring Boot? You can create a RESTful API in Spring Boot by using the
@RestController
annotation and defining request mapping methods. - What is the role of the @EnableAutoConfiguration annotation? The @EnableAutoConfiguration annotation enables Spring Boot’s auto-configuration feature.
- How can you externalize logging configuration in Spring Boot? Logging configuration can be externalized in Spring Boot using the application.properties or application.yml file.
- Explain the concept of Spring Boot Actuator health checks. Spring Boot Actuator provides health checks that can be used to monitor the application’s health status.
- How do you create custom starter dependencies in Spring Boot? Custom starter dependencies can be created by packaging a set of related dependencies into a separate module and publishing it as a JAR.
- What is the purpose of the @ConditionalOnProperty annotation? The @ConditionalOnProperty annotation allows you to conditionally enable or disable a bean based on the presence or absence of certain properties.
- Explain the use of the @Scheduled annotation. The @Scheduled annotation is used to define scheduled tasks in Spring Boot applications.
- How do you handle transaction management in Spring Boot? Transaction management can be handled in Spring Boot using Spring’s declarative transaction management or by using the
@Transactional
annotation. - What are the different types of bean scopes in Spring Boot? The different bean scopes in Spring Boot are Singleton, Prototype, Request, Session, and Application.
- Explain the concept of Spring Boot profiles. Spring Boot profiles allow you to define different configurations for different environments (e.g., dev, test, prod).
- What is the purpose of the @Valid annotation? The @Valid annotation is used to perform validation on request parameters or request bodies in Spring Boot applications.
- How can you use Spring Boot Actuator to gather application metrics? Spring Boot Actuator provides various metrics, which can be gathered and exposed using endpoints like /metrics.
- What is the role of the @Qualifier annotation? The @Qualifier annotation is used to specify which bean should be autowired when multiple beans of the same type are available.
- Explain the concept of Spring Boot’s Parent-Child Context Hierarchy. Spring Boot allows the creation of a Parent-Child Context Hierarchy, where the Parent Context contains shared beans and configurations used across multiple child contexts.
- How can you manage dependencies in Spring Boot applications? You can manage dependencies in Spring Boot applications using Maven or Gradle build tools.
- What is the purpose of the @EnableScheduling annotation? The @EnableScheduling annotation is used to enable scheduling support in Spring Boot applications, allowing the use of @Scheduled tasks.
- Mastering Qualitative Research: The Role of Focus Groups in Data Collection - July 11, 2024
- What is robots ops? - July 10, 2024
- 5 Effective Online Learning Strategies for DevOps Professionals - July 4, 2024