Unit testing in Python is the process of testing individual units of code to ensure that they are working as expected. Unit tests are typically written by software developers and are run frequently as the code is being developed and modified.
Unit testing in Python is a fundamental practice for ensuring the correctness and reliability of your code.
Unit testing frameworks in python
Python offers several unit testing frameworks to help you write and run unit tests effectively. Here are some of the most popular unit testing frameworks in Python:
- unittest (PyUnit):
unittest
is the built-in unit testing framework in Python. It follows the xUnit style and provides a structured and organized way to write and run unit tests. It is inspired by Java’s JUnit and is a good choice for projects that require a standard testing framework.Example:
import unittest
class MyTest(unittest.TestCase):
def test_addition(self):
self.assertEqual(2 + 2, 4)
pytest:
pytest
is a highly popular and user-friendly testing framework for Python. It offers concise syntax, automatic test discovery, powerful fixture support, and a rich ecosystem of plugins. pytest
is known for its simplicity and extensibility.
def test_addition():
assert 2 + 2 == 4
nose:
nose
is another test discovery and execution framework for Python. While it’s not as actively maintained as pytest
, it is still used in some projects. It provides test discovery, test grouping, and support for plugins.
doctest:
doctest
is a lightweight testing framework that allows you to embed tests directly in your docstrings. It’s primarily used for writing tests within documentation, making it easy to keep code and documentation in sync.
Hypothesis:
Hypothesis
is a property-based testing framework that generates test cases automatically based on property specifications. It’s useful for finding edge cases and unexpected issues in your code.
unittest2 (unittest2py):
unittest2
is an extended version of the built-in unittest
module with some additional features and bug fixes. It provides compatibility with earlier Python versions (2.6 and 2.7).
Difference Between unittest and pytest
unittest and pytest are both unit testing frameworks for Python, but they have some key differences.
unittest is a standard module that comes bundled with Python. It is a more traditional unit testing framework, with a focus on simplicity and ease of use. unittest provides a set of classes and methods for writing and running unit tests, including:
TestCase
: A base class for test cases.setUp()
: A method that is run before each test case.tearDown()
: A method that is run after each test case.assertEqual()
: An assertion method that compares two values and raises an exception if they are not equal.assertRaises()
: An assertion method that tests whether a function raises a specific exception.
pytest is a third-party unit testing framework that is known for its simplicity, flexibility, and extensibility. pytest provides a number of features that make it easier to write and run unit tests, including:
- Automatic test discovery: pytest can automatically discover test cases based on the names of your test files and functions.
- Fixtures: Fixtures are special functions that can be used to set up and tear down the state of your tests.
- Parameterization: Parameterization allows you to run a single test case with different input values.
- Plugin support: pytest has a rich plugin system that allows you to extend its functionality to meet your specific needs.
Here is a table that summarizes the key differences between unittest and pytest:
Feature | unittest | pytest |
---|---|---|
Bundled with Python | Yes | No |
Test discovery | Manual | Automatic |
Fixtures | No | Yes |
Parameterization | No | Yes |
Plugin support | Limited | Extensive |
Which unit testing framework to choose depends on your personal preferences and the needs of your project. If you are new to unit testing, unittest is a good choice because it is simple and easy to use. If you are looking for a more powerful and flexible unit testing framework, pytest is a good choice.
Here are some general recommendations:
- Use unittest if:
- You are new to unit testing.
- You need a simple and easy-to-use unit testing framework.
- You are working on a project that uses other Python standard modules.
- Use pytest if:
- You want a powerful and flexible unit testing framework.
- You want to use fixtures to simplify your test code.
- You want to use parameterization to run your tests with different input values.
- You need to extend the functionality of your unit testing framework with plugins.
How to do Unit Test in Python?
Unit testing in Python involves testing individual units or components of your code, such as functions or methods, in isolation to ensure that they work correctly. You can use the unittest
framework, which is part of the Python standard library, to perform unit testing. Here’s a step-by-step guide on how to do unit testing in Python:
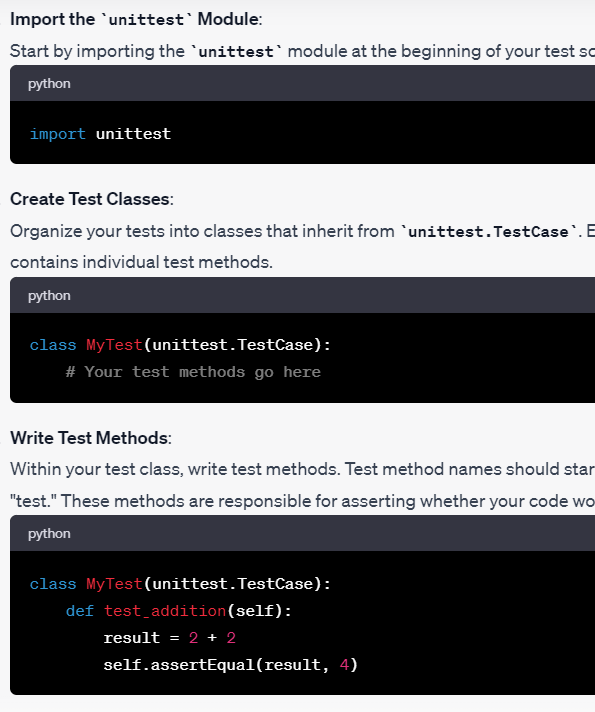
import unittest
def sum(a, b):
return a + b
class TestSum(unittest.TestCase):
def test_sum_of_two_numbers(self):
self.assertEqual(sum(1, 2), 3)
def test_sum_of_empty_list(self):
self.assertEqual(sum([]), 0)
if __name__ == '__main__':
unittest.main()
This test class defines two test methods: test_sum_of_two_numbers() and test_sum_of_empty_list(). The first test method tests that the sum() function returns the expected value when given a list of two numbers. The second test method tests that the sum() function returns the expected value when given an empty list.
To run these tests, we can simply run the test script:
$ python test_sum.py
- How Email Marketing and Website Development Join Forces to Redefine Marketing Success - April 4, 2024
- Newrelic Tutorials: Critical Violation - April 2, 2024
- What is custom software development? - March 31, 2024