AWS Approach: aws ec2 instance user data to make sure commands run in each ec2 instance in sequential not parallel
Approach # 1
To ensure that commands specified in the EC2 instance user data are executed sequentially rather than in parallel on multiple instances, you can use a synchronization mechanism such as a lock file or a distributed coordination service. Here’s an approach using a lock file:
- Create a lock file in a shared location that is accessible by all EC2 instances. For example, you can use Amazon S3 or a shared network file system (NFS) mounted on each instance.
- Modify the EC2 instance user data script to check for the existence of the lock file before executing any commands. If the lock file exists, the script waits until the lock file is released; otherwise, it creates the lock file and continues with the execution.
Here’s an example of how you can modify the user data script:
#!/bin/bash
lockfile="/path/to/lockfile"
# Check if the lock file exists
while [ -f "$lockfile" ]; do
echo "Lock file exists. Waiting for lock release..."
sleep 10
done
# Create the lock file
touch "$lockfile"
# Execute your sequential commands here
echo "Executing command 1..."
<command 1>
echo "Executing command 2..."
<command 2>
# Release the lock file
rm "$lockfile"
#!/bin/bash
bucket_name="your-s3-bucket-name"
lockfile_key="lockfile"
# Check if the lock file exists in S3 bucket
while aws s3 ls "s3://$bucket_name/$lockfile_key" >/dev/null 2>&1; do
echo "Lock file exists. Waiting for lock release..."
sleep 10
done
# Create the lock file
aws s3 cp /dev/null "s3://$bucket_name/$lockfile_key"
# Execute your sequential commands here
echo "Executing command 1..."
<command 1>
echo "Executing command 2..."
<command 2>
# Release the lock file
aws s3 rm "s3://$bucket_name/$lockfile_key"
#!/bin/bash
bucket_name="your-s3-bucket-name"
lockfile_key="lockfile"
# Check if the lock file exists in S3 bucket
while aws s3api head-object --bucket "$bucket_name" --key "$lockfile_key" >/dev/null 2>&1; do
echo "Lock file exists. Waiting for lock release..."
sleep 10
done
# Create the lock file
aws s3api put-object --bucket "$bucket_name" --key "$lockfile_key" --body /dev/null
# Execute your sequential commands here
echo "Executing command 1..."
<command 1>
echo "Executing command 2..."
<command 2>
# Release the lock file
aws s3api delete-object --bucket "$bucket_name" --key "$lockfile_key"
Approach # 2
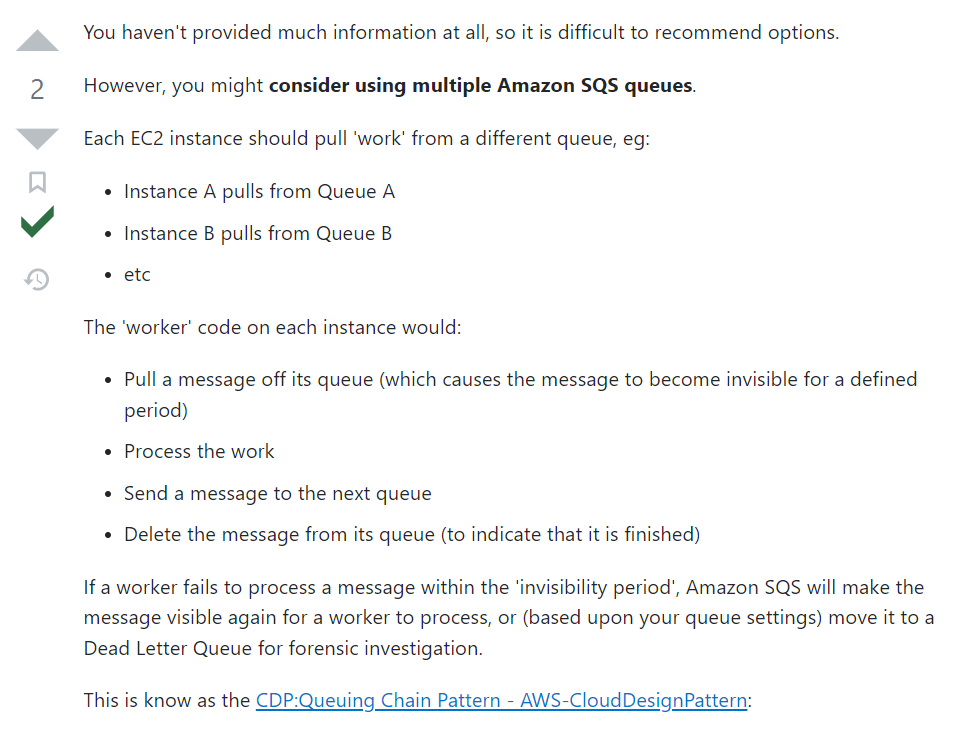
Latest posts by Rajesh Kumar (see all)
- What is Mobile Virtual Network Operator? - April 18, 2024
- What is Solr? - April 17, 2024
- Difference between UBUNTU and UBUNTU PRO - April 17, 2024