What are Object Detection Tools?
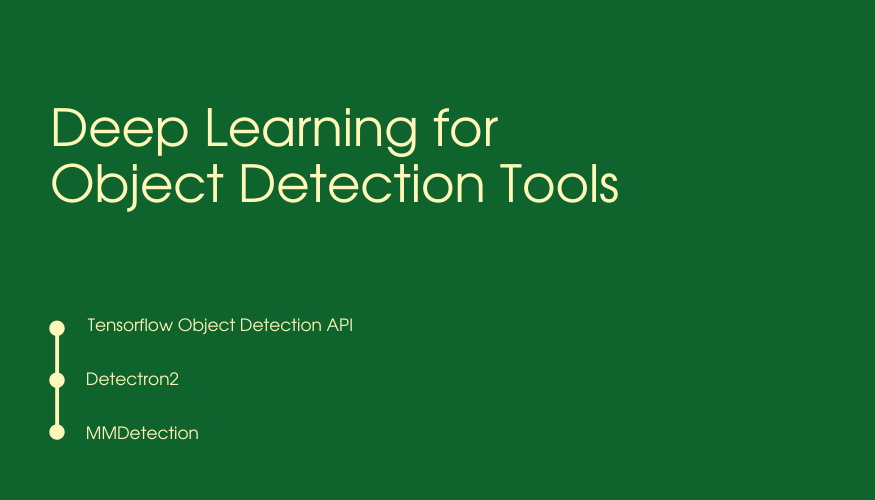
Object Detection Tools are software applications that use computer vision and machine learning techniques to locate and identify objects of interest within images or videos. These tools analyze visual data and draw bounding boxes around the detected objects, enabling various applications in fields such as surveillance, autonomous vehicles, and image analysis.
Top 10 use cases of Object Detection Tools:
- Surveillance and Security: Object detection tools are used for detecting and tracking suspicious activities in surveillance footage.
- Autonomous Vehicles: Object detection is crucial for autonomous vehicles to identify pedestrians, vehicles, and obstacles.
- Retail Analytics: Object detection tools help retailers track and analyze customer movements in stores.
- Industrial Automation: Object detection is used in robotics for pick-and-place tasks and quality control.
- Medical Imaging: Object detection assists in locating and identifying anomalies in medical images.
- Human-Computer Interaction: Object detection enables gesture recognition and hand tracking for interactive systems.
- Augmented Reality: Object detection is used in AR applications to overlay virtual objects on the real world.
- Image and Video Captioning: Object detection helps generate captions for images and videos.
- Sports Analytics: Object detection is used to track player movements and analyze gameplay.
- Agriculture: Object detection tools assist in crop monitoring and pest detection.
What are the feature of Object Detection Tools?
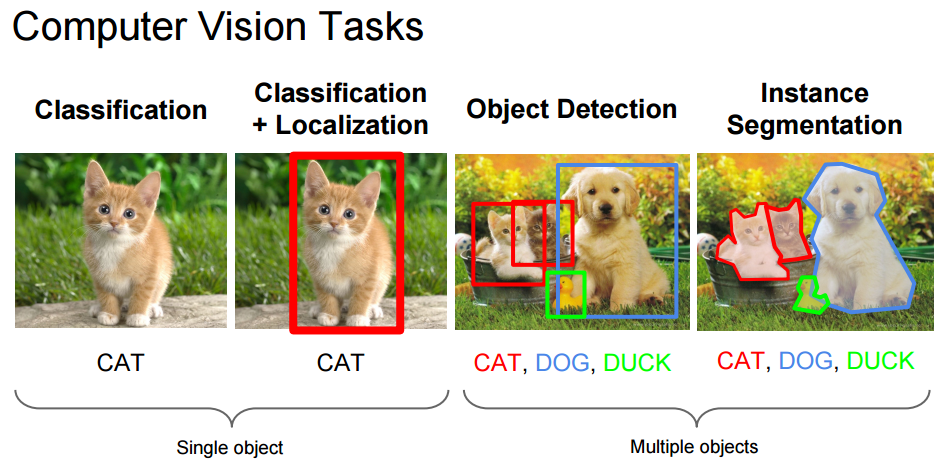
- High Accuracy: Object detection tools aim for high accuracy in locating and identifying objects.
- Real-Time Processing: Some tools provide real-time object detection capabilities.
- Customization: Some tools can be fine-tuned for specific object classes and domains.
- Multiple Object Detection: Tools can detect multiple object classes simultaneously.
How Object Detection Tools Work and Architecture?
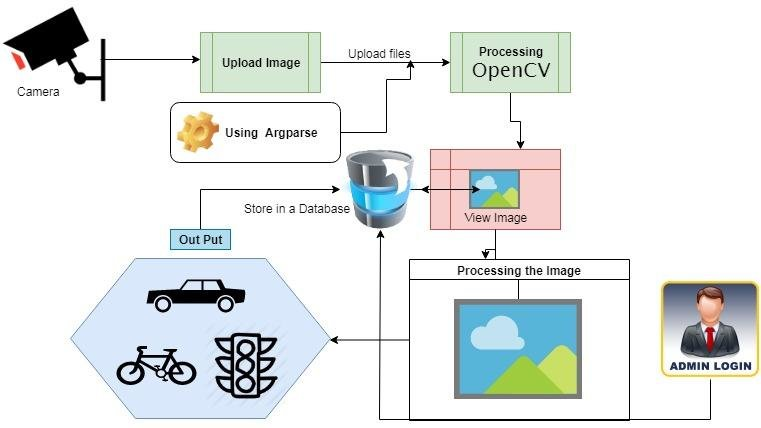
The architecture of Object Detection Tools typically involves the following stages:
- Input Image Processing: The input image is preprocessed, including resizing and normalization.
- Feature Extraction: The tool extracts features from the input image using convolutional neural networks (CNNs).
- Region Proposal: The tool generates region proposals (candidate bounding boxes) where objects might be present.
- Classification: The CNN classifies the regions to identify if each box contains an object.
- Bounding Box Refinement: The tool refines the bounding box predictions to accurately locate the objects.
- Non-Maximum Suppression: Overlapping and redundant boxes are eliminated to get the final detection results.
How to Install Object Detection Tools?
The installation process for object detection tools depends on the specific tool or library used. Some popular object detection libraries and frameworks include:
- TensorFlow Object Detection API: A powerful framework built on top of TensorFlow for object detection.
pip install tensorflow
- YOLO (You Only Look Once): A fast real-time object detection framework.
pip install darknetpy
- Detectron2: A high-performance object detection library developed by Facebook AI Research (FAIR).
pip install detectron2 -f https://dl.fbaipublicfiles.com/detectron2/wheels/cpu/index.html
- OpenCV: A computer vision library that includes object detection capabilities.
pip install opencv-python
Please note that some object detection tools may have specific hardware or platform requirements. Always refer to the official documentation and tutorials provided by the specific tool or library for detailed installation instructions and best practices. Additionally, object detection models often require pre-trained weights for specific object classes, and you may need to download these weights separately to use the models effectively.
Basic Tutorials of Object Detection Tools: Getting Started
Creating a comprehensive step-by-step tutorial for object detection tools can be quite extensive due to the variety of available tools and their specific implementations. However, I can provide a basic guide for using the TensorFlow Object Detection API, which is a popular and powerful framework for object detection tasks.
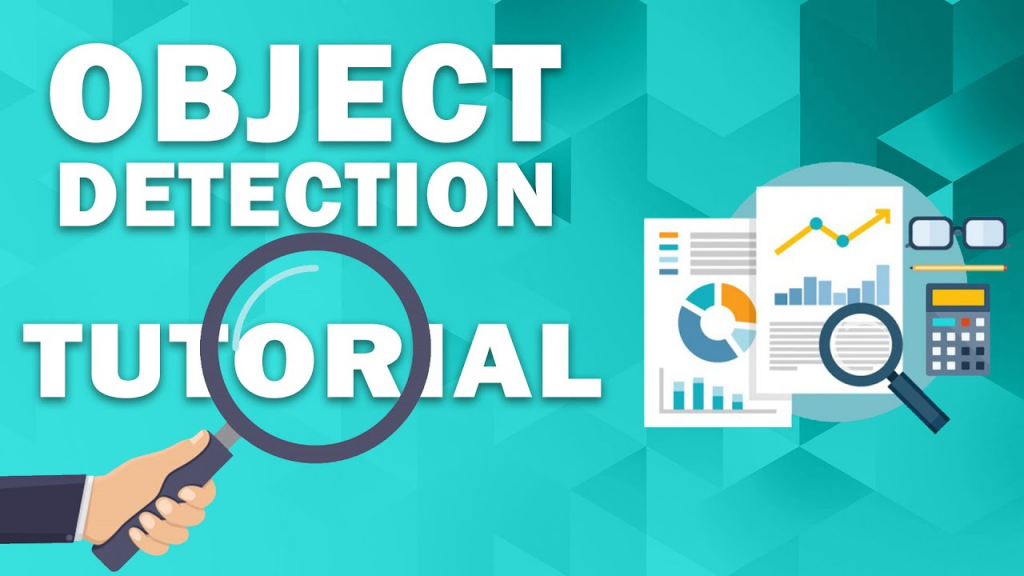
Step-by-Step Basic Tutorial for Object Detection using TensorFlow Object Detection API:
- Install Required Libraries:
- Install the required libraries, including TensorFlow and the TensorFlow Object Detection API:
pip install tensorflow pip install tf_slim
2. Set Up Object Detection Directory:
- Create a new directory for your object detection project, e.g.,
object_detection_project
.
3. Clone TensorFlow Models Repository:
- Clone the TensorFlow Models repository, which includes the Object Detection API:
git clone https://github.com/tensorflow/models.git
4. Install Protobuf Compiler:
- Download the protobuf compiler from the official Protocol Buffers repository (https://github.com/protocolbuffers/protobuf/releases).
- Extract the protobuf compiler binary to a directory, e.g.,
C:\protobuf
.
5. Compile Protobufs:
- Navigate to the
models/research
directory and compile the Protobuf files:cd models/research protoc object_detection/protos/*.proto --python_out=.
6. Set Up PYTHONPATH:
- Set the
PYTHONPATH
environment variable to include the necessary directories:set PYTHONPATH=%PYTHONPATH%;C:\path\to\models\research;C:\path\to\models\research\slim
7. Prepare Object Detection Data:
- Organize your object detection data in the required format, including labeled images and their corresponding XML files or CSV annotations.
8. Create Training and Testing Datasets:
- Divide your data into testing and training datasets.
9. Create Label Map:
- Create a label map that maps class names to integer IDs in a text file, e.g.,
label_map.pbtxt
.
item { id: 1 name: 'class_1' } item { id: 2 name: 'class_2' }
10. Create Training Configuration:
- Create a configuration file (e.g., `ssd_mobilenet_v2.config`) for your chosen object detection model. You can find example configurations in the `models/research/object_detection/samples/configs` directory.
11. Train the Object Detection Model:
- Train the object detection model using the TensorFlow Object Detection API’s
model_main.py
script:
python models/research/object_detection/model_main.py --model_dir=training/ --pipeline_config_path=training/ssd_mobilenet_v2.config --num_train_steps=10000 --sample_1_of_n_eval_examples=1 --alsologtostderr
12. Export the Trained Model:
- Export the instructed model for inference:
python models/research/object_detection/exporter_main_v2.py --input_type image_tensor --pipeline_config_path training/ssd_mobilenet_v2.config --trained_checkpoint_dir training/ --output_directory inference_graph
13. Run Object Detection on Test Images:
- Use the exported model to perform object detection on test images:
import cv2 import numpy as np import tensorflow as tf from object_detection.utils import label_map_util from object_detection.utils import visualization_utils as viz_utils # Load the model detect_fn = tf.saved_model.load('inference_graph/saved_model') # Load label map label_map_path = 'path/to/label_map.pbtxt' category_index = label_map_util.create_category_index_from_labelmap(label_map_path, use_display_name=True) # After Loading, process the test image image_path = 'path/to/test_image.jpg' image_np = cv2.imread(image_path) input_tensor = tf.convert_to_tensor(image_np) input_tensor = input_tensor[tf.newaxis, ...] # Perform inference detections = detect_fn(input_tensor) # Visualization viz_utils.visualize_boxes_and_labels_on_image_array(image_np, detections['detection_boxes'][0].numpy(), detections['detection_classes'][0].numpy().astype(np.uint32), detections['detection_scores'][0].numpy(), category_index, use_normalized_coordinates=True, max_boxes_to_draw=200, min_score_thresh=.30, agnostic_mode=False) cv2.imshow('Object Detection', cv2.cvtColor(image_np, cv2.COLOR_RGB2BGR)) cv2.waitKey(0)
Please note that this tutorial provides a basic introduction to using the TensorFlow Object Detection API for object detection. For more advanced features and to fine-tune the model on your specific dataset, refer to the official documentation and tutorials of the TensorFlow Object Detection API. Additionally, you can explore other object detection tools and libraries like Detectron2, YOLO, and OpenCV’s DNN module for different use cases and performance requirements.
- Why Can’t I Make Create A New Folder on External Drive on Mac – Solved - April 28, 2024
- Tips on How to Become a DevOps Engineer - April 28, 2024
- Computer Programming Education Requirements – What You Need to Know - April 28, 2024