What is Angular?
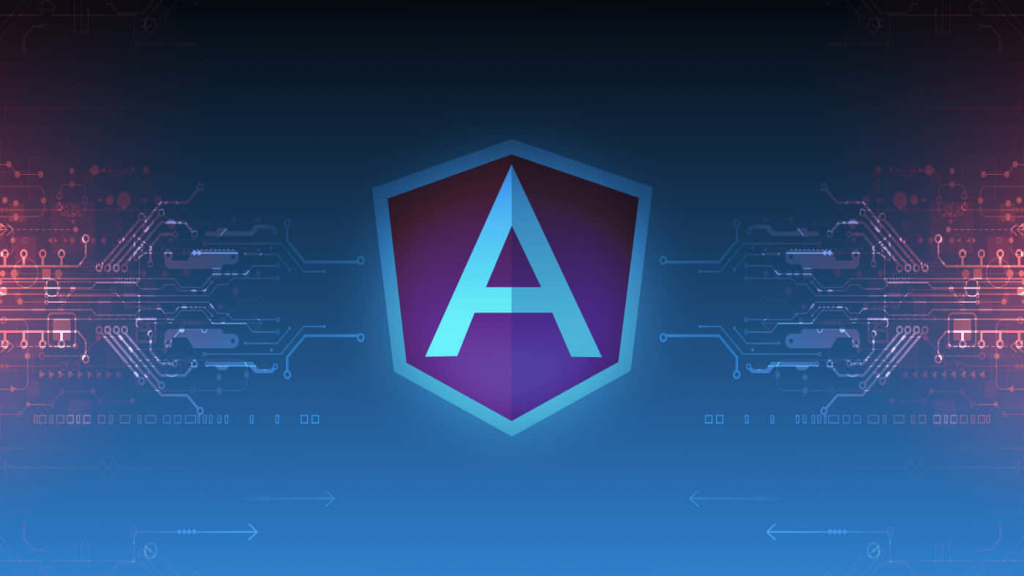
Angular is a popular open-source framework for building web applications. It was developed by Google and released in 2010. It is widely used for building dynamic, single-page web applications (SPAs) with rich user interfaces. Angular provides a comprehensive set of tools, libraries, and best practices for developing scalable and maintainable front-end applications.
Key Features of Angular:
- Component-Based Architecture: Angular follows a component-based architecture, where the application is structured into components, each responsible for a specific part of the user interface.
- Two-Way Data Binding: Angular offers two-way data binding, enabling automatic synchronization between the model (business logic) and the view (UI). Changes in one are reflected in the other without explicit manipulation.
- Dependency Injection: Angular’s dependency injection system makes it easy to manage and organize components and services. It promotes modularity and helps in creating testable and maintainable code.
- Directives: Angular allows developers to create custom HTML directives, extending the functionality of HTML with reusable and customizable components.
- Services: Services in Angular provide a way to organize and share code and functionality across different components. They are commonly used for data retrieval, business logic, and communication between components.
- Modules: Angular applications are organized into modules, each serving as a container for related components, services, and directives. This helps in organizing and modularizing the codebase.
- Routing: Angular provides a powerful routing system for building SPAs with multiple views. It enables navigation between different components without the need for a full-page reload.
- Forms: Angular offers a robust form handling system, including template-driven forms and reactive forms, for creating and managing user input.
- HTTP Client: Angular includes an HTTP client module for making HTTP requests to servers. It supports features like interceptors, error handling, and observables.
- Testing Support: Angular is planned with testability in mind. It provides tools like TestBed for unit testing and end-to-end testing using tools like Protractor.
What is top use cases of Angular?
- Single-Page Applications (SPAs): Angular is widely used for building SPAs, where a single HTML page is dynamically updated based on user interactions, without the need for full-page reloads. Popular SPAs built with Angular include Gmail and Google Analytics.
- Enterprise Applications: Angular is well-suited for building large-scale enterprise applications with complex user interfaces. Its modular and component-based architecture facilitates the development of maintainable and scalable code.
- Content Management Systems (CMS): Angular is employed in building content management systems and administrative dashboards due to its flexibility and ease of creating dynamic user interfaces.
- E-commerce Platforms: Angular is used in the development of e-commerce platforms and online marketplaces, where dynamic and responsive user interfaces are crucial for a positive user experience.
- Dashboards and Analytics Tools: Angular is often chosen for developing interactive dashboards and analytics tools, providing real-time data visualization and reporting capabilities.
- Collaborative Tools: Applications that require real-time collaboration, such as project management tools and collaborative document editing platforms, benefit from Angular’s real-time data binding and responsive UI capabilities.
- Social Networking Apps: Angular is used in the development of social networking applications and platforms where real-time updates and interactive features are essential for user engagement.
- Data Visualization Platforms: Angular is employed in applications that require complex data visualization, including charting libraries and interactive data-driven dashboards.
- Educational Platforms: Angular is used in the development of educational platforms, learning management systems (LMS), and e-learning applications that require dynamic and interactive interfaces.
- Progressive Web Apps (PWAs): Angular is suitable for building PWAs, which are web applications that provide a native app-like experience on the web, including offline functionality, responsive design, and push notifications.
Angular’s versatility, strong community support, and extensive ecosystem make it a popular choice for a variety of web development scenarios, especially when building complex and feature-rich applications.
What are feature of Angular?
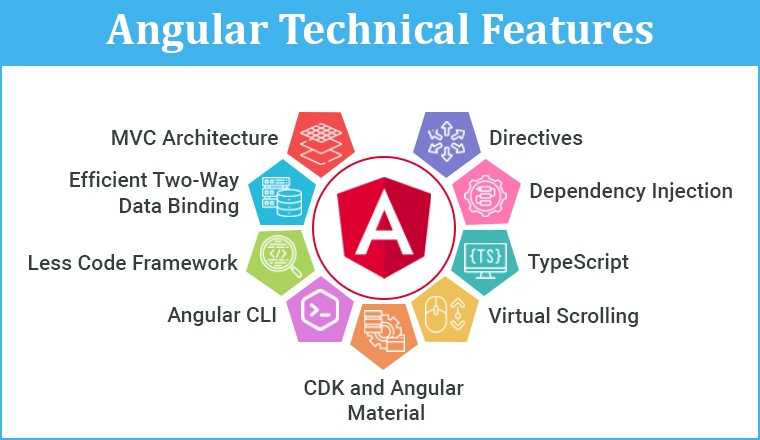
Features of Angular:
- Component-Based Architecture: Angular follows a component-based architecture, where applications are structured into modular components, each encapsulating a specific part of the user interface and its logic.
- Two-Way Data Binding: Angular provides two-way data binding, allowing automatic synchronization between the model (business logic) and the view (UI). Changes in the model are reflected in the view and vice versa.
- Dependency Injection: Angular’s dependency injection system facilitates the organization of components, services, and other objects. It promotes modularity, code reusability, and testability.
- Templates and Directives: Angular templates use HTML enhanced with directives to express the application’s components. Directives extend HTML with additional behavior and functionality.
- Services: Angular services are singleton objects that can be injected into components, providing a way to share functionality, data, or logic across different parts of an application.
- Modules: Angular applications are organized into modules, each serving as a container for components, services, and other related code. Modules help in organizing and separating concerns within an application.
- Routing: Angular provides a powerful routing system that allows developers to create SPAs with multiple views. It enables navigation between different components without the need for a full-page reload.
- Forms Handling: Angular offers a comprehensive set of tools for building forms, including template-driven forms and reactive forms. It simplifies form validation, handling user input, and managing form state.
- HTTP Client: Angular includes an HTTP client module for making HTTP requests to servers. It supports features like request and response transformations, interceptors, and observables for handling asynchronous operations.
- Testing Support: Angular is planned with testability in mind. It provides tools like TestBed for unit testing and supports end-to-end testing using tools like Protractor.
- Animation: Angular has a built-in animation system that allows developers to create smooth animations and transitions in their applications. It supports a wide range of animations for enhancing user experience.
- Internationalization (i18n): Angular supports internationalization and localization, making it easier to build applications that can be adapted to different languages and regions.
- CLI (Command Line Interface): Angular CLI provides a command-line interface for creating, building, testing, and deploying Angular applications. It streamlines common development tasks and helps in project scaffolding.
- Progressive Web App (PWA) Support: Angular includes features that support building Progressive Web Apps, allowing developers to create web applications with native app-like experiences, including offline capabilities.
What is the workflow of Angular?
- Project Setup:
- Use Angular CLI to create a new project:
ng new my-app
- Navigate to the project folder:
cd my-app
- Use Angular CLI to create a new project:
- Component Creation:
- Generate components using Angular CLI:
ng generate component my-component
- Components are the building blocks of Angular applications.
- Generate components using Angular CLI:
- Service Creation:
- Generate services for business logic:
ng generate service my-service
- Services are used for sharing functionality and data between components.
- Generate services for business logic:
- Module Configuration:
- Organize components and services into modules.
- Configure modules using the
@NgModule
decorator.
- Routing Configuration:
- Configure routing for the application in the
app-routing.module.ts
file. - Define routes and associate them with components.
- Configure routing for the application in the
- Template and Component Logic:
- Write HTML templates for components.
- Implement component logic in TypeScript files.
- Data Binding:
- Use data binding to establish connections between the model and the view.
- Implement two-way data binding for real-time updates.
- Forms Handling:
- Create forms using Angular’s form handling features.
- Implement form validation and user input handling.
- Dependency Injection:
- Use dependency injection to inject services into components.
- Encapsulate and share functionality through services.
- HTTP Requests:
- Use the Angular HTTP client to make HTTP requests to a server.
- Handle responses and data transformations.
- Testing:
- Write unit tests for components and services using tools like TestBed.
- Conduct end-to-end testing using tools like Protractor.
- Animation:
- Implement animations using Angular’s animation system.
- Enhance user experience with smooth transitions.
- Internationalization:
- Use Angular’s internationalization features for supporting multiple languages.
- Configure and translate application content.
- Build and Deployment:
- Build the application using Angular CLI:
ng build
- Deploy the built artifacts to a web server or cloud platform.
- Build the application using Angular CLI:
This workflow provides a high-level overview of the steps involved in creating an Angular application. Developers can use Angular CLI commands to streamline many of these tasks and focus on building features and functionality. The modular and component-based nature of Angular makes it well-suited for large and complex applications.
How Angular Works & Architecture?
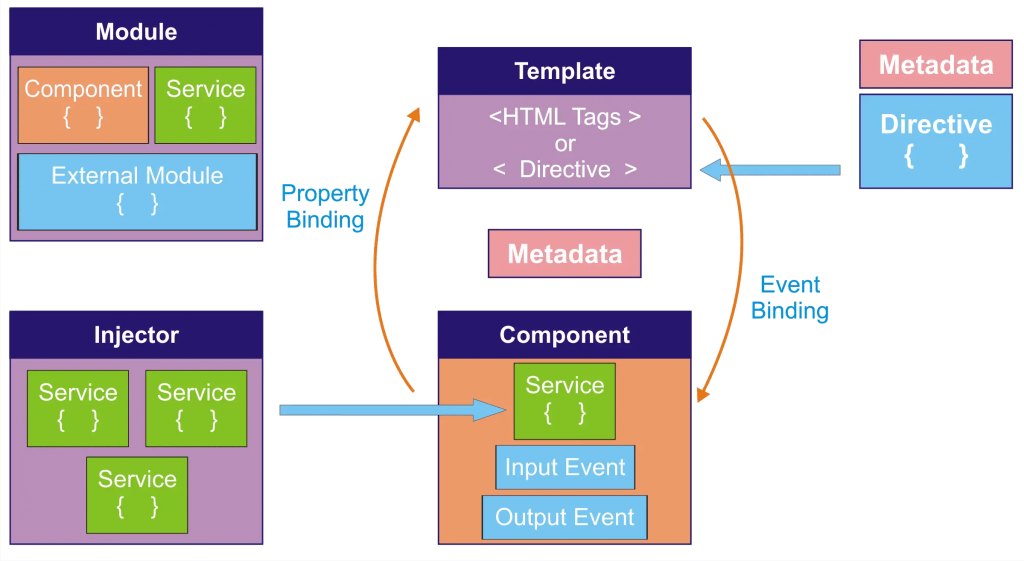
Following is an overview of how Angular works and its architecture:
Angular Overview
It is a comprehensive framework that provides a wide range of features for building modern web applications, including:
- Component-based architecture: Angular applications are built using components, which are reusable modular pieces that encapsulate logic, HTML, and CSS.
- Data binding: Angular uses a powerful data binding mechanism to automatically synchronize data between the application’s model and the view.
- Dependency injection: Angular provides a dependency injection system that makes it easy to manage dependencies and promote loose coupling between components.
- Directives: Angular provides directives, which are HTML attributes that extend the functionality of HTML elements.
- Routing: Angular provides a routing system for handling navigation between different parts of the application.
- Forms: Angular provides a comprehensive form validation and management system.
- HTTP client: Angular provides an HTTP client for making network requests.
Angular Architecture
Angular follows a layered architecture that consists of the following components:
- Modules: Angular applications are organized into modules, which are logical groupings of components, services, and other related code.
- Components: Components are the fundamental building blocks of Angular applications. They encapsulate logic, HTML, and CSS, and they can be nested to create complex UIs.
- Services: Services are singleton classes that provide functionality that can be shared across components. They are responsible for business logic, data access, and other tasks that are not directly related to the UI.
- Templates: Templates are HTML files that define the view of a component. They use directives and data binding to connect to the component’s logic and data.
- Directives: Directives are HTML attributes that extend the functionality of HTML elements. They can be used to add custom behavior, conditional rendering, and other features.
- Pipes: Pipes are transformations that can be applied to data before it is displayed in the template. They are commonly used for formatting data, such as filtering, sorting, and converting data types.
How Angular Works
When a user requests an Angular application, the following steps occur:
- Bootstrap: The Angular framework bootstraps the application by loading the necessary modules and components.
- Routing: The routing system parses the URL to determine which component to display.
- Component Creation: Based on the routing configuration, the Angular framework creates the corresponding components. Each component is responsible for managing its own data, handling user interactions, and rendering its view using the associated HTML template.
How to Install and Configure Angular?
Following is a step-by-step guide on how to install and configure Angular:
Prerequisites:
- Node.js: Ensure you have Node.js installed on your system. Node.js provides the necessary runtime environment for Angular development. You can download and install Node.js from the official website: https://node.js.org/en/download/
- NPM (Node Package Manager): NPM comes bundled with Node.js installation and is used to manage JavaScript packages, including Angular.
Installing Angular CLI:
- Open Terminal: Open a terminal window or command prompt.
- Install Angular CLI: Use the following command to install the Angular CLI globally:
Bash
npm install -g @angular/cli
The Angular CLI (Command Line Interface) is a tool that provides scaffolding and other commands for developing Angular applications.
Creating an Angular Project:
- Navigate to Project Directory: Open a terminal window or command prompt and navigate to the directory where you want to create your Angular project.
- Create Angular Project: Use the following command to create a new Angular project named
my-project
:
Bash
ng new my-project
This command will create a new project directory named my-project
and install the necessary dependencies for your Angular application.
Starting the Angular Development Server:
- Navigate to Project Directory: Open a terminal window or command prompt and navigate to the project directory created in the previous step.
- Start Development Server: Use the following command to start the Angular development server:
Bash
ng serve
This command will start a development server that will serve your Angular application at http://localhost:4200
by default.
Configuring Angular:
Angular provides various configuration options that you can customize to suit your development needs. Some common configuration options include:
- Environment variables: You can set environment variables to control various aspects of Angular compilation, such as the optimization level and the output format.
- Compiler flags: You can pass compiler flags to the Angular compiler to control various aspects of compilation, such as the target platform and the debug level.
- Project settings: You can set project-specific configuration options in
angular.json
, which is located in the root directory of your project. These settings include the project name, the target platform, and the build configuration.
Fundamental Tutorials of Angular: Getting started Step by Step
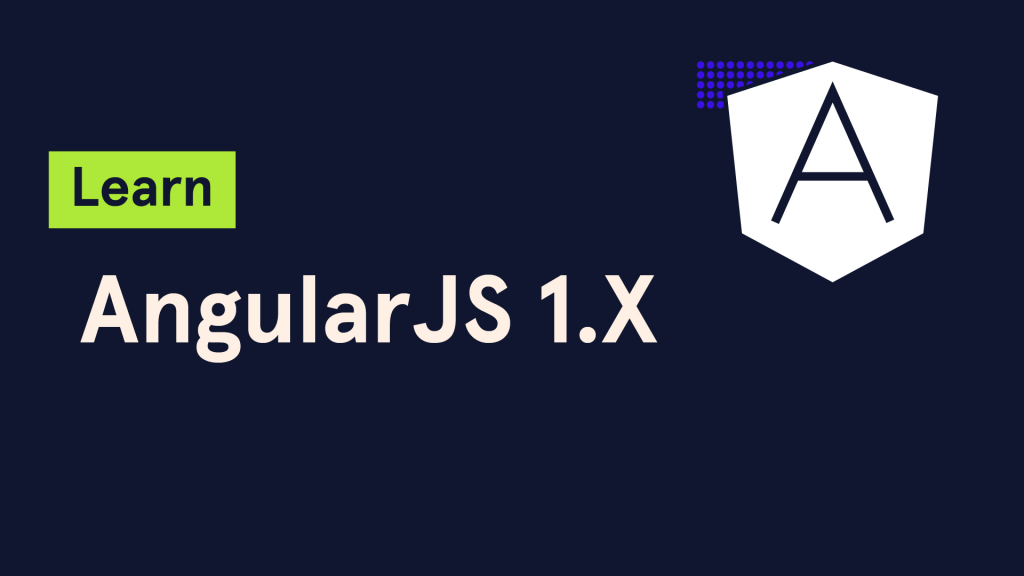
Step-by-step basic tutorial of Angular that will teach you the fundamentals of the framework:
Introduction to Angular
Angular is a TypeScript-based open-source web application framework led by the Angular Team at Google. It is a comprehensive framework that provides a wide range of features for building modern web applications, including:
- Component-based architecture: Angular applications are built using components, which are reusable modular pieces that encapsulate logic, HTML, and CSS.
- Data binding: Angular uses a powerful data binding mechanism to automatically synchronize data between the application’s model and the view.
- Dependency injection: Angular provides a dependency injection system that makes it easy to manage dependencies and promote loose coupling between components.
- Directives: Angular provides directives, which are HTML attributes that extend the functionality of HTML elements.
- Routing: Angular provides a routing system for handling navigation between different parts of the application.
- Forms: Angular provides a comprehensive form validation and management system.
- HTTP client: Angular provides an HTTP client for making network requests.
Step 1: Creating an Angular Project
- Install Angular CLI: Ensure you have Node.js and NPM installed on your system. Then, install the Angular CLI globally using the following command:
Bash
npm install -g @angular/cli
- Create a New Project: Navigate to the desired project directory and create a new Angular project using the following command:
Bash
ng new my-project
This command will create a project directory named my-project
and install the necessary dependencies for your Angular application.
Step 2: Understanding Component Structure
Components are the fundamental building blocks of Angular applications. They encapsulate logic, HTML, and CSS, and they can be nested to create complex UIs. Each component has a TypeScript file (.ts) that defines its logic and behavior, and an HTML template file (.html) that defines its presentation.
Step 3: Data Binding
Data binding is a core concept in Angular that allows you to automatically synchronize data between the application’s model and the view. Angular supports various types of data binding, including:
- Interpolation: Displaying expressions directly within HTML templates.
- Property binding: Setting component properties based on expressions.
- Event binding: Handling user interactions and updating the model accordingly.
Step 4: Dependency Injection
Dependency injection (DI) is a design pattern that allows you to decouple components from their dependencies and manage them centrally. Angular’s DI system makes it easy to inject dependencies into components through the constructor or through decorator functions.
Step 5: Routing
Routing enables navigation between different parts of your Angular application. Angular provides a routing system that handles mapping URLs to components and maintaining application state as users navigate.
Step 6: Building and Running the Application
Once you have developed your Angular application, you can build it for production using the following command:
Bash
ng build
This will generate optimized production-ready files for your application. To run the application locally, use the following command:
Bash
ng serve
This will start a development server that will serve your Angular application at http://localhost:4200
by default.
This is a brief introduction to the basics of Angular. There are many more features and concepts to explore, and numerous resources available to help you learn more about this powerful framework.
- Mutual of Omaha: Selection and Interview process, Questions/Answers - April 15, 2024
- AES: Selection and Interview process, Questions/Answers - April 15, 2024
- Amphenol: Selection and Interview process, Questions/Answers - April 15, 2024