What is Arrays?
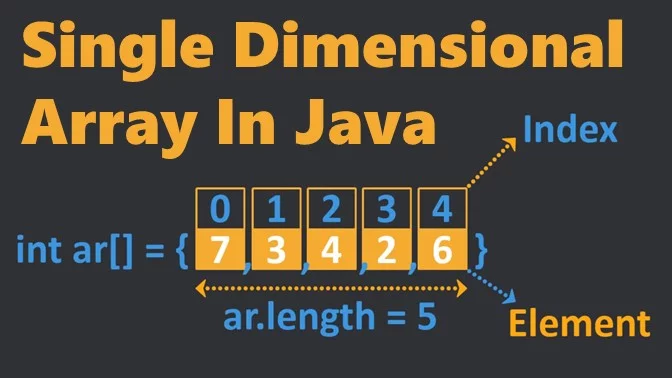
An array is a data structure that stores a collection of elements of the same data type in a sequential manner. Each element in an array is identified by its index or position, which starts from 0 for the first element. Arrays provide a way to store and access multiple values of the same type using a single variable.
Top Use Cases of Arrays:
- Data Storage: Arrays are commonly used for storing a collection of data items, such as numbers, strings, or objects, in a structured way.
- Iterating and Processing: Arrays allow you to iterate through each element using loops, making it easy to perform operations or calculations on all elements.
- Lists and Collections: Arrays can be used to implement various list or collection data structures, providing a way to manage and manipulate groups of related items.
- Matrices and Multidimensional Data: Arrays can be used to represent matrices or other multidimensional data structures used in mathematical computations, graphics, and simulations.
- Search and Retrieval: Arrays are suitable for scenarios where you need to quickly access elements by their index or position.
- Sorting and Searching: Arrays are often used as data sources for sorting algorithms like quicksort or binary search.
- Caching: Arrays can be used to cache and manage temporary data in memory, improving performance for repeated access.
- Implementing Algorithms: Many algorithms, such as sorting, searching, and dynamic programming, involve arrays for data manipulation and storage.
- Image Processing: Arrays are used to represent images as grids of pixels, enabling various image processing operations.
- Statistical Analysis: Arrays can store datasets used for statistical analysis, making it easier to compute various measures like mean, median, and standard deviation.
Arrays are a fundamental concept in programming and are widely used in various domains. They provide a simple and efficient way to work with collections of data and are the building blocks for more complex data structures and algorithms.
What are the features of Arrays?
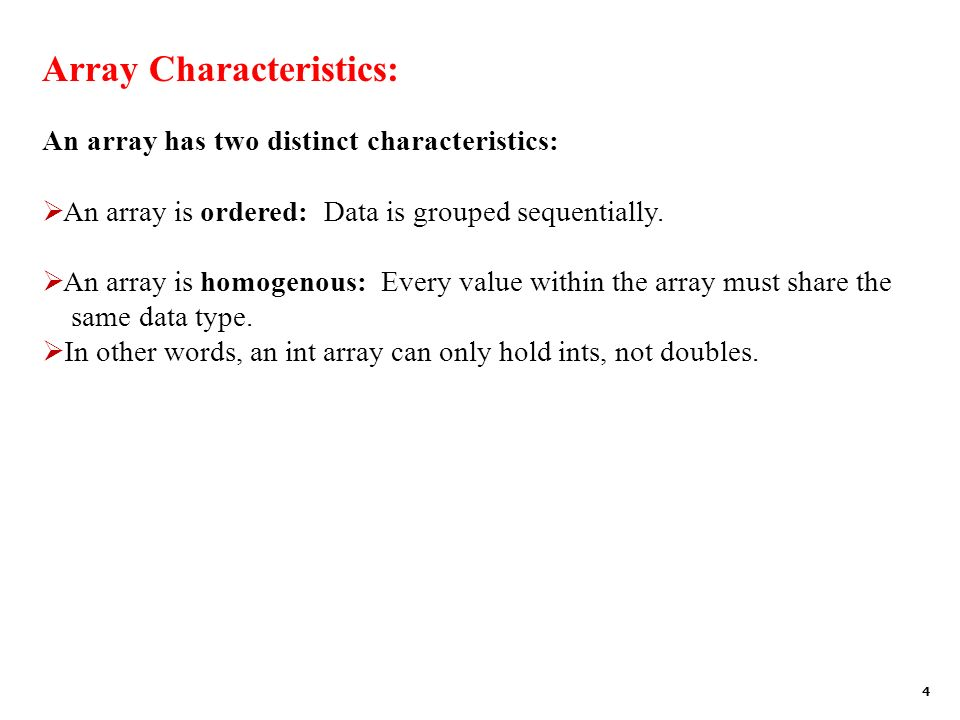
- Fixed Size: Arrays have a fixed size once they are declared. The size is determined during initialization and cannot be changed dynamically.
- Homogeneous Elements: Arrays store elements of the same data type, ensuring uniformity in the stored data.
- Index-Based Access: Elements in an array are accessed using their index or position, starting from 0 for the first element.
- Contiguous Memory: Array elements are stored in contiguous memory locations, which enables efficient access and traversal.
- Efficient Element Access: Accessing elements in an array is typically constant time (O(1)) since the index provides direct access to the memory location.
- Iterative Operations: Arrays are suited for performing iterative operations using loops, as elements can be sequentially accessed.
- Efficient Memory Usage: Arrays allocate memory for a fixed number of elements, making them memory-efficient when the size is known in advance.
- Direct Storage: Elements are stored directly in the array, making it easy to manage and manipulate data.
- Simple Syntax: Arrays have a straightforward syntax for declaration, initialization, and access, making them easy to work with.
What is the workflow of Arrays?
The workflow of using arrays involves several steps:
- Declaration: Declare an array variable with a specific data type and specify the maximum number of elements it can hold.
- Initialization: Initialize the array by assigning values to its elements. The values can be assigned individually or using loops.
- Access and Manipulation: Access individual elements by their index and perform desired operations on them, such as reading, updating, or calculating.
- Iterative Operations: Use loops to iterate through the elements of the array. Loop constructs like
for
andwhile
are commonly used for this purpose. - Searching and Sorting: Arrays can be searched for specific values or sorted using algorithms like linear search, binary search, or sorting algorithms.
- Memory Management: Arrays automatically manage the memory required to store their elements, ensuring that each element is stored in a contiguous memory location.
- Error Handling: Be cautious about accessing elements beyond the array’s size, as this can lead to runtime errors like “index out of bounds.”
- Deletion and Resize: Arrays generally have a fixed size, so adding or removing elements can be challenging. Some programming languages provide resizable arrays or dynamic arrays to address this limitation.
The workflow of arrays is relatively straightforward, making them a fundamental concept in programming. While arrays have many advantages, it’s important to be aware of their limitations, such as the fixed size and the need to manage memory explicitly.
How Arrays Works & Architecture?
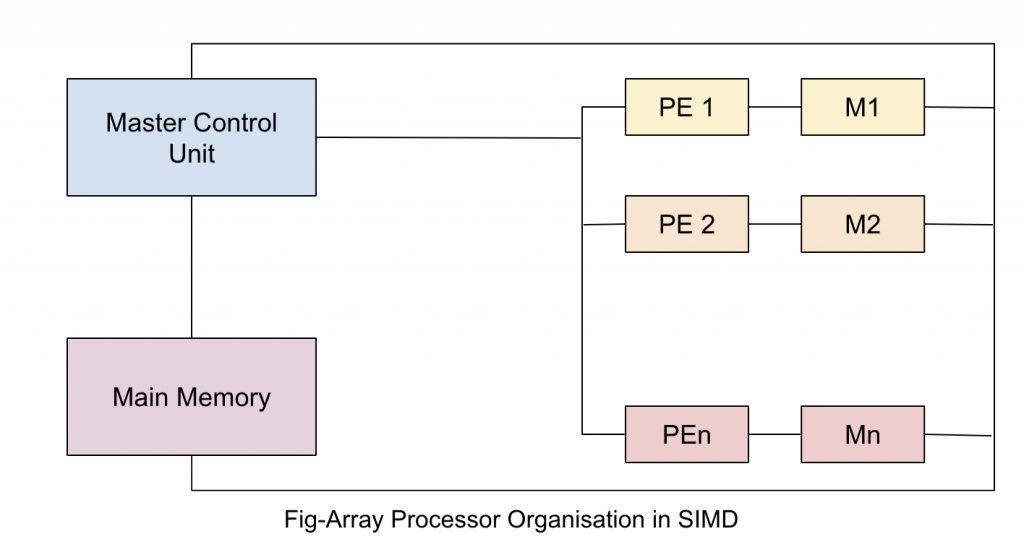
Arrays work by organizing a collection of elements of the same data type in a contiguous block of memory. The architecture of arrays involves memory allocation, element storage, and indexing for efficient access. Let’s explore how arrays work and their architecture:
Memory Allocation and Storage:
When an array is declared, memory is allocated for a specific number of elements, each of the same data type. The memory allocation is contiguous, meaning that the elements are stored one after another in memory without any gaps. This ensures efficient access, as elements can be accessed using their index and memory locations can be computed directly.
For example, in a C-style array of integers:
int numbers[5];
Memory is allocated to hold five integers in a row, allowing direct access to each element’s memory location.
Indexing and Access:
Array elements are accessed using their index, which represents their position in the array. The index starts from 0 for the first element and increments sequentially. The formula to calculate the memory location of an element at index i
is often:
memory_location = base_address + (i * element_size)
Where base_address
show the memory address of the first element and element_size
describe the size of each element in bytes.
Workflow of Array Access:
- Compute the memory location using the index and the formula above.
- Access the element directly at the calculated memory location.
Advantages of Array Architecture:
- Efficient Access: Accessing elements by index is efficient and constant time (O(1)) as it involves simple arithmetic calculations.
- Memory Locality: Array elements are stored in contiguous memory locations, improving cache utilization and reducing memory access latency.
- Predictable Memory Consumption: Arrays have a fixed size, ensuring predictable memory consumption and efficient memory management.
Limitations of Array Architecture:
- Fixed Size: The size of an array is fixed during declaration and cannot be changed dynamically.
- Memory Management: Arrays require memory management and careful index handling to prevent access errors or memory leaks.
- Efficiency vs. Dynamic Operations: Arrays excel in efficient element access and iteration, but they are less suitable for dynamic insertion, deletion, and resizing.
In languages like C, C++, and Java, arrays are a fundamental data structure with direct memory control. In higher-level languages, arrays might have some additional abstractions and automatic memory management. Understanding the architecture and behavior of arrays is crucial for efficient and correct programming when working with collections of data.
How to Install and Configure Arrays?
There are two ways to install and configure arrays:
- Using the command line:
- Open a command prompt and navigate to the directory where you want to install the arrays.
- Run the following command to install the arrays:
pip install arrays
- Using the Python Package Index (PyPI):
- Open a Python interpreter.
- Run the following command to install the arrays:
import pip
pip.main(['install', 'arrays'])
Once you have installed the arrays, you can configure them by editing the arrays.conf
file. This file is located in the etc
directory of your Python installation.
The arrays.conf
file contains the following configuration options:
- data_dir: The directory where the arrays data is stored.
- max_size: The maximum size of an array.
- num_arrays: The number of arrays to create.
You can configure the arrays according to your needs. For example, you can specify a different directory for the arrays data or you can increase the maximum size of an array.
Fundamental Tutorials of Arrays: Getting Started Step by Step
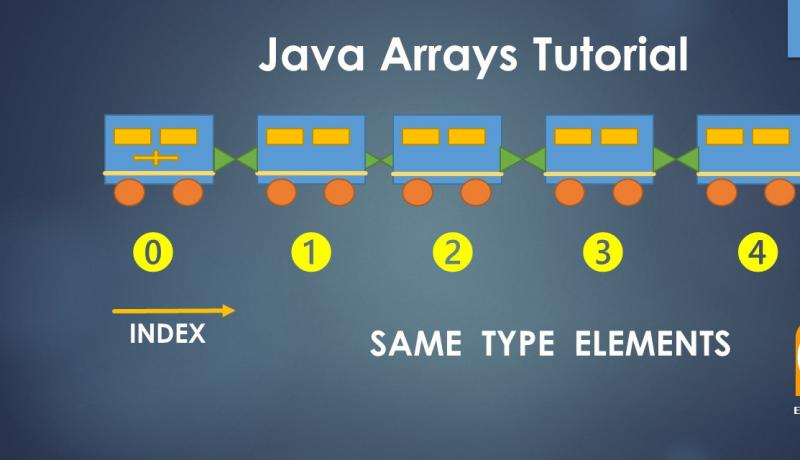
Here are some step-by-step fundamental tutorials of arrays:
- Create an array:
- Import the arrays module.
- Create an array by specifying the data type of the elements and the size of the array.
import arrays
array = arrays.Array(data_type='int', size=10)
- Store elements in an array:
Use the append() method to store elements in the array.
array.append(1)
array.append(2)
array.append(3)
- Access elements from an array:
Use the get() method to access elements from the array.
element = array.get(0)
print(element)
- Change elements in an array:
Use the set() method to change elements in the array.
array.set(0, 10)
print(array[0])
- Delete elements from an array:
Use the remove() method to delete elements from the array.
array.remove(1)
print(array)
- Resize an array:
Use the resize() method to resize the array.
array.resize(20)
print(array)
- Why Can’t I Make Create A New Folder on External Drive on Mac – Solved - April 28, 2024
- Tips on How to Become a DevOps Engineer - April 28, 2024
- Computer Programming Education Requirements – What You Need to Know - April 28, 2024