What is ASP.NET Core?
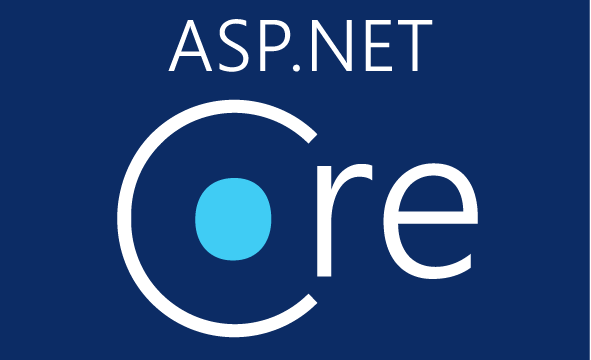
ASP.NET Core is an open-source, cross-platform framework for building modern, cloud-based, and internet-connected applications. Developed by Microsoft, ASP.NET Core is a redesigned, modular, and high-performance version of the original ASP.NET framework. It is designed to be lightweight, scalable, and suitable for building applications that can run on Windows, Linux, and macOS.
Key Features of ASP.NET Core:
- Cross-Platform:
- ASP.NET Core is designed to be cross-platform, allowing developers to build and run applications on Windows, Linux, and macOS.
- Open Source:
- ASP.NET Core is open-source, encouraging collaboration and community contributions. It is hosted on GitHub, and developers can actively participate in its development.
- Modular Architecture:
- ASP.NET Core has a modular and lightweight architecture that allows developers to include only the components needed for a particular application, reducing the overall footprint.
- .NET Core Runtime:
- ASP.NET Core applications run on the cross-platform .NET Core runtime, providing consistent behavior across different operating systems.
- Unified MVC and Web API Framework:
- ASP.NET Core unifies the previously separate ASP.NET MVC and Web API frameworks into a single, cohesive framework, making it easier to build both web pages and web APIs in the same application.
- Dependency Injection:
- ASP.NET Core includes built-in support for dependency injection, making it easy to manage and inject dependencies into components, promoting a modular and maintainable code structure.
- Razor Pages:
- Razor Pages is a lightweight web page-oriented framework that simplifies the development of web applications with less ceremony than traditional MVC applications.
- Entity Framework Core:
- ASP.NET Core includes Entity Framework Core, a lightweight, cross-platform Object-Relational Mapping (ORM) framework for data access.
- Middleware Pipeline:
- ASP.NET Core introduces a flexible middleware pipeline that allows developers to add, configure, and customize middleware components for handling various aspects of the HTTP request and response.
- Built-in Logging and Configuration:
- ASP.NET Core includes built-in support for logging and configuration, simplifying the process of logging events and managing application settings.
- Authentication and Authorization:
- ASP.NET Core provides built-in support for authentication and authorization, including integration with external identity providers and token-based authentication.
- SignalR:
- SignalR is a real-time communication library included with ASP.NET Core, enabling developers to build real-time, bi-directional communication between clients and servers.
What is top use cases of ASP.NET Core?
Top Use Cases of ASP.NET Core:
- Web Applications:
- ASP.NET Core is widely used for building modern and responsive web applications, ranging from small websites to large-scale enterprise applications.
- Web APIs:
- ASP.NET Core is well-suited for building RESTful APIs, providing a unified framework for developing web pages and web APIs within the same application.
- Microservices:
- ASP.NET Core is often used in microservices architectures, where lightweight and modular components are essential. Its cross-platform support is valuable in containerized environments.
- Cloud-Native Applications:
- ASP.NET Core is suitable for building cloud-native applications that can run on popular cloud platforms like Microsoft Azure, AWS, and Google Cloud Platform.
- Real-Time Applications:
- With SignalR, ASP.NET Core is used for building real-time applications such as chat applications, live dashboards, and collaborative tools.
- Internet of Things (IoT) Applications:
- ASP.NET Core can be employed in building applications for managing and interacting with IoT devices, especially in scenarios where cross-platform support is required.
- Cross-Platform Development:
- ASP.NET Core is ideal for organizations and developers who want to build applications that run on multiple platforms, allowing for flexibility and cost-effective development.
- Enterprise Applications:
- ASP.NET Core is used in building scalable and maintainable enterprise applications, taking advantage of its modular architecture and cross-platform support.
- Containerized Applications:
- ASP.NET Core applications can be easily containerized using technologies like Docker, making them portable and suitable for deployment in container orchestration systems.
- Single Page Applications (SPA):
- ASP.NET Core can be used in conjunction with client-side frameworks like Angular, React, or Vue.js to build powerful and interactive single-page applications.
ASP.NET Core’s versatility, performance, and cross-platform capabilities make it a popular choice for a wide range of application development scenarios, catering to the needs of modern software development.
What are feature of ASP.NET Core?
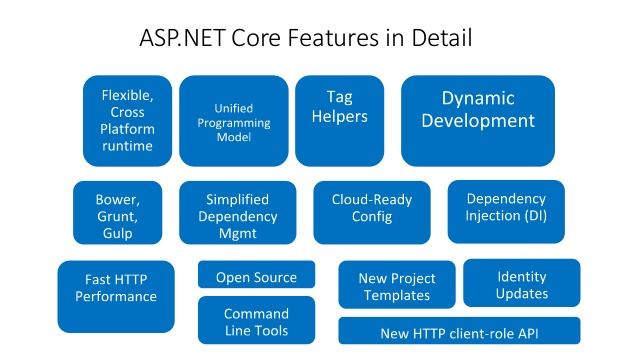
Features of ASP.NET Core:
- Cross-Platform:
- ASP.NET Core is designed to be cross-platform, allowing developers to build and run applications on Windows, Linux, and macOS.
- Open Source:
- ASP.NET Core is open-source, encouraging collaboration and community contributions. It is hosted on GitHub, and developers can actively participate in its development.
- Modular Architecture:
- ASP.NET Core has a modular and lightweight architecture that allows developers to include only the components needed for a particular application, reducing the overall footprint.
- Unified MVC and Web API Framework:
- ASP.NET Core unifies the previously separate ASP.NET MVC and Web API frameworks into a single, cohesive framework, making it easier to build both web pages and web APIs in the same application.
- Dependency Injection:
- ASP.NET Core includes built-in support for dependency injection, making it easy to manage and inject dependencies into components, promoting a modular and maintainable code structure.
- Razor Pages:
- Razor Pages is a lightweight web page-oriented framework that simplifies the development of web applications with less ceremony than traditional MVC applications.
- Entity Framework Core:
- ASP.NET Core includes Entity Framework Core, a lightweight, cross-platform Object-Relational Mapping (ORM) framework for data access.
- Middleware Pipeline:
- ASP.NET Core introduces a flexible middleware pipeline that allows developers to add, configure, and customize middleware components for handling various aspects of the HTTP request and response.
- Built-in Logging and Configuration:
- ASP.NET Core includes built-in support for logging and configuration, simplifying the process of logging events and managing application settings.
- Authentication and Authorization:
- ASP.NET Core provides built-in support for authentication and authorization, including integration with external identity providers and token-based authentication.
- SignalR:
- SignalR is a real-time communication library included with ASP.NET Core, enabling developers to build real-time, bi-directional communication between clients and servers.
- Cross-Origin Resource Sharing (CORS):
- ASP.NET Core includes support for handling Cross-Origin Resource Sharing, allowing controlled access to resources from different domains.
- Globalization and Localization:
- ASP.NET Core supports globalization and localization, making it easy to develop applications that can be adapted to different languages and regions.
- Configuration Management:
- ASP.NET Core provides a flexible and extensible configuration system that allows developers to configure an application using various sources, including JSON files, environment variables, and more.
What is the workflow of ASP.NET Core?
The workflow of developing an ASP.NET Core application typically involves the following steps:
- Environment Setup:
- Install the necessary development tools, including the .NET SDK and an integrated development environment (IDE) like Visual Studio or Visual Studio Code.
- Project Creation:
- Create a new ASP.NET Core project using the
dotnet new
command or through the IDE. Choose the desired project type, such as web application, web API, or Razor Pages.
- Create a new ASP.NET Core project using the
- Application Structure:
- Understand the structure of the ASP.NET Core application, including the
Startup.cs
file, which configures the middleware pipeline, and thewwwroot
folder for static files.
- Understand the structure of the ASP.NET Core application, including the
- Middleware Configuration:
- Configure middleware in the
Startup.cs
file to handle various aspects of the HTTP request and response, such as authentication, routing, logging, and error handling.
- Configure middleware in the
- Controllers and Views:
- Create controllers to handle requests and views (if using MVC) to define the presentation layer. Razor syntax is used in views for server-side rendering.
- Model Binding and Validation:
- Use model binding to automatically bind data from incoming requests to model objects. Implement validation to ensure the integrity of the data.
- Dependency Injection:
- Leverage the built-in dependency injection system to manage and inject dependencies into controllers and services.
- Entity Framework Core:
- Use Entity Framework Core to interact with databases. Define models, create migrations, and perform database operations.
- Authentication and Authorization:
- Implement authentication and authorization using built-in ASP.NET Core features or by integrating with external identity providers.
- Client-Side Development:
- Develop client-side components using JavaScript frameworks (if desired), such as Angular, React, or Vue.js, in conjunction with ASP.NET Core.
- Testing:
- Write unit tests and integration tests to ensure the reliability and correctness of the application.
- Logging and Monitoring:
- Implement logging to capture important events and errors. Consider integrating with monitoring tools for performance analysis.
- Configuration:
- Utilize the configuration system to manage application settings, secrets, and environment-specific configurations.
- Deployment:
- Deploy the ASP.NET Core application to a hosting environment, such as a web server, cloud platform, or containerized environment.
- Continuous Integration/Continuous Deployment (CI/CD):
- Set up CI/CD pipelines to automate the build, test, and deployment processes.
- Monitoring and Maintenance:
- Monitor the application in production, analyze logs, and perform maintenance tasks as needed. Address issues promptly and consider updates and improvements.
The workflow may vary based on the specific requirements of the project, the chosen architecture (MVC, Web API, etc.), and the development team’s preferences. ASP.NET Core’s flexibility, modular architecture, and cross-platform support make it suitable for a variety of web development scenarios.
How ASP.NET Core Works & Architecture?
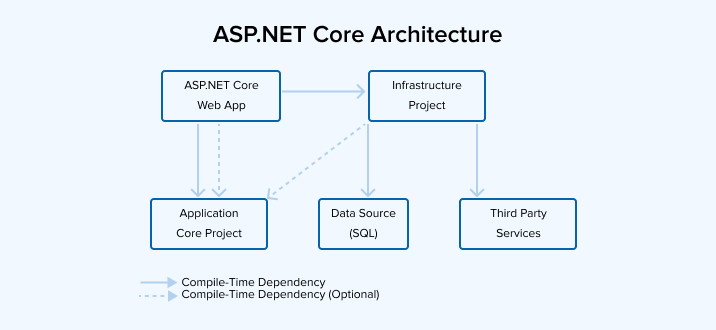
Here’s an overview of ASP.NET Core’s architecture and how it works:
Key Components:
- Modular Framework: ASP.NET Core is a lightweight and modular framework, allowing you to choose and install only the components you need for your application.
- Middleware Pipeline: Requests flow through a pipeline of middleware components, each performing specific tasks like authentication, routing, content compression, and more. You can create custom middleware for unique functionality.
- Dependency Injection (DI): ASP.NET Core uses DI to manage dependencies between components, promoting loose coupling and testability.
- Hosting Models: It supports various hosting models, including IIS, self-hosting, and deployment to containers or cloud platforms, providing flexibility in deployment choices.
Architecture:
- Request Processing:
- A request arrives at the web server.
- The server proceeds the request to the ASP.NET Core runtime.
- Middleware Pipeline:
- The request flows through the configured middleware components.
- Each middleware can inspect and modify the request/response, perform actions, and pass the request to the next middleware or the application endpoint.
- Controller Endpoint:
- If a controller endpoint matches the request, the runtime invokes the appropriate controller action method.
- View Rendering (optional):
- The controller action may return a view, which is rendered using a view engine (e.g., Razor).
- Response Generation:
- The response is generated and sent back to the client.
Additional Features:
- Cross-Platform: ASP.NET Core runs on Windows, macOS, and Linux, enabling development on different platforms.
- Cloud-Optimized: Designed for cloud and microservices architectures, it supports containerization and deployment to cloud platforms.
- Performance-Focused: Optimized for performance and scalability, handling high-traffic scenarios efficiently.
Benefits of ASP.NET Core Architecture:
- Flexibility: Modular design and middleware pipeline enable customization and adaptation to diverse application needs.
- Testability: Dependency injection facilitates unit testing and promoting code maintainability.
- Performance: Optimized for high-performance web applications.
- Cross-Platform: Enables development and deployment on various operating systems.
- Cloud-Ready: Supports modern cloud-based architectures and deployment scenarios.
How to Install and Configure ASP.NET Core?
Here are the general steps to install and configure ASP.NET Core:
Prerequisites:
- .NET SDK: Download and install the latest .NET SDK from the official website. It includes the runtime and tools for building ASP.NET Core applications.
- Code Editor: Choose a preferred code editor like Visual Studio their official website, Visual Studio Code, or any other you prefer.
Installation:
- Using Visual Studio:
- Create a new ASP.NET Core project template. The necessary components will be installed automatically.
- Using the .NET CLI:
- Open a terminal and run the command:
dotnet new web
to create a new ASP.NET Core project.
- Open a terminal and run the command:
Configuration:
- Startup Class: Configure middleware, services, and application settings in the
Startup.cs
file. appsettings.json
File: Store application configuration settings in this file.- Environment Variables: Access configuration from environment variables using
IConfiguration
.
Running the Application:
- Visual Studio: Use the built-in debugging features to run and test your application.
- .NET CLI: From the project directory, run:
dotnet run
to start the application.
Deployment:
- Self-Hosting: Publish the application as a standalone executable for deployment without a web server.
- IIS: Configure IIS to host ASP.NET Core applications.
- Containers: Package the application in a Docker container for deployment to container platforms.
- Cloud Platforms: Deploy to cloud environments like Azure App Service or AWS Elastic Beanstalk.
Important Notes:
- Virtual Environments: Consider using virtual environments to isolate project dependencies and avoid conflicts.
- Package Management: Use NuGet Package Manager to manage additional libraries and frameworks for your application.
If you encounter any issues or have specific questions about your setup, feel free to provide more details about your environment (OS, Visual Studio version, etc.) for tailored guidance.
Fundamental Tutorials of ASP.NET Core: Getting started Step by Step
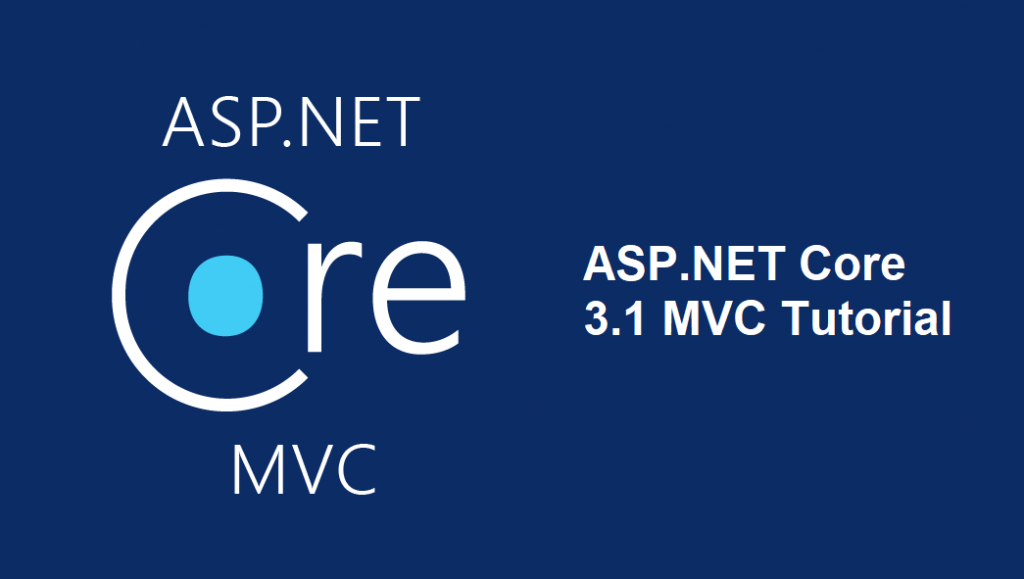
Following is a step-by-step guide to creating a fundamental ASP.NET Core web application, incorporating images for key steps:
1. Set up Your Development Environment:
- Install .NET Core: Download and install the latest .NET Core SDK from their official website. Ensure you choose the correct installer for your operating system.
- Install an IDE: Choose a code editor or IDE like Visual Studio Code (free, cross-platform) or Visual Studio (full-featured, Windows-focused).
2. Create a New ASP.NET Core Project:
- In Visual Studio Code:
- Open the Command Palette (Ctrl+Shift+P).
- Type “dotnet new” and select “ASP.NET Core Web App”.
- Choose the desired project template (e.g., “Empty” for a basic application).
- In Visual Studio:
- Go to File > New > Project.
- Choose “ASP.NET Core Web Application” and select a template.
3. Explore the Project Structure:
Image of ASP.NET Core project structure
- Key folders:
wwwroot
: Contains static files like HTML, CSS, JavaScript.Controllers
: Houses controller classes that handle requests.Views
: Stores view files for rendering UI.Models
: Holds data model classes.Startup.cs
: Configures the application.Program.cs
: Entry point for the application.
4. Write a Simple Controller:
- In the
Controllers
folder, create a new controller class (e.g.,HomeController.cs
). - Add a simple action method to handle a request:
C#
public class HomeController : Controller
{
public IActionResult Index()
{
return View();
}
}
5. Add a View:
- In the
Views
folder, create a view file matching the action name (Index.cshtml
). - Write basic HTML content:
HTML
<h1>Hello, ASP.NET Core!</h1>
6. Run the Application:
- In Visual Studio Code:
- Open the integrated terminal (Ctrl+`).
- Type “dotnet run” to start the application.
- In Visual Studio:
- Press F5 or click the “Start” button.
7. Access the Application:
- Open a web browser and navigate to
http://localhost:5000
(or the specified port). - You should see the “Hello, ASP.NET Core!” message rendered by your view.
Points to Remember:
- Explore other project templates: Try different templates for web APIs, Razor Pages, or more complex applications.
- Learn about routing: Understand how ASP.NET Core maps URLs to controller actions.
- Work with models and views: Handle data and create dynamic UI elements.
- Integrate with databases: Use Entity Framework Core for data access.
- Implement authentication and authorization: Secure your applications.
- Deploy to production: Publish your apps to hosting environments.
- Mutual of Omaha: Selection and Interview process, Questions/Answers - April 15, 2024
- AES: Selection and Interview process, Questions/Answers - April 15, 2024
- Amphenol: Selection and Interview process, Questions/Answers - April 15, 2024