What is ASP.NET MVC?
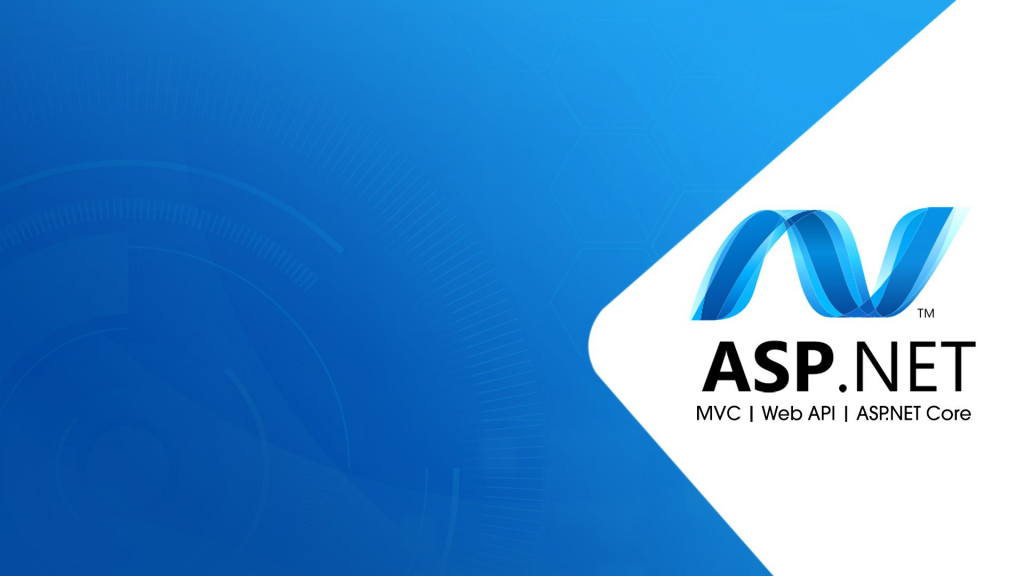
ASP.NET MVC (Model-View-Controller) is a web application framework developed by Microsoft for building dynamic, data-driven web applications. It is part of the broader ASP.NET platform and provides a pattern-based way to build scalable and maintainable web applications. ASP.NET MVC follows the MVC architectural pattern, which separates an application into three main components: Model, View, and Controller.
Following is a brief overview of each component in ASP.NET MVC:
- Model: Represents the application’s data and business logic. It is responsible for retrieving and storing data, as well as defining the rules and behaviors that govern the application.
- View: Symbolizes the user interface and is responsible for displaying the data to the user. In ASP.NET MVC, views are typically created using the Razor view engine, which allows developers to embed C# code within HTML markup.
- Controller: Handles user input and updates the model and view accordingly. Controllers receive input from users, process that input, interact with the model to retrieve or update data, and then pass the appropriate data to the view for display.
What is top use cases of ASP.NET MVC?
Top Use Cases of ASP.NET MVC:
- Web Application Development: ASP.NET MVC is primarily used for building dynamic, interactive web applications. It provides a structured and organized way to develop applications, making it suitable for a wide range of web development projects.
- Enterprise-level Applications: ASP.NET MVC is often chosen for developing large-scale enterprise applications where maintainability, scalability, and separation of concerns are critical. Its modular architecture facilitates the development of complex systems.
- Content Management Systems (CMS): Developers use ASP.NET MVC to build custom content management systems, allowing businesses to manage and update their website content efficiently.
- E-commerce Platforms: ASP.NET MVC is suitable for building e-commerce platforms and online shopping websites. It provides the flexibility to create complex business logic and integrate with databases and external services.
- Line-of-Business (LOB) Applications: Many business applications, including internal tools, project management systems, and customer relationship management (CRM) systems, are built using ASP.NET MVC due to its robust features and integration capabilities.
- API Development: ASP.NET MVC can be used to build RESTful APIs, enabling the development of backend services that power mobile apps, single-page applications, and other API-driven projects.
- Real-time Applications: ASP.NET MVC can be combined with technologies like SignalR to develop real-time, interactive applications that require instant updates and communication between the server and clients.
- Cross-platform Development: With the introduction of .NET Core, which is a cross-platform, open-source version of the .NET Framework, ASP.NET MVC applications can be developed and deployed on various platforms, including Windows, Linux, and macOS.
- Custom Web Solutions: ASP.NET MVC provides developers with the flexibility to create custom solutions tailored to specific business requirements. Its extensibility allows for the integration of third-party libraries and components.
- Web Development with Visual Studio: ASP.NET MVC is well-integrated with Microsoft Visual Studio, a popular development environment. Developers can leverage Visual Studio’s powerful features for code editing, debugging, and project management.
ASP.NET MVC is a versatile framework that caters to a broad range of web development scenarios. Its adoption is often influenced by factors such as the existing technology stack, development team expertise, and project requirements.
What are feature of ASP.NET MVC?
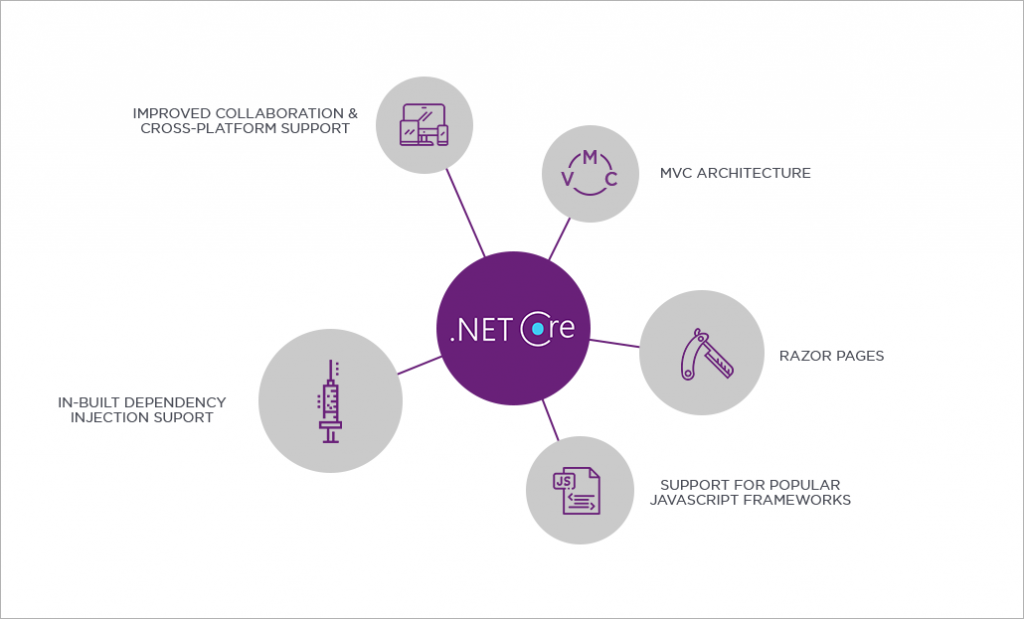
Features of ASP.NET MVC:
- MVC Architecture: ASP.NET MVC follows the Model-View-Controller architectural pattern, which promotes separation of concerns. This division enhances code organization, maintainability, and testability.
- Razor View Engine: ASP.NET MVC uses the Razor view engine, which allows developers to embed server-side code (C#) within HTML markup. This provides a clean and concise syntax for creating dynamic views.
- Routing: ASP.NET MVC includes a powerful routing system that enables developers to define clean and search engine-friendly URLs. Routes map incoming URLs to controller actions, allowing for a flexible and customizable URL structure.
- Convention over Configuration: ASP.NET MVC promotes convention-based development, reducing the need for configuration. Sensible defaults are provided, but developers have the flexibility to customize as needed.
- Extensibility: The framework is highly extensible, allowing developers to integrate third-party libraries, tools, and components seamlessly. This extensibility is valuable for accommodating various project requirements.
- Model Binding: ASP.NET MVC simplifies the process of binding HTTP request data to model objects. This feature is particularly useful for handling form submissions and processing user input.
- Action Filters: Action filters enable developers to apply cross-cutting concerns such as authentication, authorization, caching, and logging to controller actions. This forwords code reuse and enhances modularity.
- Unit Testing Support: ASP.NET MVC is designed with testability in mind. The separation of concerns in the MVC pattern makes it easier to write unit tests for controllers, models, and other components.
- Validation: The framework provides built-in validation features for both client-side and server-side validation. Developers can use data annotations or implement custom validation logic.
- HTML Helpers: ASP.NET MVC includes HTML helpers, which are methods that generate HTML markup. These helpers simplify the process of rendering HTML elements and forms, improving code readability.
- Areas: Areas allow developers to organize large projects into smaller, manageable sections. Each area can have its own controllers, views, and models, promoting a modular and scalable project structure.
- Dependency Injection: ASP.NET MVC supports dependency injection, allowing developers to inject dependencies into controllers and other components. This promotes a loosely coupled and more maintainable codebase.
What is the workflow of ASP.NET MVC?
The workflow of ASP.NET MVC involves the following steps:
- Request Handling:
- An HTTP request is received by the ASP.NET MVC application.
- The routing system matches the incoming URL to a specific controller and action based on defined routes.
- Controller Handling:
- The selected controller’s action method is invoked to process the request.
- The controller is responsible for handling user input, interacting with the model, and determining the appropriate response.
- Model Interaction:
- The controller interacts with the model to retrieve or update data. Models encapsulate the application’s business logic and data access operations.
- View Rendering:
- The controller selects the appropriate view to render. Views are responsible for showing data to the user.
- The Razor view engine processes the view, combining HTML markup with server-side code to generate the final response.
- Response Sent to the Browser:
- The generated response, typically an HTML page, is sent back to the user’s browser.
- User Interaction:
- The user interacts with the rendered view, triggering new HTTP requests as needed.
- Form Submission and Model Binding:
- If a form is submitted, the model binding mechanism binds the form data to the corresponding model objects in the controller.
- Validation and Action Filters:
- The framework performs validation based on data annotations or custom logic.
- Action filters may be applied to perform additional processing before or after the execution of the controller action.
- Response to the Browser:
- The updated response is sent back to the user’s browser, completing the round-trip cycle.
- Unit Testing:
- Developers can write unit tests to verify the correctness of controller actions, models, and other components.
ASP.NET MVC’s workflow is designed to be flexible and extensible, allowing developers to customize and extend various parts of the process to meet the requirements of their applications. The separation of concerns and adherence to the MVC pattern contribute to a clean and maintainable codebase.
How ASP.NET MVC Works & Architecture?
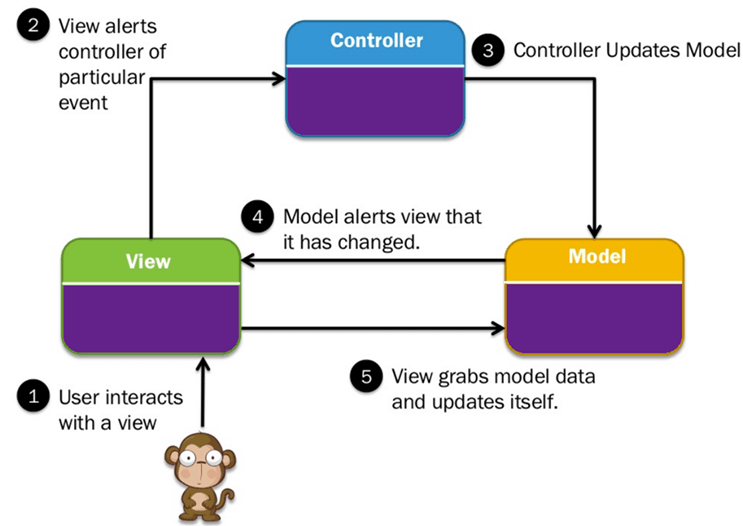
ASP.NET MVC (Model-View-Controller) is a web application framework for building dynamic websites and web applications using the C# programming language. It follows the MVC architectural pattern, separating the application logic into distinct layers for better maintainability and code organization.
Following is an overview of how ASP.NET MVC works and its architecture:
1. Core Principles:
- Model-View-Controller (MVC) Architecture: Separates the application logic into models, views, and controllers.
- Views as Templates: Uses Razor syntax to define dynamic and data-driven HTML templates.
- Routing and URL Mapping: Maps URLs to specific controller actions.
- Dependency Injection: Simplifies object management and promotes loose coupling.
- Model Binding: Automatically binds data from user requests to models.
2. Architectural Components:
- Models: Represent data entities and interact with the database or other data sources.
- Views: Responsible for presenting the user interface and are generated using Razor templates.
- Controllers: Handle user requests, interact with models, and select the appropriate view to render.
- Routes: Define mappings between URLs and controller actions.
- Razor Syntax: Embedded within HTML templates to render dynamic content and data.
- Helper Classes: Provide various utility functions for common tasks within the framework.
3. Workflow:
- User sends a request: This could be a browser navigating to a URL or submitting a form.
- Request reaches the router: The router matches the URL to a defined route.
- Route invokes the controller action: The controller action retrieves data from models, performs logic, and selects the appropriate view.
- Controller renders the view: The Razor template engine combines data with the selected view and generates the final HTML response.
- HTML response sent to the user: The browser receives the generated HTML and renders the user interface.
4. Benefits:
- Clean Separation of Concerns: Makes code easier to maintain and understand.
- Testability: Each layer can be tested independently.
- Flexibility and Extensibility: Supports various development patterns and integrations.
- Large Community and Ecosystem: Numerous resources and libraries available.
Points to Remember:
- Understanding the MVC architecture is crucial for developing effective ASP.NET MVC applications.
- Familiarize yourself with Razor syntax for creating dynamic and data-driven views.
- Utilize the available resources and communities for learning, support, and inspiration.
By learning the fundamentals of ASP.NET MVC and actively engaging with the community, you can unlock its full potential and build robust, maintainable, and dynamic web applications with ease.
How to Install and Configure ASP.NET MVC?
Installing and Configuring ASP.NET MVC:
Let’s have a look at a step-by-step guide on how to install and configure ASP.NET MVC:
1. Prerequisites:
- Operating System: Windows 10 or later
- Visual Studio: Install Visual Studio 2022 or later with the ASP.NET and web development workload.
- .NET SDK: Ensure you have the latest .NET SDK installed for your target framework.
2. Creating a New Project:
- Open Visual Studio and generate a new project.
- Select ASP.NET Core Web App (Model-View-Controller) from the templates.
- Choose a project name and click Create.
3. Configuring the Project:
- In the Configure your new project dialog, select a .NET version. For new projects, it’s recommended to use the latest version.
- Choose No HTTPS for the development environment.
- You can choose to enable Docker support if you plan to use it.
- Click Create.
4. Running the Application:
- Press
F5
or click the Run button to start the application. - The application will be launched in your default web browser.
5. Additional Configuration:
- You can further configure your application by modifying settings in the following files:
appsettings.json
: Stores configuration settings like database connection strings, logging options, and more.Startup.cs
: Defines the application startup logic, including configuring services and middleware.
- Explore the ASP.NET MVC documentation for detailed configuration options and best practices.
Important Points:
- Ensure you have the necessary prerequisites installed before proceeding.
- Follow the steps carefully when creating and configuring your ASP.NET MVC project.
- Refer to the extensive documentation and resources available for detailed guidance and support.
By following these steps and utilizing the available resources, you can efficiently install and configure ASP.NET MVC to start building your web applications.
Fundamental Tutorials of ASP.NET MVC: Getting started Step by Step
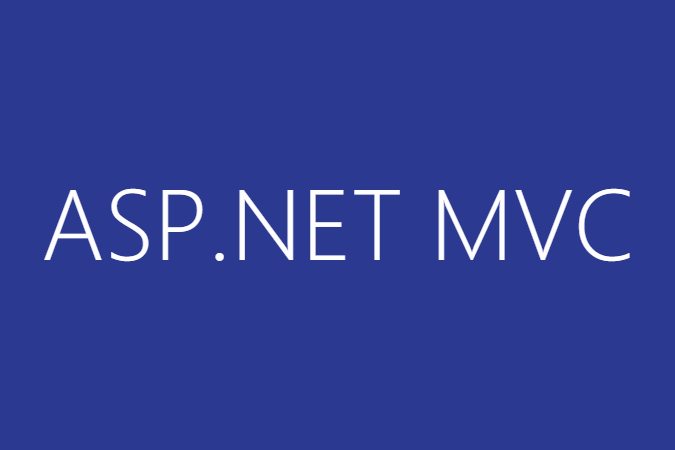
Following is a step-by-step breakdown of fundamental ASP.NET MVC tutorials to help you get started:
1. Setting Up the Environment:
- Install Visual Studio: Download and install Visual Studio 2022 or later with the ASP.NET and web development workload.
- Ensure .NET SDK: Verify you have the latest .NET SDK installed for your target framework.
- Generate a New Project: Open Visual Studio and generate a new ASP.NET Core Web App (Model-View-Controller) project.
2. Understanding the MVC Pattern:
- Learn about the MVC architecture and its components: Models, Views, and Controllers.
- Understand how requests flow through the MVC pipeline and how each component interacts with others.
- Explore the default project structure and familiarize yourself with key files like
Startup.cs
andappsettings.json
.
3. Building Your First Controller:
- Create a new controller class using Visual Studio’s scaffolding feature.
- Define action methods to handle various HTTP requests (GET, POST, etc.).
- Implement logic to interact with models and retrieve data.
- Use the
View
method to return the appropriate view for the action.
4. Creating and Using Razor Views:
- Understand the basic syntax of Razor templates for building dynamic web pages.
- Learn how to bind data from models to your views using Razor expressions and directives.
- Design layout templates to achieve consistent page structure and reduce code duplication.
- Utilize HTML helpers to simplify common tasks like generating forms and links.
5. Database Access and CRUD Operations:
- Add a database connection string to your
appsettings.json
file. - Implement data access logic using Entity Framework Core or other data access technologies.
- Perform CRUD operations (Create, Read, Update, Delete) within your controller actions.
- Display and manipulate data in your Razor views.
6. Implementing Security and Authentication:
- Learn about ASP.NET Core Identity for user management and authentication.
- Implement user registration, login, and authorization mechanisms.
- Secure your application against common vulnerabilities like SQL injection and cross-site scripting.
7. Routing and URL Mapping:
- Configure routes to map URLs to specific controller actions.
- Explore different types of routes and route parameters.
- Define route attributes directly on controller actions.
8. Validation and Error Handling:
- Implement data validation to ensure user input is correct and complete.
- Use model validation attributes and Razor helpers for data validation.
- Manage errors gracefully and give informative error messages to users.
9. Additional Features:
- Explore various features like dependency injection, middleware, and logging.
- Use Razor components for building reusable UI components.
- Utilize built-in services like configuration and caching for improved performance.
Remember:
- Start with the basics and gradually progress to more complex topics.
- Practice building simple applications to solidify your understanding.
- Utilize online resources and tutorials for guidance and learning.
- Join online communities and forums to ask questions and learn from other developers.
By following these steps and actively engaging with the process, you can acquire the necessary skills and knowledge to effectively build web applications using ASP.NET MVC.
- Mutual of Omaha: Selection and Interview process, Questions/Answers - April 15, 2024
- AES: Selection and Interview process, Questions/Answers - April 15, 2024
- Amphenol: Selection and Interview process, Questions/Answers - April 15, 2024