What is C?
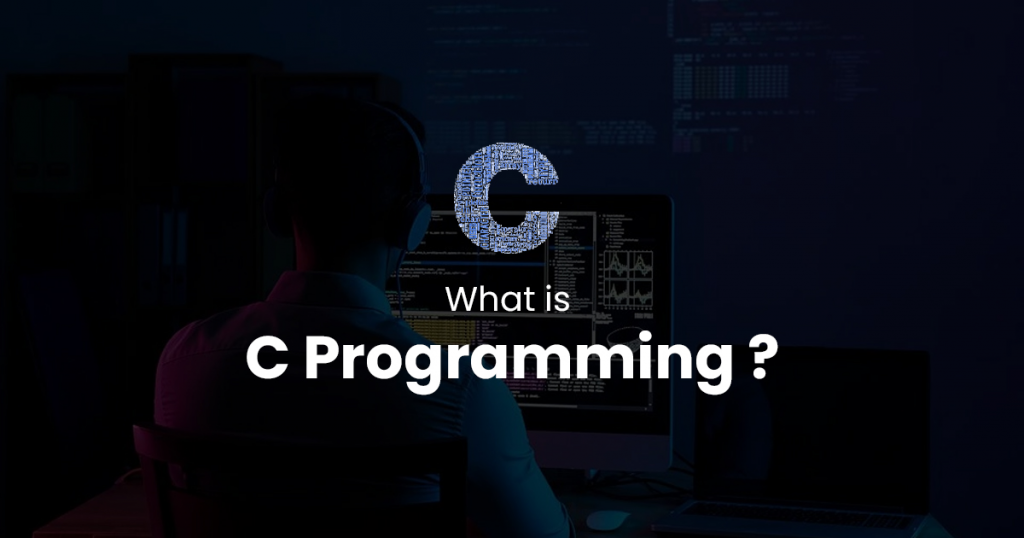
C is a high-level programming language that is often used for system programming and embedded systems. It has since become one of the most widely used and influential programming languages, serving as the foundation for many other languages and systems. C is known for its simplicity, efficiency, and low-level capabilities, making it suitable for system programming, application development, and more.
What is the top use cases of C?
Here are some of the top use cases of the C programming language:
- System Programming: C is commonly used for system programming tasks like developing operating systems, kernels, device drivers, and firmware due to its low-level memory manipulation and efficient execution.
- Embedded Systems: C’s small memory footprint and direct access to hardware make it ideal for programming embedded systems, which are often found in devices like microcontrollers, IoT devices, and robotics.
- Compilers and Interpreters: Many compilers and interpreters for other programming languages are written in C due to its ability to generate efficient machine code.
- Game Development: C is widely used in the game development industry for creating game engines, graphics libraries, and performance-critical components of video games.
- Networking and Network Protocol Development: C’s low-level socket programming capabilities make it suitable for developing network applications, protocols, and server software.
- Operating System Development: C is often the language of choice for building the core components of operating systems, such as the kernel, file systems, and system utilities.
- Performance-Critical Applications: C’s efficiency and ability to work with memory directly make it well-suited for applications that require high performance, such as scientific simulations, signal processing, and real-time systems.
- Application Development: Although C is lower-level compared to some modern languages, it’s still used to develop applications where performance and control are crucial, such as image and video processing software.
- Cross-Platform Development: C code can often be compiled to run on multiple platforms with minimal modifications, making it suitable for cross-platform software development.
- Legacy Code Maintenance: Many older systems and software are written in C, so developers may need to maintain or upgrade these systems by working with existing C codebases.
- Security Software: C is used to develop security software like encryption algorithms, secure communications protocols, and cybersecurity tools due to its control over memory and efficient code execution.
- Database Management Systems: C is used in the development of database management systems (DBMS) and database engines for efficient data storage and retrieval.
C’s versatility and capability to work closely with hardware and memory have led to its continued relevance in various domains, particularly those that demand efficient performance and low-level control over system resources.
What are the features of C?
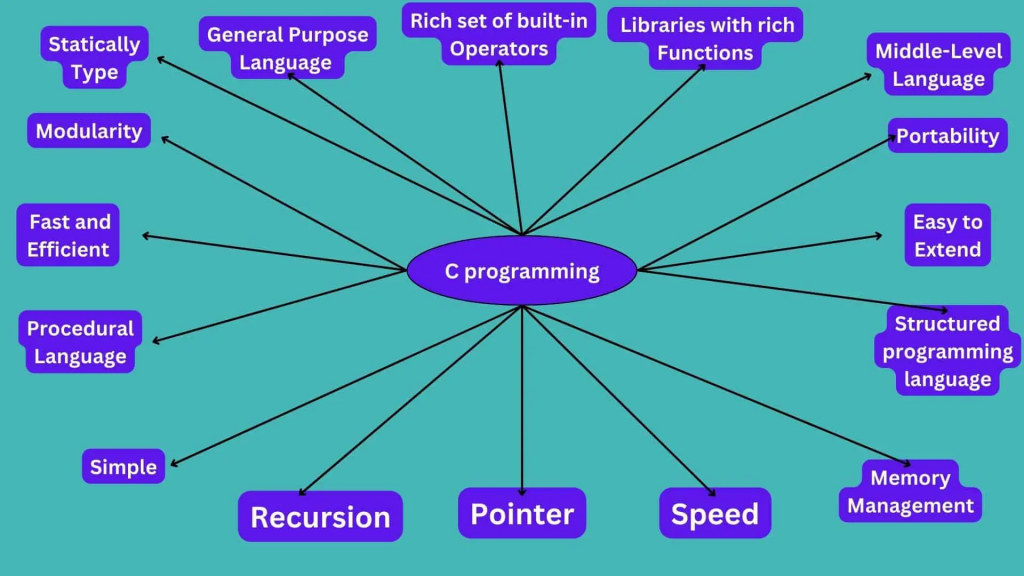
- Simple Syntax: C has a straightforward and concise syntax, making it relatively easy to learn and write code.
- Portability: C programs can be compiled and run on a wide range of hardware and operating systems, making it a versatile choice for developing applications that need to be deployed on different platforms.
- Efficiency: C provides direct access to memory and hardware resources, allowing for efficient manipulation of data and execution of code.
- Low-Level Programming: C offers low-level features like pointer manipulation and bitwise operations, making it suitable for system programming and hardware control.
- Modularity: C supports modular programming through functions, allowing code to be organized into reusable and manageable units.
- Structured Programming: C supports structured programming constructs like loops, conditionals, and functions, aiding in creating organized and maintainable code.
- Rich Standard Library: C includes a rich standard library that provides functions for common tasks like I/O operations, string manipulation, and mathematical calculations.
- Dynamic Memory Allocation: C allows dynamic memory allocation using functions like
malloc
andfree
, enabling efficient use of memory resources. - Pointers: Pointers are a powerful feature in C, allowing direct memory manipulation and creating complex data structures.
- Data Types: C provides basic and user-defined data types, enabling developers to work with various data structures and create custom data types.
- Bitwise Manipulation: C supports bitwise operators, enabling manipulation of individual bits within data.
- Preprocessor Directives: Preprocessor directives like
#include
and#define
allow code organization and conditional compilation. - Compatibility: C is often used as an interface between different programming languages and for creating libraries that can be called from other languages.
What is the workflow of C?
- Code Writing: Developers write C code using a text editor or integrated development environment (IDE). C code consists of functions, variables, control structures, and other constructs.
- Compiling: The C code is then compiled using a C compiler, which translates the human-readable code into machine-readable binary code (object code).
- Object File Generation: The compiler produces object files for each source code file. These object files contain machine code and unresolved references to external functions.
- Linking: If the program consists of multiple source code files, the linker combines the object files, resolves references to external functions, and generates an executable file.
- Executable Generation: The linked executable file is generated, which contains machine code that the computer’s CPU can understand and execute.
- Execution: The executable file is executed by the operating system, and the program’s instructions are carried out sequentially.
- Debugging and Testing: Developers may use debugging tools and techniques to identify and fix errors or bugs in the code.
- Optimization: Programmers can optimize the code for performance by using techniques like algorithmic improvements and efficient memory management.
- Documentation: Good programming practices include documenting the code, providing comments, and creating user manuals or documentation for future reference.
- Maintenance: As requirements change or bugs are discovered, developers may need to update and maintain the codebase over time.
The workflow of C involves writing code, compiling it, linking object files, generating an executable, and running the program. This sequence is followed to develop and execute C programs, allowing developers to create efficient and functional software.
How C Works & Architecture?
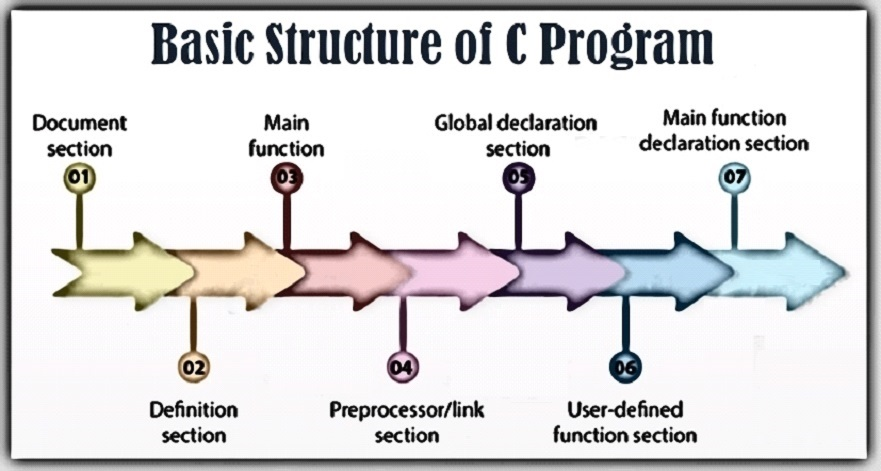
C is a compiled programming language, which means that it goes through several stages of transformation before the code is executed by the computer’s hardware. Understanding how C works and its underlying architecture involves exploring the compilation process and the interaction between the source code, compiler, linker, and hardware.
How C Works:
- Source Code: You start by writing your C code using a text editor or an integrated development environment (IDE).
- Preprocessing: The C preprocessor (
cpp
) processes the source code before actual compilation. It handles preprocessor directives like#include
,#define
, and conditional compilation (#ifdef
,#ifndef
, etc.). - Compilation: The C compiler (
gcc
for example) translates the preprocessed source code into assembly code. It performs syntax checking, type checking, and generates assembly code that represents the program’s logic. - Assembly Language: The generated assembly code is a human-readable representation of the program’s logic in a low-level language. It uses mnemonic instructions that correspond to the operations supported by the computer’s architecture.
- Assembly to Object Code: The assembly code is then converted into machine code, known as object code. This conversion is performed by the assembler. The object code consists of binary instructions that the computer’s CPU can understand and execute.
- Linking: If your program consists of multiple source files or uses external libraries, the linker (
ld
for example) combines the object files generated from different source files and resolves references to external functions and symbols. It creates a single executable file. - Executable Generation: The linker generates an executable file that contains the compiled code, data, and information necessary for the operating system to run the program. This executable file is what you run to execute your program.
- Execution: When you run the executable, the operating system loads it into memory. The CPU reads and executes the machine code instructions stored in the executable, performing the computations and tasks defined by the program.
C Architecture:
C itself doesn’t have a fixed architecture like hardware or an operating system. Instead, it generates machine code that interacts directly with the computer’s hardware architecture and follows the architecture of the target machine.
The underlying architecture that C code interacts with includes:
- CPU (Central Processing Unit): The CPU executes the machine code instructions generated by the C compiler. It performs arithmetic, logic operations, and controls the flow of the program.
- Memory: The compiled C code interacts with different regions of memory, such as the stack, heap, and data segments. Pointers and memory management are important aspects of C’s interaction with memory.
- Operating System: The operating system is responsible for managing resources, providing abstractions for hardware components, and enabling programs to run on the hardware. C code can interact with the OS through system calls.
- I/O Devices: C code can interact with input and output devices like keyboards, screens, files, and network sockets using standard I/O functions.
- Libraries: C code can use standard libraries (like
stdio.h
,stdlib.h
, etc.) and external libraries to perform various tasks without needing to write the code from scratch.
C works by taking your source code through a series of stages: preprocessing, compilation, assembly, linking, and execution. The resulting executable interacts directly with the computer’s hardware architecture and follows the architecture of the target system, including the CPU, memory, OS, and I/O devices.
How to Install and Configure C?
To install and configure C, you can follow these steps:
- Install a C compiler
There are many different C compilers available, such as GCC, Clang, and MinGW. You can choose the compiler that is best suited for your needs.
- Set up your development environment
You will need a text editor or IDE to write your C code. You can use any text editor that you are comfortable with, or you can use an IDE such as Visual Studio Code or Eclipse.
- Create a new C project
Once you have installed a C compiler and set up your development environment, you can create a new C project. You can do this by generating a new file with the .c extension.
- Write your C code
Start writing your C code in the new file. You can use the online C tutorial to learn the basics of C programming.
- Compile your C code
Once you have written your C code, you need to compile it into an executable file. You can do this by running the C compiler on your code.
- Run your C program
Once you have compiled your C code, you can run it by executing the executable file.
Here are some additional things to keep in mind when installing and configuring C:
- The specific steps for installing and configuring C will vary depending on your operating system.
- You may need to install additional libraries or packages in order to use C features such as graphics or networking.
- There are many resources available outside & online to aid you learn C programming.
Here are some of the most popular C compilers:
- GCC (GNU Compiler Collection) is a free and open-source compiler that supports many different programming languages, including C.
- Clang is a C and C++ compiler developed by Apple. It is also free and open-source.
- MinGW is a free and open-source compiler for Windows that supports C and C++.
The following are the most popular IDEs for C programming:
- Visual Studio Code is a free and open-source IDE that supports many different programming languages, including C.
- Eclipse is a free and open-source IDE that supports many different programming languages, including C.
- Code::Blocks is a free and open-source IDE that supports C and C++.
Fundamental Tutorials of C: Getting Started Step by Step
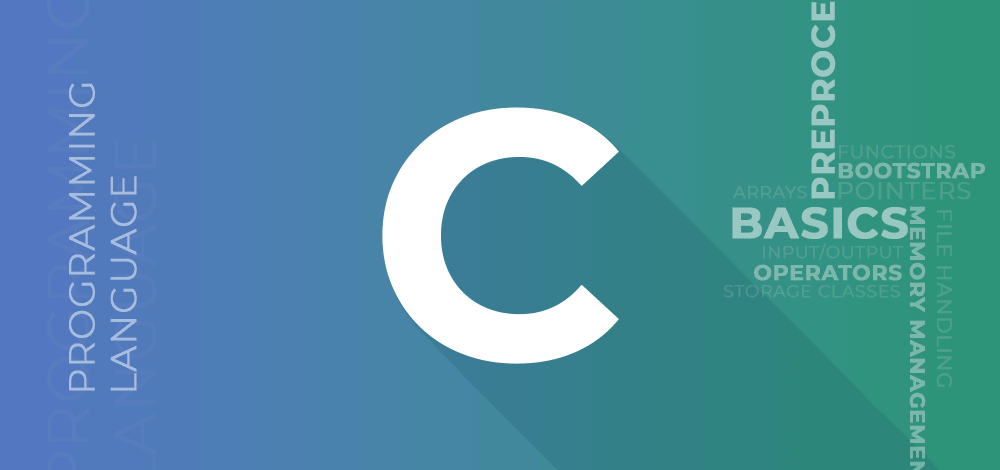
The following steps are the fundamental tutorials of C:
- Learn the basics of C
The first step is to learn the basics of C programming. This includes learning about variables, data types, operators, control statements, and functions. You can find many online tutorials and books that can help you learn the basics of C.
Here are some of the most important concepts to learn about when starting out with C:
* Variables: Variables are applied to store data in memory. They are assigned a name and a data type.
* Data types: Data types define the kind of data that can be stored in a variable. There are many different data types in C, such as integers, floats, and strings.
* Operators: Operators are applied to execute operations on data. There are many different operators in C, such as arithmetic operators, logical operators, and relational operators.
* Control statements: Control statements allow you to control the flow of execution of your program. There are many different control statements in C, such as if statements, for loops, and while loops.
* Functions: Functions are blocks of code that perform specific tasks and can be reused multiple times within a program. They are like mini-programs within a larger program, designed to carry out a particular operation or set of operations.
- Write your first C program
Once you have learned the basics of C, you can start writing your first C program. A simple C program might print “Hello, world!” to the console. You can find many online tutorials and examples that can help you write your first C program.
Here is an example of a simple C program that prints “Hello, world!” to the console:
#include <stdio.h>
int main() {
printf("Hello, world!\n");
return 0;
}
- Learn about C libraries
C has a rich library of functions that you can use to perform common tasks, such as reading and writing files, manipulating strings, and working with graphics. You can find many online tutorials and documentation that can help you learn about C libraries.
Here are some of the most important C libraries:
* The standard library: The standard library provides a variety of functions for performing basic tasks such as reading and writing files, manipulating strings, and working with numbers.
* The math library: The math library provides a variety of functions for performing mathematical operations such as trigonometric functions, logarithms, and square roots.
* The graphics library: The graphics library provides functions for drawing shapes, images, and text on the screen.
- Practice writing C programs
The ideal method to learn C programming is by practicing. Write as many C programs as you can, and try to solve different problems using C. You can find many online challenges and exercises that can help you practice your C programming skills.
- Join a C programming community
There are many online communities where you can ask questions and get help from other C programmers. Joining a community can be a great way to learn from others and get feedback on your own code.
Here are some of the best online communities for C programmers:
* Stack Overflow: Stack Overflow is a platform where developers could collaborate and help each other solve coding problems. You can ask questions about C programming and get help from other programmers.
* Reddit: Reddit is a social news website where you can find communities dedicated to C programming. You can ask questions and get help from other programmers in these communities.
* GitHub: GitHub is a code hosting platform where you can find C code examples and projects. You can also contribute to C projects on GitHub.
- Why Can’t I Make Create A New Folder on External Drive on Mac – Solved - April 28, 2024
- Tips on How to Become a DevOps Engineer - April 28, 2024
- Computer Programming Education Requirements – What You Need to Know - April 28, 2024