What is Dart?
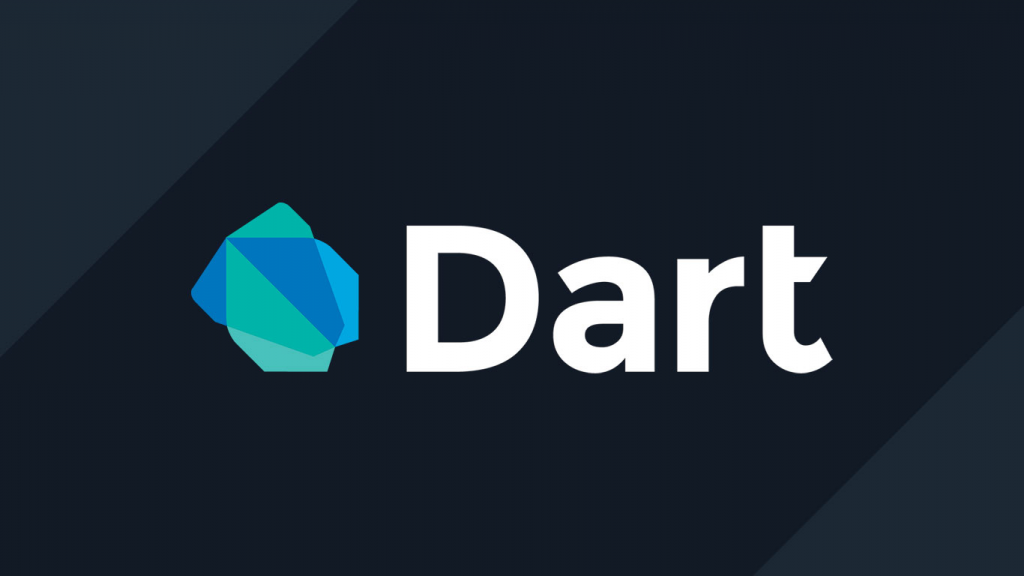
Dart is a programming language developed by Google that is designed for building web, mobile, and server applications. Dart is known for its simplicity, productivity, and strong support for building modern, reactive, and scalable applications. It is often used in combination with the Flutter framework for building cross-platform mobile applications.
Key features of Dart include:
- Object-Oriented: Dart is an object-oriented programming language, which means it is built around the concept of objects, classes, and inheritance.
- Strongly Typed: Dart is a statically-typed language, providing type safety at compile-time. This helps grab errors early in the development process.
- Just-in-Time (JIT) and Ahead-of-Time (AOT) Compilation: Dart favors both JIT and AOT compilation, enabling efficient development and optimized performance for production.
- Garbage Collection: Dart includes automatic memory management through garbage collection, which simplifies memory handling for developers.
- Asynchronous Programming: Dart has built-in support for asynchronous programming, making it well-suited for handling asynchronous tasks, such as network requests and user input.
- Dart Virtual Machine (VM): Dart has its own virtual machine that executes Dart code efficiently, allowing for standalone Dart applications.
What is top use cases of Dart?
Top use cases of Dart include:
- Web Development:
- Dart can be used for building web applications, and it is often employed with the Flutter framework to create interactive and responsive web experiences.
- Mobile App Development:
- Dart is commonly associated with the Flutter framework for building cross-platform mobile applications. Flutter uses Dart to create natively compiled applications for iOS and Android.
- Server-Side Development:
- Dart can be used for server-side development to build scalable and efficient server applications.
- Command-Line Tools:
- Dart is suitable for developing command-line tools and scripts.
- Internet of Things (IoT):
- Dart can be used for developing applications for IoT devices, providing a consistent language for both frontend and backend development in an IoT system.
- Game Development:
- While not as prevalent as some other languages in the game development space, Dart can be used for building games, particularly with the use of game development frameworks that support Dart.
- Desktop Applications:
- Dart can be used for building desktop applications, although it may not be as commonly chosen as some other languages for this purpose.
- Educational Purposes:
- Dart is often used in educational settings to teach programming concepts, especially when students are learning about web and mobile development.
The primary and most well-known use case for Dart is within the context of Flutter, where it serves as the programming language for building high-quality, natively compiled mobile applications. Flutter’s hot-reload feature, expressive UI, and cross-platform capabilities have contributed to the popularity of Dart in the mobile app development space.
What are feature of Dart?
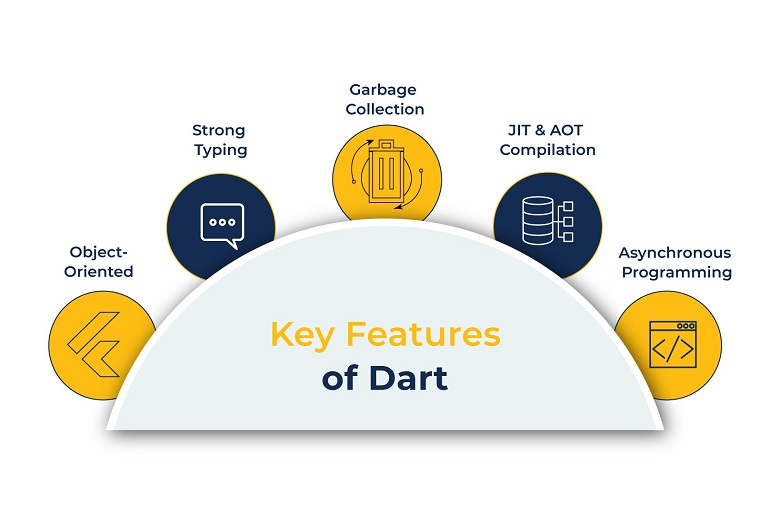
Dart, as a programming language, comes with several features that make it suitable for building web, mobile, and server applications. Here are some key features of Dart:
- Object-Oriented: Dart is an object-oriented language, supporting concepts like classes, objects, inheritance, and encapsulation.
- Strongly Typed: Dart is statically typed, which means that variable types are known at compile-time, providing better code analysis and catching errors early in the development process.
- Just-in-Time (JIT) and Ahead-of-Time (AOT) Compilation: Dart supports both JIT and AOT compilation. JIT compilation is used during development for faster iteration with features like hot-reload, while AOT compilation is used for producing optimized and standalone executables for production.
- Garbage Collection: Dart incorporates automatic memory management through garbage collection, simplifying memory handling for developers.
- Asynchronous Programming: Dart has built-in support for asynchronous programming using the
async
andawait
keywords, making it well-suited for handling asynchronous operations like network requests. - Dart SDK and Dart DevTools: Dart comes with a software development kit (SDK) that includes libraries, a runtime, and tools for Dart development. Dart DevTools provide a suite of debugging and profiling tools to enhance the development workflow.
- Dart Web: Dart supports web development through the Dart Web platform, allowing developers to build client-side web applications with Dart.
- Flutter Framework Integration: Dart is the primary language used with the Flutter framework for building cross-platform mobile applications. Flutter’s hot-reload feature allows developers to see changes instantly during development.
What is the workflow of Dart?
Now, let’s outline a general workflow for Dart development, especially in the context of building a Flutter mobile application:
- Setup Development Environment:
- Install Dart SDK and set up the development environment. For Flutter development, also install the Flutter SDK.
- Create a Dart Project:
- Create a new Dart project using the Dart command-line tools or by using an IDE like Visual Studio Code or IntelliJ IDEA.
- Write Dart Code:
- Write Dart code to implement the application’s logic. This includes creating classes, functions, and handling various aspects of the application’s behavior.
- Run and Test:
- Use the Dart development tools or the Flutter CLI to run and test the application. Flutter’s hot-reload feature allows you to see changes instantly without restarting the application.
- Debugging:
- Use Dart DevTools or the built-in debugging features of your IDE to identify and fix issues in your code.
- Dependency Management:
- Use the Dart package manager, called Pub, to manage dependencies in your project. Specify dependencies in the
pubspec.yaml
file.
- Use the Dart package manager, called Pub, to manage dependencies in your project. Specify dependencies in the
- Unit Testing:
- Write unit tests for your Dart code using the built-in testing framework. Run tests to ensure the accuracy of your code.
- Integration with Flutter Widgets:
- If building a Flutter application, integrate Dart code with Flutter widgets to create the user interface (UI) and manage the app’s state.
- Build for Production:
- Use the Flutter CLI or Dart tools to build the application for production. This involves AOT compilation to create optimized and standalone executables.
- Deployment:
- Deploy the application to the desired platform, such as app stores for mobile applications or web hosting for web applications.
- Continuous Integration and Delivery (CI/CD):
- Set up CI/CD pipelines to automate the testing, building, and deployment processes.
This workflow provides a general outline for Dart development, but specific details may vary depending on the type of application being developed (web, mobile, or server) and the tools and frameworks used in the project.
How Dart Works & Architecture?
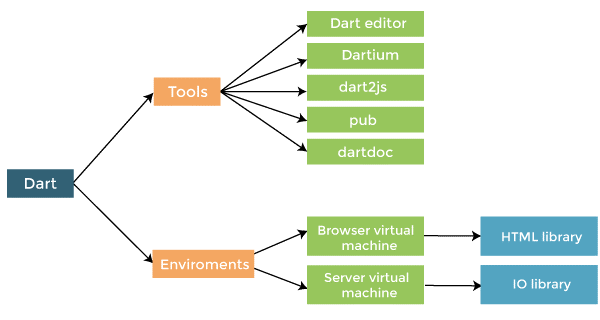
Dart is a modern, object-oriented, and garbage-collected programming language designed for building web, mobile, desktop, and command-line applications. Understanding Dart’s underlying structure can help you leverage its power and write efficient code. Here’s a breakdown of how Dart works and its key architectural components:
Core Features:
- Single-threaded: Dart executes on a single thread, but utilizes asynchronous programming paradigms for efficient resource management.
- Just-in-time (JIT) compilation: Dart code is compiled to machine code on the fly during runtime, offering fast performance.
- Ahead-of-time (AOT) compilation: Optional AOT compilation generates native code for enhanced performance and smaller build sizes.
- Strong typing: Statically typed variables ensure type safety and reduce runtime errors.
- Garbage collection: Automatic memory management simplifies resource allocation and prevents memory leaks.
- Clean and expressive syntax: Dart boasts a familiar C-style syntax with modern features for conciseness and readability.
Architecture:
- Source code: The developer writes Dart code using a text editor or IDE.
- Lexer and Parser: Analyze the code, convert it into tokens, and build a parse tree to represent the source code structure.
- Type Analyzer: Analyzes the parse tree and infers types for variables and expressions, ensuring type safety.
- Resolver: Resolves references to functions, classes, and other identifiers.
- Constant Evaluator: Evaluates constant expressions at compile time for static optimizations.
- Backend Compiler:
- JIT Compilation: Creates machine code on the fly during runtime execution.
- AOT Compilation (optional): Generates native code for faster performance and smaller size (e.g., for mobile apps).
- Runtime: Executes the generated machine code, managing memory, garbage collection, and interaction with the underlying platform.
Benefits of Dart’s Architecture:
- Efficiency: JIT compilation offers good performance with fast startup times.
- Portability: The generated machine code runs on various platforms with minimal modifications.
- Small size: AOT compilation minimizes the application size for deployment.
- Safety: Strong typing and garbage collection reduce errors and simplify memory management.
- Developer experience: Clean syntax and advanced features enhance code readability and maintainability.
How to Install and Configure Dart?
Installing and configuring Dart is a breeze! Here’s how you can do it based on your chosen platform:
1. Windows:
- Download: Get the Dart SDK for Windows from the official website.
- Install: Run the downloaded installer and use the on-screen instructions.
- Verify: Open a terminal and type
dart --version
. If you see the installed version, you’re good to go! - Optional: Set environment variables for convenience:
PATH
: AddC:\dart\bin
to your PATH for accessing Dart tools from any directory.FLUTTER_HOME
: If using Flutter, set this to the Flutter SDK directory.
2. macOS:
- Download: Get the Dart SDK for macOS from the official website.
- Install: Double-click the downloaded DMG file and follow the on-screen instructions.
- Verify: Open a terminal and type
dart --version
. If you see the installed version, you’re good to go! - Optional: Set environment variables for convenience:
PATH
: Add/usr/local/bin
to your PATH for accessing Dart tools from any directory.FLUTTER_HOME
: If using Flutter, set this to the Flutter SDK directory.
3. Linux:
- Download: Get the Dart SDK for Linux from the official website.
- Extract: Extract the downloaded archive to a desired directory (e.g.,
/opt/dart
). - Set permissions: Make the extracted files executable:
sudo chmod +x dart-sdk/bin/*
- Verify: Open a terminal and type
dart --version
. If you see the installed version, you’re good to go! - Optional: Set environment variables for convenience:
PATH
: Add the Dart SDK bin directory (e.g.,/opt/dart/bin
) to your PATH.FLUTTER_HOME
: If using Flutter, set this to the Flutter SDK directory.
Fundamental Tutorials of Dart: Getting started Step by Step
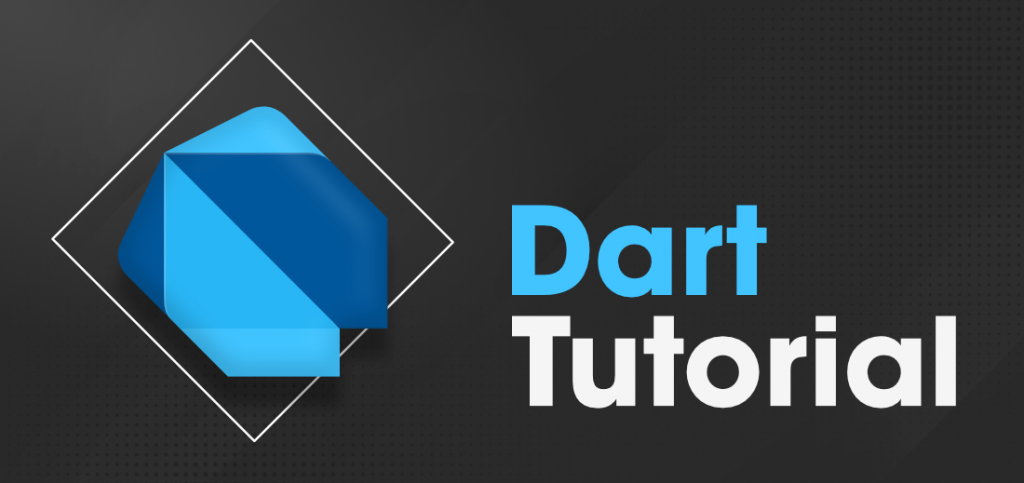
To create the perfect step-by-step fundamental tutorials for Dart, I need a bit more information about your needs and preferences:
1. Your experience: Are you a complete beginner to programming, or do you have some experience with other languages?
2. Your learning style: Do you prefer written tutorials, video lessons, interactive exercises, or a combination?
3. Your goals: What do you hope to achieve with Dart? This could be anything from building basic web applications to exploring mobile development with Flutter. 4. Tools and environment: Do you have a preferred text editor or IDE (integrated development environment)?
Once I have this information, I can tailor the tutorials to your specific needs and ensure they are both engaging and effective.
Here’s a general outline of what you can expect to learn in fundamental Dart tutorials:
Step 1: Setting Up:
- Download and install the Dart SDK according to your operating system.
- Set up your development environment, including your preferred editor or IDE.
- Learn basic syntax elements like variables, data types, and operators.
Step 2: Control Flow:
- Work with conditional statements like
if
andswitch
to handle different scenarios. - Use loops like
for
andwhile
to iterate over data structures. - Break and continue statements for controlling loop execution.
Step 3: Functions:
- Define your own functions for code reuse and modularity.
- Understand function parameters, return values, and local variables.
- Learn about lambda expressions for concise and expressive code.
Step 4: Classes and Objects:
- Define classes to represent real-world entities with attributes and methods.
- Understand object creation and member access syntax.
- Learn about constructors and inheritance for building complex object hierarchies.
Step 5: Collections:
- Work with data structures like lists, maps, and sets to store and organize data.
- Understand collection operations like adding, removing, and iterating over elements.
- Learn about accessing specific elements and filtering data based on conditions.
Step 6: Asynchronous Programming:
- Implement asynchronous operations like fetching data from the web using
Future
s andasync
await`. - Understand how to handle asynchronous results and error handling.
Step 7: Introduction to Flutter (Optional):
- If your goal is mobile development, explore the basics of Flutter, the popular framework built on Dart.
- Learn about Flutter widgets and how to build simple user interfaces.
Practice is key! Don’t hesitate to experiment with the concepts you learn and explore additional resources to solidify your understanding.
- PPG Industries: Selection and Interview process, Questions/Answers - April 3, 2024
- Fiserv: Selection and Interview process, Questions/Answers - April 3, 2024
- Estee Lauder: Selection and Interview process, Questions/Answers - April 3, 2024