What are Deep Learning Frameworks?
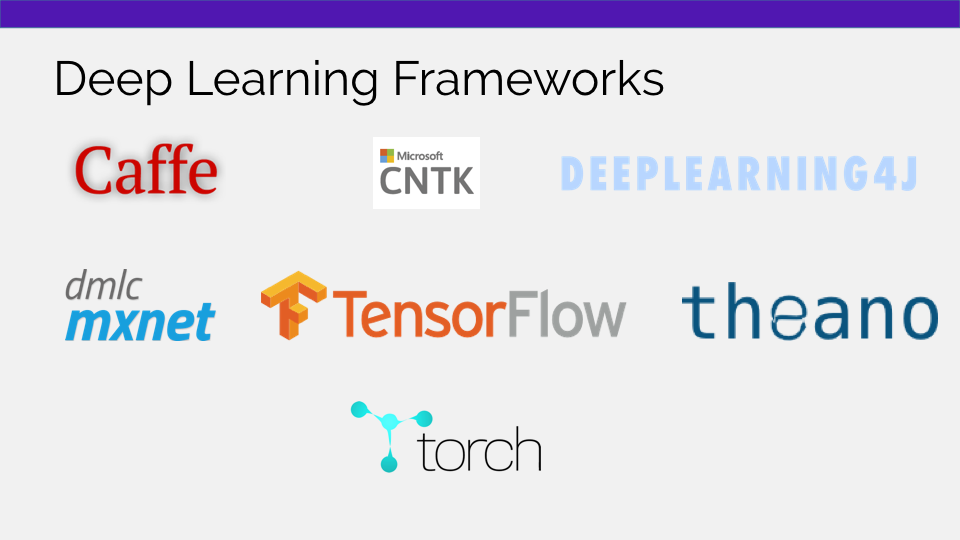
Deep Learning Frameworks are specialized software libraries or platforms that provide tools and abstractions to build, train, and deploy deep neural networks efficiently. Deep learning frameworks are essential for working with complex neural network architectures, such as convolutional neural networks (CNNs), recurrent neural networks (RNNs), and transformers, which have shown exceptional performance in various machine learning tasks. These frameworks offer a range of functionalities, including automatic differentiation, optimization algorithms, GPU acceleration, and support for various neural network layers.
Top 10 use cases of Deep Learning Frameworks:
- Image Classification: Deep learning frameworks are widely used for image classification tasks, such as recognizing objects in images.
- Object Detection: They power object detection algorithms to locate and classify multiple objects within an image.
- Natural Language Processing (NLP): Deep learning frameworks are extensively used for language modeling, sentiment analysis, machine translation, and other NLP tasks.
- Speech Recognition: They enable speech-to-text applications and voice assistants.
- Text Generation: Deep learning frameworks are used to generate text, including language models and chatbots.
- Recommendation Systems: They are employed for building personalized recommendation systems used in various industries.
- Anomaly Detection: Deep learning frameworks help in identifying anomalies in data, useful in fraud detection and fault monitoring.
- Image Segmentation: They enable segmenting an image into multiple regions and identifying different objects.
- Handwriting Recognition: Deep learning frameworks are used for recognizing handwritten text or characters.
- Autonomous Vehicles: They play a significant role in self-driving cars and autonomous vehicle research.
What are the feature of Deep Learning Frameworks?
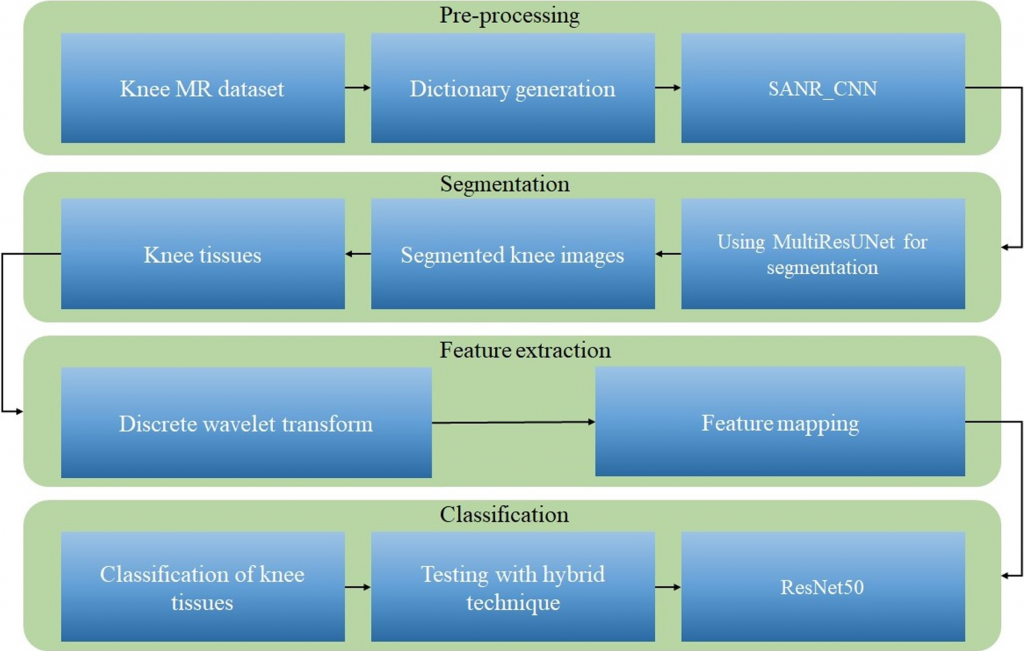
- Neural Network Layers: They provide a wide range of pre-implemented layers like convolutional layers, dense layers, recurrent layers, and more.
- Optimization Algorithms: Deep learning frameworks offer various optimization algorithms for training neural networks, such as stochastic gradient descent (SGD), Adam, RMSprop, etc.
- Automatic Differentiation: They support automatic differentiation, enabling gradient-based optimization of neural network parameters.
- GPU Acceleration: Many deep learning frameworks utilize GPUs to accelerate the training process of large neural networks.
- Model Deployment: They facilitate exporting and deploying trained deep learning models for real-world applications.
- Pre-trained Models: Deep learning frameworks often provide pre-trained models, allowing users to fine-tune them for specific tasks.
- Visualization Tools: They offer visualization tools for understanding model architectures, training progress, and performance metrics.
How Deep Learning Frameworks Work and Architecture?
The architecture of deep learning frameworks varies, but the general workflow involves:
- Data Preparation: Input data is preprocessed and transformed into suitable formats for training and evaluation.
- Model Design: Users design the neural network architecture, specifying the layers, activation functions, and connections.
- Model Compilation: The model is compiled, where loss functions, optimization algorithms, and metrics are defined.
- Model Training: The neural network is trained on the training data using gradient-based optimization.
- Model Evaluation: The trained model is evaluated on validation data to assess its performance.
- Model Deployment: The model is deployed in production environments for making predictions on new data.
How to Install Deep Learning Frameworks?
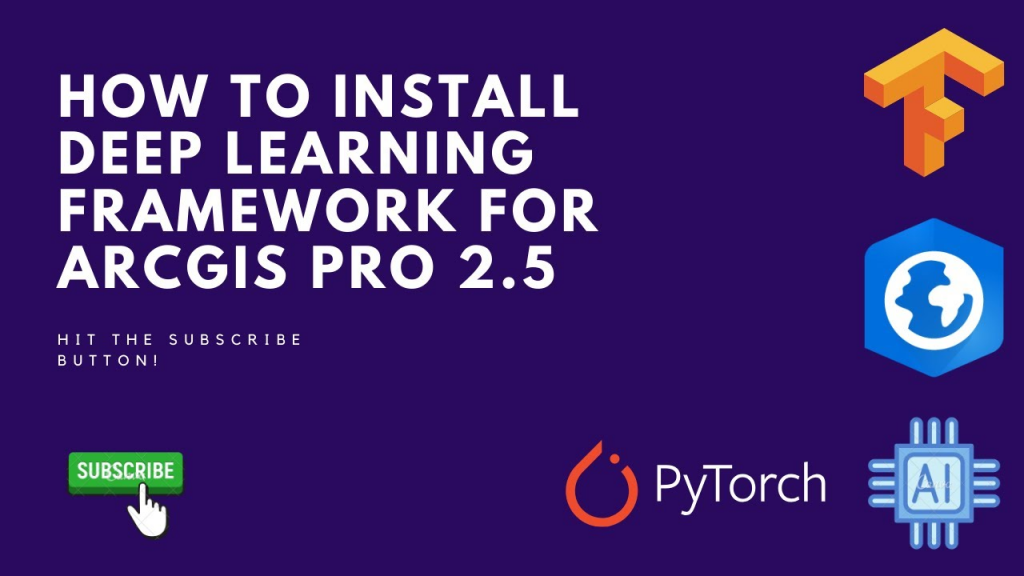
Installing a deep learning framework depends on the specific framework you want to use. Some popular deep learning frameworks include TensorFlow, PyTorch, Keras, MXNet, and Caffe. Most of these frameworks can be installed using package managers like pip or conda.
For example, to install TensorFlow using pip, you can use the following command:
pip install tensorflow
For PyTorch, you can use:
pip install torch torchvision
For Keras, you can use:
pip install keras
For MXNet, you can use:
pip install mxnet
For Caffe, you can follow the installation instructions provided in the official documentation.
Before installing a deep learning framework, ensure you have the required dependencies and hardware resources, such as GPU drivers (if using GPU acceleration) and libraries for numerical computations.
Please refer to the official documentation and websites of the specific deep learning framework you wish to install for detailed and up-to-date installation instructions.
Basic Tutorials of Deep Learning Frameworks: Getting Started
Sure! Below are basic tutorials for getting started with deep learning frameworks step-by-step:
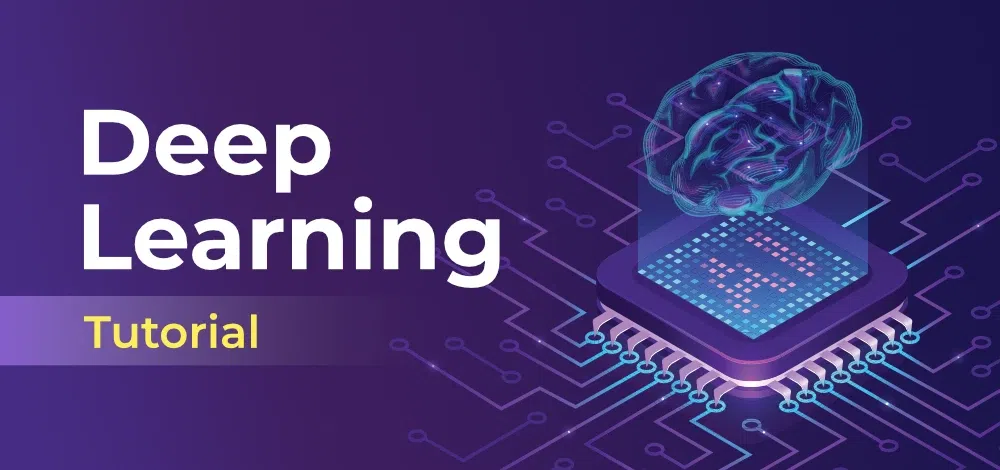
Deep learning frameworks are interfaces, libraries, or tools that simplify the process of implementing machine learning and AI. These frameworks provide various functionalities that allow developers to easily upload data, train models, and perform accurate predictive analysis[^0^].
This guide will introduce you to several popular deep learning frameworks and provide a basic tutorial for each of them.
- Deeplearning4j (DL4J): Developed by a machine learning group, DL4J is a deep learning framework written in Java, Scala, C++, C, and CUDA. It supports different types of neural networks, such as Convolutional Neural Network (CNN), Recurrent Neural Network (RNN), and Long Short-Term Memory (LSTM)[^0^].
// Initialize a MultiLayerConfiguration
MultiLayerConfiguration conf = new NeuralNetConfiguration.Builder()
.list()
.layer(0, new DenseLayer.Builder().nIn(numRows * numColumns).nOut(1000).build())
.layer(1, new OutputLayer.Builder(LossFunctions.LossFunction.NEGATIVELOGLIKELIHOOD)
.nIn(1000)
.nOut(outputNum)
.activation(Activation.SOFTMAX)
.build())
.build();
// Initialize a MultiLayerNetwork
MultiLayerNetwork model = new MultiLayerNetwork(conf);
model.init();
- Keras: Keras is a high-level neural network API, written in Python and capable of running on top of TensorFlow. It is known for its speed and ease of use, especially for high-level computations[^1^].
from keras.models import Sequential
from keras.layers import Dense
# Initialize a Sequential model
model = Sequential()
# Add an input layer
model.add(Dense(12, input_dim=8, activation='relu'))
# Add one hidden layer
model.add(Dense(8, activation='relu'))
# Add an output layer
model.add(Dense(1, activation='sigmoid'))
- MXNet: MXNet is a highly scalable deep learning framework that supports multiple programming languages. It provides support for state-of-the-art deep learning models such as CNNs and LSTMs[^1^].
import mxnet as mx
# Define the network
data = mx.sym.Variable('data')
fc1 = mx.sym.FullyConnected(data, name='fc1', num_hidden=128)
act1 = mx.sym.Activation(fc1, name='relu1', act_type="relu")
fc2 = mx.sym.FullyConnected(act1, name='fc2', num_hidden = 64)
softmax = mx.sym.SoftmaxOutput(fc2, name = 'softmax')
- Gluon: Gluon is an open-source deep learning interface that simplifies the creation of machine learning models. It provides a straightforward and concise API for defining machine learning and deep learning models[^1^].
from mxnet import gluon
# Define a network
net = gluon.nn.Sequential()
with net.name_scope():
net.add(gluon.nn.Dense(128, activation='relu'))
net.add(gluon.nn.Dense(64, activation='relu'))
net.add(gluon.nn.Dense(10))
- Chainer: Chainer is an open-source deep learning framework that introduces the define-by-run approach. It offers ease of debugging and flexibility in describing control flows[^1^].
import chainer.links as L
import chainer.functions as F
from chainer import Chain
# Define a network
class Net(Chain):
def __init__(self):
super(Net, self).__init__()
with self.init_scope():
self.l1 = L.Linear(None, 100)
self.l2 = L.Linear(100, 10)
def forward(self, x):
h = F.relu(self.l1(x))
return self.l2(h)
model = Net()
- PaddlePaddle: PaddlePaddle provides an intuitive and flexible interface for loading data and specifying model structures. It supports CNN, RNN, and multiple variants[^3^].
import paddle.v2 as paddle
# Initialize PaddlePaddle
paddle.init(use_gpu=False, trainer_count=1)
# Configure the neural network
images = paddle.layer.data(name='pixel', type=paddle.data_type.dense_vector(784))
label = paddle.layer.data(name='label', type=paddle.data_type.integer_value(10))
hidden1 = paddle.layer.fc(input=images, size=200, act=paddle.activation.Tanh())
hidden2 = paddle.layer.fc(input=hidden1, size=200, act=paddle.activation.Tanh())
prediction = paddle.layer.fc(input=hidden2, size=10, act=paddle.activation.Softmax())
These are just the basics to get you started with these deep learning frameworks. Each framework has its own advantages and is suitable for different kinds of tasks.
- Degree Pursuit: Navigating the Path to Educational Excellence - July 4, 2024
- Why Is Studying English Important in a Business Environment? - July 4, 2024
- Top 10 Data Science Skills You Need in 2024 - July 3, 2024