What is Express?
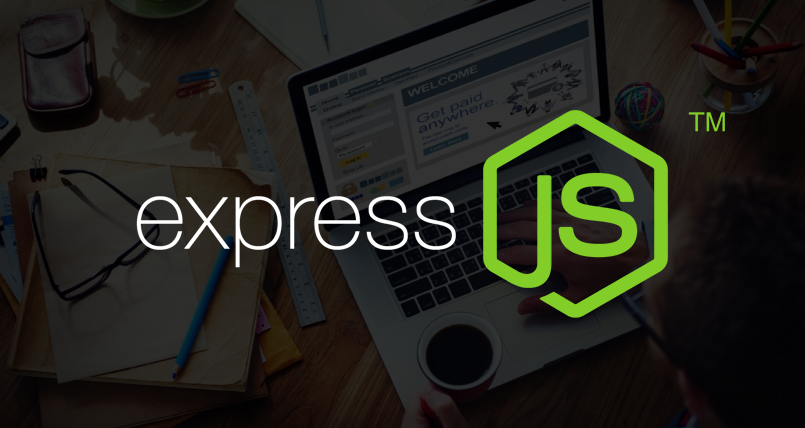
Express, also known as Express.js or simply Express, is a minimal and flexible web application framework for Node.js. It is one of the most popular frameworks for building web and mobile applications using JavaScript on the server side. Express provides a robust set of features for web and mobile application development, making it efficient and scalable for building APIs and web services.
What is top use cases of Express?
Top Use Cases of Express:
- Web Application Development:
- Express is commonly used for building web applications, whether they are single-page applications (SPAs) or traditional multi-page applications. Its simplicity and flexibility make it suitable for a wide range of web development projects.
- API Development:
- Express is often chosen for building RESTful APIs (Application Programming Interfaces). It provides a clean and straightforward way to define routes, handle HTTP methods, and manage data, making it an excellent choice for creating API endpoints.
- Microservices Architecture:
- In a microservices architecture, where applications are broken down into small, independent services, Express can be used to build lightweight and scalable microservices. Its modular design and support for route handling make it suitable for this architectural style.
- Real-Time Applications:
- Express, when combined with technologies like Socket.io, is well-suited for building real-time applications such as chat applications, collaborative tools, or online gaming platforms. It enables bidirectional communication between the server and clients.
- Content Management Systems (CMS):
- Express can be used to build custom content management systems where developers have the flexibility to design and structure content based on specific requirements.
- E-commerce Platforms:
- For developing e-commerce platforms or online shopping websites, Express can be employed to handle product listings, user authentication, and transaction processing. It integrates well with databases and other components required for e-commerce functionality.
- Authentication and Authorization Systems:
- Express is often used to implement authentication and authorization mechanisms in web applications. Middleware and libraries, such as Passport.js, can be easily integrated to handle user authentication.
- Single-Page Applications (SPAs):
- Express is suitable for building backends for single-page applications, where the server provides RESTful APIs and serves as the backend logic for the application.
- Blog Platforms:
- Developers can use Express to create custom blog platforms, handling features such as content creation, user comments, and content retrieval.
- Middleware for Frameworks:
- Express can serve as middleware for other web frameworks, enhancing their functionality. For example, it can be used with frameworks like Meteor or NestJS to handle specific aspects of request processing.
- Prototyping and MVPs:
- Express is a great choice for quickly prototyping ideas and building Minimum Viable Products (MVPs). Its simplicity allows developers to rapidly iterate and test concepts.
- Server-Side Rendering (SSR):
- For applications requiring server-side rendering, Express can be used to render views on the server before sending them to the client. This is particularly useful for optimizing page load times and improving SEO.
- Data Dashboards:
- Express can be used to build data dashboards and visualization tools. Its ability to handle data from various sources and serve dynamic content makes it suitable for creating interactive dashboards.
- Educational Platforms:
- Express can be employed in the development of educational platforms and e-learning systems where users interact with content, quizzes, and other educational materials.
- Job Boards and Recruitment Platforms:
- Job boards and recruitment platforms can use Express to handle job listings, user authentication, and communication between employers and job seekers.
Express’s lightweight nature and extensive middleware support contribute to its popularity for various use cases in web and mobile application development. Its flexibility and scalability make it adaptable to a wide range of project requirements.
What are feature of Express?
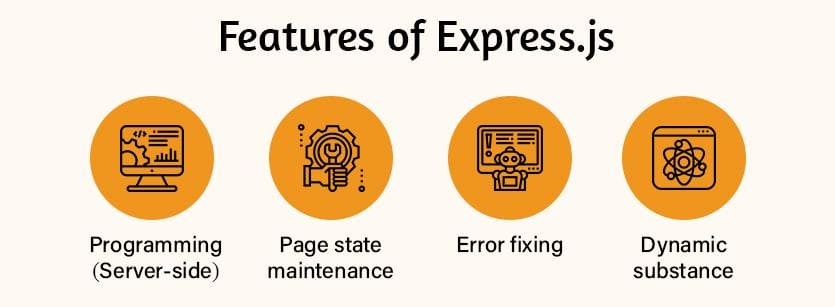
Express is a web application framework for Node.js that simplifies the method of building robust and scalable web applications. Here are some key features of Express:
- Routing:
- Express provides a simple and effective routing system that allows developers to define routes for handling different HTTP methods and URL patterns. This makes it easy to structure an application and handle various types of requests.
- Middleware:
- Middleware functions in Express are crucial for handling tasks between the incoming request and the server’s response. They can perform tasks such as logging, authentication, and error handling. Express middleware functions have access to the request and response objects, allowing for flexible request processing.
- HTTP Utility Methods:
- Express simplifies the handling of HTTP methods with utility methods such as
get
,post
,put
, anddelete
. These methods correspond to the standard HTTP methods and make route handling more readable.
- Express simplifies the handling of HTTP methods with utility methods such as
- Templating Engines:
- While Express itself is unopinionated about the view engine, it seamlessly integrates with various templating engines like EJS, Pug, and Handlebars. This allows developers to render dynamic views on the server and send them to the client.
- Static File Serving:
- Express can serve static files, such as images, stylesheets, and client-side scripts, using the
express.static
middleware. This is useful for serving assets that do not require server-side processing.
- Express can serve static files, such as images, stylesheets, and client-side scripts, using the
- Error Handling:
- Express simplifies error handling with middleware functions dedicated to handling errors. Developers can define error-handling middleware to manage errors that occur during the request-response cycle.
- RESTful API Support:
- Express is commonly used for building RESTful APIs. Its simplicity and flexibility make it easy to define routes, handle HTTP methods, and manage data, making it suitable for creating API endpoints.
- Request and Response Objects:
- Express provides a request object (
req
) and a response object (res
) that encapsulate information about the incoming request and facilitate the generation of the response. These objects simplify interactions with the request and response lifecycle.
- Express provides a request object (
- Session and Cookie Handling:
- Express supports session management and cookie parsing. Middleware such as
express-session
can be used for handling user sessions, authentication, and managing stateful information.
- Express supports session management and cookie parsing. Middleware such as
- Security Middleware:
- Express can integrate with security-focused middleware to enhance application security. Middleware like Helmet helps secure an Express application by setting various HTTP headers.
- Database Integration:
- Express can be easily integrated with databases through various database drivers or Object-Relational Mapping (ORM) libraries, allowing developers to interact with databases seamlessly.
- WebSocket Support:
- While not a core feature, Express can work with WebSocket libraries like Socket.io to enable real-time bidirectional communication between the server and clients.
What is the workflow of Express?
The workflow for developing applications with Express involves several key steps:
- Installation:
- Install Node.js and then use npm (Node Package Manager) to install Express.
npm install express
- Project Setup:
- Create a new directory for your project and set up necessary files, such as
package.json
andapp.js
(or another entry point).
3. Application Configuration:
- Configure your Express application by setting up middleware, defining routes, and configuring any necessary settings. This includes adding middleware for parsing JSON, handling static files, etc.
4. Define Routes:
- Define routes for your application. Routes handle specific HTTP methods and URL patterns, specifying the logic to execute when a particular route is accessed.
5. Middleware:
- Use middleware functions to perform tasks such as authentication, logging, and error handling. Middleware functions are implemented in the order they are defined.
6. Views and Templates (If Applicable):
- If your application involves rendering views, set up a templating engine (EJS, Pug, etc.) and define views for different routes.
7. Database Integration:
- Integrate your Express application with a database by using appropriate drivers or ORM libraries. Set up database connections and define models if needed.
8. Testing:
- Implement testing for your application using testing frameworks like Mocha, Chai, or Jest. Write tests for routes, middleware, and any custom functions.
9. Development and Debugging:
- Develop and debug your application. Use tools like
nodemon
for automatic server restarts during development.
10. Security Considerations:
- Implement security best practices, such as using secure middleware (Helmet), validating user input, and protecting against common web vulnerabilities.
11. Deployment:
- Deploy your Express application to a hosting service or server. Common deployment platforms include Heroku, AWS, and DigitalOcean. Configure environment variables for sensitive information.
12. Monitoring and Logging:
- Set up monitoring and logging to track the application’s performance, detect issues, and gather insights into user behavior.
13. Documentation:
- Document your code, especially API endpoints and any significant functionalities. This documentation is useful for both developers working on the project and external users.
14. Continuous Integration (CI) and Continuous Deployment (CD):
- Implement CI/CD pipelines to automate testing, build processes, and deployment. Tools like Jenkins, Travis CI, or GitHub Actions can be applied for this purpose.
15. Maintenance and Updates:
- Regularly update dependencies, apply security patches, and address any issues that arise. Consider adding new features or improving existing ones based on user feedback.
This workflow is a general guideline, and the specific steps may vary based on the nature of your project and development preferences. The modular and flexible nature of Express allows developers to adapt the workflow to meet the needs of their specific applications.
How Express Works & Architecture?
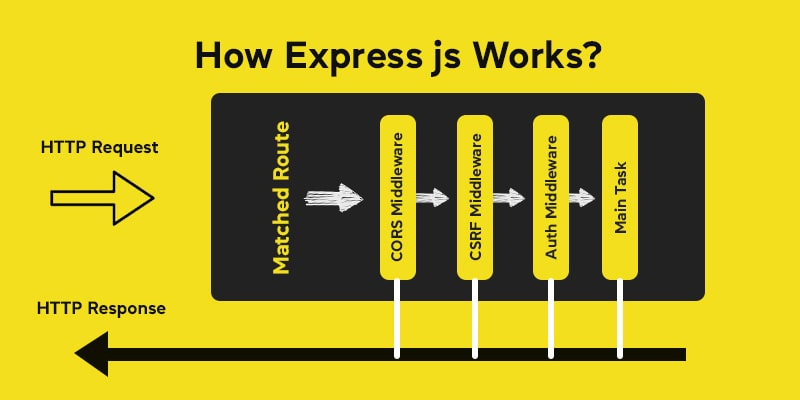
Since you haven’t specified which “Express” you’re interested in, I’ll offer brief explanations for both possibilities from your previous questions:
1. AWS Step Functions Express Workflows:
How it works:
- Event-driven: Express Workflows react to events (like SNS notifications or SQS messages) and trigger workflow executions.
- State machines: Workflows define sequences of states representing possible execution steps.
- Distributed execution: States can be executed on different Lambda functions or Fargate tasks for scalability and parallelism.
- Asynchronous processing: Each state execution is independent, allowing other steps to proceed without blocking.
- Error handling and retries: Built-in mechanisms handle errors and automatically retry executions if necessary.
Architecture:
- Central orchestrator: The Step Functions service manages workflow definition and execution.
- Execution workers: Lambda functions or Fargate tasks execute individual states in parallel.
- Event sources: Various AWS services or external applications can trigger workflow executions.
- Data storage: Workflows can access data from DynamoDB, S3, or other AWS databases.
2. Express.js (web framework):
How it works:
- Request-response cycle: Handles incoming HTTP requests, processes them through middleware and route handlers, and returns responses.
- Middleware: Chains of functions pre-process requests and responses, adding functionalities like authentication or logging.
- Routing: Matches incoming requests to specific route handlers based on URL patterns and HTTP methods.
- Templating: Generates dynamic HTML content using template engines like Pug or EJS.
- Data handling: Connects to databases and data sources using modules like MongoDB or Sequelize.
Architecture:
- Modular structure: Applications are built with separate modules for routes, middleware, and data access.
- Express core: Provides the underlying framework for managing requests, responses, and middleware.
- Third-party modules: Developers can extend Express with modules for various functionalities like templating, databases, and authentication.
Remember, these are simplified explanations. Each “Express” has its own intricacies and functionalities. If you’d like a deeper dive into a specific aspect of either Express, please provide more details about your area of interest. I’m happy to help you understand them better!
How to Install and Configure Express?
Now that you’ve clarified you’re interested in installing and configuring Express.js (the Node.js web framework), I can guide you through the process! Here are the steps:
1. Install Node.js:
- Download the appropriate Node.js installer for your operating system from the official website.
- Run the installer and use the on-screen instructions.
- Verify Node.js installation by opening a terminal and typing
node -v
. You should see the installed Node.js version.
2. Install Express.js:
- Open your terminal and navigate to your project directory (where you want your Express application to reside).
- Run the following command to install Express.js globally:
npm install express -g
This installs Express.js globally, accessible from any project directory. Alternatively, you can install Express.js locally within your project directory:
npm install express
3. Create a basic Express application:
- Create a new file named
app.js
in your project directory. - Paste the following code into
app.js
:
JavaScript
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello, World!');
});
app.listen(3000, () => {
console.log('Server is listening on port 3000');
});
This code creates a basic Express application that listens for requests on port 3000. When you access http://localhost:3000
in your browser, you should see “Hello, World!” displayed.
4. Configure and extend your application:
- Express.js offers numerous features and functionalities you can explore to build more complex applications.
- You can define additional routes, middleware functions, and use various modules for tasks like database access, templating, and authentication.
Important Tips:
- Consider using a text editor or IDE with Node.js debugging capabilities for a smoother development experience.
- Explore beginner-friendly tutorials and resources online to learn more about Express.js and web development.
- Don’t hesitate to ask if you encounter any problems during the installation or configuration process. I’m here to help!
Remember, this is just a basic overview. As you progress with Express.js, you can delve deeper into its features and build robust and scalable web applications.
Fundamental Tutorials of Express: Getting started Step by Step
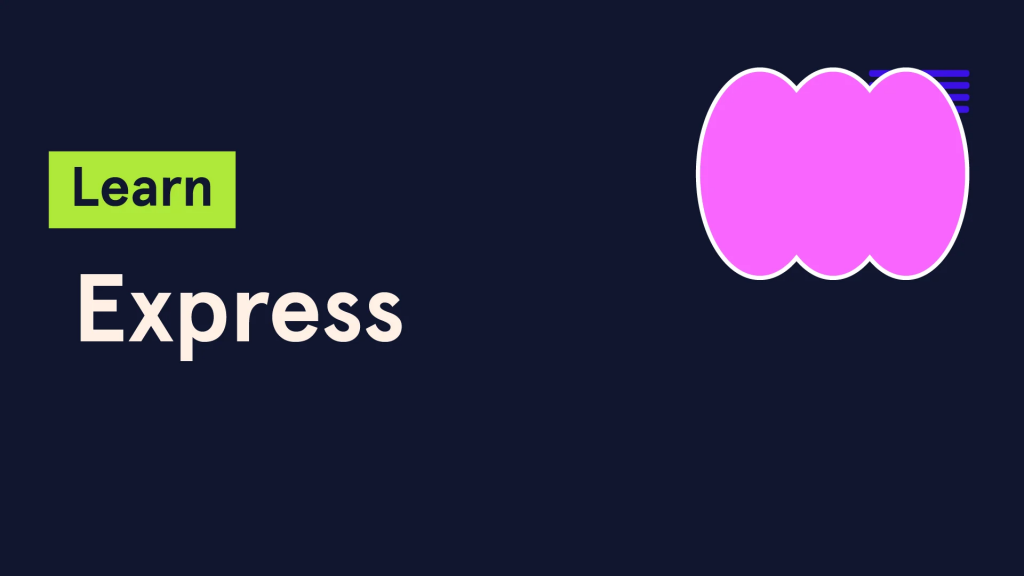
I’m excited to guide you through your first steps with Express.js! Here’s a breakdown of fundamental tutorials to get you started:
1. Setting Up:
- Install Node.js: Download and install the appropriate version for your operating system from the official website.
- Open Terminal: Navigate to your terminal (Command Prompt on Windows).
- Verify Node.js: Type
node -v
and press Enter. You should see the installed version. - Install Express.js: You can install Express globally for any project or locally within your project directory.
- Global installation:
npm install express -g
- Local installation:
npm install express
(inside your project folder)
- Global installation:
2. Hello World Application:
- Create a file: Create a file named
app.js
in your project directory. - Write the code: Paste the following code into
app.js
:
JavaScript
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello, World!');
});
app.listen(3000, () => {
console.log('Server is listening on port 3000');
});
- Run the application: Save the file and run
node app.js
in your terminal. - Open http://localhost:3000 in your browser. You should see “Hello, World!” displayed.
3. Adding Routes and Middleware:
- Routes: Define different routes to handle different URL paths. For example:
JavaScript
app.get('/about', (req, res) => {
res.send('About Page');
});
app.post('/contact', (req, res) => {
// Handle form submission data
});
- Middleware: Use middleware functions to pre-process requests and responses. For example:
JavaScript
app.use((req, res, next) => {
console.log('Request URL:', req.url);
next();
});
4. Connecting to Databases:
- Install a database driver (e.g.,
mongoose
for MongoDB) usingnpm install
. - Configure your database connection and access data within your Express application.
- Use database queries in route handlers to retrieve or modify data.
5. Templating:
- Use templating engines like Pug or EJS to generate dynamic HTML content based on data.
- Install the desired templating engine and configure your Express app.
- Create template files and use them within your route handlers to render dynamic content.
Bonus Tips:
- Explore online tutorials and resources for deeper understanding of Express functionalities.
- Experiment with different features like body parsing, session management, and error handling.
- Consider using frameworks like Express Generator to scaffold your application structure.
Always keep in mind, these are just the initial steps. As you progress, you can build more complex and interactive web applications with Express.js. Don’t hesitate to ask if you encounter any challenges or have questions about specific topics.
Enjoy your Express.js journey!
- Mutual of Omaha: Selection and Interview process, Questions/Answers - April 15, 2024
- AES: Selection and Interview process, Questions/Answers - April 15, 2024
- Amphenol: Selection and Interview process, Questions/Answers - April 15, 2024