What is Flask?
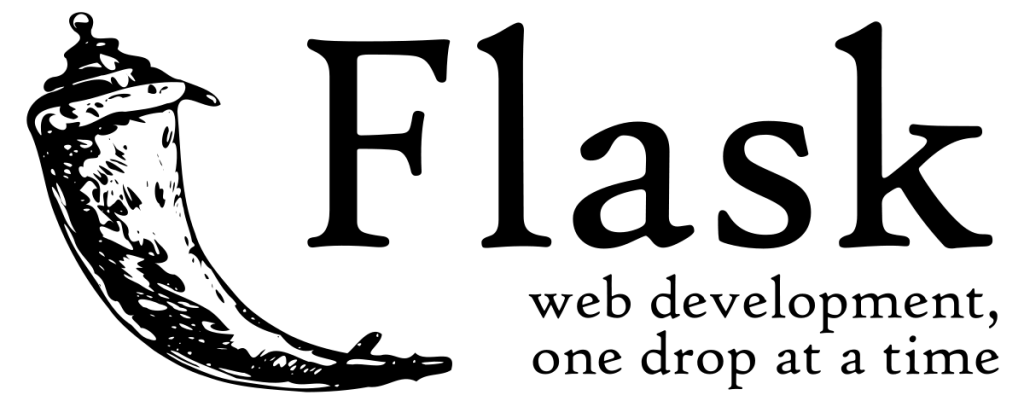
Flask is an extensible and lightweight web framework for Python. It is designed to be simple and easy to use, making it an excellent choice for building web applications and APIs. Flask follows the WSGI (Web Server Gateway Interface) standard and is known for its simplicity, flexibility, and a large and active community.
What is top use cases of Flask?
Top Use Cases of Flask:
- Web Applications:
- Flask is commonly used to build web applications ranging from small projects to larger, more complex applications. Its simplicity and minimalistic design make it well-suited for various web development tasks.
- RESTful APIs:
- Flask is widely used for developing RESTful APIs. Its modular design allows developers to create endpoints for serving data, interacting with databases, and integrating with other services.
- Prototyping and MVPs (Minimum Viable Products):
- Flask is an excellent choice for quickly prototyping web applications and building MVPs. Its simplicity and ease of use enable rapid development, allowing developers to test ideas and concepts.
- Microservices:
- Flask is suitable for building microservices due to its lightweight nature. Developers can create independent microservices that communicate with each other, providing a scalable and maintainable architecture.
- Data Visualization Dashboards:
- Flask, in combination with libraries like Plotly or Bokeh, is used to create interactive data visualization dashboards. This is beneficial for presenting data analytics and insights in a web-based format.
- Content Management Systems (CMS):
- Flask can be employed to build custom content management systems, allowing developers to create tailored solutions for managing and presenting content on websites.
- Educational Projects:
- Flask is often used in educational settings to teach web development and programming. Its simplicity makes it accessible for beginners, and it provides a solid foundation for understanding web development concepts.
- Blog and Content Websites:
- Flask can be used to develop simple blog platforms or content websites. Developers have the flexibility to structure and design the site according to their specific requirements.
- Online Forms and Surveys:
- Flask is suitable for creating web applications that involve forms and surveys. Its support for handling HTTP requests and form submissions makes it a good choice for collecting and processing user input.
- E-commerce Applications:
- While larger e-commerce platforms may opt for more feature-rich frameworks, Flask is used for smaller e-commerce applications or prototypes. It provides the flexibility to implement custom features as needed.
- Authentication and Authorization Systems:
- Flask can be used to build authentication and authorization systems for web applications. It supports various authentication methods and allows developers to implement custom authorization logic.
- Internet of Things (IoT) Projects:
- Flask is used in IoT projects to develop web interfaces for controlling and monitoring connected devices. Its lightweight nature is advantageous for resource-constrained environments.
- Task Scheduling and Automation:
- Flask, when combined with tools like Celery, is used for task scheduling and automation. Developers can build applications that perform background tasks, such as sending emails or processing data, at scheduled intervals.
- Real-time Applications:
- While not designed specifically for real-time applications, Flask can be used to build real-time features using technologies like WebSockets. It is suitable for scenarios where real-time updates are required.
- Integration with Other Technologies:
- Flask can be integrated with other technologies and frameworks, such as machine learning libraries (e.g., Flask with TensorFlow for serving models), making it versatile for a wide range of use cases.
Flask’s flexibility, simplicity, and ease of integration make it a versatile choice for a variety of web development projects, ranging from small-scale applications and prototypes to more complex and feature-rich solutions.
What are feature of Flask?
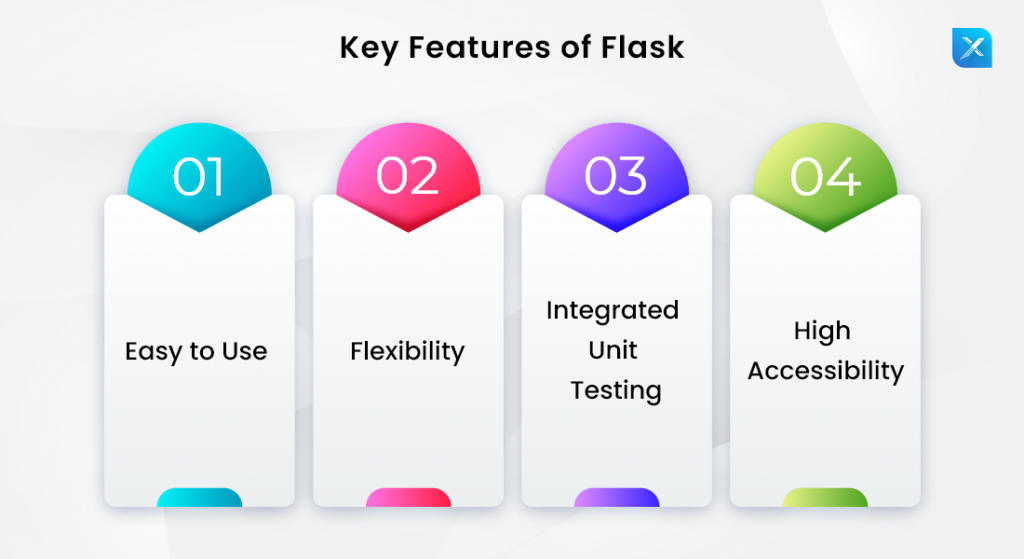
Features of Flask:
- Lightweight and Minimalistic:
- Flask is designed to be lightweight and follows a minimalistic philosophy. It provides the essential features for web development without imposing unnecessary complexity.
- Modular and Extensible:
- Flask follows a modular design, allowing developers to choose and integrate components based on their specific needs. Extensions and third-party libraries can be easily added to extend functionality.
- Built-in Development Server:
- Flask includes a built-in development server that is convenient for local development and testing. However, for production deployments, a more robust server (e.g., Gunicorn or uWSGI) is recommended.
- Routing:
- Flask supports URL routing, allowing developers to define routes for different parts of an application. Routes are associated with specific functions (view functions) that handle the corresponding HTTP requests.
- HTTP Request Handling:
- Flask simplifies handling of HTTP requests by providing decorators (e.g.,
@app.route
) to bind URL patterns to view functions. Developers can access request parameters, form data, and other request attributes easily.
- Flask simplifies handling of HTTP requests by providing decorators (e.g.,
- Template Engine (Jinja2):
- Flask uses the Jinja2 template engine, which allows developers to create dynamic HTML templates. Templates support template inheritance, macros, and conditional rendering, facilitating the generation of dynamic content.
- Werkzeug Integration:
- Flask is built on top of the Werkzeug WSGI utility library. Werkzeug provides features like request and response handling, URL routing, and middleware support, enhancing the capabilities of Flask.
- URL Building:
- Flask includes a URL building feature that generates URLs based on the specified route and parameters. This makes it easier to create links within the application.
- Middleware Support:
- Flask supports middleware, allowing developers to plug in additional functionality to handle tasks such as authentication, logging, or custom request processing.
- Error Handling:
- Flask provides mechanisms for handling errors gracefully. Developers can define error handlers for specific HTTP error codes or handle generic errors at the application level.
- Session Management:
- Flask supports session management for storing user-specific information across requests. Session variables can be easily accessed and manipulated in view functions.
- Cookie Support:
- Flask simplifies working with cookies by providing a convenient interface for setting, getting, and deleting cookies. This is useful for managing client-side data.
- RESTful Request Handling:
- Flask can be used to build RESTful APIs by leveraging its request handling features. Developers can create routes, handle different HTTP methods, and respond with JSON or other data formats.
- Testing Support:
- Flask includes support for testing, allowing developers to write unit tests and integration tests for their applications. The testing client provided by Flask facilitates simulating HTTP requests.
- ORM (Object-Relational Mapping) Integration:
- While Flask itself does not include a built-in ORM, it can be easily integrated with popular ORMs like SQLAlchemy. This enables developers to interact with databases and manage data models.
- Security Features:
- Flask incorporates security features to help developers mitigate common web application vulnerabilities. It includes mechanisms for handling cross-site scripting (XSS) and cross-site request forgery (CSRF) protection.
What is the workflow of Flask?
Workflow of Flask:
- Installation:
- Install Flask using a package manager like pip. Create a virtual environment to manage dependencies.
2. Project Structure:
- Organize the project structure by creating directories for templates, static files, and application modules. Define the main application file (e.g.,
app.py
).
3. Import Flask:
- Import the Flask class from the Flask package to create a Flask application instance.
from flask import Flask
app = Flask(__name__)
- Define Routes:
- Use the
@app.route
decorator to define routes and associate them with view functions. View functions handle specific HTTP requests and return responses.
@app.route('/')
def home():
return 'Hello, Flask!'
- Run the Development Server:
- Use the
app.run()
method to start the development server. This server is suitable for local development and testing.
if __name__ == '__main__':
app.run(debug=True)
- Templates and Rendering:
- Create HTML templates using the Jinja2 syntax. Use the
render_template
function to render templates within view functions.
from flask import render_template
@app.route('/greet/<name>')
def greet(name):
return render_template('greet.html', name=name)
- Accessing Request Data:
- Access data from HTTP requests using the
request
object. Parameters from URLs, form data, and other request attributes can be accessed.
from flask import request
@app.route('/login', methods=['POST'])
def login():
username = request.form['username']
password = request.form['password']
# Handle login logic
- Static Files:
- Serve static files (e.g., CSS, JavaScript) by placing them in the
static
directory. Flask automatically maps the/static/
route to this directory.
<!-- In HTML template -->
<link rel="stylesheet" href="{{ url_for('static', filename='styles.css') }}">
- Redirects and Responses:
- Apply the
redirect
andabort
functions for redirects and error responses, respectively.
from flask import redirect, abort
@app.route('/old-url')
def redirect_to_new_url():
return redirect('/new-url')
@app.route('/restricted')
def restricted():
abort(403) # Forbidden
- Middleware and Extensions:
- Integrate middleware or extensions as needed for additional functionality, such as authentication, session management, or custom request processing.
- Error Handling:
- Define error handlers for specific HTTP error codes or handle generic errors. Use the
errorhandler
decorator.
- Define error handlers for specific HTTP error codes or handle generic errors. Use the
@app.errorhandler(404)
def not_found(error):
return '404 Not Found', 404
- Testing:
- Write unit tests and integration tests for the application using Flask’s testing support. Simulate HTTP requests and assert expected responses.
- Deployment:
- For production deployment, use a production-ready WSGI server like Gunicorn or uWSGI. Configure the server to run the Flask application.
- Database Integration (Optional):
- Integrate Flask with a database using an ORM like SQLAlchemy. Define data models and perform database operations within view functions.
- Security Measures:
- Implement security features such as input validation, secure session management, and protection against common vulnerabilities like XSS and CSRF.
- Scaling and Optimization (Optional):
- Optimize the application for performance and scalability as needed. Consider strategies like caching, asynchronous tasks, and load balancing for larger deployments.
- Ongoing Maintenance:
- Regularly update dependencies, address security vulnerabilities, and monitor the application’s performance. Make adjustments to the codebase based on evolving requirements.
The Flask workflow emphasizes simplicity and flexibility, allowing developers to quickly create web applications and APIs. The modular design and integration with popular Python libraries make it a versatile choice for a variety of web development projects.
How Flask Works & Architecture?
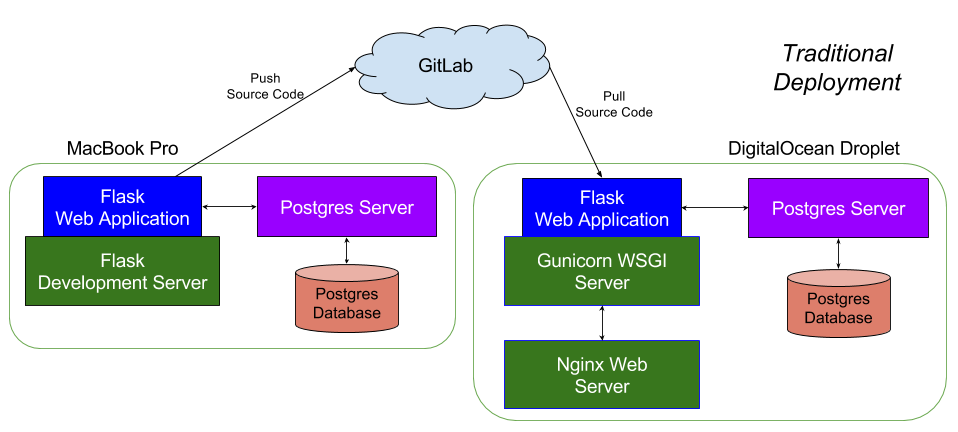
Flask is a popular web framework in Python known for its lightweight and flexible design. It allows you to build powerful web applications with clean and easy-to-understand code. Here’s a breakdown of how Flask works and its architecture:
Core Principles:
- Microframework: Minimal core functionality, encouraging extensions and plugins.
- Werkzeug-based: Built on the Werkzeug WSGI toolkit for handling HTTP requests and responses.
- Route-based: URL patterns map to Python functions (views) that process requests and generate responses.
- Templates: Jinja2 template engine enables dynamic content generation.
Workflow:
- Client request: Browser sends an HTTP request for a specific URL.
- Routing: Flask matches the requested URL to a defined route in your application.
- View execution: The corresponding Python function (view) associated with the route is called.
- Processing: The view function accesses data, performs logic, and renders a response (e.g., HTML, JSON).
- Template rendering (optional): If templates are used, the response template is filled with relevant data and converted to HTML.
- Response sent: Flask sends the final response (HTML, JSON, etc.) back to the client.
Architecture:
- Application object: Main entry point for your Flask application, initializes components and configuration.
- Routes: URL patterns associated with Python functions (views) that handle requests.
- Views: Python functions that process requests and generate responses.
- Templates: Optional Jinja2 templates for dynamic content generation.
- Extensions: Plugins to add functionalities like databases, authentication, etc.
- WSGI server: Interfaces with external web servers like Apache or Nginx to serve your application.
Key Benefits:
- Simplicity: Easy to learn and use, even for beginners.
- Flexibility: Highly customizable with extensions and plugins.
- Scalability: Can handle small and large projects efficiently.
- Lightweight: Minimal footprint, efficient in resource usage.
- Community: Large and active community for support and resources.
Use Cases:
- Web APIs: Build RESTful APIs for communication with other applications.
- Web applications: Develop dynamic and interactive websites.
- Microservices: Create small, focused services for larger applications.
- Internal tools: Build custom dashboards and internal tools.
Tips:
- Flask is a powerful tool, but understanding its basics is crucial.
- Practice with tutorials and small projects to solidify your skills.
- Don’t hesitate to ask for help from the Flask community if you encounter challenges.
How to Install and Configure Flask?
Here’s how to install and configure Flask:
1. Installation:
- Using pip: Bash
pip install Flask
2. Create a Basic Application:
- app.py:
from flask import Flask
app = Flask(name)
@app.route(‘/’) def hello_world(): return ‘Hello, World!’
if name == ‘main‘: app.run(debug=True) # Enable debug mode for development
**3. Run the Application:**
- **In your terminal:**
```bash
python app.py
- Access http://127.0.0.1:5000/ in your browser to see “Hello, World!”
4. Configuration (Optional):
- Set configuration variables: Python
app.config['DEBUG'] = True # Enable debug mode app.config['SECRET_KEY'] = 'your_secret_key' # Required for sessions app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///mydatabase.db' # Example database configuration
- Load configuration from a file: Python
app.config.from_pyfile('config.py')
5. Extensions:
- Install extensions: Bash
pip install flask-sqlalchemy flask-login # Examples
- Initialize extensions: Python
from flask_sqlalchemy import SQLAlchemy from flask_login import LoginManager db = SQLAlchemy(app) login_manager = LoginManager(app)
Important Notes:
- Development vs. Production: Use
app.run(debug=True)
for development, but switch to a production WSGI server like Gunicorn or uWSGI for deployment. - Configuration files: Store sensitive information in separate configuration files, not directly in your Python code.
- Explore extensions: Enhance your application with various extensions available for database integration, user authentication, forms, testing, and more.
Fundamental Tutorials of Flask: Getting started Step by Step
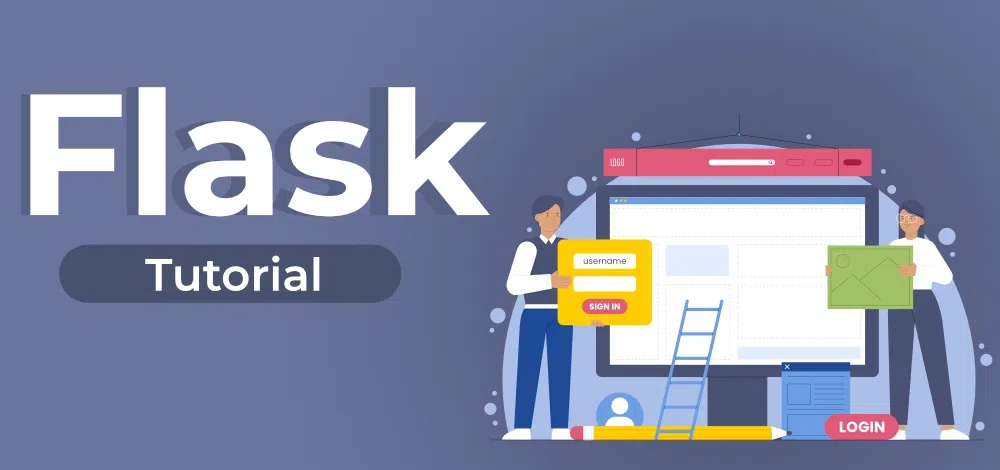
Fantastic! Since you’d like stepwise fundamental tutorials on Flask, let’s start with setting up and running a basic application. This will give you a solid foundation before delving into more advanced features.
Here’s the step-by-step breakdown:
1. Installation:
- Open your terminal or command prompt.
- Install Flask using pip:
pip install Flask
- Verify the installation by running:
python -m pip show Flask
2. Create a Project Directory:
- Create a new directory for your Flask app (e.g.,
my_flask_app
). - Navigate to the directory:
cd my_flask_app
3. Create an Application File:
- Create a file named
app.py
within your project directory. This will be your application’s central file.
4. Define the Flask Application:
- In
app.py
, import the Flask class:from flask import Flask
- Create a Flask application instance:
app = Flask(__name__)
- This instance handles routing, request processing, and response generation.
5. Create a Route and View:
- Define a route using the
@app.route
decorator:@app.route('/')
- This decorator associates a URL path with a Python function (view).
- The
/
path in this case represents the application’s main page. - Next, define the view function:
def hello_world():
- This function will be called when the user accesses the
/
path. - Inside the function, write code to return a response:
return 'Hello, World!'
- This can be plain text, HTML code, or anything you want to send back to the user.
6. Run the Application:
- Add the below code at the end of
app.py
:
Python
if __name__ == '__main__':
app.run(debug=True)
- This line checks if the script is being run directly and starts the application in debug mode.
- Debug mode helps identify errors and display detailed logs.
7. Run the App and See the Result:
- In your terminal, run:
python app.py
- This starts the Flask server.
- Open your web browser and navigate to http://localhost:5000/
- You should see the response “Hello, World!” displayed on the page.
Congratulations! You’ve successfully created and run your first Flask application.
Notes:
- This is just the beginning! You can build on this foundation to add more features like templates, forms, databases, and authentication.
- Don’t hesitate to ask if you have any questions or need clarification at any point.
I’m excited to guide you further on your Flask journey! Let me know when you’re ready to explore more advanced features, and we can take the next step together.
- Mutual of Omaha: Selection and Interview process, Questions/Answers - April 15, 2024
- AES: Selection and Interview process, Questions/Answers - April 15, 2024
- Amphenol: Selection and Interview process, Questions/Answers - April 15, 2024