What is Git?
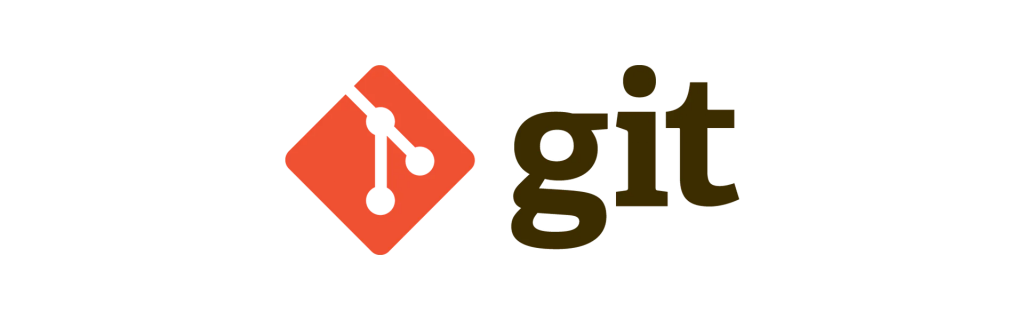
Git is a distributed version control system (DVCS) planned to track changes in source code during software development. It was developed by Linus Torvalds in 2005 and has become the de facto standard for version control in the software development industry.
What is top use cases of Git?
Top Use Cases of Git:
- Source Code Version Control:
- Description: Git is primarily used for tracking changes in source code, enabling developers to collaborate on projects, maintain version history, and manage codebase modifications.
- Collaborative Development:
- Description: Git facilitates collaboration among multiple developers working on the same project. It allows them to work on different features or bug fixes concurrently and merge their changes seamlessly.
- Branching and Merging:
- Description: Git’s branching and merging capabilities enable developers to create isolated branches for new features or bug fixes. After development and testing, branches can be merged back into the main codebase.
- Code Review:
- Description: Developers use Git to create feature branches and submit pull requests for code review. Team members can review the changes, provide feedback, and discuss modifications before merging.
- Undoing Changes:
- Description: Git provides mechanisms to revert or undo changes in the codebase. This is valuable for rolling back to a previous state in case of errors or undesired modifications.
- Release Management:
- Description: Git supports the creation of release branches, allowing teams to manage different versions of their software. This is crucial for maintaining and deploying stable releases while ongoing development continues.
- Continuous Integration and Continuous Deployment (CI/CD):
- Description: Git integrates seamlessly with CI/CD pipelines, allowing automated testing, building, and deployment processes. This ensures that changes are automatically validated and deployed to production environments.
- Distributed Development:
- Description: Git’s distributed nature enables developers to work offline and synchronize changes when they reconnect to the network. This is particularly useful for distributed teams and remote collaboration.
- Codebase Auditing:
- Description: Git’s version history provides a detailed audit trail of changes made to the codebase. Developers and project managers can track who made changes, when, and what modifications were introduced.
- Open Source Collaboration:
- Description: Many open-source projects use Git for collaboration among contributors around the world. It allows for easy forking, cloning, and contribution to projects hosted on platforms like GitHub, GitLab, and Bitbucket.
- Bug Tracking:
- Description: Developers can use Git to track and investigate the introduction of bugs. By examining the commit history, it becomes easier to identify when and where issues were introduced.
- Documentation Management:
- Description: Developers can version control documentation alongside code, ensuring that documentation stays in sync with code changes. This is essential for maintaining accurate and up-to-date project documentation.
- Experimentation and Prototyping:
- Description: Developers can create branches to experiment with new features or changes without affecting the main codebase. This allows for prototyping and testing ideas before incorporating them into the main project.
- Forking and Pull Requests:
- Description: In open-source and collaborative projects, developers can fork repositories, make changes, and submit pull requests to contribute their modifications. This process facilitates collaboration and code review.
- Historical Analysis:
- Description: Git’s version history provides a historical view of the codebase, helping developers analyze trends, understand the evolution of the project, and make informed decisions about future development.
Git’s versatility and efficiency make it an essential tool for version control in software development, enabling teams to collaborate effectively, manage codebase changes, and maintain the integrity and stability of their projects.
What are feature of Git?
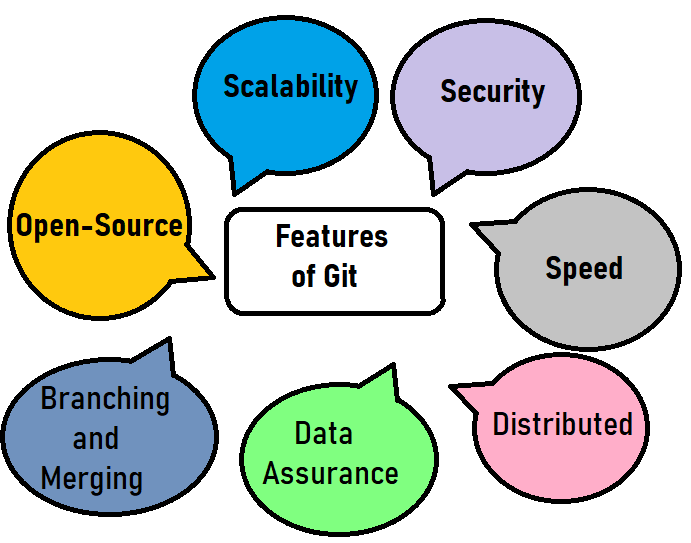
Features of Git:
- Distributed Version Control:
- Description: Git is a distributed version control system, allowing each developer to have a local copy of the entire project history. This enables offline work and easy collaboration.
- Branching and Merging:
- Description: Git provides powerful branching and merging capabilities. Developers can create branches for new features or bug fixes and merge them back into the main branch.
- Commit History:
- Description: Git maintains a detailed commit history, recording every change made to the codebase. Each commit is associated with a unique hash and includes information about the author, timestamp, and changes.
- Staging Area (Index):
- Description: Git uses a staging area, also known as the index, where developers can selectively choose which changes to include in the next commit. This permits for more controlled and granular commits.
- Fast and Lightweight:
- Description: Git is designed to be fast and lightweight, with operations such as committing, branching, and merging being quick and efficient even in large codebases.
- Security and Integrity:
- Description: Git ensures the integrity of the codebase by using cryptographic hashing for content. Each commit is identified by a unique SHA-1 hash, making it resistant to tampering.
- Merge Conflict Resolution:
- Description: When multiple developers make conflicting changes to the same file, Git helps in resolving merge conflicts. Developers can manually resolve conflicts during the merge process.
- Easy Collaboration:
- Description: Git facilitates collaboration by allowing developers to clone repositories, contribute changes, and merge contributions from multiple contributors. Platforms like GitHub, GitLab, and Bitbucket enhance collaboration further.
- Tagging:
- Description: Git allows developers to create tags to mark specific points in history, such as releases or milestones. Tags provide a stable reference to specific commits.
- Submodule Support:
- Description: Git supports submodules, allowing developers to include external repositories as part of their project. This is useful for managing dependencies and incorporating external libraries.
- Customizable Workflows:
- Description: Git is flexible, allowing teams to define and customize their workflows. Whether using a feature branching model, Gitflow, or another workflow, Git adapts to the team’s preferences.
- Blame and Annotate:
- Description: Git provides tools like
git blame
andgit annotate
to trace changes in a file back to specific commits and authors. This aids in understanding the history and context of code changes.
- Description: Git provides tools like
- Parallel Development:
- Description: Developers can work in parallel on different branches, making it easy to implement new features or bug fixes without interfering with each other’s work.
- Rebasing:
- Description: Git supports rebasing, a process that allows developers to rewrite commit history. This can be useful for cleaning up history or incorporating changes from one branch into another.
- Hooks:
- Description: Git supports hooks, allowing developers to trigger custom scripts at specific points in the version control process. Hooks can automate tasks such as running tests before commits or sending notifications.
What is the workflow of Git?
Workflow of Git:
- Clone:
- Action: Clone a Git repository to create a local copy on your machine.
- Command:
git clone <repository-url>
- Branch:
- Action: Create a new branch to work on a specific feature or bug fix.
- Command:
git branch <branch-name>
- Switch Branch:
- Action: Switch to the branch where you want to make changes.
- Command:
git checkout <branch-name>
orgit switch <branch-name>
(Git version 2.23 and later)
- Work and Stage:
- Action: Make changes to files and use the staging area to select changes for the next commit.
- Commands:
- Make changes: Edit files.
- Stage changes:
git add <file>
orgit add .
(to stage all changes).
- Commit:
- Action: Commit the staged changes with a descriptive commit message.
- Command:
git commit -m "Descriptive message"
- Push:
- Action: Push the committed changes to the remote repository.
- Command:
git push origin <branch-name>
- Pull:
- Action: Pull changes from the remote repository to sync with others’ contributions.
- Command:
git pull origin <branch-name>
- Merge:
- Action: Merge changes from one branch into another.
- Commands:
- Switch to the target branch:
git checkout <target-branch>
- Merge changes:
git merge <source-branch>
- Switch to the target branch:
- Rebase (Optional):
- Action: Rebase commits to incorporate changes from one branch into another.
- Commands:
- Switch to the target branch:
git checkout <target-branch>
- Rebase:
git rebase <source-branch>
- Switch to the target branch:
- Tagging:
- Action: Create a tag to mark a specific point in history.
- Command:
git tag -a <tag-name> -m "Tag message"
- Fetch:
- Action: Fetch changes from the remote repository without merging.
- Command:
git fetch
- Resolve Conflicts:
- Action: Resolve merge conflicts if they occur during the pull or merge process.
- Commands: Manually edit conflicted files, then
git add
andgit commit
.
- View History:
- Action: View the commit history and changes made in the repository.
- Commands:
git log
,git show
, or use graphical tools.
- Push Tags:
- Action: Push tags to the remote repository.
- Command:
git push --tags
How Git Works & Architecture?
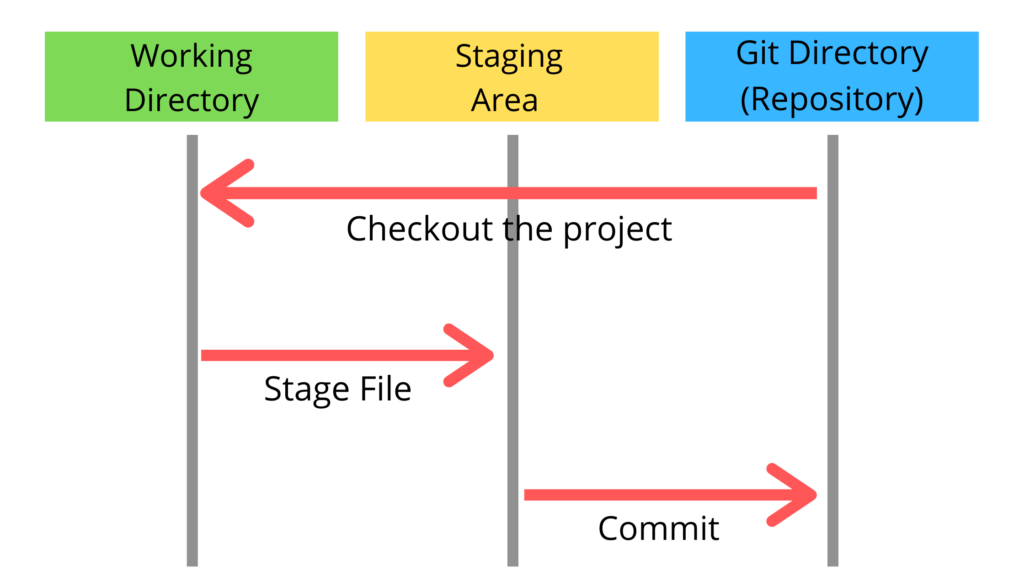
Git is a distributed version control system (DVCS) that helps developers track changes in their code and collaborate effectively. It allows teams to work on the same codebase simultaneously while maintaining a clear history of changes.
Following is a breakdown of how Git works and its architecture:
1. Core Concepts:
- Repository: A directory containing all the project files and their history.
- Branch: A distribute line of development within a repository.
- Commit: A snapshot of the project files at a specific point in time.
- Working directory: The local copy of the repository where developers make changes.
- Staging area: A temporary area where developers prepare changes before committing them.
2. Git Workflow:
- Clone: Create a local copy of a remote repository.
- Make changes: Modify files in the working directory.
- Stage changes: Add modified files to the staging area.
- Commit changes: Create a snapshot of the staged changes with a descriptive message.
- Push changes: Upload local commits to the remote repository.
- Pull changes: Download changes from the remote repository to your local repository.
- Branching and merging: Create branches to work on different features or to isolate changes before merging them back into the main codebase.
3. Git Architecture:
- Distributed: Every developer has a complete copy of the repository, allowing offline work and collaboration.
- Content-addressable: Git stores files by their content, not their names, ensuring data integrity and efficient history tracking.
- Object-based: Git stores files and metadata as individual objects, making it lightweight and efficient.
- Branching: Enables parallel development on different features without affecting the main codebase.
- Merging: Allows integrating changes from different branches into a single codebase.
4. Benefits of Git:
- Version control: Track changes, revert to previous versions, and collaborate effectively.
- Branching and merging: Work on different features simultaneously and seamlessly integrate them.
- Offline work: Make changes without an internet connection and synchronize with the remote repository later.
- Distributed development: Collaborate with others without relying on a central server.
- Security: Data integrity is protected through content-addressing and cryptographic hashing.
By understanding how Git works and its architecture, you can leverage its power for version control, collaboration, and efficient development workflows. Start practicing with the basic commands, explore branching and merging, and utilize online resources to master this essential tool for modern software development.
How to Install and Configure Git?
Installing and Configuring Git
1. Installation:
- Download and install Git:
- Linux: Use the package manager for your distribution.
- Mac: Download the installer from https://git-scm.com/download/mac
- Windows: Download the installer from https://git-scm.com/downloads
2. Basic Configuration:
- Open Git Bash: This is the command-line interface for Git.
- Set your username and email address: Use the
git config
command:git config --global user.name "Your Name" git config --global user.email "your_email@example.com"
- Verify your configuration: Use the
git config --list
command to see your settings.
3. Optionally Install Additional Tools:
- GitHub Desktop: A graphical user interface for Git. Available for Windows, Mac, and Linux.
- SourceTree: Another popular Git GUI client. Available for Windows and Mac.
- Visual Studio Code: Includes built-in Git integration.
4. Basic Git Commands:
- Initialize a repository:
git init
- Clone an existing repository:
git clone https://github.com/username/repository.git
- Add files to the staging area:
git add <file_name>
- Commit changes:
git commit -m "Commit message"
- Push changes to a remote repository:
git push origin main
- Pull changes from a remote repository:
git pull origin main
- View the commit history:
git log
Important Tips:
- Start with the basic commands and learn them well before moving on to more advanced features.
- Apply online resources and tutorials to enhance your understanding.
- Practice regularly with real projects to gain practical experience.
- Engage with the Git community for support and advice.
By following these steps and practicing consistently, you can become proficient in using Git for your version control needs.
Fundamental Tutorials of Git: Getting started Step by Step
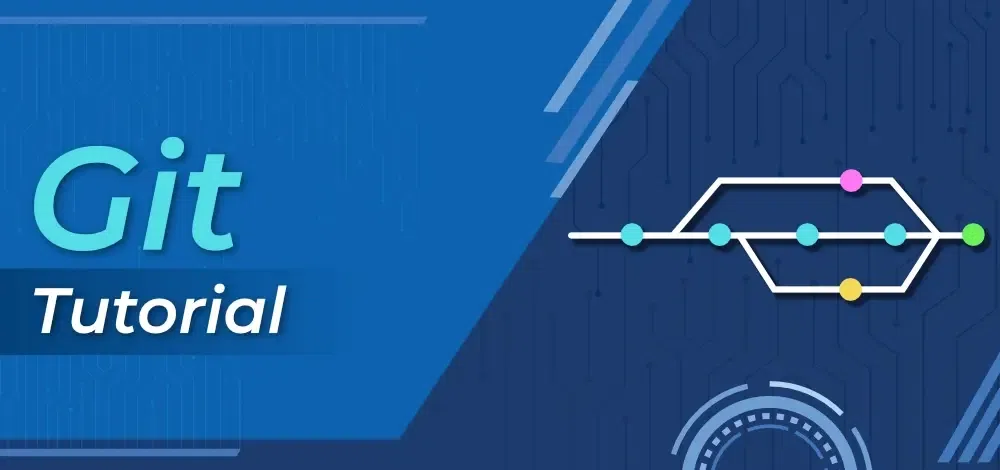
Let’s have a look at some step-by-step fundamental tutorials to get you started with Git:
1. Setting Up:
- Install Git: Download and install Git for your operating system.
- Open Git Bash: This is your command-line interface for Git.
- Configure Git: Use
git config
to set your username and email.
2. Creating a Repository:
- Open a terminal window in the directory where you want to create the repository.
- Run
git init
to initialize a new Git repository. - This creates a
.git
directory within your project directory, which stores all the Git data.
3. Adding and Committing Changes:
- Create or edit files in your project directory.
- Use
git add
to add the changed files to the staging area. - This tells Git that you want to track these files in the version history.
- Run
git commit -m "Commit message"
to commit the staged changes. - The commit message should be a brief description of what you changed.
4. Working with Branches:
- By default, you are working on the
main
branch. - To create a new branch, run
git branch <branch_name>
. - To switch to a different branch, run
git checkout <branch_name>
. - You can use
git branch
to see a list of all branches.
5. Pushing and Pulling Changes:
- To push your local changes to a remote repository, run
git push origin main
. - This will upload your changes to the
main
branch on the remote repository. - To pull changes from a remote repository, run
git pull origin main
. - This will download the latest changes from the
main
branch on the remote repository and merge them into your local repository.
6. Undoing Changes:
- To undo your last commit, use
git reset HEAD~1
. - This will uncommit the last commit and move your working directory back to the previous state.
- To discard all changes in your working directory, use
git checkout .
. - This will overwrite your working directory with the latest version from the Git repository.
7. Useful Commands:
git status
: Shows the current status of your working directory and staging area.git log
: Shows the commit history of your repository.git diff
: Shows the difference between your working directory and the last commit.
Important Tips:
- Start with the basic commands and practice them frequently.
- Utilize online resources for further learning and troubleshooting.
- Work on real projects to gain practical experience with Git.
- Engage with the Git community for support and guidance.
Remember, becoming proficient in Git takes time and practice. By following these steps and consistently working with Git, you can master this essential tool for version control and collaboration.
- Mutual of Omaha: Selection and Interview process, Questions/Answers - April 15, 2024
- AES: Selection and Interview process, Questions/Answers - April 15, 2024
- Amphenol: Selection and Interview process, Questions/Answers - April 15, 2024