What is Go?
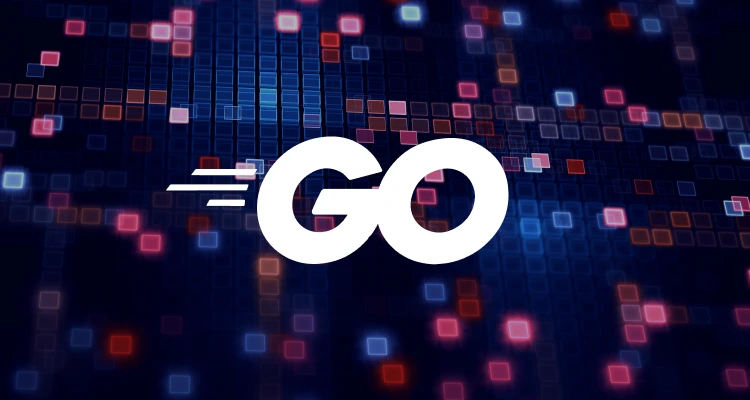
Go, also known as Golang, is a statically typed, compiled programming language planned by Google engineers Robert Griesemer, Rob Pike, and Ken Thompson. It was first announced in 2009 and has gained popularity for its simplicity, efficiency, and built-in support for concurrent programming.
Key Features of Go:
- Concurrency Support:
- Go has built-in support for concurrent programming through goroutines (lightweight threads) and channels. This makes it easy to write concurrent and parallel programs.
- Efficiency:
- Go is designed for efficiency and speed. It compiles to machine code, and its runtime is lightweight, resulting in fast execution and low memory usage.
- Simplicity:
- Go emphasizes simplicity and readability. It has a clean and straightforward syntax, making it easy for developers to write and maintain code.
- Garbage Collection:
- Go includes automatic garbage collection, simplifying memory management for developers and reducing the risk of memory leaks.
- Static Typing:
- Go is statically typed, providing compile-time type checking. This helps find errors early in the development process.
- Standard Library:
- Go comes with a comprehensive standard library that includes packages for networking, cryptography, file I/O, and more. This decreases the need for external dependencies.
- Cross-Platform Support:
- Go is designed to be cross-platform, and applications written in Go can be easily compiled and run on various operating systems without modification.
- Open Source:
- Go is open-source, encouraging community contributions and fostering a collaborative development environment.
- Tooling:
- Go has a powerful set of built-in tools, including a formatter (
gofmt
), a package manager (go get
), and a testing framework. These tools enhance the development experience.
- Go has a powerful set of built-in tools, including a formatter (
- Static Binary Compilation:
- Go compiles code into static binaries, which simplifies deployment by eliminating the need for external runtime dependencies.
What is top use cases of Go?
Top Use Cases of Go:
- Web Development:
- Go is used for building web applications and APIs. Popular web frameworks like Gin and Echo are written in Go. Its efficiency and concurrency support make it well-suited for handling web requests.
- Backend Development:
- Go is commonly used for backend development, powering server-side components of applications. It is used in scalable and high-performance backend systems.
- DevOps Tools:
- Go is widely adopted in the development of DevOps tools and infrastructure software. Tools like Docker, Kubernetes, and Terraform are written in Go.
- Cloud Services:
- Go is used in the development of cloud services and microservices. Its efficient runtime and concurrency support make it suitable for building scalable and distributed systems.
- Networking Tools:
- Go is popular for building networking tools and applications due to its efficiency and support for concurrent programming. Networking libraries like net/http are part of the standard library.
- Command-Line Utilities:
- Go is well-suited for building command-line utilities and tools. The simplicity and efficiency of Go make it a good choice for writing utilities that perform various tasks.
- Containers and Orchestration:
- Go is used extensively in the container ecosystem. Docker, a containerization platform, is written in Go. Additionally, Go is used in container orchestration systems like Kubernetes.
- Data Pipelines:
- Go is employed in building data pipelines and processing systems. Its concurrent programming features make it suitable for parallelizing data processing tasks.
- Game Development:
- Go is used in game development, particularly for building server-side components of online games. Its efficiency and low-latency characteristics make it a good fit for certain aspects of game servers.
- Monitoring and Logging Tools:
- Go is used to build monitoring and logging tools that help developers track the performance and health of applications in production.
- Blockchain and Cryptocurrency:
- Go is used in the development of blockchain and cryptocurrency-related projects. The efficiency and performance of Go make it suitable for building components of decentralized systems.
- Embedded Systems:
- Go is used in the development of software for embedded systems. Its efficiency and static binary compilation are beneficial in resource-constrained environments.
- Machine Learning and Data Science:
- While not as prevalent as some other languages in the machine learning domain, Go is used in certain machine learning and data science applications. Libraries like Gorgonia provide machine learning capabilities.
- Cross-Platform Development:
- Go is used for cross-platform development, allowing developers to write applications that can run on multiple operating systems without modification.
Go’s design principles of simplicity, efficiency, and concurrency make it well-suited for modern software development, particularly in areas where performance and scalability are crucial. Its adoption continues to grow across various industries and use cases.
What are feature of Go?
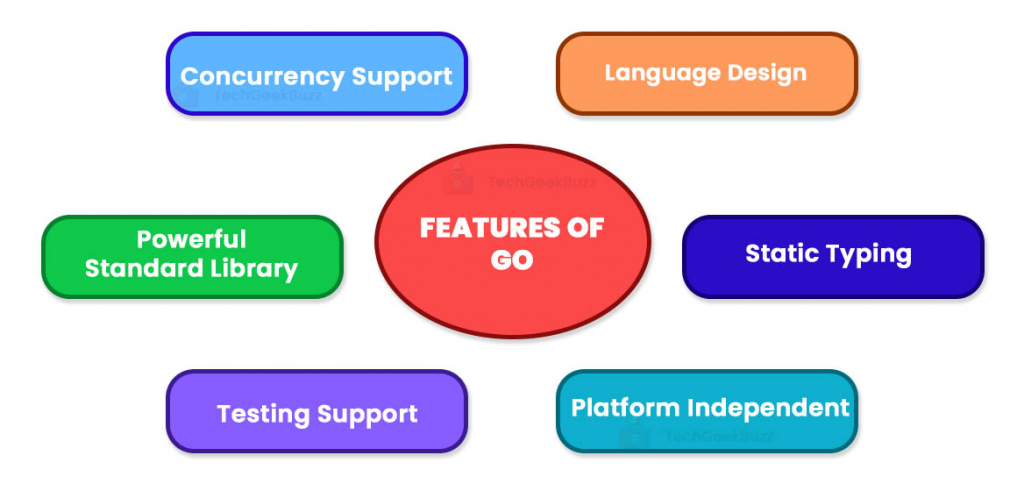
Features of Go (Golang):
- Concurrency Support:
- Go is designed with concurrency in mind. Goroutines and channels facilitate concurrent programming, allowing developers to write efficient and concurrent code.
- Efficiency:
- Go is compiled to machine code, resulting in fast execution. Its runtime is lightweight, leading to low memory usage and efficient resource utilization.
- Simplicity:
- Go emphasizes simplicity and readability. Its syntax is clean and straightforward, making it easy for developers to write and maintain code.
- Garbage Collection:
- Go includes automatic garbage collection, simplifying memory management for developers and reducing the risk of memory leaks.
- Static Typing:
- Go is statically typed, providing compile-time type checking. This helps find errors early in the development process.
- Standard Library:
- Go comes with a comprehensive standard library that covers a wide range of functionalities, including networking, cryptography, file I/O, and more. This decreases the need for external dependencies.
- Cross-Platform Support:
- Go is designed to be cross-platform, and applications written in Go can be easily compiled and run on various operating systems without modification.
- Open Source:
- Go is open-source, fostering a collaborative development environment and encouraging community contributions.
- Tooling:
- Go has a powerful set of built-in tools, including a formatter (
gofmt
), a package manager (go get
), and a testing framework. These tools enhance the development experience.
- Go has a powerful set of built-in tools, including a formatter (
- Static Binary Compilation:
- Go compiles code into static binaries, simplifying deployment by eliminating the need for external runtime dependencies.
- Concurrency Primitives:
- Go provides powerful concurrency primitives, such as goroutines (lightweight threads) and channels, which simplify the development of concurrent and parallel programs.
- Error Handling:
- Go uses a simple and explicit error handling mechanism, where functions that may return errors explicitly indicate this in their return types.
- Interfaces:
- Go features interfaces, allowing developers to define sets of methods that types can implement. This enables polymorphism and code reuse.
- Reflection:
- Go supports reflection, allowing programs to inspect and manipulate their own structure at runtime. While reflection is discouraged in idiomatic Go code, it can be useful in certain situations.
What is the workflow of Go?
The typical workflow for developing a Go application involves the following steps:
- Install Go:
- Install the Go programming language on your development machine. The official website provides installation instructions.
- Set Up Workspace:
- Organize your Go projects within a workspace. The workspace typically includes a
src
directory for source code, apkg
directory for package objects, and abin
directory for compiled binaries.
- Organize your Go projects within a workspace. The workspace typically includes a
- Create a Go Module:
- Use Go modules to manage dependencies and versioning. Initialize a new Go module using the
go mod init
command.
- Use Go modules to manage dependencies and versioning. Initialize a new Go module using the
- Write Code:
- Create Go source files with the desired functionality. Use a text editor or integrated development environment (IDE) for code writing.
- Package Structure:
- Organize your code into packages. A package is a collection of Go source files in the same directory that can be compiled together.
- Testing:
- Write unit tests using the
testing
package. Run tests with thego test
command.
- Write unit tests using the
- Formatting:
- Format your code using the
gofmt
tool to ensure a consistent code style. You can also usegolint
for additional code analysis.
- Format your code using the
- Dependency Management:
- Use the
go get
command to download and install external dependencies. Thego mod
commands help manage dependencies and versions.
- Use the
- Build and Run:
- Use the
go build
command to compile your Go code into an executable binary. Run the executable to test your application.
- Use the
- Documentation:
- Write documentation for your code using comments. The
godoc
tool generates documentation based on these comments.
- Write documentation for your code using comments. The
- Benchmarking:
- Use the
testing
package to create benchmarks for critical parts of your code. Run benchmarks with thego test -bench
command.
- Use the
- Profiling:
- Profile your code for performance analysis using tools like
pprof
. Identify bottlenecks and optimize as needed.
- Profile your code for performance analysis using tools like
- Version Control:
- Apply version control systems like Git to track changes in your code. Provide your code on platforms like GitLab, GitHub, or Bitbucket.
- Continuous Integration (CI):
- Set up CI pipelines to automate testing and deployment processes. Platforms like Travis CI, GitHub Actions, and GitLab CI integrate well with Go projects.
- Code Review:
- Conduct code reviews to ensure code quality and adherence to best practices. Use tools like
golangci-lint
for additional static analysis.
- Conduct code reviews to ensure code quality and adherence to best practices. Use tools like
- Deployment:
- Deploy your Go application to the desired environment. Since Go produces statically linked binaries, deployment is often as simple as copying the binary to the target system.
- Monitoring and Maintenance:
- Monitor your deployed application for performance and issues. Address bugs promptly and consider updates and improvements.
The Go workflow is designed to be straightforward, emphasizing simplicity and efficiency. It encourages best practices such as testing, documentation, and version control, making it conducive to building reliable and maintainable software.
How Go Works & Architecture?
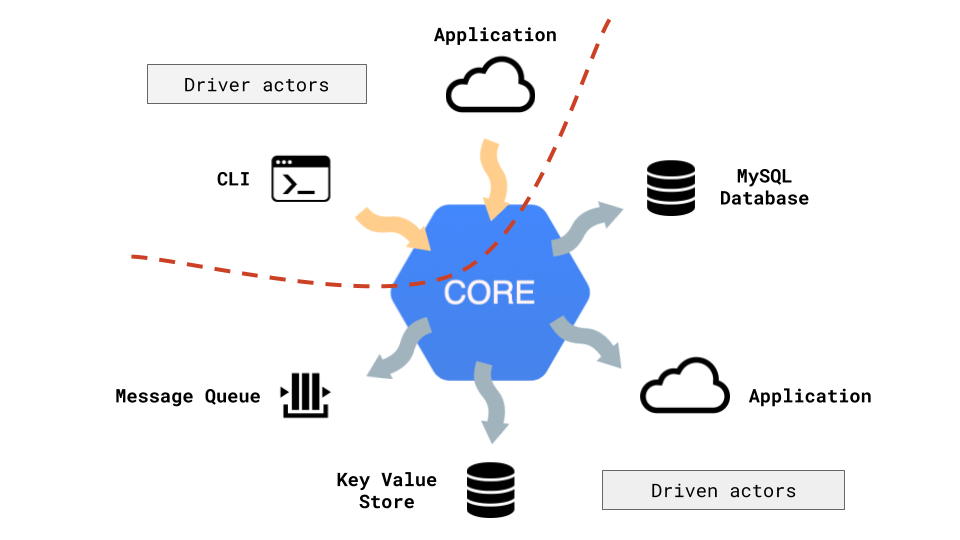
Go, also known as Golang, is a powerful and popular programming language known for its simplicity, concurrency features, and performance. Here’s a breakdown of how Go works and its core architectural principles:
1. Compiled Language:
Go is a compiled language, meaning your code is translated into machine code before execution. This offers faster running times compared to interpreted languages.
2. Statically Typed:
Go is statically typed, meaning you need to declare the type of your variables upfront. This provides better type safety and helps prevent runtime errors.
3. Concurrency Primitives:
Go excels in concurrency, facilitating parallel execution of tasks through several mechanisms:
- Goroutines: Lightweight threads that share the same memory space, enabling efficient asynchronous code.
- Channels: Communication channels for goroutines to safely exchange data without race conditions.
- Select Statements: Non-blocking synchronization mechanism for choosing which channel to receive from or operation to perform.
4. Garbage Collection:
Go features automatic garbage collection, automatically clearing unused memory, simplifying memory management for developers.
5. Package-Based Organization:
Go code is organized into packages, promoting modularity and code reuse. Each package has its own namespace and can be imported by other packages.
6. Simple Syntax:
Go aims for clean and concise syntax, minimizing boilerplate code and focusing on expressiveness. This makes it easier to learn and write maintainable code.
7. Standard Library:
Go provides a comprehensive standard library offering essential functionalities like file I/O, networking, cryptography, data structures, and more.
8. Build System:
Go features a simple and versatile build system that allows efficient compilation and testing of your code.
9. Cross-Platform:
Go is compiled code, but the compiler itself is written in Go and runs on various platforms like Linux, Windows, macOS, and more. This makes Go code inherently portable.
10. Open Source Community:
Go has a large and active open-source community contributing to its development and providing extensive learning resources and libraries.
Benefits of Go:
- Speed and performance: Efficient concurrency and compiled nature offer high performance.
- Simplicity and maintainability: Clear syntax and minimal boilerplate code make it easy to read and write.
- Concurrency features: Built-in mechanisms simplify building robust asynchronous applications.
- Standard library: Comprehensive library reduces dependence on external packages.
- Cross-platform: Code runs on various platforms without modification.
- Active community: Continuous development and ample resources for learning and support.
Go’s architectural principles focus on simplicity, efficiency, and concurrency, making it a versatile and powerful language for various applications, from web development and cloud computing to systems programming and machine learning.
How to Install and Configure Go?
Following is a comprehensive guide to installing and configuring Go on different platforms:
1. Download the Installer:
- Visit the official Go website.
- Download the installer suitable for your operating system (Windows, macOS, Linux).
2. Run the Installer:
- Launch the installer and use the on-screen instructions.
- Accept the license agreement and choose the installation directory (default is usually fine).
3. Set Up Environment Variables (Optional):
- Windows:
- Add the Go installation directory’s bin folder to your PATH environment variable.
- Access system settings, search for “environment variables,” and edit the PATH variable.
- macOS/Linux:
- The installer usually sets up environment variables automatically.
- If needed, edit your .bashrc or .zshrc file to add the Go bin directory to PATH.
4. Check Installation:
- Open a terminal or command prompt.
- Type
go version
and press Enter. - If installed correctly, you’ll see the Go version displayed.
5. Create a Workspace (Optional but Recommended):
- Go recommends organizing projects within a workspace directory:
- Create a directory for your workspace (e.g.,
mkdir go_projects
). - Set the GOPATH environment variable to this directory.
- This helps manage multiple Go projects effectively.
- Create a directory for your workspace (e.g.,
6. Install Go Tools (Optional):
- Install helpful Go tools like:
gocode
: Code completiongofmt
: Code formattinggorename
: Renaming variables and packages- Use a package manager like
go get
to install these tools.
Important Tips:
- IDEs/Editors: Choose a code editor or IDE with Go support (e.g., Visual Studio Code, GoLand).
- Version Management: Use tools like
gvm
orgoenv
to manage multiple Go versions.
Troubleshooting:
- If you encounter issues, consult Go’s documentation, community forums, or seek help from experienced Go developers.
Fundamental Tutorials of Go: Getting started Step by Step
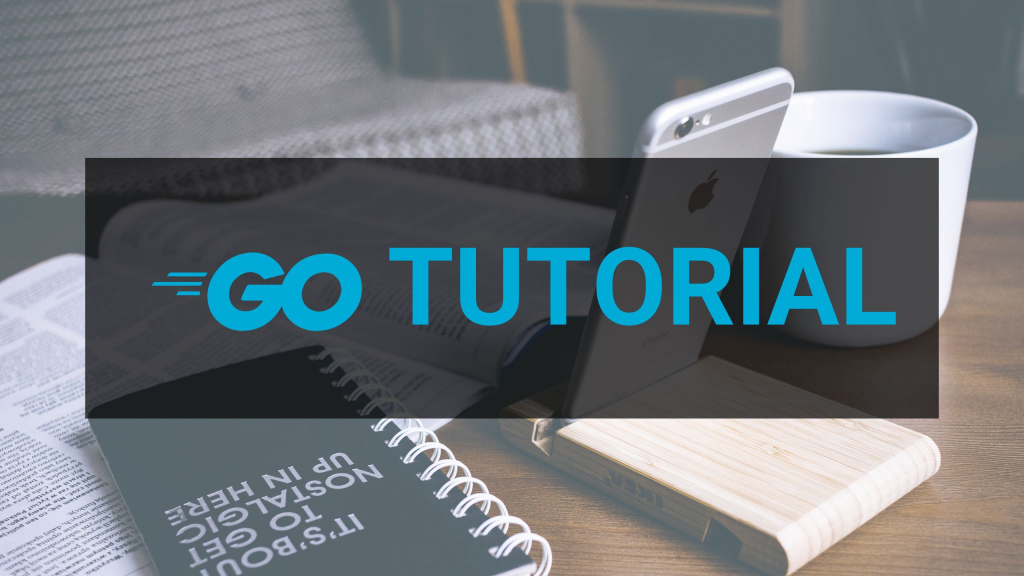
Welcome to the world of Go programming! Following is a guide to get you started with simple Go tutorials:
1. Hello World!:
Open your terminal/command prompt and type:
Go
package main
import "fmt"
func main() {
fmt.Println("Hello, Go!")
}
Save this code as hello.go
and run it using:
go run hello.go
You will see “Hello, Go!” printed in the console after run the above command.
2. Variables and Constants:
Declare variables using var
or :=
(automatic type inference):
Go
var name string = "John Doe"
age := 30
const pi = 3.14159
3. Data Types:
Go offers various data types like int
, float64
, bool
, string
, and complex types like struct
and array
.
4. Basic Operators:
Arithmetic, comparison, and logical operators work as expected.
5. Control Flow:
Use if
, else
, for
, and switch
statements for conditional execution.
6. Functions:
Define functions with func
and call them with arguments:
Go
func add(a, b int) int {
return a + b
}
var sum = add(5, 3) // sum will be 8
7. Packages and Imports:
Organize code into packages and import them with import
:
Go
package mymath
func square(x int) int {
return x * x
}
package main
import "mymath"
func main() {
fmt.Println(mymath.square(5)) // prints 25
}
8. Error Handling:
Use error
type to handle errors:
Go
func readFile(filename string) (string, error) {
// ...read file content
if err != nil {
return "", err // return error if any
}
return content, nil // return content and nil if no error
}
9. Pointers:
Pointers reference memory locations of variables for advanced data manipulation.
10. Concurrency:
Go’s strong suit! Explore Go routines and channels for parallel execution and communication.
Feel free to ask further questions about specific Go concepts or challenges you encounter while practicing these tutorials. Happy coding!
- Mutual of Omaha: Selection and Interview process, Questions/Answers - April 15, 2024
- AES: Selection and Interview process, Questions/Answers - April 15, 2024
- Amphenol: Selection and Interview process, Questions/Answers - April 15, 2024