What is Haskell?
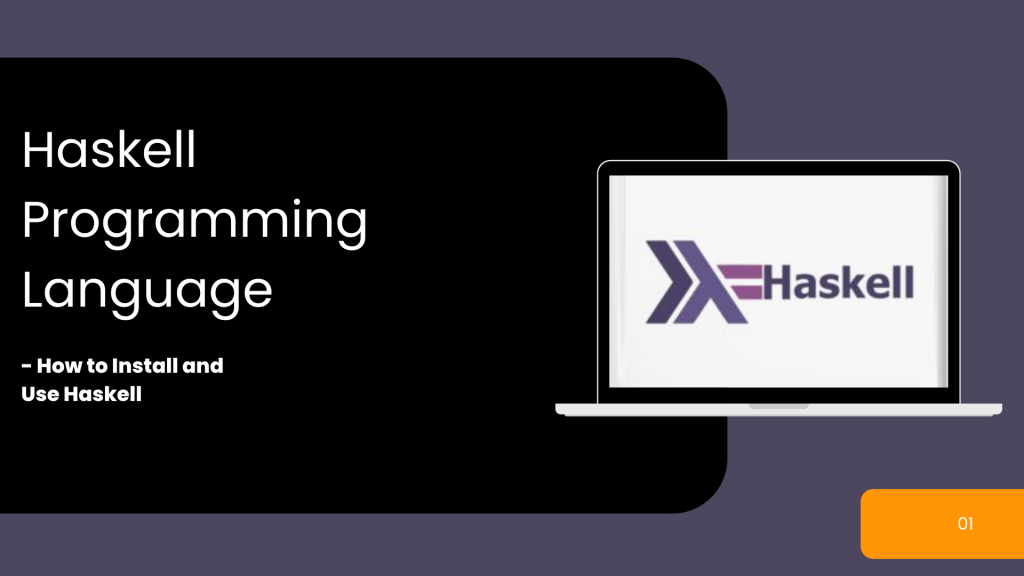
Haskell is a functional programming language that is known for its strong static typing, lazy evaluation, and expressive syntax. It is named after the logician Haskell Curry and has roots in mathematical logic. Haskell is designed to be purely functional, meaning that functions in Haskell are mathematical entities without side effects.
What is top use cases of Haskell?
Top Use Cases of Haskell:
- Academic Research:
- Haskell is widely used in academic research, especially in the fields of programming languages, formal methods, and functional programming. Its strong emphasis on mathematical principles makes it an ideal language for exploring new ideas and concepts.
- Functional Programming Paradigm:
- Haskell serves as a primary example of a purely functional programming language. It is often used to teach functional programming concepts, such as immutability, higher-order functions, and lazy evaluation.
- Compiler Construction:
- Haskell is used in the construction of compilers and interpreters. The language’s expressive type system and powerful abstractions make it well-suited for the implementation of language processors.
- Domain-Specific Languages (DSLs):
- Haskell is used for building domain-specific languages (DSLs) due to its expressive syntax and powerful abstractions. DSLs built in Haskell are often concise and expressive, making them suitable for specific problem domains.
- Concurrent and Parallel Programming:
- Haskell’s functional and pure nature makes it well-suited for concurrent and parallel programming. The language provides abstractions for concurrent and parallel execution, and it avoids many of the pitfalls associated with shared mutable state.
- Financial Modeling:
- Haskell is used in the financial industry for tasks such as risk analysis, modeling, and algorithmic trading. The language’s strong type system and mathematical foundation make it suitable for building reliable financial software.
- Data Analysis and Visualization:
- Haskell is employed in data analysis and visualization tasks. Libraries like
Haskell Data Analysis Library (haskell-data-analysis)
anddiagrams
make it possible to process and visualize data effectively.
- Haskell is employed in data analysis and visualization tasks. Libraries like
- Formal Verification:
- Haskell’s strong type system and emphasis on mathematical correctness make it suitable for formal verification tasks. It is used in the development of verified software, where correctness is rigorously proven.
- Web Development:
- Haskell is used for web development, particularly in building backend services. Frameworks like Yesod and Servant provide web development capabilities with type-safe routing and strong type checking.
- Artificial Intelligence and Machine Learning:
- Haskell is utilized in the development of AI and machine learning applications. Libraries such as
haskell-learn
andhaskell-ml
provide functional programming capabilities for machine learning tasks.
- Haskell is utilized in the development of AI and machine learning applications. Libraries such as
- Networking and Protocol Implementations:
- Haskell is used for networking applications and the implementation of various network protocols. Its strong type system and expressive syntax contribute to the development of reliable and maintainable networking software.
- Mathematical and Symbolic Computing:
- Haskell is well-suited for mathematical and symbolic computing tasks. It is used in areas such as symbolic mathematics, theorem proving, and mathematical modeling.
- Bioinformatics:
- Haskell is employed in bioinformatics for tasks like sequence analysis, genome mapping, and bioinformatics algorithm development. The language’s expressive nature is beneficial in handling complex biological data.
- Game Development:
- Haskell is used in the development of certain game engines and components. While not as common as some other languages in the game development industry, Haskell’s functional paradigm has found applications in specific contexts.
- Education and Teaching:
- Haskell is used as an educational tool for teaching programming concepts, functional programming, and advanced language features. Its focus on mathematical abstractions provides a solid foundation for students studying computer science.
Haskell’s unique features, including lazy evaluation, type classes, and monads, make it a distinctive language with applications in diverse areas, particularly where a strong emphasis on correctness, expressiveness, and mathematical foundations is desired.
What are feature of Haskell?
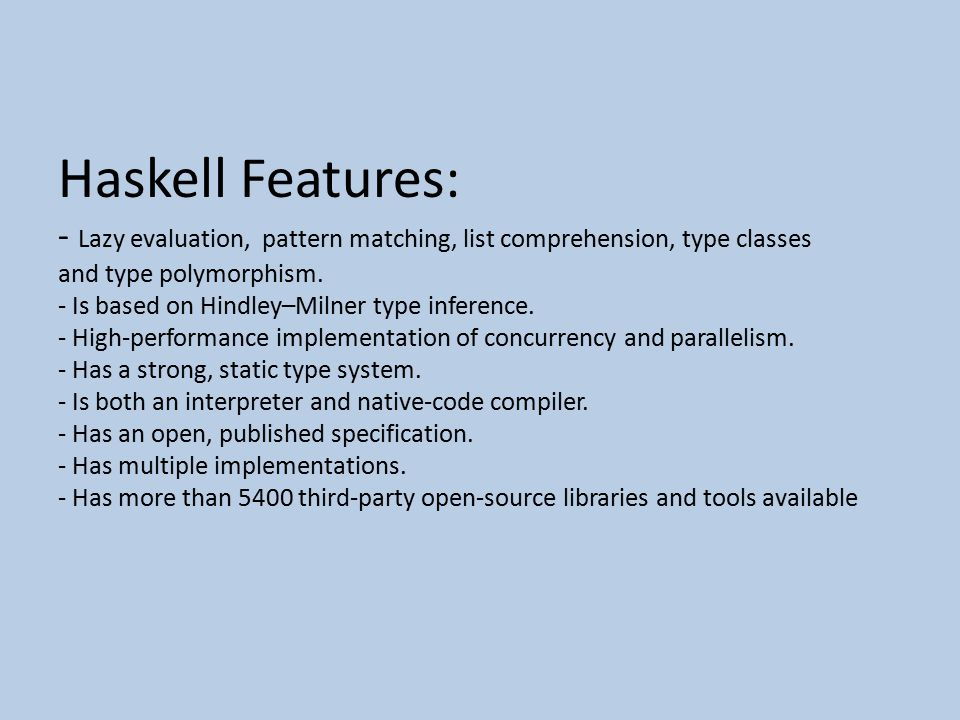
Features of Haskell:
- Functional Programming Paradigm:
- Haskell is a purely functional programming language, which means that functions are first-class citizens, and programs are built using a functional programming paradigm. It supports concepts such as immutability, higher-order functions, and referential transparency.
- Strong Static Typing:
- Haskell features a strong and static type system. Type inference is used to deduce types, and the type system helps catch errors at compile time, ensuring greater program correctness.
- Lazy Evaluation:
- Haskell applies lazy evaluation, which means that expressions are not evaluated until their values are actually needed. This can lead to more efficient use of resources and enables the creation of potentially infinite data structures.
- Purely Functional:
- Haskell promotes a purely functional approach, where functions do not have side effects. This purity facilitates reasoning about programs and makes it easier to understand and test code.
- Type Classes:
- Haskell introduces the concept of type classes, which is a powerful mechanism for overloading functions based on the types of their arguments. This allows for ad-hoc polymorphism and is a key feature in Haskell’s type system.
- Pattern Matching:
- Haskell supports pattern matching, allowing developers to destructure data and match it against patterns. Pattern matching is a concise and expressive way to handle different cases in functions.
- Monads:
- Haskell introduced monads as a way to structure programs with side effects in a purely functional way. Monads provide a way to sequence computations while maintaining referential transparency.
- Immutable Data:
- Haskell emphasizes immutability, meaning that once a data structure is created, it cannot be modified. Instead, new values are created, which contributes to the overall safety and predictability of programs.
- Higher-Order Functions:
- Haskell supports higher-order functions, allowing functions to take other functions as arguments or return functions as results. This enables the creation of more abstract and reusable code.
- Expressive Type System:
- Haskell has a rich and expressive type system that includes features such as type polymorphism, type classes, and algebraic data types. The type system facilitates strong static typing while providing flexibility and abstraction.
- Algebraic Data Types:
- Haskell supports algebraic data types, allowing developers to define complex data structures using sum types (e.g.,
Either
) and product types (e.g., tuples and records).
- Haskell supports algebraic data types, allowing developers to define complex data structures using sum types (e.g.,
- Concurrency and Parallelism:
- Haskell provides abstractions for concurrent and parallel programming. It includes features like lightweight threads and parallel list comprehensions, making it suitable for concurrent and parallel applications.
- Higher-Kinded Types:
- Haskell supports higher-kinded types, allowing the definition of generic types that abstract over other types. This contributes to the development of more generic and reusable abstractions.
- Type Inference:
- Haskell features powerful type inference, allowing developers to write code without explicitly specifying types in many cases. The type system can deduce types, providing a balance between static typing and expressive code.
What is the workflow of Haskell?
Workflow of Haskell:
- Project Initialization:
- Start by initializing a new Haskell project using tools like
stack
orcabal
.
- Start by initializing a new Haskell project using tools like
- Code Editor/IDE Setup:
- Set up a code editor or integrated development environment (IDE) with Haskell support. Popular choices include Visual Studio Code with the Haskell extension or the Haskell-specific IDE, Haskell IDE Engine.
- Write Haskell Code:
- Write Haskell code in source files with a
.hs
extension. Define functions, data types, and modules using Haskell syntax.
- Write Haskell code in source files with a
- Type Check and Compile:
- Use the GHC (Glasgow Haskell Compiler) or another Haskell compiler to type-check and compile the Haskell code. The compiler ensures that the code adheres to the type system and produces executable binaries.
- Test and Debug:
- Write unit tests using frameworks like HUnit or QuickCheck. Use GHCi (GHC Interactive) for interactive development, testing, and debugging. The REPL (Read-Eval-Print Loop) in GHCi allows developers to experiment with code snippets.
- Package Management:
- Manage project dependencies and versions using tools like
stack
orcabal
. Declare dependencies in the project configuration file (stack.yaml
orcabal.project
) and use commands to fetch and build dependencies.
- Manage project dependencies and versions using tools like
- Build and Run:
- Build the Haskell project using the chosen build tool (
stack build
orcabal build
). Run the executable to test the application.
- Build the Haskell project using the chosen build tool (
- Documentation:
- Generate documentation for the Haskell code using tools like Haddock. Include comments and annotations in the code to enhance documentation generation.
- Version Control:
- Apply version control systems like Git to track changes in the codebase. Commit changes, generate branches, and collaborate with team members using established version control practices.
- Continuous Integration (CI):
- Set up continuous integration for the Haskell project. CI tools like Travis CI or GitHub Actions can be configured to automatically build, test, and verify the project on every commit.
- Deployment:
- Deploy the Haskell application if applicable. Depending on the nature of the project, deployment might involve packaging the application, configuring servers, and ensuring proper runtime environments.
- Maintenance and Updates:
- Monitor the project for issues and user feedback. Address bug reports, implement new features, and update dependencies as needed. Follow best practices for software maintenance.
The Haskell workflow involves an iterative process of writing code, testing, building, and deploying. The strong emphasis on correctness and the availability of tools like GHCi contribute to a development process that encourages exploration and experimentation.
How Haskell Works & Architecture?
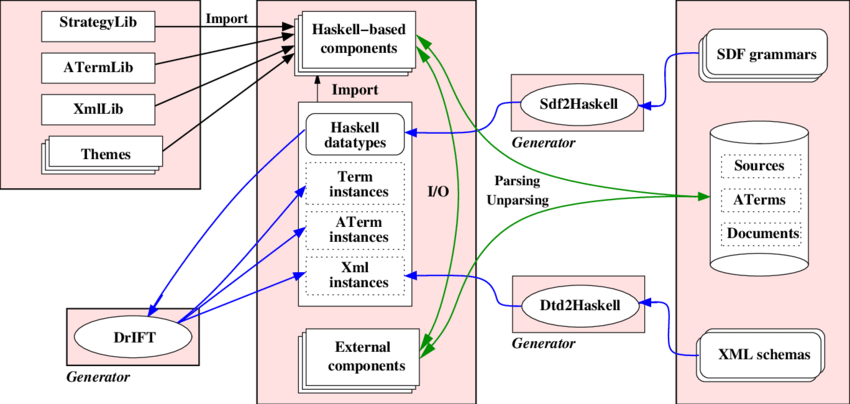
Haskell is a powerful and expressive functional programming language known for its purity, laziness, and strong type system. Let’s dive into its workings and architecture:
Key Principles:
- Purity: Functions have no side effects and always return the same output for the same input. This simplifies reasoning about code and makes it easier to test and debug.
- Laziness: Values are only evaluated when needed, leading to efficient memory usage and potentially simpler code.
- Strong Type System: Types are statically enforced, catching errors early and improving code reliability.
- Pattern Matching: Powerful tool for deconstructing data structures and handling different cases efficiently.
- Immutability: Data is immutable by default, promoting referential transparency and simplifying reasoning about state.
Architecture:
- Compiler: The Glasgow Haskell Compiler (GHC) is the primary compiler for Haskell. It translates Haskell code into efficient machine code.
- Core Language: A small, minimal core language defines the fundamental semantics of Haskell.
- Intermediate Representations: The compiler translates Haskell code through various intermediate representations, like Core and STG, before generating machine code.
- Libraries: A rich ecosystem of open-source libraries provides functionality for various tasks, like data structures, algorithms, and web development.
- Packages: Code is organized into packages for modularity and reuse.
Benefits:
- Expressive and concise: Haskell allows for concise and elegant code solutions compared to imperative languages.
- Safe and reliable: Strong type system and purity prevent many common errors, leading to reliable and predictable code.
- Modular and reusable: Libraries and packages promote code reuse and modularity, making large projects manageable.
- Parallelism potential: Functional nature makes Haskell suitable for parallel programming and efficient utilization of multiple cores.
Use Cases:
- Web development: Frameworks like Yesod and Scotty provide tools for building high-performance web applications.
- Data analysis and scientific computing: Libraries like Hask and Data.Array enable efficient data manipulation and scientific calculations.
- Financial modeling and analysis: Haskell’s precision and type safety make it suitable for financial modeling and analysis tasks.
- System programming: Libraries like Haskos and Helium allow building low-level systems and drivers.
Notes:
- Haskell has a learning curve, but its power and expressiveness make it rewarding to learn.
- Don’t hesitate to ask for help from the vast online community and resources available.
- Embrace experimentation and explore different aspects of Haskell to unlock its full potential!
How to Install and Configure Haskell?
Here’s a guide on how to install and configure Haskell:
1. Download the Haskell Platform:
- Visit the official Haskell website.
- Select the appropriate installer for your operating system (Windows, macOS, Linux).
- Download and run the installer. It will include the GHC compiler, libraries, and tools.
2. Set Environment Variables (if necessary):
- Windows: Add
C:\Program Files\Haskell Platform\<version>\bin
(or your installation path) to the PATH environment variable. - macOS/Linux: Usually, the installer sets up environment variables automatically. If not, add the path to GHC’s
bin
directory to your shell configuration file (e.g.,.bashrc
).
3. Verify Installation:
- Open a terminal or command prompt and type
ghci
. If installed correctly, you’ll enter the GHC interactive prompt. - Type
:quit
to exit GHCi.
4. Install Additional Packages (optional):
- Use the
cabal
package manager to install additional libraries or tools:- Open a terminal and type
cabal update
to refresh package list. - Install a package using
cabal install <package-name>
.
- Open a terminal and type
5. Configure Editor or IDE (optional):
- If using a code editor or IDE, set it up for Haskell development:
- Install Haskell syntax highlighting and code completion plugins.
- Configure build tools for Haskell projects.
Key Points:
- Multiple installations: Manage different Haskell versions using tools like
stack
orghcup
. - Updating Haskell: Download and install a newer version of the Haskell Platform.
I’m ready to assist further with any specific configuration questions or tasks you have within Haskell!
Fundamental Tutorials of Haskell: Getting started Step by Step
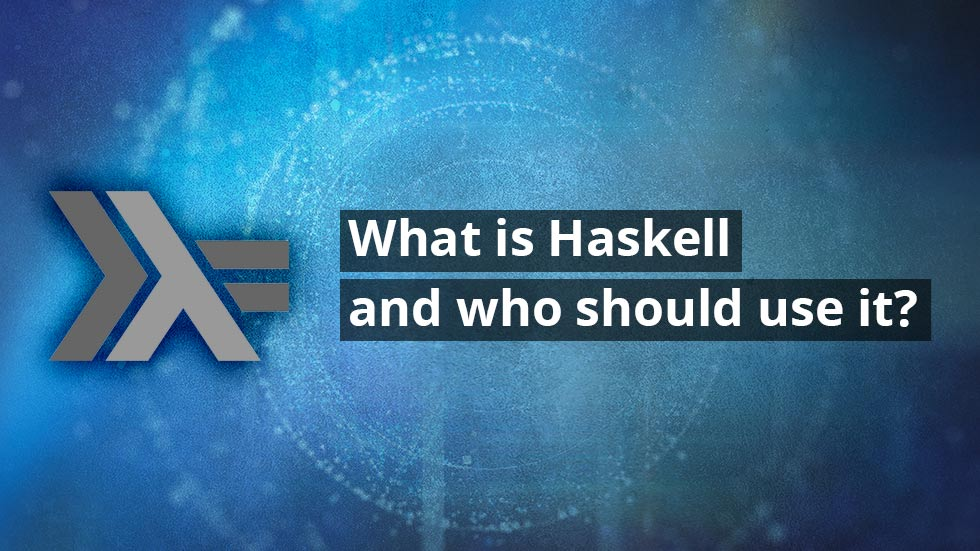
I’m thrilled you’re excited to dive into Haskell! Since you’re open to suggestions, let’s start with a classic beginner project: writing your first Haskell program! This will introduce you to fundamental concepts like code syntax, function definition, and working with data types.
Step 1: Open GHCi:
- Launch GHCi, the interactive Haskell environment. You should see a prompt like
Prelude>
.
Step 2: Define a Simple Function:
- Type the following code and press Enter:
Haskell
greet name = "Hello, " ++ name ++ "!"
This defines a function named greet
that takes a name as input (a string) and returns a greeting message.
Step 3: Use the Function:
- In GHCi, type the following:
Haskell
greet "Haskell"
Step 4: See the Result:
- You should see “Hello, Haskell!” printed in the output.
Congratulations! You’ve written and executed your first Haskell program!
Here’s what you learned:
- Function definition:
greet name = ...
defines a function namedgreet
with parametername
. - String concatenation:
++
joins two strings together. - Data types:
String
is a built-in data type for character sequences. - Evaluating code: Typing expressions in GHCi evaluates them immediately.
Next Steps:
- Experiment with different names in the
greet
function. - Modify the message returned by
greet
. - Try out other built-in functions like
length
to find the string length. - Explore different data types like
Integer
andBool
.
I’m here to support you every step of the way! Let’s unlock the power of functional programming together!
- Mutual of Omaha: Selection and Interview process, Questions/Answers - April 15, 2024
- AES: Selection and Interview process, Questions/Answers - April 15, 2024
- Amphenol: Selection and Interview process, Questions/Answers - April 15, 2024