What is Hibernate?
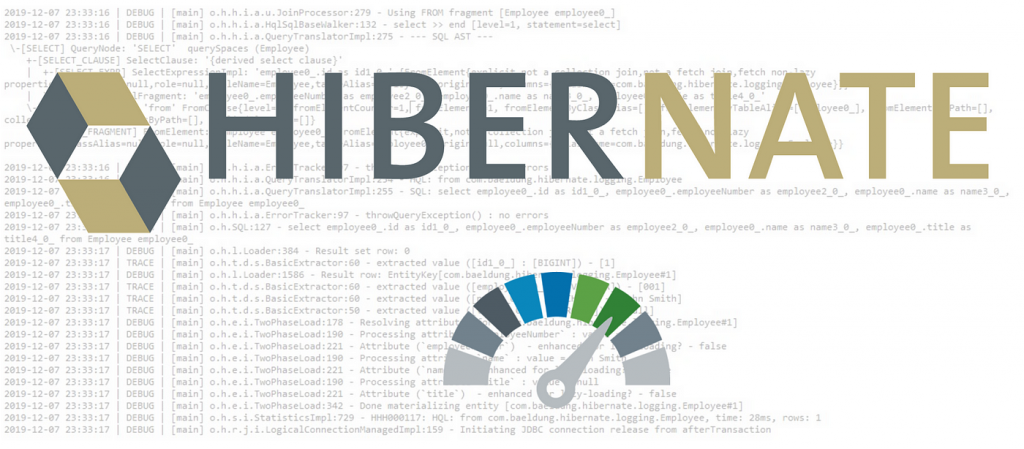
Hibernate is an open-source framework for Java that provides an object-relational mapping (ORM) solution. It simplifies the interaction between a Java application and a relational database by mapping Java objects to database tables and vice versa. Hibernate handles the translation of Java objects’ state to and from the corresponding database tables, abstracting away the complexities of SQL queries and database interactions.
Key features of Hibernate include:
- Object-Relational Mapping (ORM): Hibernate allows developers to work with Java objects and their relationships, and it automatically generates the corresponding SQL queries to interact with the database.
- Database Independence: Hibernate provides a database-independent layer, allowing developers to write database-agnostic code. This means that you can switch from one database system to another without changing the application code.
- Automatic Table Generation: Hibernate can automatically generate database tables based on the Java objects (entities) in your application. This feature simplifies database schema management.
- Caching: Hibernate includes a caching mechanism that can significantly improve performance by caching frequently accessed data in memory, reducing the need for repeated database queries.
- Transaction Management: Hibernate supports transaction management, ensuring data consistency and integrity by allowing developers to define transactional boundaries in their code.
- Query Language: Hibernate Query Language (HQL) is a powerful and flexible query language that is similar to SQL but operates on Java objects. It allows developers to write queries using object-oriented concepts.
What is top use cases of Hibernate?
Top use cases of Hibernate include:
- Enterprise Applications: Hibernate is widely used in enterprise-level applications where data persistence is a critical aspect. It simplifies the development of applications by providing a high-level, object-oriented interface to interact with databases.
- Web Applications: Hibernate is commonly used in web applications, especially those built using Java-based frameworks like Spring. It allows developers to focus on business logic and application functionality rather than dealing with low-level database interactions.
- Data-Driven Applications: Applications that heavily rely on data storage and retrieval, such as content management systems, customer relationship management (CRM) systems, and e-commerce platforms, benefit from Hibernate’s ORM capabilities.
- Microservices Architecture: In microservices-based architectures, where each service may have its own database, Hibernate helps manage data persistence for individual services while abstracting the underlying database technology.
- Prototyping and Rapid Development: Hibernate can be beneficial in projects that require rapid development and prototyping, as it abstracts away many of the complexities of database interaction, allowing developers to focus on building features.
Overall, Hibernate is a powerful tool for Java developers to simplify database-related tasks and improve the efficiency of data-driven applications.
What are feature of Hibernate?
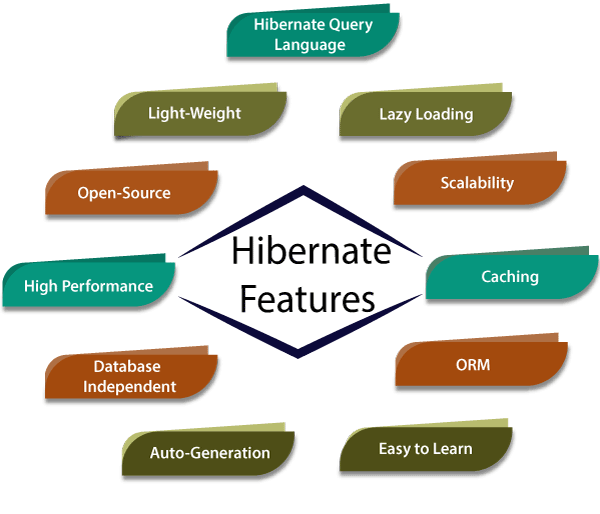
Hibernate comes with a set of features that make it a popular choice for Java developers working with databases. Here are some key features of Hibernate:
- Object-Relational Mapping (ORM): Hibernate provides a way to map Java objects to database tables and vice versa, allowing developers to work with objects in their code and have the framework handle the translation to and from the database.
- Database Independence: Hibernate abstracts away the differences between various database systems, allowing developers to write database-agnostic code. This means you can switch from one database to another without changing the application code.
- Automatic Table Generation: Hibernate can automatically generate database tables based on the Java objects (entities) in your application, reducing the need for manual database schema management.
- Caching: Hibernate includes a caching mechanism that helps improve performance by storing frequently accessed data in memory, reducing the need for repeated database queries.
- Lazy Loading: Hibernate supports lazy loading, a feature that loads data from the database only when it is explicitly requested. This can help optimize resource usage and increase performance.
- Transaction Management: Hibernate supports transactions, ensuring that operations on the database either complete successfully or leave the database in a consistent state. Developers can define transactional boundaries in their code.
- Query Language: Hibernate Query Language (HQL) is a powerful and flexible query language similar to SQL, but it operates on Java objects. It allows developers to write queries using object-oriented concepts, making it easier to work with complex data models.
- Annotations and XML Configuration: Hibernate supports both annotations and XML configuration for mapping Java objects to database tables, giving developers flexibility in choosing their preferred approach.
What is the workflow of Hibernate?
Now, let’s briefly outline the workflow of Hibernate:
- Entity Classes: Define Java classes that represent entities (objects) in your application. These classes are annotated with Hibernate annotations or configured using XML to specify how they map to database tables.
- SessionFactory: Configure and build a
SessionFactory
, which is a thread-safe and immutable representation of the database connection. It is typically generated once during application startup. - Session: Obtain a
Session
from theSessionFactory
whenever you need to interact with the database. TheSession
serves as the primary interface for database operations in Hibernate. - Transaction: Begin a transaction using the
Session
. This is pivotal for ensuring data consistency and integrity. Hibernate supports both programmatic and declarative transaction management. - CRUD Operations: Use the
Session
to perform CRUD (Create, Read, Update, Delete) operations on your entities. Hibernate automatically generates and executes the corresponding SQL queries. - Query Language: Optionally, use HQL or Criteria API to perform more complex queries. HQL allows you to express queries using object-oriented concepts rather than SQL.
- Caching: Leverage Hibernate’s caching mechanisms to improve performance by reducing the number of database queries.
- Commit or Rollback: After completing your database operations, commit the transaction to persist changes or rollback to undo any changes made during the transaction.
- Close Session: Close the
Session
when it is no longer needed. Sessions are typically short-lived and are opened and closed as needed.
By following this workflow, developers can leverage Hibernate to handle the details of database interactions, allowing them to focus on writing business logic and building robust applications.
How Hibernate Works & Architecture?
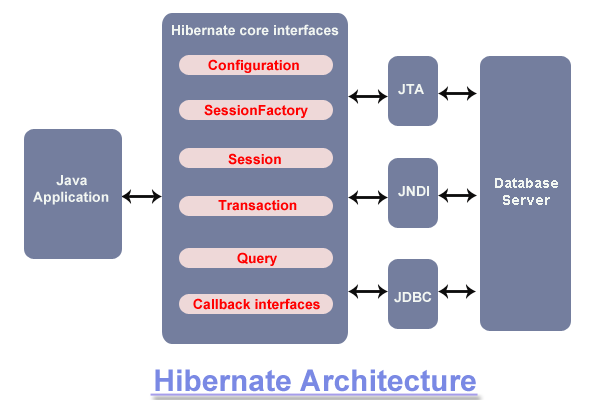
Hibernate is a powerful object-relational persistence (ORM) framework for Java applications. It bridges the gap between object-oriented programming (OOP) and relational databases, allowing you to work with objects directly instead of writing raw SQL queries. Here’s an overview of how Hibernate works and its architecture:
How it works:
- Mapping: You define mappings between your Java classes and database tables using annotations or XML configuration files. This tells Hibernate how to persist and retrieve your objects from the database.
- Sessions: A session acts as a unit of work with the database. It manages connections, queries, and object-database synchronization.
- Persistence: When you save an object in your application, Hibernate translates it into appropriate SQL statements and inserts it into the corresponding database table.
- Retrieval: When you retrieve an object by its identifier, Hibernate fetches the data from the database and builds the corresponding object in memory.
- Caching: Hibernate uses a built-in cache to store recently accessed objects, improving performance by reducing database roundtrips.
- Transactions: You can define transactions for managing database updates with atomicity and consistency. If any part of the transaction fails, the whole operation is rolled back.
Architecture:
Hibernate has a layered architecture consisting of several key components:
- Core API: Provides basic functionalities for object-database mapping, querying, and transaction management.
- Session Factory: Creates and manages sessions, connecting to the database and providing access to other Hibernate components.
- Connection Provider: Manages the underlying database connections and handles JDBC interactions.
- Transaction API: Defines interfaces for managing transactions and ensuring data consistency.
- Query API: Provides different mechanisms for building and executing database queries.
- Caching: Manages different levels of caching to improve performance and optimize database interactions.
- Mapping Metamodel: Represents the metadata about your object-database mappings, enabling introspection and dynamic query generation.
Benefits of using Hibernate:
- Reduced development time: By eliminating the need for writing manual SQL queries, Hibernate simplifies data access and increases developer productivity.
- Improved code maintainability: Object-oriented code is easier to understand and maintain compared to complex SQL manipulations.
- Database portability: Hibernate applications can be easily ported to different databases with minimal code changes thanks to its abstraction layer.
- Increased performance: Caching and other optimization techniques in Hibernate can lead to significant performance improvements compared to direct SQL access.
I hope this explanation provides a clear understanding of how Hibernate works and its architecture. If you have any further questions or want to delve deeper into specific aspects, feel free to ask!
How to Install and Configure Hibernate?
Here’s, I have assembled stepwise guide to installing and configuring Hibernate in your Java project:
1. Prerequisites:
- Java Development Kit (JDK): Ensure you have JDK 8 or later installed.
- Build tool (optional): While not strictly required, using a build tool like Maven or Gradle simplifies dependency management.
- Database: Choose a relational database supported by Hibernate (e.g., MySQL, PostgreSQL, Oracle, SQL Server).
2. Download Hibernate:
- Maven: Add the following dependency to your
pom.xml
:
XML
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-core</artifactId>
<version>5.6.9.Final</version>
</dependency>
- Gradle: Add the dependency to your
build.gradle
:
Gradle
implementation 'org.hibernate:hibernate-core:5.6.9.Final'
- Manual download: Download the Hibernate JAR files from the official website.
3. Add database driver:
- Include the JDBC driver for your chosen database in your project’s classpath.
- Maven example:
XML
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.29</version>
</dependency>
4. Create configuration file:
- Create a
hibernate.cfg.xml
file to specify database connection details and Hibernate properties:
XML
<!DOCTYPE hibernate-configuration PUBLIC
“-//Hibernate/Hibernate Configuration DTD 3.0//EN”
“http://www.hibernate.org/dtd/hibernate-configuration-3.0.dtd”>
<hibernate-configuration>
<session-factory>
<property name=”hibernate.connection.driver_class”>com.mysql.cj.jdbc.Driver</property>
<property name=”hibernate.connection.url”>jdbc:mysql://localhost:3306/your_database_name</property>
<property name=”hibernate.connection.username”>your_username</property>
<property name=”hibernate.connection.password”>your_password</property>
<property name=”hibernate.dialect”>org.hibernate.dialect.MySQLDialect</property>
</session-factory>
</hibernate-configuration>
5. Create mappings:
- Define mappings between your Java classes and database tables using annotations or XML:
Java
@Entity
@Table(name = "users")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(name = "name")
private String name;
// ... getters and setters
}
6. Use Hibernate in your code:
- Obtain a
SessionFactory
instance:
Java
SessionFactory sessionFactory = new Configuration().configure().buildSessionFactory();
- Create
Session
objects for database interactions:
Java
Session session = sessionFactory.openSession();
- Perform CRUD operations using Hibernate’s API:
Java
// Save an object
session.save(user);
// Retrieve an object
User retrievedUser = session.get(User.class, 1L);
// ... other operations
Important tips:
- Consult Hibernate documentation for detailed configuration options and advanced features.
- Consider using a dependency injection framework to manage Hibernate sessions and transactions.
- Utilize Hibernate’s caching mechanisms for performance optimization.
- Properly handle transactions to ensure data consistency.
Fundamental Tutorials of Hibernate: Getting started Step by Step
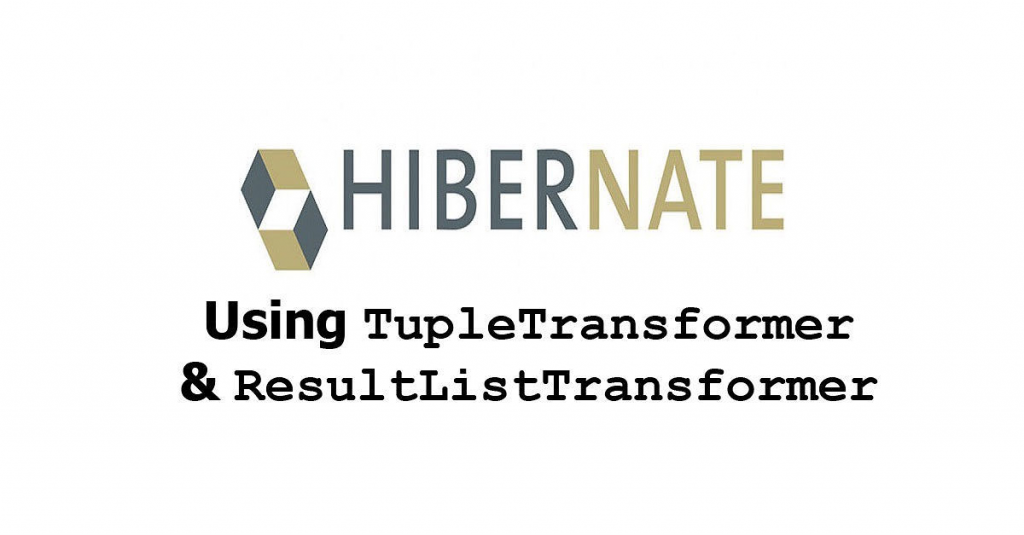
To create the perfect step-by-step fundamentals tutorials for Hibernate, I need a bit more information about your learning goals and preferred style. Please tell me:
- Your experience with Java: Are you a beginner, intermediate, or experienced Java developer?
- Your familiarity with databases: Do you have basic understanding of relational databases and SQL queries?
- What you want to achieve with Hibernate: Are you interested in basic data persistence, querying, or specific features like relationships and transactions?
- Your preferred learning style: Do you prefer written tutorials with code examples, video lectures, interactive exercises, or a combination?
Once I have this information, I can tailor the tutorials to your specific needs and ensure they provide a smooth learning experience.
In the meantime, here are some general steps for getting started with fundamental Hibernate tutorials:
Step 1: Set Up Your Development Environment:
- Install JDK 8 or later and a text editor/IDE like Eclipse or IntelliJ IDEA.
- Download and configure your database driver (e.g., MySQL Connector/J).
- Choose a build tool (optional): Maven or Gradle can simplify dependency management.
Step 2: Download and Install Hibernate:
- Include the Hibernate core JAR file in your project’s classpath.
- If using a build tool, add the appropriate dependency (e.g.,
org.hibernate:hibernate-core
in Maven).
Step 3: Create a Basic Entity Class:
- Define a simple Java class representing your data (e.g., User with attributes like name and email).
- Use annotations like
@Entity
and@Id
to mark the class and primary key.
Step 4: Configure Hibernate Session Factory:
- Create a
hibernate.cfg.xml
file to specify database connection details and Hibernate properties. - Include elements like
session-factory
,property
(for connection details and dialect), andmapping
(if using XML for entity mappings).
Step 5: Perform Basic CRUD Operations:
- Obtain a
SessionFactory
instance from the configuration. - Create a
Session
object for interacting with the database. - Use Hibernate’s API to save, retrieve, update, and delete objects of your entity class.
Step 6: Explore Additional Features:
- Learn about relationships (one-to-one, one-to-many, etc.) and how to map them in Hibernate.
- Understand transactions and how to manage data consistency in your application.
- Experiment with Hibernate queries (HQL) for more complex data retrieval.
Remember, practice is key! Don’t hesitate to experiment with the fundamentals and explore different tutorials to solidify your understanding. I’m here to help you take your first steps with Hibernate and answer any questions you might have along the way.
- Mutual of Omaha: Selection and Interview process, Questions/Answers - April 15, 2024
- AES: Selection and Interview process, Questions/Answers - April 15, 2024
- Amphenol: Selection and Interview process, Questions/Answers - April 15, 2024