What is Jquery?
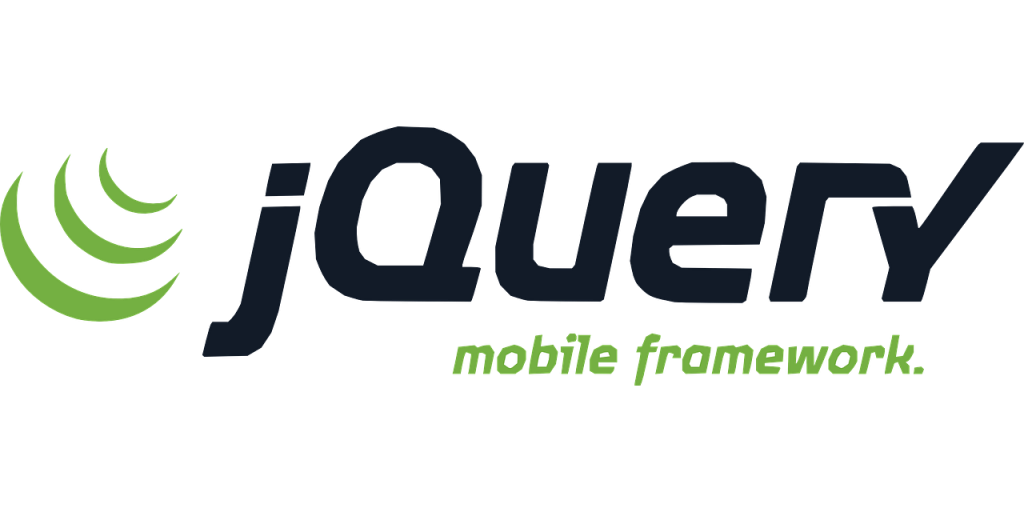
Jquery is a fast and concise JavaScript library that simplifies HTML document traversing, event handling, animating, and Ajax interactions for rapid web development. It is a cross-platform software that is compatible with major web browsers like Chrome, Firefox, Safari, and Internet Explorer. Jquery was created by John Resig in January 2006 and is currently maintained by the Jquery Foundation.
What is the top use cases of Jquery?
Jquery is widely used in web development for creating dynamic and responsive web pages. Some of the top use cases of Jquery are:
- DOM Manipulation: Jquery makes it easy to manipulate the Document Object Model (DOM) of a web page. You can add or remove elements, change their attributes, and modify their content using simple and concise syntax.
- Event Handling: Jquery simplifies the process of handling user events like clicks, mouseovers, and keypresses. You can attach event listeners to DOM elements and execute custom functions when the events occur.
- Ajax Interactions: Jquery provides a powerful and flexible API for making asynchronous HTTP requests and handling server responses. You can load data from remote servers, submit forms, and update web pages without reloading the entire page.
- Animations and Effects: Jquery offers a wide range of built-in animations and effects that can be applied to DOM elements. You can create sliding panels, fading images, and other visual effects with just a few lines of code.
What are the features of Jquery?
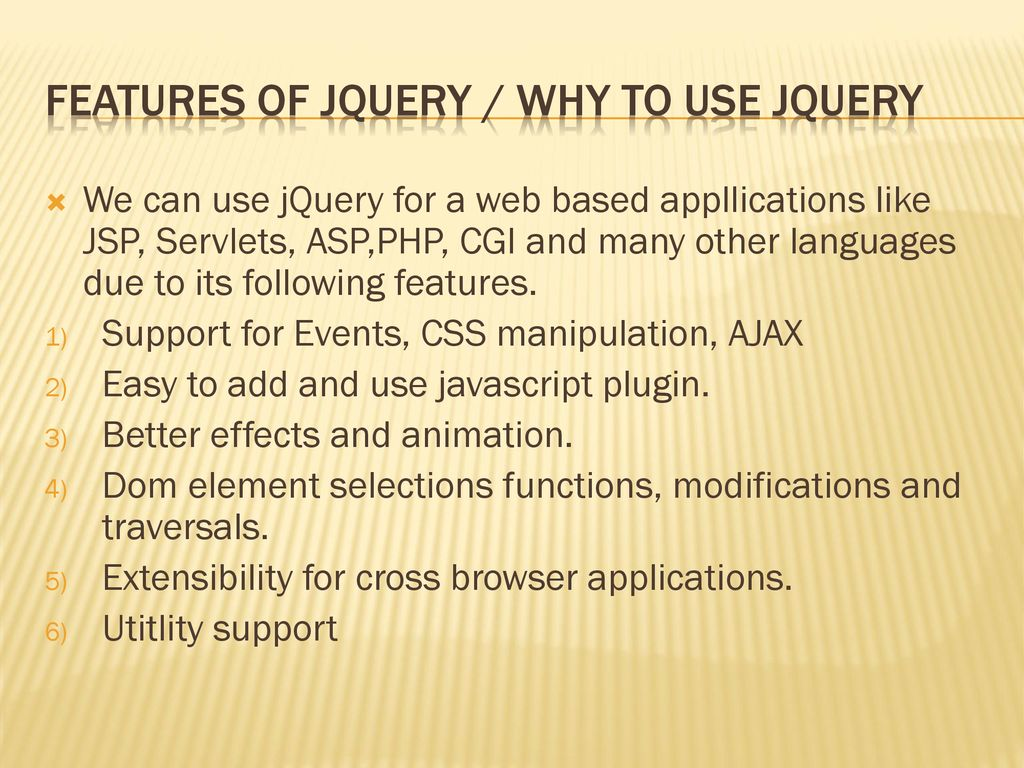
Jquery has a rich set of features that make it a popular choice for web development. Some of the key features of Jquery are:
- CSS Selectors: Jquery uses CSS selectors to identify and manipulate DOM elements. This makes it easy to target specific elements based on their class, ID, or tag name.
- Method Chaining: Jquery allows you to chain multiple methods together in a single statement. This reduces the amount of code you need to write and makes your code more readable.
- Cross-Browser Compatibility: Jquery is designed to work seamlessly across different web browsers. It uses feature detection to determine the capabilities of the browser and adapts its behavior accordingly.
- Plugin Architecture: Jquery has a modular architecture that allows you to extend its functionality with third-party plugins. There are thousands of plugins available for Jquery that can add new features and enhance its performance.
What is the workflow of Jquery?
The workflow of Jquery typically involves the following steps:
- Include Jquery Library: The first step is to include the Jquery library in your HTML document. You can either download the library from the Jquery website or use a CDN to load it from a remote server.
- Select DOM Elements: Once the library is loaded, you can use CSS selectors to select the DOM elements you want to manipulate. For example, you can select all the paragraphs in a web page using the following code:
$('p')
- Apply Methods: After selecting the DOM elements, you can apply Jquery methods to manipulate them. For example, you can change the text content of all the paragraphs using the following code:
$('p').text('Hello, World!')
- Handle Events: You can also attach event listeners to DOM elements using Jquery. For example, you can execute a function when a button is clicked using the following code:
$('button').click(function() {
alert('Button clicked!');
});
How Jquery Works & Architecture?
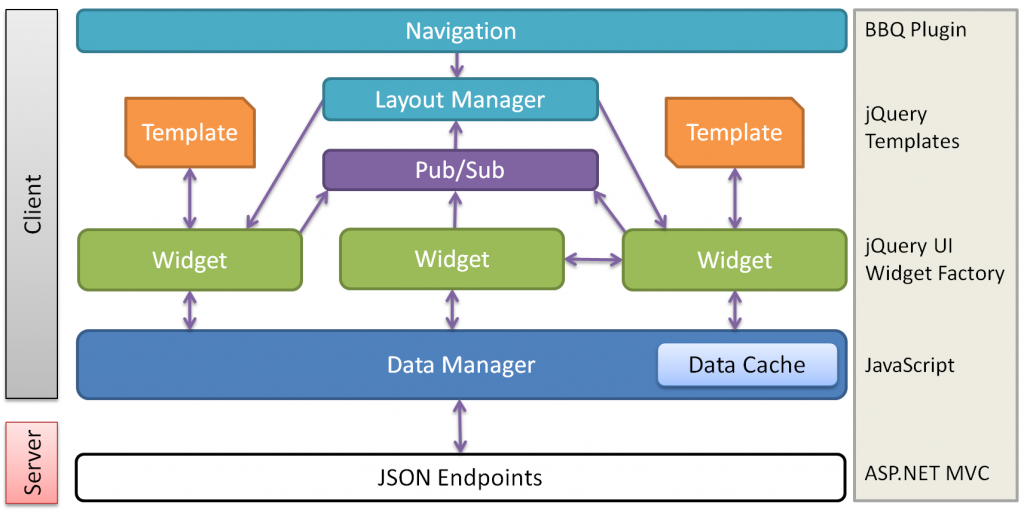
Jquery works by providing a set of functions and methods that simplify common web development tasks like DOM manipulation, event handling, and Ajax interactions. It uses a modular architecture that allows you to use only the features you need and extend its functionality with third-party plugins.
Jquery is built on top of the JavaScript programming language and uses a combination of native JavaScript functions and custom Jquery functions to achieve its functionality. It also uses the Document Object Model (DOM) of the web browser to manipulate HTML elements.
How to Install and Configure Jquery?
To install Jquery, you can either download the library from the Jquery website or use a CDN to load it from a remote server. To configure Jquery, you need to include the library in your HTML document using a script tag. Here’s an example:
<!DOCTYPE html>
<html>
<head>
<title>My Page</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<p>Hello, World!</p>
<button>Click me</button>
<script>
// Jquery code goes here
</script>
</body>
</html>
Fundamental Tutorials of Jquery: Getting Started Step by Step
Getting Started with jQuery
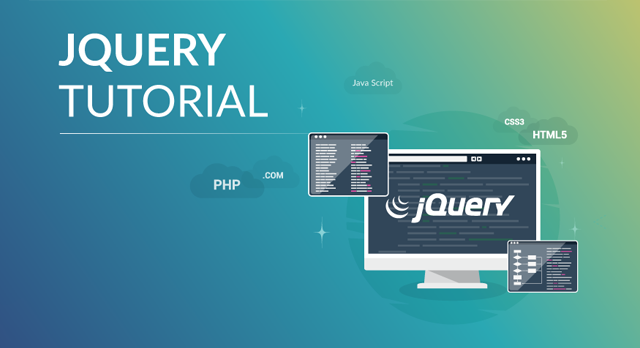
Before we dive into the tutorials, let’s first understand how to get started with jQuery. You can include jQuery in your HTML document by either downloading it from the website or including it from a CDN (Content Delivery Network). Here’s an example of how to include jQuery from a CDN:
<head>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
Once you have included jQuery in your HTML document, you can start using jQuery functions.
Selectors
In jQuery, selectors are used to select HTML elements based on their name, id, class, attributes, and values. Here are some examples of selectors:
$("p")
selects all<p>
elements on the page.$("#myDiv")
selects the element withid="myDiv"
.$(".myClass")
selects all elements withclass="myClass"
.$("input[type='text']")
selects all<input>
elements withtype="text"
.
Events
In jQuery, events are actions or occurrences that happen on a webpage. Examples of events include clicking a button, hovering over an element, and scrolling down a page. Here are some examples of events in jQuery:
$("button").click(function() {
alert("Button clicked!");
});
$("p").hover(function() {
$(this).css("background-color", "yellow");
}, function() {
$(this).css("background-color", "white");
});
Effects
In jQuery, effects are used to create animations and transitions on HTML elements. Examples of effects include fading in/out, sliding up/down, and animating properties. Here are some examples of effects in jQuery:
$("#myDiv").fadeIn();
$("#myDiv").slideUp();
$("#myDiv").animate({
opacity: 0.5,
left: "+=50",
height: "toggle"
}, 2000);
AJAX
In jQuery, AJAX (Asynchronous JavaScript and XML) is used to send and receive data asynchronously from a server without reloading the entire webpage. Here’s an example of how to use AJAX with jQuery:
$.ajax({
url: "myServerScript.php",
type: "POST",
data: { name: "John", age: 30 },
success: function(result) {
alert("Data sent successfully!");
},
error: function(xhr, status, error) {
alert("Error: " + error);
}
});
Conclusion
Congratulations! You have learned the fundamental tutorials of jQuery step by step. You can now use jQuery to simplify your HTML document manipulation, event handling, and animation for rapid web development. Keep practicing and exploring more jQuery functions to enhance your web development skills. Happy coding!
- Degree Pursuit: Navigating the Path to Educational Excellence - July 4, 2024
- Why Is Studying English Important in a Business Environment? - July 4, 2024
- Top 10 Data Science Skills You Need in 2024 - July 3, 2024