What is JSON?
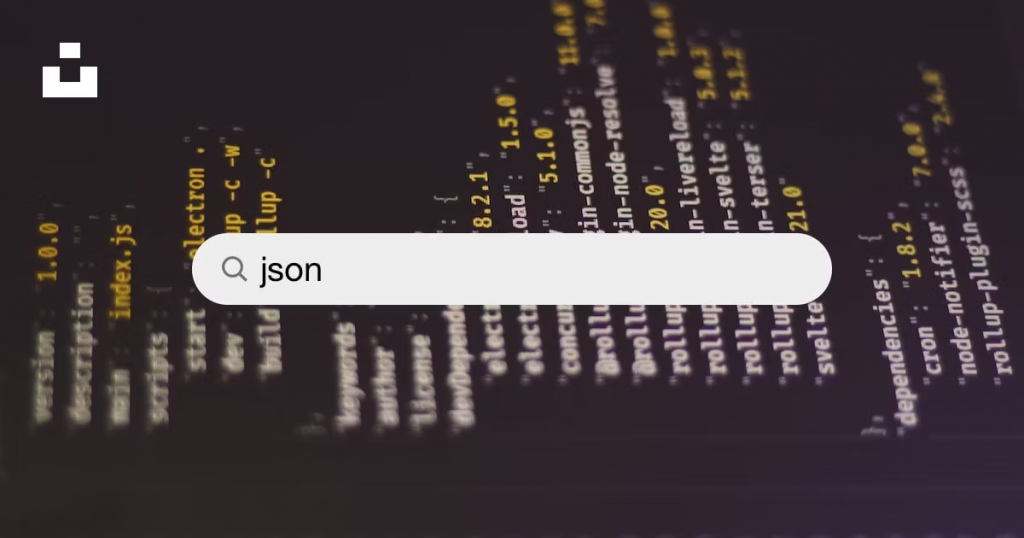
JSON is a widely used data-interchange format that offers simplicity, flexibility, and compatibility across different systems and programming languages. Understanding JSON is essential for any developer working with web applications. It is a text-based format that represents structured data in a human-readable and machine-readable way. JSON is widely used for data exchange between a server and a web application, as well as for configuration files, APIs, and more.
Key characteristics of JSON:
- Simple Syntax: JSON uses a straightforward and easy-to-understand syntax, consisting of key-value pairs, arrays, and data types like strings, numbers, booleans, and null.
- Data Types: JSON supports various data types, including strings (enclosed in double quotes), numbers, booleans (true or false), null (representing the absence of a value), objects (collections of key-value pairs), and arrays (ordered lists of values).
- Hierarchical Structure: JSON allows for the nesting of objects and arrays, providing a hierarchical structure that can represent complex data relationships.
- Human-Readable: JSON is designed to be readable by both humans and machines. This makes it useful for configuration files and settings.
- Language Independence: JSON is not tied to any specific programming language, making it a versatile format for data interchange between different platforms and languages.
What is top use cases of JSON?
- Web APIs: JSON is a popular format for defining the structure of data exchanged between a web server and a client application. Many web APIs use JSON as their data interchange format because of its simplicity and ease of use.
- Configuration Files: JSON is often used for configuration files in software applications. Developers can define application settings, preferences, and parameters in JSON format, which can be easily read and modified.
- Data Storage: JSON is used for storing and retrieving structured data in databases or NoSQL data stores. It allows for the flexible representation of data and can be easily mapped to objects in programming languages.
- Serialization and Deserialization: JSON is used to serialize complex data structures in programming languages into a format that can be easily stored or transmitted. Deserialization is the reverse process of reconstructing the data from JSON.
- Logging and Monitoring: JSON is used in log files and monitoring data to record events, errors, and performance metrics in a structured and machine-readable format. This makes it easier to analyze and troubleshoot issues.
- Interchange Formats: JSON is commonly used as an interchange format for data exchange between systems or applications. It is often used in data pipelines, ETL (Extract, Transform, Load) processes, and data integration.
- Client-Server Communication: JSON is employed in client-server communication in web development. It allows servers to send structured data to web clients, which can then parse and display the data.
- Configuration Management: JSON is used in configuration management tools like Ansible and Terraform to define infrastructure configurations, policies, and provisioning instructions.
- Mobile App Development: JSON is used in mobile app development for exchanging data between the mobile app and the server, often via RESTful APIs.
- Data Interchange in IoT: JSON is used for data exchange in the Internet of Things (IoT) ecosystem, enabling devices and sensors to send and receive structured data.
- NoSQL Databases: Some NoSQL databases, such as MongoDB and CouchDB, use JSON-like document formats to store and query data.
- Web Configuration and Routing: JSON is used in web development for defining configuration settings, routing rules, and data structures in web applications.
JSON is a versatile and widely adopted data interchange format that is used in a variety of contexts, including web development, configuration management, data storage, and more. Its simplicity and compatibility with multiple programming languages make it a popular choice for exchanging structured data in modern software applications.
What are the features of JSON?
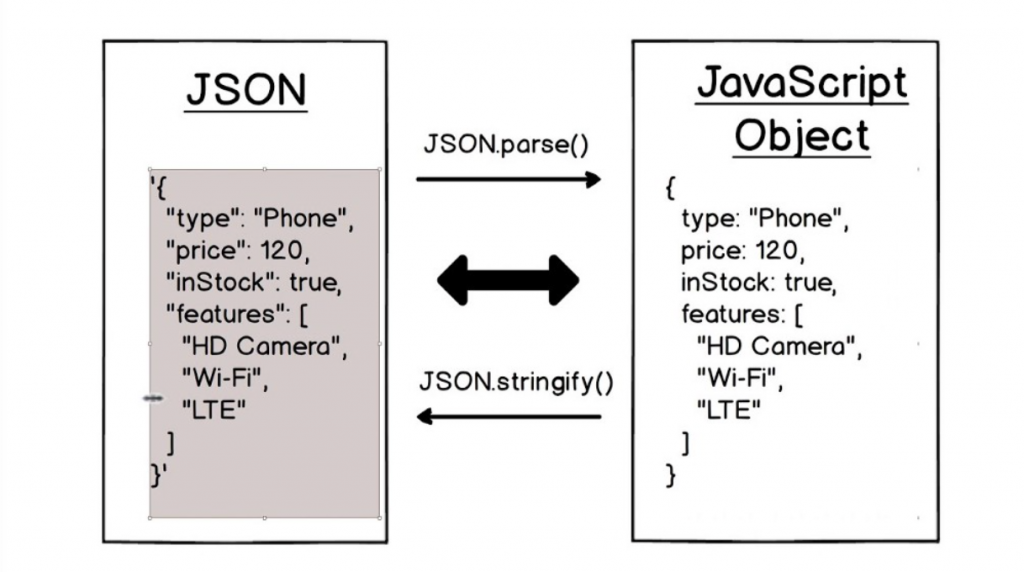
JSON (JavaScript Object Notation) is a simple and lightweight data interchange format with several key features that make it popular for representing structured data. Below are the main features of JSON:
- Text-Based Format: JSON is a text-based format, which means that data is represented as human-readable and writable text. This format is platform-independent and can be easily understood by both humans and machines.
- Data Types: JSON supports several data types, including:
- Strings: Enclosed in double quotation marks (e.g., “Hello, World”).
- Numbers: Integers or floating-point values (e.g., 42 or 3.14).
- Booleans: True or false.
- Null: Represents the absence of a value.
- Objects: Collections of key-value pairs (e.g., {“name”: “John”, “age”: 30}).
- Arrays: Ordered lists of values (e.g., [1, 2, 3]).
- Hierarchical Structure: JSON allows for the nesting of objects and arrays, creating a hierarchical structure that can represent complex data relationships. Objects can contain other objects or arrays as values, enabling the representation of multi-level data structures.
- Key-Value Pairs: JSON data is organized into key-value pairs, where keys are strings enclosed in double quotation marks, followed by a colon, and then a value. Multiple key-value pairs in an object are separated by commas.
- Order Preservation: JSON maintains the order of elements within arrays, making it suitable for data where element order matters.
- Language Independence: JSON is not tied to any specific programming language, making it a versatile format for data interchange between different platforms and languages. JSON data can be processed by virtually any programming language that has JSON parsing libraries or modules.
- Ease of Parsing and Generation: JSON is easy for machines to parse and generate because of its simple and predictable syntax. This makes it efficient for data serialization and deserialization.
- Compact and Lightweight: JSON is a compact format that minimizes unnecessary whitespace, making it efficient for data transmission and storage.
What is the workflow of JSON?
- Data Creation: JSON data is created by defining objects, arrays, and their respective key-value pairs and values. This data can be generated by software applications, configuration files, or by manual entry.
- Serialization: When it’s necessary to transmit or store data, it is serialized into a JSON string. Serialization is the process of converting data structures, such as objects or arrays, into a JSON format. Most programming languages provide libraries or functions for this purpose.
- Data Transmission: Serialized JSON data can be transmitted over networks, stored in files, or sent between different components of a software system. This is often seen in web applications when data is exchanged between a server and a client.
- Deserialization: Upon receiving JSON data, it needs to be deserialized to reconstruct the original data structures. Deserialization is the process of converting a JSON string back into native data structures in a programming language.
- Data Processing: Once the data is deserialized, it can be processed, queried, and manipulated as needed within the application. For example, in a web application, JSON data received from a server may be processed to update the user interface or make decisions.
- Data Storage: JSON data can be stored in databases, files, or other data storage systems in its serialized form or as part of larger data records.
- Data Presentation: In various applications, including web development, data from JSON may be presented to users through graphical interfaces, reports, or visualizations.
- Data Interchange: JSON is commonly used for data interchange between systems, such as web services, APIs, and microservices, where data needs to be exchanged between different software components or between different organizations.
JSON is a versatile and straightforward format for representing structured data, and its workflow involves creating, serializing, transmitting, deserializing, processing, storing, presenting, and interchanging data in JSON format. It is widely used for various data exchange scenarios in modern software development.
How JSON Works & Architecture?
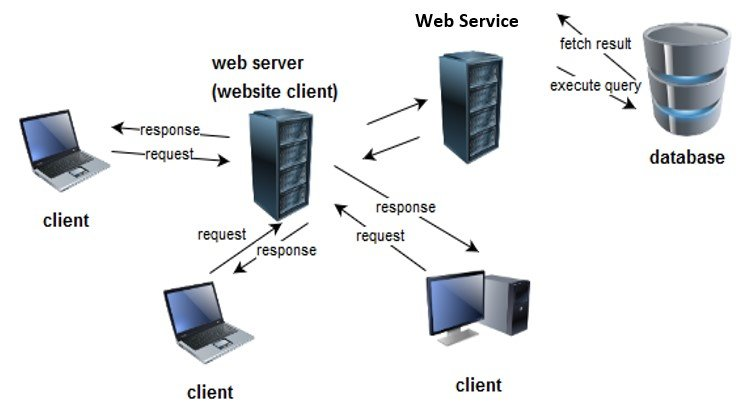
JSON stands for JavaScript Object Notation. It is a lightweight data-interchange format. JSON is text only, and uses conventions that are familiar to programmers of the C-family of languages, including C, C++, C#, Java, JavaScript, Perl, Python, and many others.
JSON is often used in conjunction with AJAX, a technique for making asynchronous requests from a web browser to a web server. This allows web pages to update without having to reload the entire page.
JSON is also used in a variety of other applications, such as:
- RESTful APIs
- WebSockets
- Cloud computing
- Mobile development
- Game development
The basic syntax of JSON is as follows:
{
"key1": "value1",
"key2": "value2",
"key3": [
"value3_1",
"value3_2"
]
}
In this example, the JSON object has three key-value pairs. The first key-value pair has the key key1
and the value value1
. The second key-value pair has the key key2
and the value value2
. The third key-value pair has the key key3
and the value of an array. The array has two elements, value3_1
and value3_2
.
JSON is a very simple format, but it is very powerful. It is easy to read and write, and it is easy for computers to parse and generate. This makes it a popular choice for data interchange between different systems.
How to Install and Configure JSON?
To install JSON, you will need to install a JSON parser. There are many different JSON parsers available, so you can choose one that is compatible with your programming language.
Once you have installed a JSON parser, you can configure it to your specific needs. This may involve setting the following options:
- The location of the JSON file
- The encoding of the JSON file
- The format of the output
Once you have configured the JSON parser, you can use it to read and write JSON files.
Some of the benefits of using JSON:
- It is lightweight and easy to transmit.
- It is human-readable and easy to debug.
- It is a standard format that is supported by many programming languages.
- It is easy to parse and generate.
Here are some of the limitations of using JSON:
- It is not as efficient as some other data formats, such as binary formats.
- It is not as secure as some other data formats, such as XML.
- It is not as flexible as some other data formats, such as YAML.
Overall, JSON is a popular and versatile data format that is easy to use and understand. It is a good choice for a variety of applications, including RESTful APIs, WebSockets, cloud computing, mobile development, and game development.
Fundamental Tutorials of JSON: Getting Started Step by Step
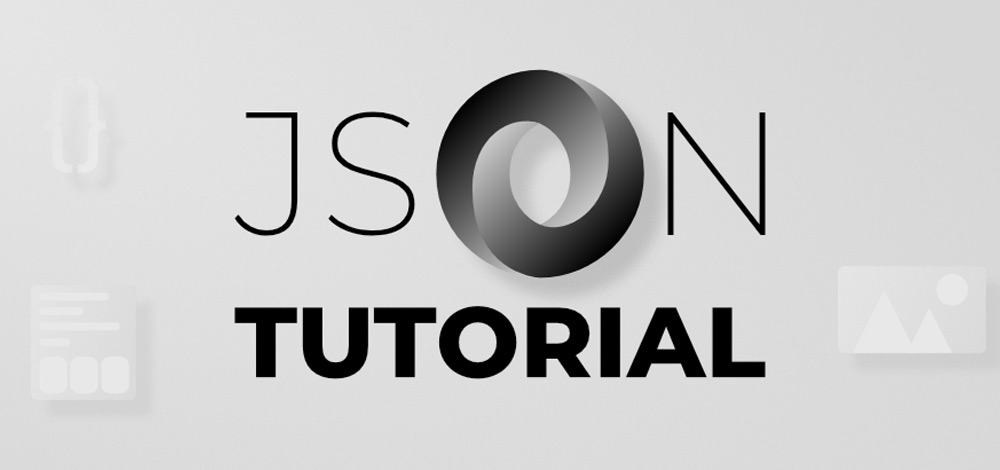
The following are the step-by-step fundamental tutorials of JSON:
- Create a simple JSON object
A JSON object is a collection of key-value pairs. The keys are strings, and the values can be strings, numbers, booleans, null, or arrays.
To create a simple JSON object, you can use the following steps:
1. Open a text editor.
2. Create a new file and save it with a .json extension.
3. In the file, type the below code:
{
"name": "John Doe",
"age": 30
}
This code creates a JSON object with two key-value pairs. The first key-value pair has the key name
and the value John Doe
. The second key-value pair has the key age
and the value 30
.
- Save the file.
- Open the file in a JSON parser to verify that the JSON object is valid.
2. Create a JSON array
A JSON array is a collection of values. The values can be strings, numbers, booleans, null, or other arrays.
To create a JSON array, you can use the following steps:
1. Open a text editor.
2. Create a new file and save it with a .json extension.
3. In the file, type the below code:
[
"John Doe",
30,
true
]
This code creates a JSON array with three values. The first value is the string John Doe
, the second value is the number 30
, and the third value is the boolean true
.
- Save the file.
- Open the file in a JSON parser to verify that the JSON array is valid.
3. Nest JSON objects and arrays
JSON objects and arrays can be nested. This means that you can put JSON objects or arrays inside other JSON objects or arrays.
To nest JSON objects and arrays, you can use the following steps:
1. Open a text editor.
2. Create a new file and save it with a .json extension.
3. In the file, type the below code:
{
"name": "John Doe",
"age": 30,
"children": [
{
"name": "Jane Doe",
"age": 20
},
{
"name": "Peter Doe",
"age": 10
}
]
}
This code creates a JSON object with three key-value pairs. The first key-value pair has the key name
and the value John Doe
. The second key-value pair has the key age
and the value 30
. The third key-value pair has the key children
and the value of an array. The array has two elements, which are JSON objects. The first JSON object has the key-value pair name
with the value Jane Doe
. The second JSON object has the key-value pair name
with the value Peter Doe
.
- Use JSON with a programming language
JSON can be applied with a variety of programming languages. To use JSON with a programming language, you will need to use a JSON library. A JSON library is a set of functions that can be used to read, write, and parse JSON data.
To use a JSON library, you will need to import the library into your program. Once the library is imported, you can use the functions in the library to work with JSON data.
Here are some examples of how to use JSON with different programming languages:
- JavaScript: The
JSON
object in JavaScript provides methods for working with JSON data. - Python: The
json
module in Python supplies functions for working with JSON data. - Java: The
org.json
library in Java provides classes for working with JSON data. - C#: The
System.Text.Json
namespace in C# provides classes for working with JSON data.
- Tips on How to Become a DevOps Engineer - April 28, 2024
- Computer Programming Education Requirements – What You Need to Know - April 28, 2024
- The Role of Big Data in Higher Education - April 28, 2024