History & Origin of jsp
JSP, a specification of Sun Microsystems, first appeared in 1998. The official versions, 1.0 and 1.1, both appeared in 1999, and both were very popular. The current version, 1.2, appeared in 2001, and is the most popular implementation yet; this is the version we’ll be using in this book.
What is jsp?
JavaServer Pages (JSP) is a Java standard technology that enables you to write dynamic, data-driven pages for your Java web applications. JSP is built on top of the Java Servlet specification. The two technologies typically work together, especially in older Java web applications.
While JSP may not be your first choice for building dynamic web pages, it is a core Java web technology. JSP pages are relatively quick and easy to build, and they interact seamlessly with Java servlets in a servlet container like Tomcat. You will encounter JSP in older Java web applications, and from time to time you may find it useful for building simple, dynamic Java web pages. As a Java developer, you should at least be familiar with JSP.
This article will be a quick introduction to JavaServer Pages, including the JSP Standard Tag Library (JSTL). Examples show you how to write a simple HTML page, embed JSP tags to connect to a Java servlet, and run the page in a servlet container.
See previous articles in this series to learn more about Java servlets and JavaServer Faces.
Writing JSP pages
A simple JSP page (.jsp) consists of HTML markup embedded with JSP tags. When the file is processed on the server, the HTML is rendered as the application view, a web page. The embedded JSP tags will be used to call server-side code and data. The diagram in Figure 1 shows the interaction between HTML, JSP, and the web application server.
Listing 1. A simple JSP page
<html>
<body>
<p>${2 * 2} should equal 4</p>
</body>
</html>
In Listing 1, you see a block of HTML that includes a JSP expression, which is an instruction to the Java server written using Expression Language (EL). In the expression “${2 * 2}
“, the “${}
” is JSP syntax for interpolating code into HTML. When executed, the JSP will output the results of executing whatever is inside the expression. In this case, the output will be the number 4.
How jsp works aka jsp architecture?
JSP Architecture
JSP architecture gives a high-level view of the working of JSP. JSP architecture is a 3 tier architecture. It has a Client, Web Server, and Database. The client is the web browser or application on the user side. Web Server uses a JSP Engine i.e; a container that processes JSP. For example, Apache Tomcat has a built-in JSP Engine. JSP Engine intercepts the request for JSP and provides the runtime environment for the understanding and processing of JSP files. It reads, parses, build Java Servlet, Compiles and Executes Java code, and returns the HTML page to the client. The webserver has access to the Database. The following diagram shows the architecture of JSP.
Now let us discuss JSP which stands for Java Server Pages. It is a server-side technology. It is used for creating web applications. It is used to create dynamic web content. In this JSP tags are used to insert JAVA code into HTML pages. It is an advanced version of Servlet Technology. It is a Web-based technology that helps us to create dynamic and platform-independent web pages. In this, Java code can be inserted in HTML/ XML pages or both. JSP is first converted into a servlet by JSP container before processing the client’s request. JSP Processing is illustrated and discussed in sequential steps prior to which a pictorial media is provided as a handful pick to understand the JSP processing better which is as follows:
Step 1: The client navigates to a file ending with the .jsp extension and the browser initiates an HTTP request to the webserver. For example, the user enters the login details and submits the button. The browser requests a status.jsp page from the webserver.
Step 2: If the compiled version of JSP exists in the web server, it returns the file. Otherwise, the request is forwarded to the JSP Engine. This is done by recognizing the URL ending with .jsp extension.
Step 3: The JSP Engine loads the JSP file and translates the JSP to Servlet(Java code). This is done by converting all the template text into println() statements and JSP elements to Java code. This process is called translation.
Step 4: The JSP engine compiles the Servlet to an executable .class file. It is forwarded to the Servlet engine. This process is called compilation or request processing phase.
Step 5: The .class file is executed by the Servlet engine which is a part of the Web Server. The output is an HTML file. The Servlet engine passes the output as an HTTP response to the webserver.
Step 6: The web server forwards the HTML file to the client’s browser.
Use case of jsp.
JSPs are usually used to deliver HTML and XML documents, but through the use of OutputStream, they can deliver other types of data as well. The Web container creates JSP implicit objects like request, response, session, application, config, page, pageContext, out and exception.
Feature and Advantage of using jsp.
JSP pages are more advantageous than Servlet:
- They are easy to maintain.
- No recompilation or redeployment is required.
- JSP has access to entire API of JAVA .
- JSP are extended version of Servlet.
Features of JSP
- Coding in JSP is easy :- As it is just adding JAVA code to HTML/XML.
- Reduction in the length of Code :- In JSP we use action tags, custom tags etc.
- Connection to Database is easier :-It is easier to connect website to database and allows to read or write data easily to the database.
- Make Interactive websites :- In this we can create dynamic web pages which helps user to interact in real time environment.
- Portable, Powerful, flexible and easy to maintain :- as these are browser and server independent.
- No Redeployment and No Re-Compilation :- It is dynamic, secure and platform independent so no need to re-compilation.
- Extension to Servlet :- as it has all features of servlets, implicit objects and custom tagsJSP syntax
Syntax available in JSP are following- Declaration Tag :-It is used to declare variables.
Syntax:- <%! Dec var %> Example:- <%! int var=10; %>
- Java Scriplets :- It allows us to add any number of JAVA code, variables and expressions.
Syntax:- <% java code %>
- JSP Expression :- It evaluates and convert the expression to a string.
Syntax:- <%= expression %> Example:- <% num1 = num1+num2 %>
- JAVA Comments :- It contains the text that is added for information which has to be ignored.
Syntax:- <% -- JSP Comments %>
Process of Execution
Steps for Execution of JSP are following:-- Create html page from where request will be sent to server eg try.html.
- To handle to request of user next is to create .jsp file Eg. new.jsp
- Create project folder structure.
- Create XML file eg my.xml.
- Create WAR file.
- Start Tomcat
- Run Application
Example of Hello World
We will make one .html file and .jsp filedemo.jsp <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1"> <title>Hello World - JSP tutorial</title> </head> <body> <%= "Hello World!" %> </body> </html>
Advantages of using JSP
- It does not require advanced knowledge of JAVA
- It is capable of handling exceptions
- Easy to use and learn
- It can tags which are easy to use and understand
- Implicit objects are there which reduces the length of code
- It is suitable for both JAVA and non JAVA programmer
Disadvantages of using JSP
- Difficult to debug for errors.
- First time access leads to wastage of time
- It’s output is HTML which lacks features.
- Declaration Tag :-It is used to declare variables.
Best Alternative of jsp.
For JSP there are other alternatives. For example you could program all your web pages with Servlet, even thought it is not very professional but it is possible. There were Struts, tapestry and some other alternative. The one I have used and mastered is JSF (Java server faces).
Servlets are the Java programs that run on the Java-enabled web server or application server. They are used to handle the request obtained from the webserver, process the request, produce the response, then send the response back to the webserver. Below are some alternatives to servlets:
1. Common Gateway Interface (CGI)
It is the most typical server-side solution. CGI application is an independent program that receives requests from the online server and sends it back to the webserver. The use of CGI scripts was to process forms. it’s the technology that permits web browsers to submit forms and connect with programs over an internet server. The common gateway interface provides an even way for data to be passed from the user’s request to the appliance program and back to the user.
Example: When you fill-up the shape and submit the shape applying, click the submit button and it goes, what are the results from this level is CGI.
A common problem in this when a new process is created every time the web server receives a CGI request so it results in a delay of response time.
2. Proprietary API
Many proprietary web servers have built-in support for server-side programming. These also are called non-free software, or closed-source software, is computer software that the software’s publisher or another person retains property right usual copyright of the ASCII text file, but sometimes patent rights. It’s a software library interface “specific to at least one device or, more likely to a variety of devices within a specific manufacturer’s product range”. The motivation for employing a proprietary API is often vendor lock-in or because standard APIs don’t support the device’s functionality.
Examples: Netscape’s NSAPI, Microsoft’s ISAPI, and O’Reilly’s WSAPI.
Drawback: Most of these are developed in C/C++ and hence can contain memory leaks and core dumps that can crash the webserver.
3. Active Server Pages (ASP)
Microsoft’s ASP is another technology that supports server-side programming. Only Microsoft’s Internet Information Server (IIS) supports this technology which isn’t free. They work with simple HTML pages, the client (a web surfer) requests an internet page from a server. The server just sends the file to the client, and therefore the page is shown on the client’s browser. ASP is now obsolete and replaced with ASP.NET. ASP.NET may be a compiled language and relies on the .NET Framework, while ASP is strictly an interpreted language.
4. Serverside JavaScript
It is another alternative to servlets. The sole known servers that support it are Netscape’s Enterprise and FastTrack servers. This ties you to a specific vendor. Server-side JavaScript can be a JavaScript code that runs over a server local resources and it is similar to Java or C#, but the syntax is predicated on JavaScript.
Example: An ideal of this is often Node.JS. The advantage of server-side scripting is that the ability to highly customize the response supported the user’s requirements, access rights, or queries into data stores.
Best Resources, Tutorials and Guide for jsp.
Free Video Tutorials of jsp
Interview Questions and Answer for JSP
1. What is JSP?
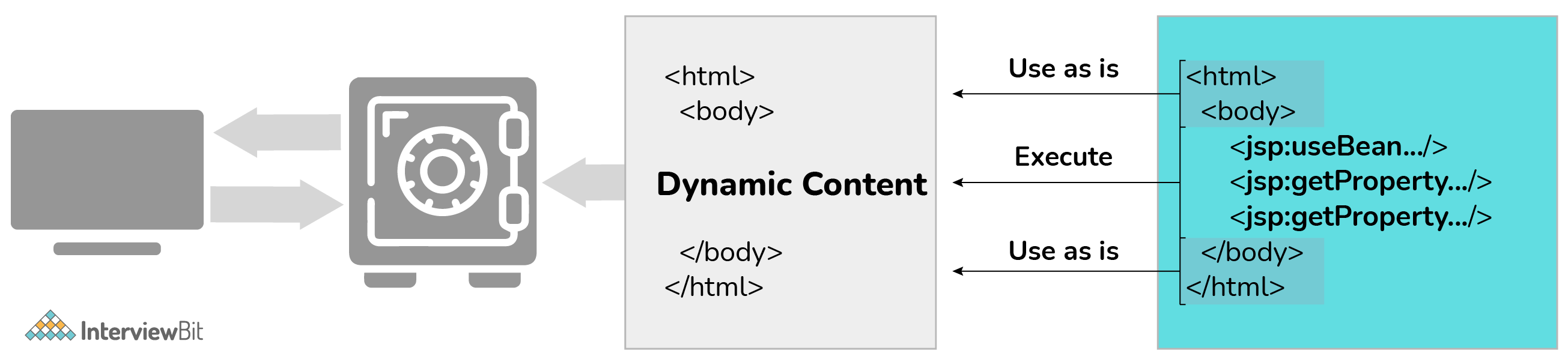
2. How does JSP work?
3. How does JSP Initialization take place?
public void jspInit(){
// Initialization code...
}
4. What is the use of JSP?
Alternatives such as ISAPI from Microsoft, and Java Servlets from Sun Microsystems, offer better performance and scalability. However, they generate web pages by embedding HTML directly in programming language code. JavaServer Pages (JSP) changes all of that.
5. What are some of the advantages of using JSP?
- Better performance and quality as JSP is a specification and not a product.
- JSP pages can be used in combination with servlets.
- JSP is an integral part of J2EE, a complete platform for Enterprise-class applications.
- JSP supports both scripting and element-based dynamic content.
6. What is Java Server Template Engines?
Two popular template engines are WebMacro (http://www.webmacro.org) and FreeMarker (http://freemarker.sourceforge.net).
7. What are Servlets?
- Platform and vendor independence
- Integration
- Efficiency
- Scalability
- Robustness and security
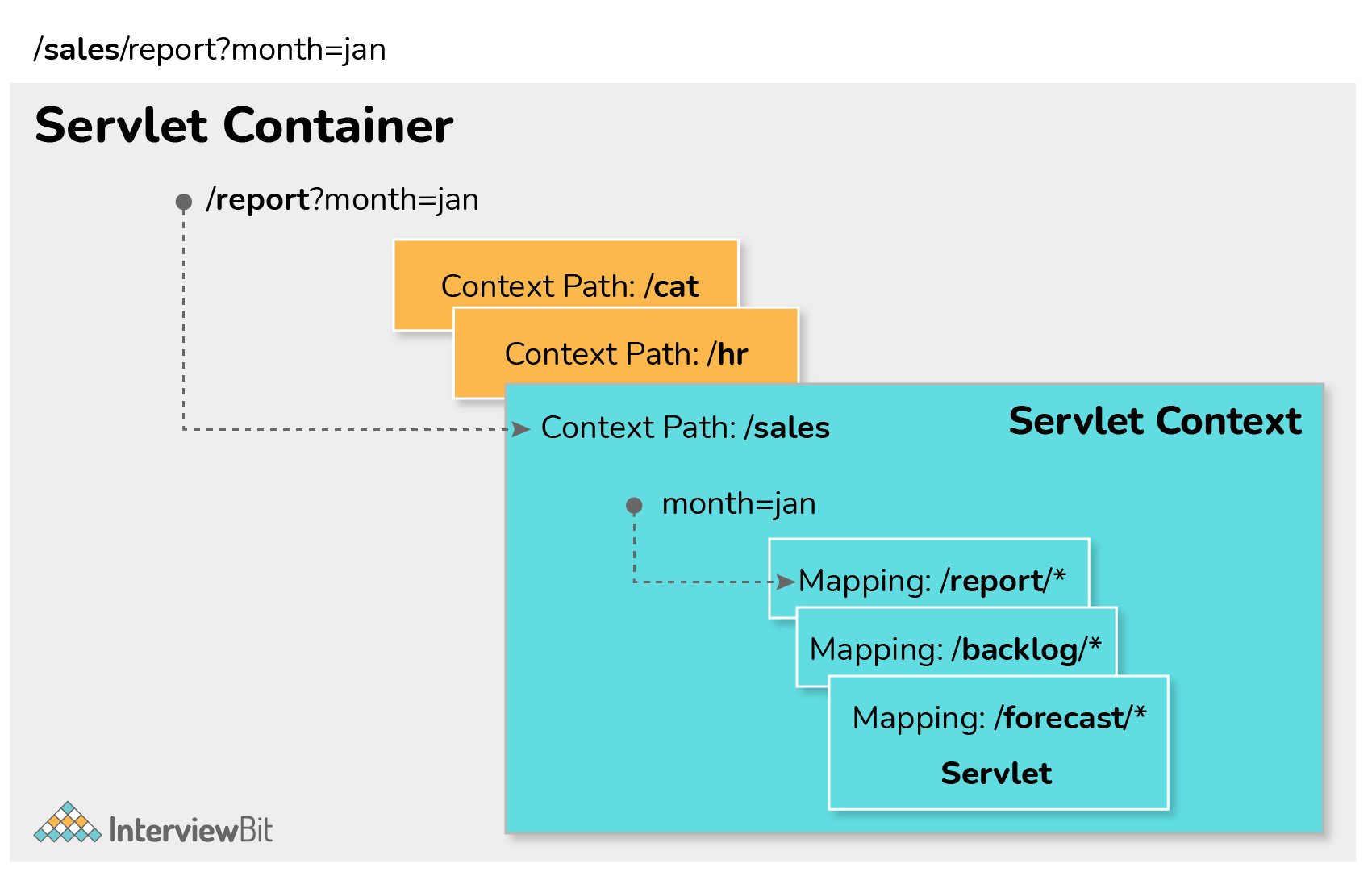
8. Explain the Life Cycle of a servlet.
A servlet is loaded when a web server receives a request that should be handled by it. Once a servlet has been loaded, the same servlet instance (object) is called to process succeeding requests. Eventually, the webserver needs to shut down the servlet, typically when the web server itself is shut down.
The 3 life cycle methods are:
- public void init(ServletConfig config)
- public void service(ServletRequest req, ServletResponse res)
- public void destroy( )
These methods define the interactions between the web server and the servlet.
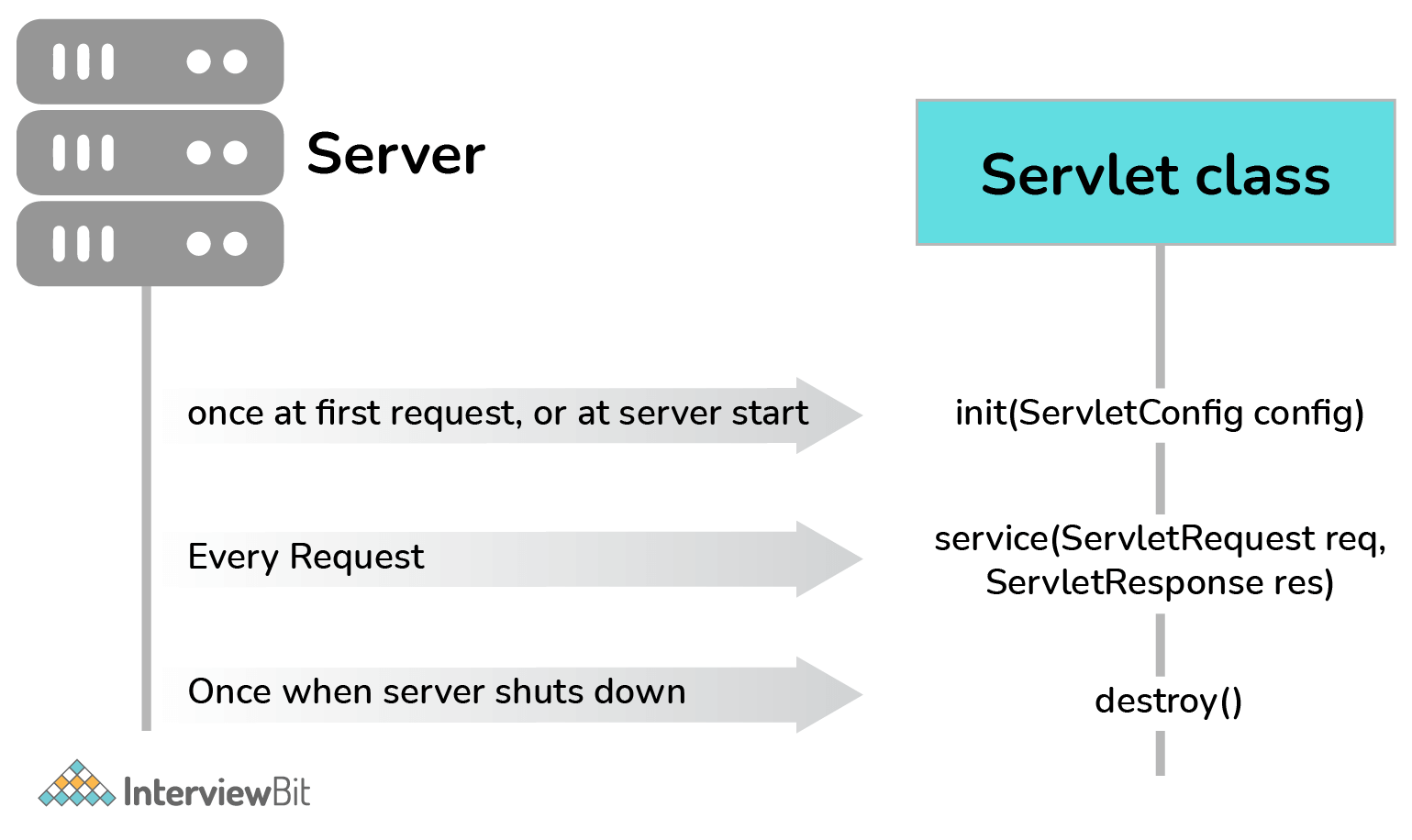
9. What are the types of elements with Java Server Pages (JSP)?
Following are the Directive Elements:
Element | Description |
---|---|
<%@ page … %> | Defines page-dependent attributes, such as scripting language, error page, and buffering requirements. |
<%@ include … %> | Includes a file during the translation phase. |
<%@ taglib … %> | Declares a tag library, containing custom actions, used on the page. |
The Action elements are:
Element | Description |
---|---|
<jsp:useBean> | This is for making the JavaBeans component available on a page. |
<jsp:getProperty> | This is used to get a property value from a JavaBeans component and to add it to the response. |
<jsp:setProperty> | This is used to set a value for the JavaBeans property. |
<jsp:include> | This includes the response from a servlet or JSP page during the request processing phase. |
<jsp:forward> | This is used to forward the processing of a request to a JSP page or servlet. |
<jsp:param> | This is used for adding a parameter value to a request given to another servlet or JSP page by using <jsp:include> or <jsp:forward> |
<jsp:plugin> | This is used to generate HTML that contains the proper client browser-dependent elements which are used to execute an Applet with Java Plugin software. |
And lastly, the Scripting elements are:
Element | Description |
---|---|
<% … %> | Scriptlet used to embed scripting code. |
<%= … %> | Expression, used to embed Java expressions when the result shall be added to the response. Also used as runtime action attribute values. |
<%! … %> | Declaration used to declare instance variables and methods in the JSP page implementation class. |
10. What is the difference between JSP and Javascript?
11. What is JSP Expression Language (EL)?
- What is Mobile Virtual Network Operator? - April 18, 2024
- What is Solr? - April 17, 2024
- Difference between UBUNTU and UBUNTU PRO - April 17, 2024