What is Keras?
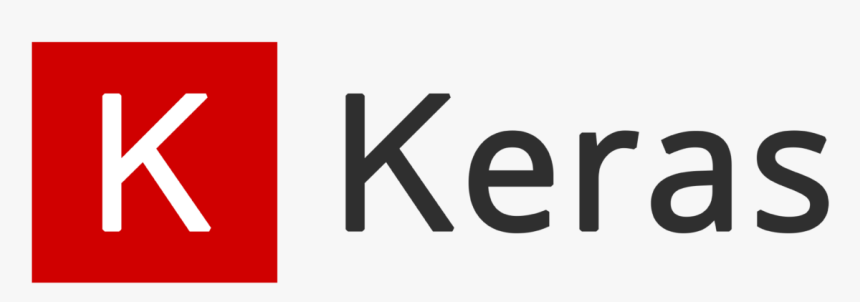
Keras is a powerful deep learning library that allows you to build and train neural networks with ease. It was initially developed as an independent project but has become an integral part of the TensorFlow project, one of the most popular deep learning libraries. Keras provides a high-level API that allows users to build, experiment with, and deploy deep learning models with ease. Its user-friendly interface makes it suitable for both beginners and experienced researchers.
Top 10 use cases of Keras:
Here are the top 10 use cases of Keras:
- Image Classification: Keras is often used for image classification tasks, such as identifying objects or characters within images. Common applications include facial recognition, object detection, and medical image analysis.
- Natural Language Processing (NLP): Keras enables the creation of neural networks for text processing tasks like sentiment analysis, named entity recognition, and language translation. Its Sequential and functional API make building NLP models straightforward.
- Speech Recognition: Keras can be employed to build models for speech recognition and synthesis. It’s used to create systems that convert spoken language into written text and vice versa.
- Anomaly Detection: Keras can help build anomaly detection systems that identify unusual patterns or outliers within data, which is valuable for fraud detection, network security, and industrial equipment monitoring.
- Recommendation Systems: Keras can be used to construct recommendation systems that suggest products, services, or content based on user preferences and historical data.
- Time Series Analysis: Keras models can be applied to time series forecasting tasks, such as predicting stock prices, weather conditions, or sales trends. Recurrent Neural Networks (RNNs) and Long Short-Term Memory (LSTM) networks are commonly used for these tasks.
- Generative Models: Keras provides tools for creating generative models, including Variational Autoencoders (VAEs) and Generative Adversarial Networks (GANs). These models are used for generating new images, music, text, and more.
- Medical Imaging: Keras is used in medical research and diagnostics, where deep learning models are applied to analyze medical images, identify diseases, and assist doctors in making diagnoses.
- Robotics and Autonomous Systems: Keras can be used to create models for robot control, navigation, and perception. Deep learning can enhance robots’ ability to understand their surroundings and perform complex tasks.
- Game AI: Keras can be applied to building AI agents for playing games, both in traditional settings and video games. Reinforcement learning algorithms can be combined with Keras to train agents to excel in specific games.
Remember that while Keras is a powerful tool for building deep learning models, it primarily focuses on ease of use and quick prototyping. For more complex and specialized applications, it may be necessary to dive deeper into TensorFlow and other deep learning frameworks.
What are the feature of Keras?
Keras offers certain features that make it a popular choice for experimenting and building with deep learning models:
- User-Friendly API: Keras provides a high-level and user-friendly API for building neural networks and deep learning models. Its simple syntax allows users to quickly define and configure complex architectures.
- Modularity: Keras encourages a modular approach to building models. It provides a variety of pre-built layers, activations, optimizers, loss functions, and other components that can be easily combined to create custom models.
- Flexibility: Keras supports both Sequential and Functional APIs, allowing you to create a wide range of neural network architectures, including multi-input and multi-output models.
- Wide Range of Layers: Keras offers a rich set of built-in layers for various tasks, including dense (fully connected), convolutional, recurrent, normalization, and more.
- Pre-Trained Models: Keras provides access to pre-trained models that have been trained on large datasets. These models can be fine-tuned or used as feature extractors for specific tasks.
- Multiple Backends: Keras can run on top of different deep learning frameworks, such as TensorFlow, Theano, and Microsoft Cognitive Toolkit (CNTK), although in recent versions, TensorFlow has become the recommended backend.
- Ease of Experimentation: Keras makes it easy to experiment with different architectures and hyperparameters, allowing you to quickly iterate and fine-tune your models.
- Visualization: Keras offers tools for visualizing model architectures and training history, making it easier to understand and analyze the behavior of your models.
- Integration with Other Libraries: Keras can be seamlessly integrated with other Python libraries like NumPy, SciPy, and Matplotlib, enhancing its capabilities for data manipulation, analysis, and visualization.
How Keras Works and Architecture?
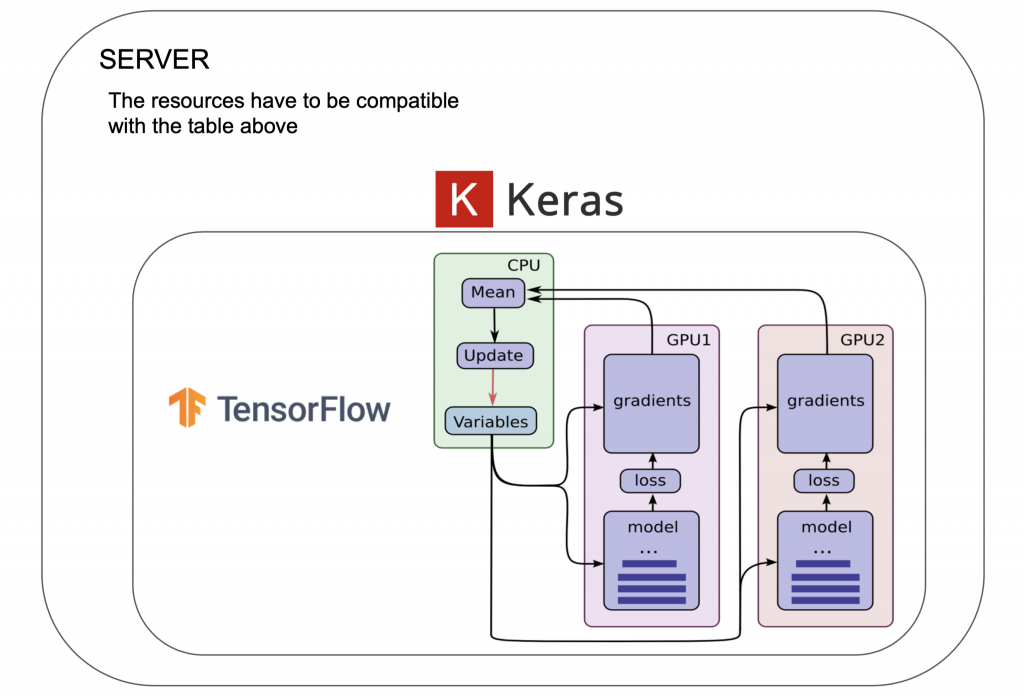
Keras follows a modular architecture that makes it easy to create and configure deep learning models. Here’s a simplified overview of how Keras works:
- Import Keras and Required Modules: Import the necessary modules from Keras to create and configure your deep learning model. This includes importing layers, optimizers, and other components.
- Define Model Architecture: Use the Sequential or Functional API to define the architecture of your model. You can stack layers on top of each other, specifying the number of neurons, activation functions, and other properties.
- Compile the Model: After defining the model architecture, you compile it using the
compile
method. During compilation, you specify the optimizer, loss function, and evaluation metrics that the model will use during training. - Data Preparation: Prepare your data in the appropriate format, often as NumPy arrays. Data preprocessing steps like scaling, normalization, and splitting into training and validation sets should be performed at this stage.
- Model Training: Use the
fit
method to train the model on your training data. You provide input data and corresponding target values, and Keras adjusts the model’s parameters to minimize the specified loss function. - Model Evaluation: After training, you can use the model’s
evaluate
method to assess its performance on the validation or test data. This provides metrics such as accuracy, loss, and other user-defined evaluation criteria. - Model Prediction: Once the model is trained and evaluated, you can use the
predict
method to make predictions on new, unseen data. - Fine-Tuning and Experimentation: You can iterate on the model architecture, hyperparameters, and other settings to improve performance. Keras allows you to easily experiment with different configurations.
Keras abstracts many of the complexities of building and training deep learning models, allowing users to focus on designing architectures and experimenting with various configurations. Under the hood, Keras interfaces with the chosen backend (usually TensorFlow) to perform the computations required for model training and prediction. This abstraction makes Keras an ideal tool for rapid prototyping and experimentation in deep learning projects.
How to Install Keras?
There are a few ways to install Keras. Here are the steps for each method:
Using pip
- Open a terminal window.
- Check if pip is installed by running the following command:
pip --version
If pip is not installed, you can install it by running the following command:
python -m pip install --user pip
- Once pip is installed, you can install Keras by running the following command:
pip install keras
Using Anaconda
- Install Anaconda. Anaconda is a distribution of Python that comes with many pre-installed packages, including Keras.
- Once Anaconda is installed, you can open a terminal window and type the following command to install Keras:
conda install keras
Building from source
- Download the Keras source code from the Keras website: https://keras.io/.
- Unzip the source code archive.
- Open a terminal window and navigate to the Keras source code directory.
- Run the following command to build Keras:
python setup.py install
Which method should I use?
The best method to install Keras depends on your operating system and your preferences. If you are not sure which method to use, I recommend using pip.
Here are some additional things to keep in mind when installing Keras:
- You need to have Python 3 installed.
- You need to have pip installed.
- If you are installing Keras from source, you need to have the following dependencies installed:
- TensorFlow
- NumPy
- SciPy
- Matplotlib
Basic Tutorials of Keras: Getting Started
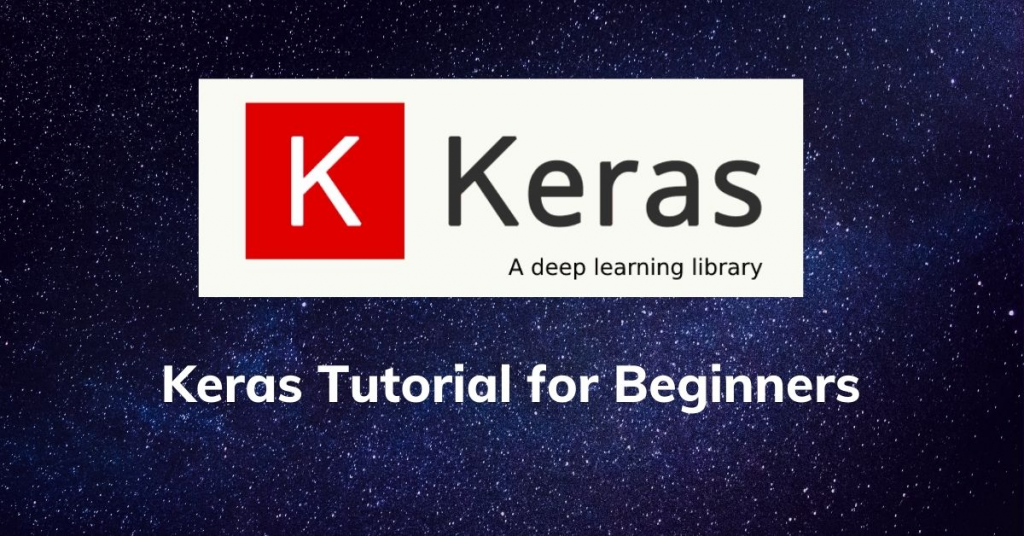
The following steps are the basic tutorials of Keras:
- Create a simple neural network.
Python
import keras
model = keras.Sequential([
keras.layers.Dense(10, activation='relu'),
keras.layers.Dense(10, activation='softmax')
])
This creates a simple neural network with two dense layers. The first layer has 10 neurons and applys the relu
activation function. The second layer has 10 neurons and applys the softmax
activation function.
- Compile the model.
Python
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics= ['accuracy'])
This compiles the model with the Adam optimizer, the categorical crossentropy loss function, and the accuracy metric.
- Load a dataset.
Python
(x_train, y_train), (x_test, y_test) = keras.datasets.mnist.load_data()
This loads the MNIST dataset, which is a handwritten digits dataset. The x_train
and y_train
tensors contain the training data, and the x_test
and y_test
tensors contain the testing data.
- Train the model.
Python
model.fit(x_train, y_train, epochs=10)
This trains the model on the training data for 10 epochs.
- Evaluate the model.
Python
model.evaluate(x_test, y_test)
This evaluates the model on the testing data.
- Make predictions.
Python
predictions = model.predict(x_test)
This makes predictions for the testing data.
These are just the basic steps involved in creating and training a simple neural network using Keras. There are many other things you can do with Keras, such as using different types of layers, adding regularization, and saving and loading models.
- Why Can’t I Make Create A New Folder on External Drive on Mac – Solved - April 28, 2024
- Tips on How to Become a DevOps Engineer - April 28, 2024
- Computer Programming Education Requirements – What You Need to Know - April 28, 2024