What is Kotlin?
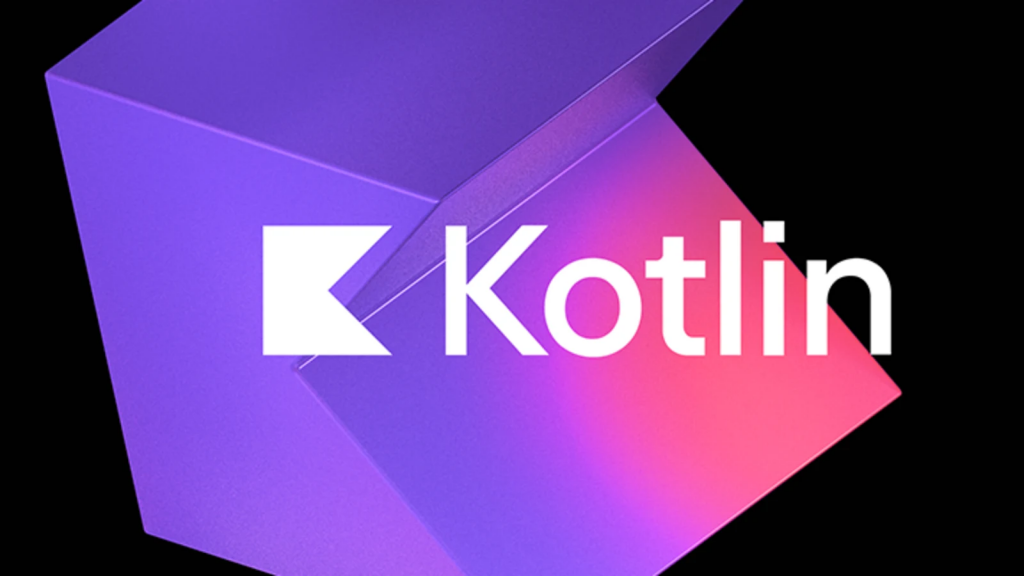
Kotlin is a modern programming language that runs on the JVM(Java Virtual Machine) and can also be assembled to JavaScript or native code. It was developed by JetBrains and officially endorsed by Google for Android app development. Kotlin is designed to be concise, expressive, and interoperable with existing Java code, making it a popular choice for a variety of applications.
Key features of Kotlin include:
- Conciseness: Kotlin is known for its concise syntax, reducing boilerplate code and making it more readable than Java. This can lead to increased productivity for developers.
- Interoperability: Kotlin is fully interoperable with Java, which means that existing Java code can be used in Kotlin projects and vice versa. This makes it easy for developers to adopt Kotlin gradually in their projects.
- Null Safety: Kotlin addresses the issue of null pointer exceptions by introducing null safety features. Nullable and non-nullable types are explicitly defined, reducing the likelihood of null-related runtime errors.
- Smart Casts: Kotlin includes smart casts that automatically cast types within a block of code after a type check. This helps eliminate redundant casting and improves code clarity.
- Extension Functions: Kotlin permits developers to add new functions to existing classes without changing their source code. These are called extension functions and can enhance the functionality of third-party libraries.
- Coroutines: Kotlin introduces coroutines, a lightweight and efficient concurrency framework. Coroutines make it easier to write asynchronous code and perform concurrent tasks without the complexity of traditional threading.
- Data Classes: Kotlin simplifies the creation of classes that are primarily used for storing data by providing concise syntax for data classes. These classes automatically generate common methods such as equals(), hashCode(), and toString().
- Functional Programming Features: Kotlin supports functional programming concepts, including higher-order functions, lambda expressions, and immutable data structures. This makes it suitable for developers who prefer functional programming paradigms.
What is top use cases of Kotlin?
Top use cases of Kotlin include:
- Android App Development: Kotlin is officially supported by Google for Android app development. Many Android developers have adopted Kotlin due to its modern features and improved syntax compared to Java.
- Backend Development: Kotlin can be used to build server-side applications, web services, and microservices. It is often used with frameworks like Spring Boot to create efficient and scalable backend systems.
- Cross-platform Development: Kotlin Multiplatform allows developers to write shared code that can be used across multiple platforms, including JVM, Android, iOS, and JavaScript. This enables the development of cross-platform applications with a single codebase.
- Desktop Application Development: Kotlin can be used for developing desktop applications using frameworks such as TornadoFX or JavaFX. Its concise syntax and interoperability with Java make it a suitable choice for desktop development.
- Libraries and Tools: Kotlin is used to develop libraries, tools, and utilities. Many open-source projects and tools have adopted Kotlin due to its expressive syntax and compatibility with existing Java code.
- Scripting: Kotlin can be used for scripting purposes, offering a more structured and statically-typed alternative to traditional scripting languages.
- Educational Purposes: Kotlin is used in educational settings to teach programming concepts and modern language features. Its simplicity and interoperability make it accessible for both beginners and experienced developers.
Kotlin’s versatility and compatibility with existing Java code have contributed to its widespread adoption in various domains, making it a language of choice for many developers and organizations.
What are feature of Kotlin?
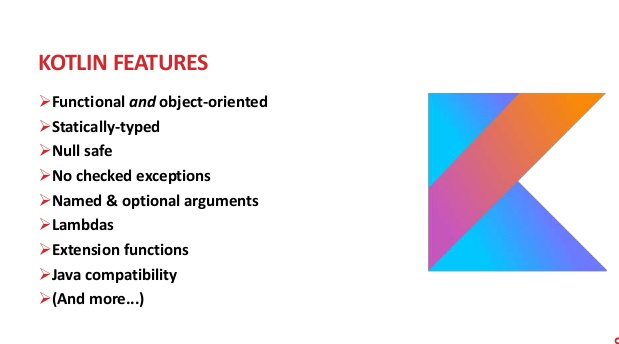
Kotlin comes with a range of features that make it a powerful and modern programming language. let’s have a look at some key features of Kotlin:
- Conciseness: Kotlin is designed to be concise, reducing boilerplate code compared to languages like Java. This leads to more readable and expressive code.
- Interoperability with Java: Kotlin is fully interoperable with Java, allowing developers to use existing Java libraries and frameworks seamlessly. This facilitates the gradual adoption of Kotlin in projects with existing Java codebases.
- Null Safety: Kotlin addresses the null pointer exception problem with a strong null safety system. Nullable and non-nullable types are explicitly defined, reducing the risk of null-related runtime errors.
- Extension Functions: Kotlin supports extension functions, allowing developers to add new functions to existing classes without modifying their source code. This promotes clean and modular code.
- Smart Casts: Kotlin includes smart casts, which automatically cast types within a block of code after a type check. This eliminates the need for explicit casting and improves code readability.
- Coroutines: Kotlin introduces coroutines, a lightweight and efficient concurrency framework. Coroutines simplify asynchronous programming, making it easier to write non-blocking and concurrent code.
- Data Classes: Kotlin provides a concise syntax for creating data classes, which are used for storing and manipulating data. Data classes automatically create common process such as equals(), hashCode(), and toString().
- Functional Programming Features: Kotlin supports functional programming concepts, including higher-order functions, lambda expressions, and immutable data structures. This permits developers to write more expressive and concise code.
- Type Inference: Kotlin has a strong static type system, but it also features type inference, reducing the need for explicit type annotations in many cases. This strikes a balance between static typing and concise syntax.
- Comprehensive Standard Library: Kotlin comes with a comprehensive standard library that provides useful utilities and extension functions, making common tasks more convenient for developers.
What is the workflow of Kotlin?
Now, let’s outline a general workflow for using Kotlin:
- Setting Up Development Environment:
- Install a Kotlin-compatible Integrated Development Environment (IDE) such as IntelliJ IDEA, Android Studio, or use the Kotlin command-line compiler.
- Creating a Kotlin Project:
- Create a new Kotlin project using your preferred build tool, such as Gradle or Maven.
- Writing Kotlin Code:
- Write Kotlin code in source files with a
.kt
extension. Define functions, classes, and other code structures as needed.
- Write Kotlin code in source files with a
- Interoperability with Java:
- If working in a mixed-language environment, leverage Kotlin’s interoperability with Java. Use Java libraries and frameworks in your Kotlin code seamlessly.
- Compiling Kotlin Code:
- Use the Kotlin compiler to compile your code. The compiler produces Java bytecode that can run on the Java Virtual Machine (JVM).
- Running and Debugging:
- Run and debug your Kotlin applications within the IDE or through the command line.
- Testing:
- Write tests for your Kotlin code using testing frameworks such as JUnit or KotlinTest.
- Version Control:
- Manage your codebase using a version control system (e.g., Git). Collaborate with other developers if working on a team.
- Building and Packaging:
- Use your build tool to build and package your Kotlin application. This may involve creating executable JAR files, Android APKs, or other deployment artifacts.
- Deployment:
- Deploy your Kotlin application to the desired platform, whether it’s a server, desktop, mobile device, or web browser.
- Maintenance and Iteration:
- Maintain and iterate on your Kotlin codebase as needed. Leverage the features of the language to enhance code quality and add new features.
This workflow is a general guideline and may vary based on the specific type of application you are developing (e.g., Android app, server-side application, web application) and the tools you choose to use.
How Kotlin Works & Architecture?
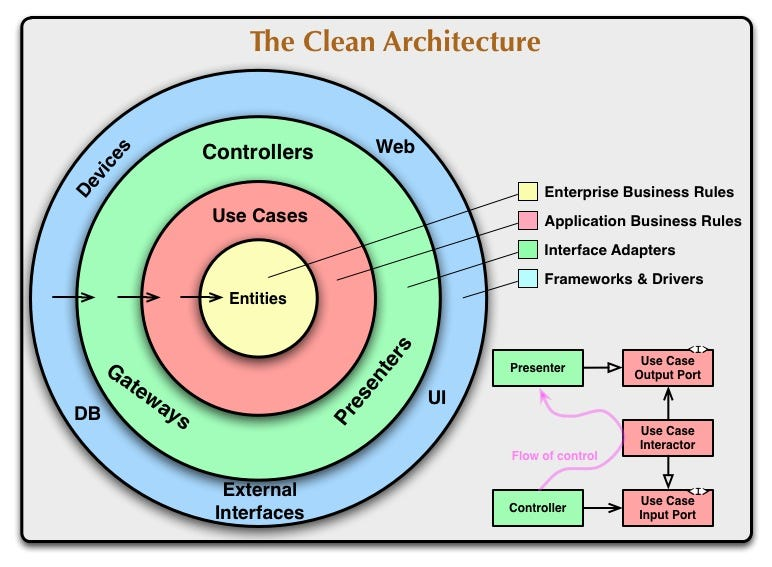
Kotlin is a modern statically typed programming language designed for building robust and expressive applications. Its unique features and architecture offer several advantages over traditional languages like Java. Here’s a breakdown of how Kotlin works and its key architectural aspects:
Core Features:
- Statically typed: Like Java, Kotlin ensures type safety through static type checking, preventing runtime errors.
- Concise and readable syntax: Kotlin emphasizes clarity and brevity, reducing boilerplate code compared to Java.
- Null safety: Kotlin eliminates null pointer exceptions through its built-in null-safety system, improving code reliability.
- Immutable data by default: Data immutability promotes safer concurrency and simplifies reasoning about program behavior.
- Higher-order functions and lambdas: Functional programming features enable concise and elegant code for complex tasks.
- Interoperability with Java: Kotlin code seamlessly integrates with existing Java libraries and frameworks.
Architecture:
- Compiler: Kotlin uses a dedicated compiler that translates Kotlin code into bytecode compatible with the Java Virtual Machine (JVM) or native code for platforms like Android and iOS.
- Standard Library: Kotlin offers a rich standard library providing essential functionalities like collections, concurrency, and input/output.
- Type system: Kotlin’s type system is more flexible than Java’s, allowing for type inference and smart casts based on context.
- Object-oriented programming (OOP): Kotlin supports traditional OOP concepts like classes, inheritance, and interfaces.
- Functional programming (FP): Kotlin embraces FP principles like immutability, higher-order functions, and lambdas, enabling functional programming styles.
Benefits of using Kotlin:
- Increased developer productivity: Concise syntax and smart features minimize boilerplate and enhance code readability, leading to faster development cycles.
- Reduced errors: Null safety and improved type checking contribute to more reliable and stable applications.
- Flexibility and expressiveness: Kotlin’s blend of OOP and FP features empowers developers to express their code in diverse and effective ways.
- Modern and evolving: Kotlin is actively maintained and receives regular updates with new features and improvements.
- Wide adoption and community: Kotlin is gaining traction across various domains, with a growing community and abundance of learning resources.
How to Install and Configure Kotlin?
Following is a comprehensive guide on installing and configuring Kotlin, tailored to different development environments:
1. Standalone Installation:
- Download: Get the Kotlin compiler and libraries from the official website.
- Extract: Unzip the downloaded package to a suitable directory.
- Set environment variables: Add the
bin
directory to your system’sPATH
to access the Kotlin compiler (kotlinc
) from the command line.
2. IDE Integration:
- IntelliJ IDEA: Kotlin is natively supported. No separate installation is needed.
- Eclipse: Install the Kotlin plugin from the Eclipse Marketplace.
- Visual Studio Code: Install the Kotlin Language Extension.
- Other IDEs: Check for available Kotlin plugins or extensions.
3. Android Development:
- Android Studio: Kotlin is fully integrated. No extra setup required.
- Gradle: Add the Kotlin Gradle plugin to your project’s
build.gradle
files.
4. Build Tools:
- Gradle: Use the Kotlin Gradle plugin for building Kotlin projects.
- Maven: Add the Kotlin Maven plugin to your project’s
pom.xml
file. - Command-line: Use
kotlinc
directly for basic compilation and execution.
Configuration Options:
- Target platforms: Specify the target platform (JVM, Android, JavaScript, etc.) using appropriate flags or configuration settings in your build tools.
- Compatibility: Adjust settings for compatibility with different Java versions or libraries.
Important Tips:
- Update regularly: Stay up-to-date with the latest Kotlin version for bug fixes and feature improvements.
- Explore libraries and frameworks: Kotlin has a rich ecosystem of libraries and frameworks to enhance development.
Troubleshooting:
- If you encounter issues, check error messages, consult online forums or communities, and refer to the official documentation for guidance.
Fundamental Tutorials of Kotlin: Getting started Step by Step
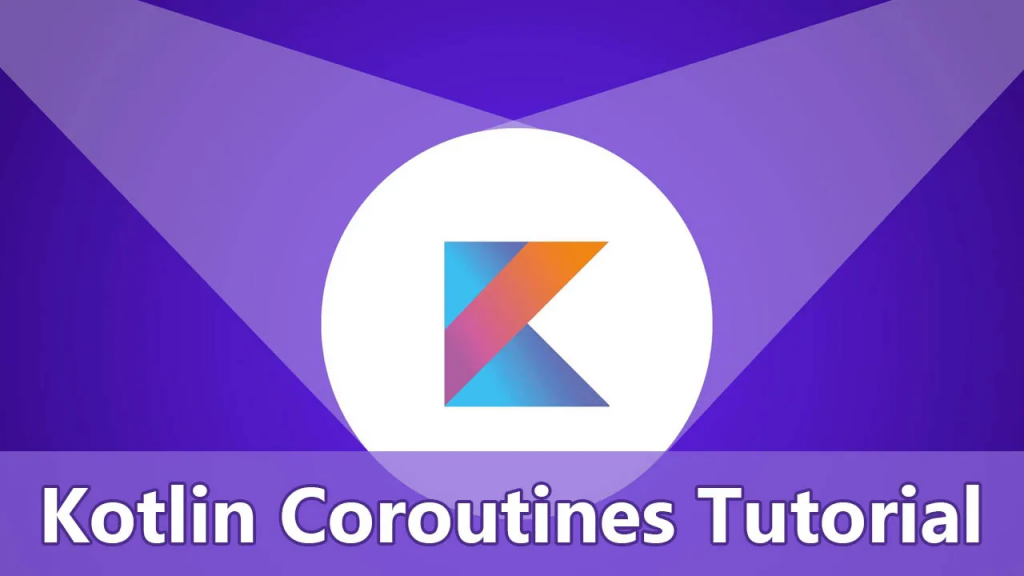
I’d be happy to guide you through step-by-step fundamental tutorials for Kotlin! To tailor the experience to your needs, I need a little more information:
1. Your experience: Are you a complete beginner to programming, or do you have some experience with other languages like Java or Python?
2. Your learning style: Do you prefer written tutorials, video lessons, interactive exercises, or a combination?
3. Your chosen development environment: Will you be using a standalone editor, an IDE like IntelliJ IDEA, or Android Studio?
Once I have this information, I can create a personalized learning path with specific tutorials and exercises to help you master the essentials of Kotlin.
In the meantime, here’s a general overview of what you can expect to learn in fundamental Kotlin tutorials:
Step 1: Setting Up:
- Download and install Kotlin based on your chosen environment.
- Understand basic syntax elements like variables, data types, and operators.
- Learn how to write simple expressions and statements.
Step 2: Control Flow:
- Implement conditional statements like
if
andwhen
to handle different scenarios. - Use loops like
for
andwhile
to iterate over data structures. - Break and continue statements for controlling loop execution.
Step 3: Functions:
- Define your own functions for code reuse and modularity.
- Understand function parameters, return values, and local variables.
- Learn about higher-order functions like lambdas for concise and expressive code.
Step 4: Classes and Objects:
- Define classes to represent real-world entities with attributes and methods.
- Understand object creation and member access syntax.
- Learn about constructors and inheritance for building complex object hierarchies.
Step 5: Collections:
- Work with data structures like lists, maps, and sets to store and organize data.
- Understand collection operations like adding, removing, and iterating over elements.
- Learn about accessing specific elements and filtering data based on conditions.
Step 6: Additional Features (Optional):
- Null safety in Kotlin and how to handle potential null references.
- Type inference and smart casts for automatic type determination.
- Object-oriented programming (OOP) concepts in Kotlin like interfaces and abstract classes.
- Functional programming paradigms like immutability and higher-order functions.
Once you’ve grasped these fundamentals, you can move on to more advanced topics like Kotlin’s concurrency features, libraries, and frameworks.
Always keep in mind, practice is key! Don’t hesitate to experiment with the concepts you learn and explore additional resources to solidify your understanding.
- PPG Industries: Selection and Interview process, Questions/Answers - April 3, 2024
- Fiserv: Selection and Interview process, Questions/Answers - April 3, 2024
- Estee Lauder: Selection and Interview process, Questions/Answers - April 3, 2024