What is Laravel?
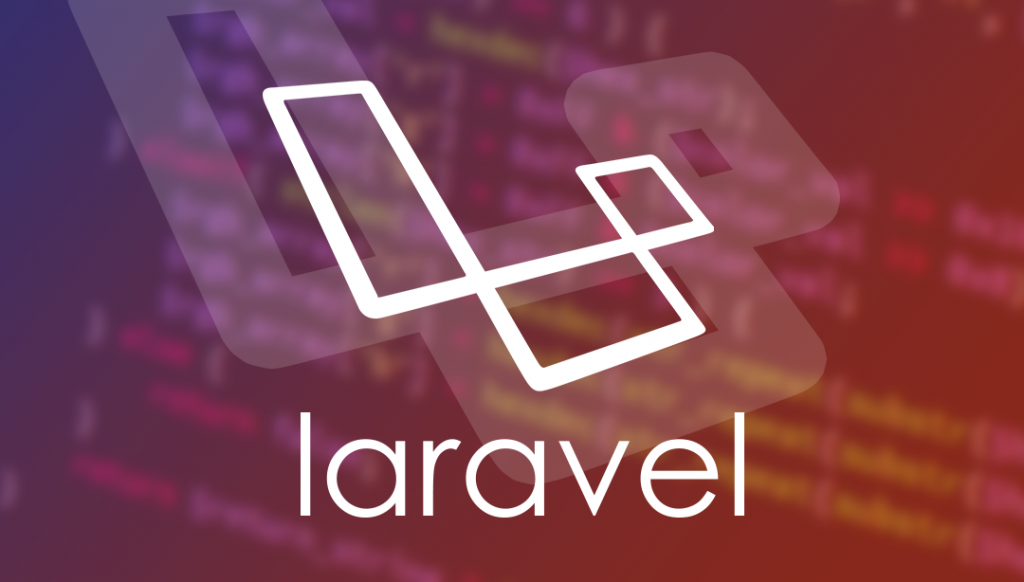
Laravel is an open-source, free PHP web framework designed for the development of web applications following the model-view-controller (MVC) architectural pattern. It was generated by Taylor Otwell and was first released in 2011. Laravel provides an elegant syntax and a set of tools and features that aim to make web development tasks easier and more efficient.
Some key features and components of Laravel include:
- Eloquent ORM (Object-Relational Mapping): A powerful and expressive ORM that allows developers to interact with the database using an object-oriented syntax, making database operations more intuitive.
- Blade Templating Engine: A lightweight yet powerful templating engine for creating dynamic, reusable views in Laravel.
- Artisan Console: A command-line interface that provides various helpful commands for tasks like database migrations, job scheduling, and more.
- Middleware: Allows developers to filter HTTP requests entering the application, providing a mechanism for handling tasks like authentication, logging, etc.
- Laravel Mix: An asset compilation tool that simplifies the process of working with CSS and JavaScript assets.
- Laravel Ecosystem: Laravel has a rich ecosystem with numerous packages and extensions that can be easily integrated to extend the functionality of your applications.
What is top use cases of Laravel?
Top Use Cases of Laravel:
- Web Application Development: Laravel is widely used for building web applications of various sizes and complexities, from small business websites to large enterprise applications.
- E-commerce Platforms: Laravel is often chosen for developing e-commerce platforms due to its robust features, ease of use, and the availability of packages like Laravel Cashier for handling subscription billing.
- Content Management Systems (CMS): Developers often use Laravel to build custom CMS solutions, allowing clients to manage and update their website content easily.
- API Development: Laravel provides tools for building RESTful APIs, making it a popular choice for creating backend services that power mobile apps, single-page applications, and other API-driven projects.
- Enterprise Applications: Laravel’s modular and scalable architecture makes it suitable for developing large-scale enterprise applications with complex business logic and requirements.
- Startups and MVPs: Due to its rapid development capabilities and a wide range of built-in features, Laravel is often chosen for developing Minimum Viable Products (MVPs) and prototypes.
- Authentication and Authorization Systems: Laravel provides a built-in authentication system that simplifies the process of user authentication and authorization, making it easy to secure applications.
- Task Scheduling and Job Queues: Laravel’s task scheduling and job queue system (powered by technologies like Redis or Beanstalkd) are used for automating repetitive tasks and handling background processing.
Laravel’s popularity is attributed to its developer-friendly syntax, comprehensive documentation, and the active community that continuously contributes to its improvement.
What are feature of Laravel?
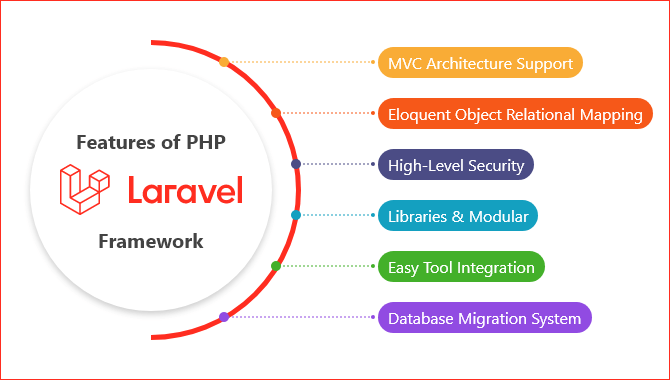
Features of Laravel:
- Eloquent ORM (Object-Relational Mapping): Laravel includes Eloquent, a powerful ORM that allows developers to interact with databases using an object-oriented syntax, making database operations more expressive and easier to manage.
- Blade Templating Engine: Laravel’s Blade templating engine provides a simple yet powerful way to create dynamic views in PHP. It allows for the creation of reusable templates and includes features like template inheritance and sections.
- Artisan Console: Laravel comes with a command-line interface called Artisan. Developers can use Artisan to perform various tasks such as database migrations, seed databases, generate boilerplate code, and more.
- Middleware: Laravel uses middleware to filter HTTP requests entering the application. This provides a mechanism to perform tasks like authentication, logging, and other filtering operations before the request reaches the application.
- Routing: Laravel provides a clean and expressive way to define web routes in the application. Routes can be defined in the
routes/web.php
file for web routes androutes/api.php
for API routes. - Authentication and Authorization: Laravel simplifies the process of implementing user authentication and authorization. It includes pre-built controllers, middleware, and methods for handling user registration, login, and access control.
- Laravel Mix: Laravel Mix is an asset compilation tool that streamlines the process of working with CSS and JavaScript. It provides a fluent API for defining webpack build steps for the application’s assets.
- Database Migrations and Seeding: Laravel’s migration system allows developers to version-control the database schema and share it with the team. Database seeding allows for the populating of database tables with sample or default data.
- Task Scheduling and Job Queues: Laravel provides a concise API for scheduling tasks to run at specified intervals. It also supports job queues, allowing developers to defer the execution of time-consuming tasks to improve application responsiveness.
- Testing Support: Laravel supports PHPUnit for writing and running tests. It includes convenient helper methods and classes for testing the application’s functionality, making it easier to ensure code reliability.
What is the workflow of Laravel?
The typical workflow of a Laravel application involves the following steps:
- Installation: Start by installing Laravel using Composer, the PHP dependency manager. The
composer create-project
command is commonly used to set up a new Laravel project. - Routing: Define the application’s routes in the
routes/web.php
file for web routes orroutes/api.php
for API routes. Specify the associated controllers and methods for handling each route. - Controllers: Create controllers to handle the logic associated with each route. Controllers are responsible for processing user input, interacting with models, and returning the appropriate response.
- Models: Define Eloquent models to interact with the database. Models represent database tables and are used to perform database operations such as querying, inserting, updating, and deleting records.
- Views: Use Blade templates to create dynamic views for the application. Views are responsible for presenting data to the user and can include HTML, PHP, and Blade syntax.
- Middleware: Implement middleware for filtering and processing HTTP requests. Middleware can perform tasks such as authentication, logging, and modifying the request or response.
- Database Migrations: Use migrations to create and modify database tables. Migrations are version-controlled and allow for easy collaboration among team members.
- Artisan Commands: Leverage Artisan commands to perform various tasks, including running migrations, generating boilerplate code, creating controllers, and more.
- Testing: Write tests using PHPUnit to ensure the correctness of the application’s functionality. Laravel provides convenient testing helpers and features for writing both unit and feature tests.
- Deployment: Deploy the Laravel application to a web server or hosting environment. Configure the server to serve the application, set up environment variables, and ensure proper file permissions.
Laravel’s workflow emphasizes convention over configuration, making it easy for developers to build robust and maintainable web applications. The framework provides a structured and organized approach to development, promoting best practices and scalability.
How Laravel Works & Architecture?
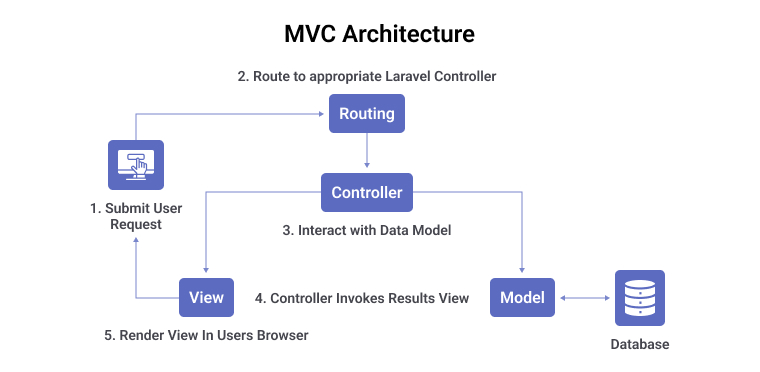
It provides a robust and elegant way to develop web applications with features like routing, database access, authentication, and more.
Following is a breakdown of how Laravel works and its architecture:
1. Core Principles:
- MVC Architecture: Separates application logic into models, views, and controllers for better organization and maintainability.
- Dependency Injection: Simplifies object management by injecting required dependencies into classes.
- Eloquent ORM: Provides a powerful and expressive object-relational mapper for interacting with databases.
- Artisan CLI: Command-line interface for managing application tasks and generating code.
- Service Providers: Offer modular architecture for organizing application components and services.
2. Architectural Components:
- Model: Represents data entities and interacts with the database through Eloquent ORM.
- View: Responsible for presenting the user interface and generates HTML using templates and Blade templating engine.
- Controller: Handles user requests, interacts with models, and directs the application flow.
- Routes: Define mappings between URLs and controller actions.
- Middleware: Intercepts requests and performs tasks like authentication and authorization before reaching controllers.
- Facades: Provide static methods for accessing Laravel functionalities without directly instantiating classes.
3. Workflow:
- User sends a request: This could be a browser navigating to a URL or submitting a form.
- Request reaches the router: The router matches the URL to a defined route.
- Route invokes the controller action: The controller action retrieves data from models, performs logic, and prepares data for the view.
- Controller returns a response: This is typically a Blade template with data to be rendered.
- View generates the HTML: The Blade template engine combines data with HTML and generates the final response sent to the user.
4. Benefits:
- Rapid Development: Laravel provides tools and features that speed up development by automating tasks and reducing boilerplate code.
- Security: Laravel offers built-in security features like CSRF protection and user authentication to protect your applications.
- Scalability: Laravel is designed to handle large and complex applications with features like caching and queueing.
- Community and Ecosystem: Laravel has a large and active community with numerous packages and extensions available to extend its functionalities.
Points to Remember:
- Understanding the MVC architecture and Laravel’s core principles is crucial for effective development.
- Utilize Laravel’s features like Eloquent ORM, Artisan CLI, and service providers to streamline development.
- Explore the extensive Laravel community and resources for learning, support, and inspiration.
By learning the fundamentals of Laravel and actively engaging with the community, you can unlock its full potential and build modern, secure, and scalable web applications with ease.
How to Install and Configure Laravel?
Installing and Configuring Laravel:
Let’s have a look at a step-by-step guide on installing and configuring Laravel:
1. Prerequisites:
- Web Server: Apache or Nginx are popular choices.
- PHP: Version 8.1 or later is recommended.
- Composer: Dependency management tool for PHP.
2. Installing Laravel:
- Composer Create-Project: Run the following command in your terminal:
composer create-project laravel/laravel your-project-name
- This will download and install Laravel into a directory named
your-project-name
.
3. Web Server Configuration:
- Apache: Modify your virtual host configuration to point the document root to the
public
directory of your Laravel project. - Nginx: Create a server block configuration with the same document root setting.
4. Database Configuration:
- Copy the
.env.example
file to.env
and edit the database settings according to your database configuration. - Run the following command to migrate and seed the database:
php artisan migrate --seed
5. Key Generation:
- Run the following command to generate an application key:
php artisan key:generate
6. Additional Configuration:
- Review the configuration files in the
config
directory and adjust settings as needed. - Consider installing additional packages using Composer for functionalities like authentication or social login.
- Explore the Laravel documentation for detailed configuration options and best practices.
Points to Remember:
- Choose the web server and database configuration that best suits your needs.
- Configure the
.env
file with your specific database credentials. - Generate an application key for security purposes.
- Consult the Laravel documentation for detailed instructions and troubleshooting assistance.
By following these steps and using available resources, you can efficiently install and configure Laravel to start building your web applications.
Fundamental Tutorials of Laravel: Getting started Step by Step
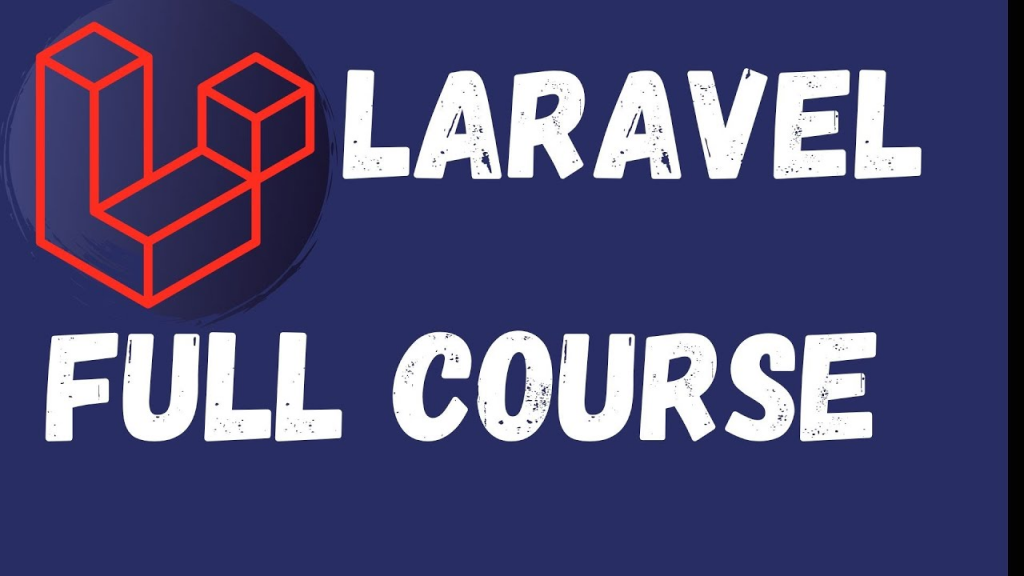
Let’s have a look at some step-by-step basic tutorials for learning Laravel:
1. Setting Up the Environment:
- Install PHP: Ensure you have PHP 8.1 or later installed.
- Install Composer: Composer is a dependency management tool for PHP. Download and install it following the official guide.
- Create a Project Directory: Create a directory for your Laravel project.
- Install Laravel: Open your terminal and navigate to the project directory. Run the following command to install Laravel:
composer create-project laravel/laravel your-project-name
2. Starting a Web Server:
- Apache: If you’re using Apache, configure your virtual host to point to the
public
directory of your Laravel project. - Nginx: If you’re using Nginx, create a server block configuration with the same document root setting.
- Start the web server: Once your web server is configured, start it.
3. Database Configuration:
- Create a Database: Create a database in your preferred database management system (e.g., MySQL, PostgreSQL).
- Copy
.env.example
: Copy the.env.example
file to.env
and edit the database settings with your database credentials. - Migrate and Seed Database: Run the following command to migrate and seed the database:
php artisan migrate --seed
4. Creating Your First Route:
- Open
routes/web.php
: This file defines routes for your web application. - Add a Route: Add a simple route to return a message. For example:
PHP
Route::get('/', function () {
return 'Hello, world!';
});
5. Creating Controllers and Views:
- Create a Controller: Run the following command to create a new controller:
php artisan make:controller HomeController
- Edit the Controller: Open the
HomeController
file and define a method to return a view. - Create a View: Create a new Blade template file in the
resources/views
directory. - Render the View: In your controller method, return the view name using the
view
function.
6. Using Eloquent ORM:
- Define Models: Create model classes to represent your database tables.
- Interact with Database: Use Eloquent methods to perform CRUD operations on your data.
- Display Data in Views: Access data from your models in your Blade templates.
7. Additional Features:
- Learn about authentication, authorization, and user management.
- Explore middleware to perform tasks before a request reaches the application.
- Use Laravel queues to process background tasks asynchronously.
- Utilize packages and libraries from the Laravel ecosystem to extend your application’s functionality.
Points to Remember:
- Start with the basics and gradually progress to more advanced topics.
- Practice building simple applications to solidify your understanding.
- Utilize online resources and tutorials for guidance and learning.
- Join online communities and forums to ask questions and learn from other developers.
By following these steps and actively engaging with the learning process, you can acquire the necessary skills and knowledge to effectively use Laravel and build robust and scalable web applications.
- PPG Industries: Selection and Interview process, Questions/Answers - April 3, 2024
- Fiserv: Selection and Interview process, Questions/Answers - April 3, 2024
- Estee Lauder: Selection and Interview process, Questions/Answers - April 3, 2024