What is LINQ?
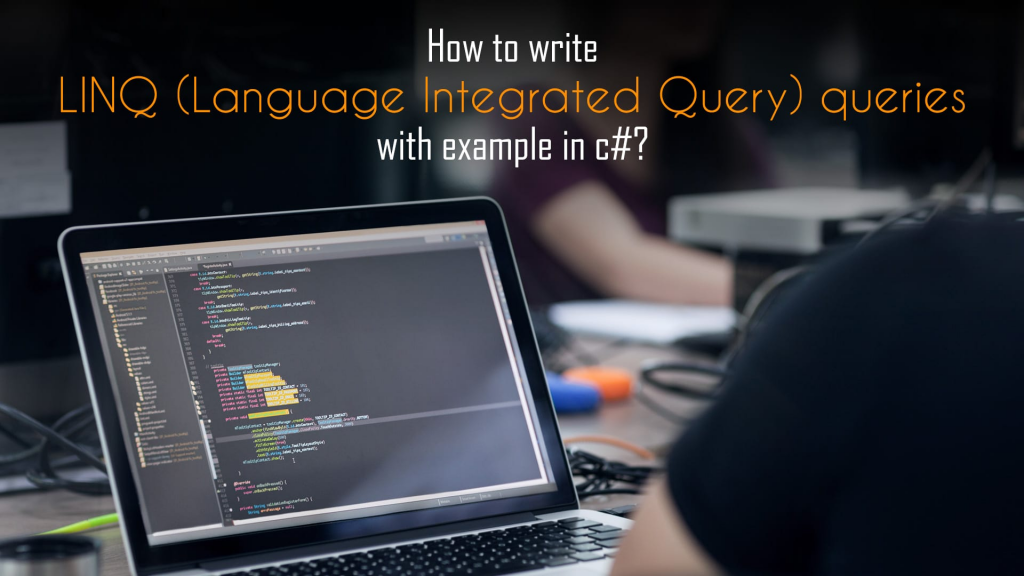
LINQ, or Language-Integrated Query, is a feature of Microsoft’s .NET framework that enables developers to write queries directly within the programming language (C# or Visual Basic) rather than using SQL or other query languages. LINQ provides a unified way to query and manipulate data from different types of data sources, including databases, XML, collections, and more. It is particularly associated with the LINQ to Objects, LINQ to SQL, and LINQ to XML implementations.
Key features of LINQ include:
- Integration with Programming Languages: LINQ is integrated into C# and Visual Basic, allowing developers to write queries using syntax that is similar to the host language. This integration provides a seamless experience for developers.
- Strongly Typed Queries: LINQ uses strongly typed queries, which means that the compiler can catch syntax and type errors at compile-time rather than runtime. This improves code reliability.
- Support for Different Data Sources: LINQ supports querying data from various sources, including in-memory objects (LINQ to Objects), databases (LINQ to SQL), XML documents (LINQ to XML), and other data providers.
- Declarative Syntax: LINQ queries use a declarative syntax, making the code more expressive and readable. Developers describe the desired result rather than specifying how to achieve it, allowing for a more natural representation of queries.
- Filtering, Sorting, and Grouping: LINQ supports a wide range of operations, including filtering, sorting, grouping, and aggregation. This makes it powerful for manipulating and shaping data.
- Deferred Execution: LINQ queries use deferred execution, meaning that the query is not executed immediately when it is defined. Instead, it is executed when the results are actually needed. This can lead to more efficient query execution.
What is top use cases of LINQ?
Top use cases of LINQ include:
- Data Retrieval and Manipulation:
- Use LINQ to retrieve and manipulate data from databases, making it easier to express queries directly in the programming language.
- In-Memory Object Queries (LINQ to Objects):
- Query and manipulate collections and arrays in memory using LINQ to Objects. This is particularly useful for working with data in memory without needing a database.
- XML Document Processing (LINQ to XML):
- Query and manipulate XML documents using LINQ to XML. LINQ simplifies the process of navigating, querying, and modifying XML structures.
- Database Querying (LINQ to SQL, Entity Framework):
- Use LINQ to SQL or LINQ with Entity Framework to query relational databases directly from code. This provides a more integrated and expressive way to work with databases.
- Data Transformation:
- Use LINQ to transform and project data from one form to another. LINQ’s expressive syntax makes it easy to define and apply transformations.
- Data Filtering and Aggregation:
- Employ LINQ for filtering, sorting, grouping, and aggregating data in a concise and readable manner.
- Data Binding:
- Use LINQ to bind data directly to user interface elements in applications, simplifying the process of updating and displaying data.
- Parallel Query Execution:
- In certain scenarios, LINQ queries can be parallelized to take advantage of multi-core processors, improving performance for large datasets.
LINQ is widely used in the Microsoft ecosystem, especially in C# and Visual Basic applications, to simplify data access and manipulation tasks. Its integration with the .NET framework and the ability to work with various data sources make it a versatile tool for developers.
What are feature of LINQ?
Features of LINQ:
- Integration with Programming Languages:
- LINQ is integrated into C# and Visual Basic, allowing developers to use native language constructs for querying and manipulating data.
- Strongly Typed Queries:
- LINQ queries are strongly typed, which means that the compiler can catch errors related to syntax and type mismatches at compile-time.
- Declarative Syntax:
- LINQ uses a declarative syntax, making queries more readable and expressive. Developers focus on specifying what they want rather than how to achieve it.
- Support for Various Data Sources:
- LINQ supports querying data from different sources such as databases (LINQ to SQL, Entity Framework), in-memory objects (LINQ to Objects), XML documents (LINQ to XML), and more.
- Standard Query Operators:
- LINQ provides a set of standard query operators (methods) that can be used across various data sources. These operators include
Where
,Select
,OrderBy
,GroupBy
,Join
, and more.
- LINQ provides a set of standard query operators (methods) that can be used across various data sources. These operators include
- Deferred Execution:
- LINQ queries use deferred execution, meaning that the query is not executed immediately upon creation. Execution is deferred until the results are actually needed.
- Implicitly Typed Variables:
- LINQ queries often use implicitly typed variables (
var
keyword), making the syntax more concise while preserving strong typing.
- LINQ queries often use implicitly typed variables (
- Anonymous Types:
- LINQ supports the creation of anonymous types, allowing developers to shape query results dynamically without creating explicit classes.
- Lambda Expressions:
- LINQ leverages lambda expressions for defining inline functions, providing a concise way to express transformations and predicates within queries.
- Concurrency Support:
- LINQ provides some support for concurrent query execution through the
AsParallel
andParallelEnumerable
classes, allowing for parallel processing of data.
- LINQ provides some support for concurrent query execution through the
What is the workflow of LINQ?
The workflow of using LINQ typically involves the following steps:
- Data Source Selection:
- Identify the data source you want to query. This could be a collection, array, database, XML document, or another LINQ-compatible data source.
- Data Source Access:
- Access the data source using the appropriate LINQ provider. For example, LINQ to Objects is used for in-memory collections, LINQ to SQL for databases, and LINQ to XML for XML documents.
- Query Construction:
- Construct a LINQ query using the standard query operators and syntax provided by LINQ. Define the criteria for selecting, filtering, sorting, or grouping data.
- Deferred Execution:
- Keep in mind that LINQ queries are lazily executed, meaning they are not executed until the results are needed. This allows for efficient query optimization.
- Query Execution:
- Execute the query to obtain the results. The execution can happen implicitly when iterating over the results or explicitly by using methods like
ToList()
,ToArray()
, orFirstOrDefault()
.
- Execute the query to obtain the results. The execution can happen implicitly when iterating over the results or explicitly by using methods like
- Result Processing:
- Process and use the results of the query according to your application’s requirements. This could involve further transformations, data binding, or presenting the data to users.
- Error Handling:
- Handle exceptions or errors that may occur during query execution, such as database connection issues, data format errors, or null references.
- Optimization:
- Consider optimizing your queries for performance, especially when dealing with large datasets. This might involve using appropriate indexing, caching, or parallel execution where applicable.
- Integration with Application Logic:
- Integrate the LINQ queries seamlessly into your application’s logic, ensuring that the queried data is used effectively to achieve the desired functionality.
By following this workflow, developers can leverage the features of LINQ to create expressive and efficient queries across various data sources within their applications.
How LINQ Works & Architecture?
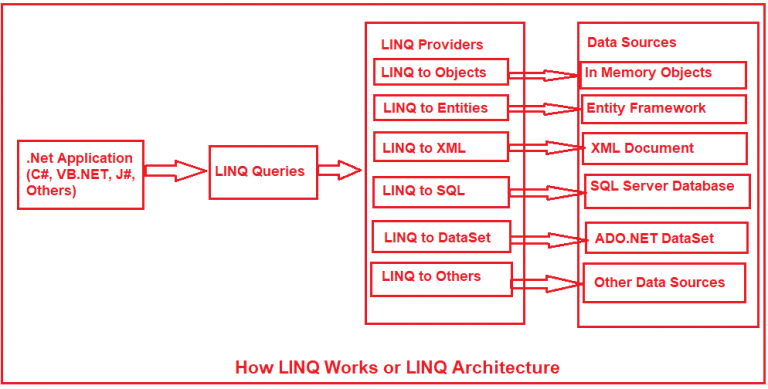
LINQ, short for Language Integrated Query, is a powerful technology in C# and VB.NET that allows you to query various data sources using familiar language syntax. Its underlying architecture revolves around three key components:
1. Query Expressions:
- LINQ queries are written in a syntax similar to C# or VB.NET expressions, making them intuitive and easy to understand.
- These expressions specify the data source to query, the data to retrieve, and any filtering or transformations to apply.
2. Query Providers:
- Different types of data sources (e.g., in-memory collections, databases, XML documents) require different approaches for querying.
- LINQ provides built-in provider implementations for common data sources (e.g.,
Enumerable
for in-memory collections,LinqToObjects
for objects,LinqToXml
for XML). - Custom providers can be developed to integrate LINQ with other data sources.
3. Expression Trees:
- LINQ queries are translated into internal representation called expression trees.
- These trees capture the logic of the query, including data source, filters, and transformations.
- The query provider then utilizes the expression tree to navigate and manipulate the specific data source efficiently.
Here’s how LINQ works in a nutshell:
- You write a LINQ query using familiar C# or VB.NET syntax.
- The compiler analyzes the query and translates it into an internal expression tree.
- The appropriate query provider receives the expression tree.
- The provider traverses the tree and executes the operations on the specific data source (e.g., filter, select, projection).
- The results of the query are returned in a format consistent with the query syntax.
Benefits of LINQ Architecture:
- Uniform Syntax: LINQ provides a single, consistent syntax for querying different data sources, reducing complexity and learning curve.
- Declarative Queries: You express what data you want, not how to obtain it, making the code more readable and maintainable.
- Lazy Execution: LINQ queries are often evaluated lazily, meaning data is retrieved and processed only when necessary, improving efficiency.
- Extensible: Custom providers allow querying additional data sources, extending LINQ’s reach beyond built-in options.
By understanding the core principles of LINQ’s architecture and its strengths, you can leverage this powerful technology to write concise, efficient, and expressive code for working with data in C# and VB.NET projects.
How to Install and Configure LINQ?
LINQ is not a standalone library that needs separate installation. It’s a core feature integrated into the .NET Framework (version 3.5 and later) and .NET Core. To use LINQ in your C# or VB.NET projects, follow these steps:
1. Target the Appropriate .NET Framework Version:
- Ensure your project targets a .NET Framework version that supports LINQ (3.5 or above).
- Check this in your project properties in Visual Studio or your preferred IDE.
2. Add Required References:
- For basic LINQ to Objects functionality, no additional references are needed.
- If you need LINQ to Entities (for databases), add a reference to
System.Data.Entity
. - For LINQ to XML, add a reference to
System.Xml.Linq
.
3. Use the using
Statements:
- Include the necessary namespaces in your code files to access LINQ features:
C#
using System.Linq; // For basic LINQ to Objects
using System.Linq.Expressions; // For advanced features
using System.Data.Entity; // For LINQ to Entities
using System.Xml.Linq; // For LINQ to XML
4. Start Writing LINQ Queries:
- Once the references and namespaces are in place, you can start writing LINQ queries directly in your code using familiar C# or VB.NET syntax.
Important Notes:
- LINQPad: If you want to experiment with LINQ queries without a full project setup, consider using LINQPad official site.
- Third-Party LINQ Providers: Explore third-party query providers for other data sources, such as NoSQL databases or REST APIs.
- Custom Providers: If you have specific data access needs, you can create custom LINQ providers to integrate with various data sources.
LINQ is a fundamental part of the .NET Framework and .NET Core, so you don’t need separate installations or complex configurations to start using it in your projects.
Fundamental Tutorials of LINQ: Getting started Step by Step
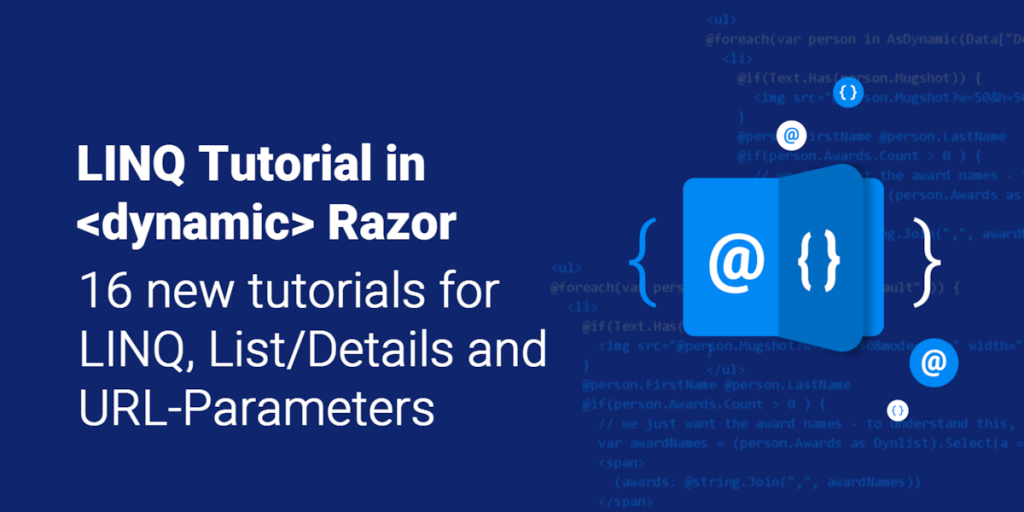
Following are Step-by-Step fundamental Tutorials of LINQ in C# (adaptable to VB.NET with minor syntax changes):
1. Introduction to LINQ:
- LINQ stands for Language Integrated Query and allows you to query various data sources using familiar C# syntax.
- It simplifies data manipulation and retrieval with expressions instead of traditional loops and conditional statements.
2. Working with Collections:
- Start by creating a simple list of integers:
C#
List<int> numbers = new List<int>() { 1, 5, 10, 15, 20 };
3. Filtering with Where
:
- Use the
Where
clause to filter elements based on a condition:
C#
List<int> evenNumbers = numbers.Where(x => x % 2 == 0).ToList();
// evenNumbers will now contain: 10, 20
4. Selecting with Select
:
- Use the
Select
clause to transform elements into new values:
C#
List<string> squares = numbers.Select(x => x * x).ToList();
// squares will now contain: 1, 25, 100, 225, 400
5. Ordering with OrderBy
:
- Use the
OrderBy
clause to sort elements based on a specified property:
C#
List<int> orderedNumbers = numbers.OrderBy(x => x).ToList();
// orderedNumbers will now be: 1, 5, 10, 15, 20
6. Combining Clauses:
- You can combine multiple clauses for more complex queries:
C#
List<int> largeEvenNumbers = numbers.Where(x => x > 10).Select(x => x * 2).ToList();
// largeEvenNumbers will now contain: 20, 40
7. Using Enumerable:
- For basic queries on in-memory collections, use the built-in
Enumerable
class:
C#
IEnumerable<int> filteredNumbers = Enumerable.Where(numbers, x => x % 3 == 0);
// filteredNumbers will be an enumeration of numbers divisible by 3
8. Working with Objects:
- LINQ can also query properties and methods of objects:
C#
class Student
{
public string Name { get; set; }
public int Grade { get; set; }
}
List<Student> students = new List<Student>
{
new Student { Name = "Alice", Grade = 20 },
new Student { Name = "Bob", Grade = 16 },
new Student { Name = "Charlie", Grade = 22 },
// Add more students as needed
};
List<string> adultNames = students
.Where(s => s.Grade > 18) // Update the property name in the LINQ query
.Select(s => s.Name)
.ToList();
Remember, these are just fundamental steps. As you learn more, you can tackle more complex data sources and manipulate data in powerful ways using LINQ!
- Mutual of Omaha: Selection and Interview process, Questions/Answers - April 15, 2024
- AES: Selection and Interview process, Questions/Answers - April 15, 2024
- Amphenol: Selection and Interview process, Questions/Answers - April 15, 2024