What is Maven?
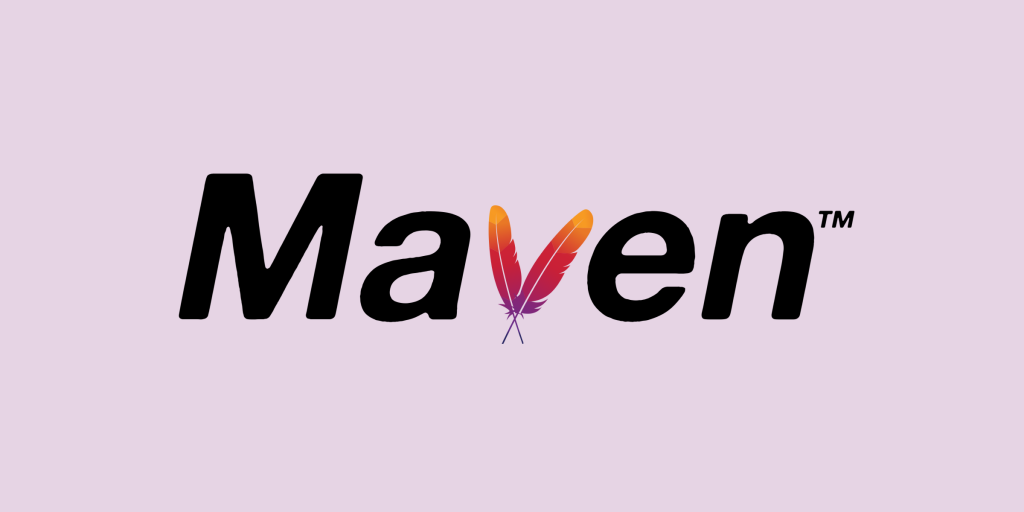
Maven is a powerful and widely-used build automation and project management tool. It simplifies the process of managing a software project’s build lifecycle, handling dependencies, and facilitating project documentation. Maven uses a declarative approach to project configuration and relies on conventions to streamline the build process.
Key features of Maven include:
- Build Lifecycle: Maven defines a standardized build lifecycle with phases such as compile, test, package, install, and deploy. This makes it easy to understand and manage the build process.
- Dependency Management: Maven manages project dependencies, automatically downloading and resolving libraries and frameworks required for the project. It simplifies dependency management and helps ensure consistency across development environments.
- Convention over Configuration: Maven follows the principle of convention over configuration, reducing the need for extensive build configurations. It uses default configurations based on project conventions, but developers can customize settings when needed.
- Plugins: Maven supports a rich ecosystem of plugins that extend its functionality. Plugins can be used to perform various tasks, such as code generation, documentation generation, and deployment to different environments.
- Project Object Model (POM): Maven uses a Project Object Model (POM) file, typically named
pom.xml
, to define project configuration, dependencies, and other settings. The POM file is at the core of Maven-based projects. - Transitive Dependency Resolution: Maven automatically resolves and downloads transitive dependencies—dependencies required by other dependencies. This ensures that the project has all the necessary libraries to build and run.
- Consistent Project Structure: Maven enforces a consistent project structure, making it easier for developers to navigate and understand the layout of a project.
- Integration with IDEs: Maven integrates seamlessly with popular integrated development environments (IDEs) such as Eclipse, IntelliJ IDEA, and NetBeans. This ensures a consistent development experience across different tools.
What is top use cases of Maven?
Top use cases of Maven include:
- Java Project Builds:
- Maven is widely used for building and managing Java projects. It automates tasks like compilation, testing, packaging, and dependency management for Java applications.
- Dependency Management:
- Maven excels at managing project dependencies, allowing developers to declare dependencies in the POM file. This simplifies the process of including third-party libraries in a project.
- Continuous Integration:
- Maven is often used in conjunction with continuous integration (CI) tools such as Jenkins, Travis CI, or GitLab CI to automate the build and testing processes in a CI/CD pipeline.
- Web Application Development:
- Maven is commonly used for building web applications, including those based on Java frameworks like Spring or servlet-based applications.
- Automated Testing:
- Maven integrates with testing frameworks, making it easier to automate the execution of unit tests, integration tests, and other types of testing within a project.
- Documentation Generation:
- Maven can be used to generate project documentation, including reports, by utilizing plugins like Maven Site and Maven Surefire Report.
- Release Management:
- Maven provides release management capabilities through plugins like Maven Release Plugin, enabling developers to create releases, tag versions, and update project versions.
- Multi-Module Projects:
- Maven supports multi-module projects, allowing developers to manage and build multiple interdependent projects as a single unit.
Maven’s versatility and widespread adoption have made it a standard tool in the Java development ecosystem, and it is also used in projects beyond the Java ecosystem in some cases.
What are feature of Maven?
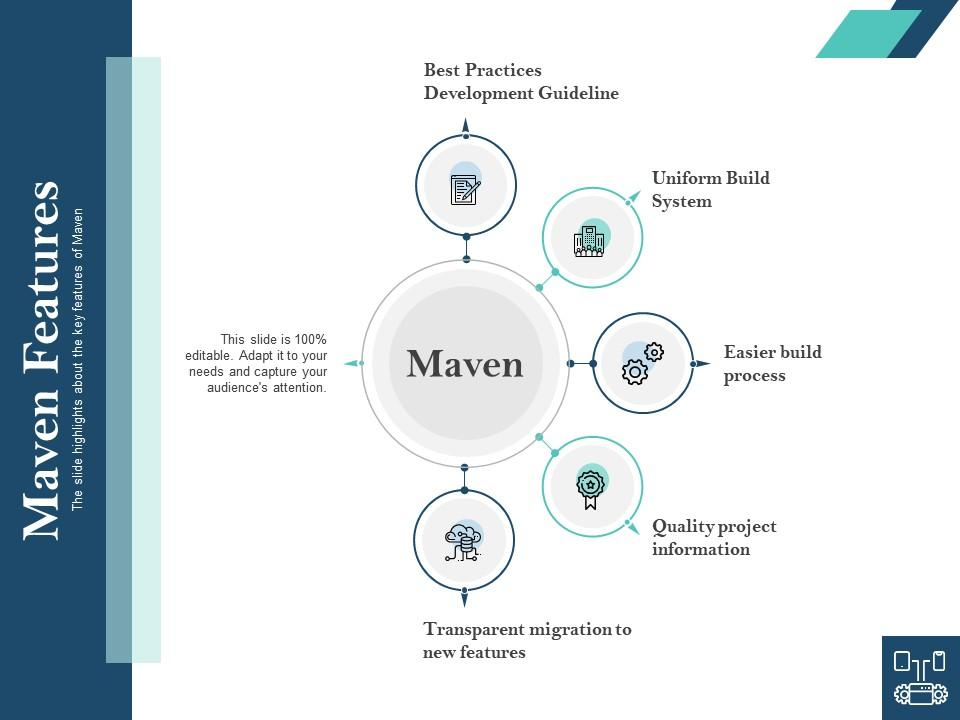
Maven, a widely used build automation and project management tool, comes with several features that streamline the software development process. Below are key features of Maven along with a typical workflow:
- Build Lifecycle:
- Maven defines a standardized build lifecycle consisting of phases (e.g., compile, test, package, install, deploy). Each phase represents a step in the software development and deployment process.
- Project Object Model (POM):
- Maven uses a Project Object Model (POM) file (
pom.xml
) to configure and describe the project. This XML file includes project metadata, dependencies, build settings, and plugins.
- Maven uses a Project Object Model (POM) file (
- Dependency Management:
- Maven simplifies dependency management by automatically downloading and handling project dependencies. It supports transitive dependencies, ensuring that all required libraries are available.
- Convention over Configuration:
- Maven follows the convention over configuration paradigm, providing default configurations based on project conventions. This reduces the need for extensive build configurations.
- Plugin System:
- Maven’s plugin architecture allows developers to extend its functionality. There are numerous plugins available for various tasks, such as compilation, testing, packaging, and more.
- Consistent Project Structure:
- Maven enforces a consistent project structure, making it easier for developers to understand and navigate projects. Standardized directory layouts help maintain a clean and organized codebase.
- Repository System:
- Maven uses a repository system to store and retrieve project artifacts (JARs, WARs, etc.). It supports local repositories for development and remote repositories for sharing and distributing artifacts.
- Integration with IDEs:
- Maven integrates seamlessly with popular integrated development environments (IDEs) such as Eclipse, IntelliJ IDEA, and NetBeans. IDEs can import Maven projects and leverage Maven’s build capabilities.
- Dependency Scoping:
- Maven supports different dependency scopes, such as compile, test, provided, and runtime. These scopes define when dependencies are required during the build and runtime processes.
- Effective Pom:
- Maven provides an “Effective POM” feature, allowing developers to view the effective configuration of a project after inheriting settings from parent POMs and applying profiles.
What is the workflow of Maven?
Let’s have a look at some Maven Workflow:
- Project Initialization:
- Create a new Maven project using the
mvn archetype:generate
command or an integrated development environment (IDE) with Maven support.
- Create a new Maven project using the
- Edit POM File:
- Configure the project by editing the
pom.xml
file. Define project metadata, dependencies, plugins, and other settings.
- Configure the project by editing the
- Build Lifecycle:
- Run Maven build commands to execute specific phases of the build lifecycle (e.g.,
mvn clean
,mvn compile
,mvn test
,mvn package
).
- Run Maven build commands to execute specific phases of the build lifecycle (e.g.,
- Dependency Management:
- Maven automatically resolves and downloads project dependencies, making it unnecessary for developers to manage libraries manually.
- Customize Build Process:
- Customize the build process by configuring plugins and defining goals. Maven plugins extend functionality and execute tasks during specific phases.
- Run Tests:
- Execute tests using Maven’s built-in test framework or integrate with external testing frameworks. The
mvn test
command runs unit tests.
- Execute tests using Maven’s built-in test framework or integrate with external testing frameworks. The
- Package Artifacts:
- Package the project artifacts (e.g., JAR, WAR) using the
mvn package
command. Maven produces the packaged artifacts in thetarget
directory.
- Package the project artifacts (e.g., JAR, WAR) using the
- Install Artifacts Locally:
- Install the project artifacts in the local Maven repository using the
mvn install
command. This makes the artifacts available for other projects on the same machine.
- Install the project artifacts in the local Maven repository using the
- Deploy Artifacts:
- Deploy artifacts to a remote repository or artifact repository manager using the
mvn deploy
command. This step is typically done for release versions.
- Deploy artifacts to a remote repository or artifact repository manager using the
- Continuous Integration:
- Integrate Maven into continuous integration (CI) systems such as Jenkins, Travis CI, or GitLab CI to automate the build and test processes.
- Documentation Generation:
- Generate project documentation using the
mvn site
command. Maven generates reports and documentation based on configured plugins.
- Generate project documentation using the
- Release Management:
- Manage project releases using the Maven Release Plugin. This includes versioning, tagging, and updating the project version for the next development cycle.
Understanding and following this workflow helps developers effectively use Maven for building, managing dependencies, and automating various aspects of the software development process.
How Maven Works & Architecture?
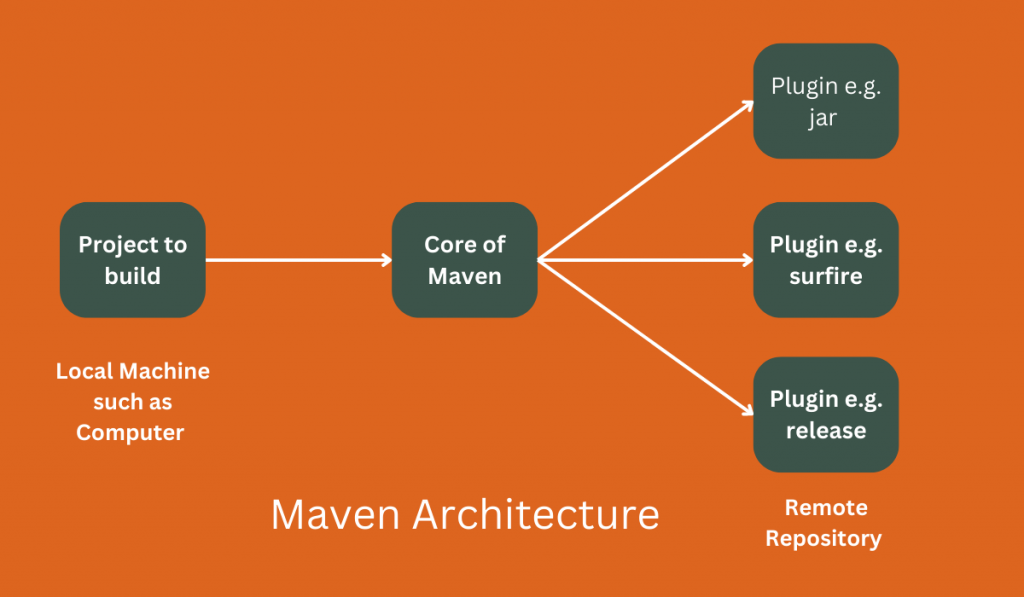
Maven is a popular build automation tool for Java projects. It helps streamline the development process by automating repetitive tasks like compiling, testing, packaging, and deploying software. Its architecture revolves around:
Key Components:
- Project Object Model (POM): The central configuration file describing the project, including dependencies, build phases, and plugins.
- Maven Repository: A central repository where libraries and artifacts are stored and can be retrieved by projects. The most popular is Maven Central, although organizations can have their own internal repositories.
- Lifecycle Phases: A predefined sequence of stages performed during a build, such as “clean”, “compile”, “test”, “package”, “deploy”, etc.
- Plugins: Reusable modules extending Maven’s functionalities for tasks like code generation, continuous integration, or custom build steps.
Workflow:
- Define Project in POM: Developers specify project details, dependencies, and build configurations in the POM file.
- Resolve Dependencies: Maven downloads all required libraries from the repositories according to the POM’s dependency declarations.
- Execute Lifecycle Phases: Each phase in the Maven lifecycle is executed sequentially based on the specified command or build trigger.
- Perform Specific Actions: Plugins are invoked depending on the phase to carry out tasks like compiling code, running tests, generating documentation, or deploying the application.
- Generate Artifacts: The final stages result in build artifacts like JAR files or WAR files (depending on the project type) ready for deployment or further processing.
Benefits of Maven Architecture:
- Standardization: Defines a consistent development and build process across projects, reducing complexity and errors.
- Modularity: Plugins provide granular control over build functionalities, allowing customization and extension.
- Reusability: POM files and plugins leverage shared configurations and best practices, improving efficiency.
- Transparency: The clear structure and defined phases simplify build process understanding and troubleshooting.
By understanding how Maven works and its architecture, you can leverage its features and benefits to optimize your Java development process.
How to Install and Configure Maven?
Following are the steps on how to install and configure Maven:
1. Download Maven:
- Visit the official Maven website and download the latest binary distribution for your operating system.
2. Extract Files:
- Extract the downloaded archive to a suitable location on your system, such as
C:\Program Files\Maven
on Windows or/usr/local/apache-maven
on Linux/macOS.
3. Set Environment Variables:
Windows:
- Right-click “This PC” or “My Computer” and select “Properties.”
- Move to “Advanced system settings” and press “Environment Variables.”
- Under “System variables,” press “New”:
- Variable name:
MAVEN_HOME
- Variable value: The path to your Maven installation directory (e.g.,
C:\Program Files\Maven
)
- Variable name:
- Edit the “Path” variable under “System variables” and append
;%MAVEN_HOME%\bin
to the end.
Linux/macOS:
- Open a terminal and edit your shell profile file (e.g.,
.bashrc
or.zshrc
). - Add the following lines: Bash
export MAVEN_HOME=/usr/local/apache-maven (replace with your Maven installation path) export PATH=$PATH:$MAVEN_HOME/bin
- Save the changes and reload the profile using
source ~/.bashrc
orsource ~/.zshrc
.
4. Verify Installation:
- Open a command prompt or terminal and type
mvn -v
. If Maven is installed correctly, you’ll see its version information.
5. Configure Settings (Optional):
- Maven’s default settings are located in
MAVEN_HOME/conf/settings.xml
. You can customize settings like local repository location, proxy settings, and profiles here.
6. Create a Maven Project:
- Use the
mvn archetype:generate
command to create a new Maven project structure with a POM file.
7. Build Your Project:
- Use the
mvn compile
command to compile your project’s source code. - Use the
mvn package
command to create a distributable package (e.g., a JAR file). - Explore other Maven commands for tasks like testing, running, and deploying your application.
Important Tips:
- Consider using an integrated development environment (IDE) with Maven support for a more streamlined development experience.
- Keep your Maven installation up-to-date to benefit from bug fixes and new features.
- Explore the extensive Maven documentation and resources available online for further learning and troubleshooting.
Fundamental Tutorials of Maven: Getting started Step by Step
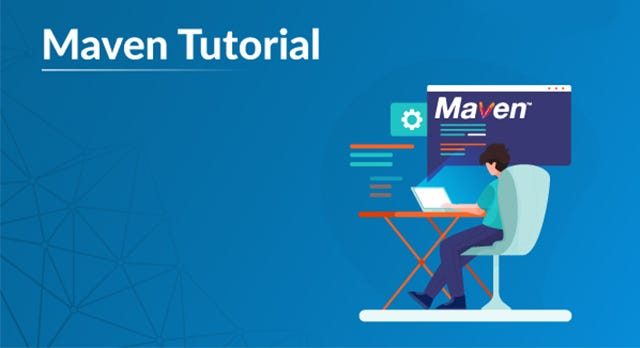
Following are the Step-by-Step Fundamental Tutorials of Maven:
1. Installing and Configuring Maven:
- Download Maven: Go to the maven official site and download the latest binary distribution for your operating system.
- Extract Files: Extract the downloaded archive to a suitable location, like
C:\Program Files\Maven
(Windows) or/usr/local/apache-maven
(Linux/macOS). - Set Environment Variables:Windows:
- Right-click “This PC” or “My Computer” and select “Properties.”
- Go to “Advanced system settings” and click “Environment Variables.”
- Under “System variables,” click “New”:
- Variable name:
MAVEN_HOME
- Variable value: Your Maven installation directory (e.g.,
C:\Program Files\Maven
)
- Variable name:
- Edit the “Path” variable under “System variables” and append
;%MAVEN_HOME%\bin
to the end.
- Open a terminal and edit your shell profile file (e.g.,
.bashrc
or.zshrc
). - Add the following lines: Bash
export MAVEN_HOME=/usr/local/apache-maven (replace with your path) export PATH=$PATH:$MAVEN_HOME/bin
- Save the changes and reload the profile using
source ~/.bashrc
orsource ~/.zshrc
.
- Verify Installation: Open a command prompt or terminal and type
mvn -v
. If Maven is installed correctly, you’ll see its version information.
2. Creating a Simple Maven Project:
- Open a terminal in your desired project directory.
- Run the command:
mvn archetype:generate
- Choose an archetype (e.g., “maven-java-web-app”) and follow the prompts to customize your project details.
- This will create a basic project structure with a POM file (
pom.xml
) containing project configurations.
3. Understanding the POM File:
The POM file is the heart of your Maven project. It defines important aspects like:
- Project name and version
- Dependencies on other libraries
- Build lifecycle phases (e.g., compile, test, package)
- Plugins for specific tasks (e.g., code generation)
4. Building and Running the Project:
- In your project directory, run the command:
mvn compile
to compile your code. - Run the command:
mvn package
to create a distributable package (e.g., a JAR file). - The specific execution command for your project type might differ, refer to your project documentation or tutorials.
5. Exploring Further:
- Learn about common Maven commands like
mvn clean
,mvn test
, andmvn install
. - Discover useful plugins for tasks like generating documentation, running unit tests, or deploying your application.
- Check out online resources like the official Maven documentation and tutorials for a deeper understanding.
Important Notes:
- Consider using an IDE with Maven support for a more user-friendly development experience.
- Keep your Maven installation up-to-date for bug fixes and new features.
- Practice creating different types of Maven projects to solidify your understanding.
This is a fundamental introduction to Maven. Remember, exploring and practicing on your own is key to mastering this valuable tool!
- Mutual of Omaha: Selection and Interview process, Questions/Answers - April 15, 2024
- AES: Selection and Interview process, Questions/Answers - April 15, 2024
- Amphenol: Selection and Interview process, Questions/Answers - April 15, 2024