What is .NET?
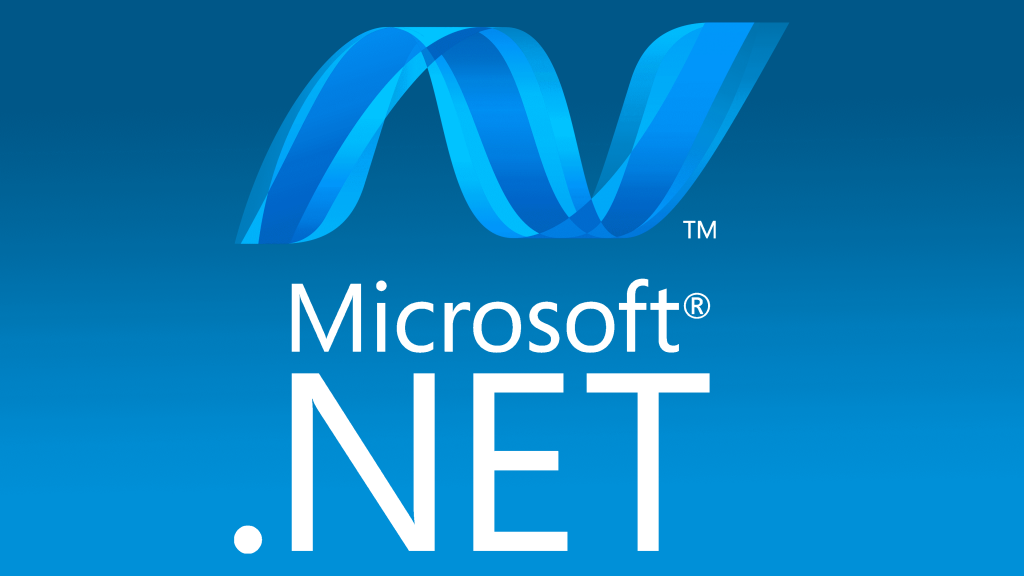
.NET is a software framework developed by Microsoft that allows developers to create applications and services for different platforms. It provides a framework and tools for building various types of applications, including web applications, desktop applications, mobile apps, cloud-based services, and more. .NET supports multiple programming languages, including C#, F#, and Visual Basic, allowing developers to choose the language that best suits their project.
Key components of the .NET platform include:
- Common Language Runtime (CLR): This is the runtime environment that manages the execution of .NET code. It provides features like memory management, security, and exception handling.
- .NET Framework: This is the original implementation of .NET and is primarily used for building Windows desktop applications. It includes a vast class library for common programming tasks.
- .NET Core: .NET Core is a cross-platform, open-source framework that is used for building modern, high-performance applications that can run on Windows, macOS, and Linux. It has since evolved into .NET 5 and later .NET 6, which are part of the unified .NET platform.
- ASP.NET: ASP.NET is a web framework within the .NET ecosystem for building web applications, including dynamic web pages and web APIs.
- Xamarin: Xamarin is a set of tools and libraries for building native mobile applications for iOS and Android using C# and .NET.
- Entity Framework: This is an Object-Relational Mapping (ORM) framework that simplifies database access and management in .NET applications.
- .NET Core/.NET 5 and Later: These versions of .NET are particularly well-suited for building modern web applications, microservices, and cloud-based applications.
What is top use cases of .NET?
- Web Development: ASP.NET is widely used for building dynamic web applications, ranging from small websites to large-scale web applications.
- Desktop Applications: .NET can be used to develop Windows desktop applications using technologies like Windows Presentation Foundation (WPF) and Windows Forms.
- Mobile App Development: Xamarin allows developers to create cross-platform mobile apps using .NET, which can run on both iOS and Android devices.
- Cloud-Based Applications: .NET Core and .NET 5/6 are designed for building cloud-native applications that can be easily deployed to platforms like Azure, AWS, and Google Cloud.
- Microservices: .NET is well-suited for building microservices-based architectures, enabling the development of scalable and modular applications.
- Game Development: Unity, a popular game development engine, uses C# and .NET for game development.
- IoT (Internet of Things): .NET can be used in IoT applications, providing connectivity and data processing capabilities for IoT devices.
- Machine Learning: .NET provides libraries and frameworks like ML.NET for machine learning and data science applications.
- Cross-Platform Development: With .NET 5 and later, you can write cross-platform applications that run on Windows, macOS, and Linux, making it suitable for a wide range of scenarios.
- Enterprise Applications: Many large enterprises use .NET for building robust and scalable enterprise-level applications due to its stability, security, and extensive tooling.
.NET’s versatility and the broad range of technologies it encompasses make it a popular choice for a variety of application development needs. The choice of which .NET variant to use depends on factors like platform compatibility, project requirements, and development team preferences.
What are the features of .NET?
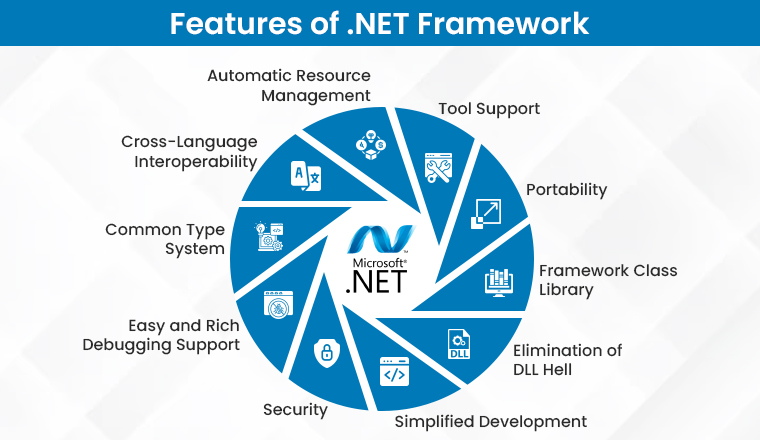
.NET is a versatile and feature-rich software development platform. Some of its key features:
- Language Independence: .NET supports multiple programming languages, including C#, F#, and Visual Basic, allowing developers to choose the language that best suits their project and team’s expertise.
- Common Language Runtime (CLR): The CLR is the runtime environment of .NET. It provides features like automatic memory management (garbage collection), security, and exception handling. It also enables interoperability between different .NET languages.
- Unified Class Library: .NET provides a comprehensive class library (also known as the Base Class Library or BCL) that contains reusable classes and components for common programming tasks, reducing the need to write repetitive code.
- Cross-Platform Development: With .NET 5 and later (.NET 6 at the time of my last update), .NET is cross-platform, allowing developers to build applications that run on Windows, macOS, and Linux.
- Open-Source: .NET Core and later versions are open-source, making it accessible to a broader community of developers and allowing for contributions and extensions.
- ASP.NET for Web Development: ASP.NET is a powerful framework for building web applications, including support for building dynamic web pages, web APIs, and real-time applications.
- Xamarin for Mobile Development: Xamarin allows developers to build cross-platform mobile applications for iOS and Android using .NET languages, sharing code across platforms.
- Entity Framework: This ORM framework simplifies database access by providing an object-oriented way to interact with databases, reducing the need for writing SQL queries.
- High Performance: .NET Core and later versions are designed for high performance, making them suitable for building scalable and efficient applications.
- Tooling: .NET offers a rich set of development tools, including Visual Studio (for Windows), Visual Studio Code (cross-platform), and various command-line tools for building, testing, and deploying .NET applications.
- Security: .NET has built-in security features, including code access security, role-based security, and support for secure communication protocols.
- Version Compatibility: .NET provides a mechanism for side-by-side versioning, which allows multiple versions of the runtime and libraries to coexist on the same machine. This ensures that older applications continue to run correctly even as new versions of .NET are installed.
What is the workflow of .NET?
Here’s a simplified workflow for developing applications with .NET:
- Choosing a .NET Variant: Depending on your application’s requirements and target platforms, you choose the appropriate .NET variant. This could be .NET Framework for Windows desktop apps, .NET Core (or .NET 5/6) for cross-platform or web apps, or Xamarin for mobile app development.
- Development: You write your application code using a .NET-compatible programming language (e.g., C#, F#, Visual Basic) in an integrated development environment (IDE) like Visual Studio or Visual Studio Code.
- Compilation: The code is compiled into Intermediate Language (IL) code, which is platform-independent and can run on the Common Language Runtime (CLR).
- Runtime Execution: The IL code is executed by the CLR, which manages memory, security, and other runtime aspects.
- Testing and Debugging: You use debugging tools to test and refine your code, ensuring it behaves as expected.
- Deployment: Depending on your target platform, you package your application for deployment. This could involve creating installers, Docker containers, or cloud-based deployment packages.
- Monitoring and Maintenance: After deployment, you monitor your application’s performance and handle maintenance tasks, such as updates and patches.
- Scaling: As your application grows, you can scale it horizontally or vertically to handle increased load and demand.
- Optimization: Continuously optimize your code and infrastructure for better performance, scalability, and security.
The exact workflow may vary depending on the type of application and development practices, but these steps provide a general overview of the .NET development process.
How .NET Works & Architecture?
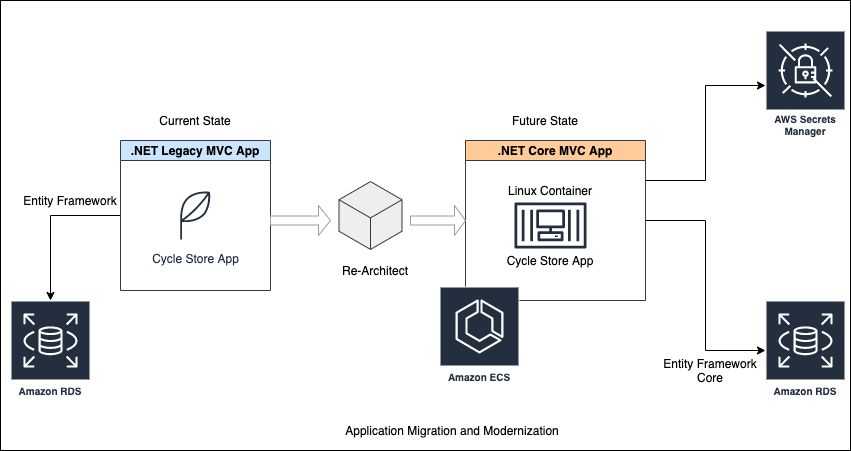
The following are the information on how .NET works and its architecture, as well as how to install and configure it:
How .NET Works
.NET is a software development platform that enables developers to build a variety of applications, including web applications, mobile applications, and desktop applications. It is a modular platform that consists of the following components:
- The Common Language Runtime (CLR): The CLR is a virtual machine that executes .NET applications. It is responsible for loading and running the code, managing memory, and providing security.
- The Framework Class Library (FCL): The FCL is a library of classes that can be used to create .NET applications. It includes classes for things like data structures, input/output, and networking.
- The .NET Framework: The .NET Framework is a set of APIs that provide additional functionality to .NET applications. It includes APIs for things like web development, database access, and graphics.
.NET Architecture
The .NET architecture is divided into two main layers: the runtime layer and the framework layer.
- The runtime layer is responsible for executing .NET applications. It consists of the CLR and the garbage collector.
- The framework layer is a library of classes that can be used to create .NET applications. It consists of the FCL and the .NET Framework.
How to Install and Configure .NET?
To install .NET, you can apply these steps:
- Go to the .NET download page: https://dotnet.microsoft.com/download/dotnet/latest and select the installer for your operating system.
- Run the installer and follow the instructions.
- Once the installer is finished, .NET will be installed on your computer.
To configure .NET, you may need to set environment variables or add .NET to your path. You can explore more information about configuring .NET in the .NET documentation: https://docs.microsoft.com/en-us/dotnet/.
To create a .NET application, you can use a variety of tools. Some popular tools include:
- Visual Studio: Visual Studio is a comprehensive IDE that includes everything you need to create, debug, and deploy .NET applications.
- Visual Studio Code: Visual Studio Code is a lightweight editor that is popular among .NET developers. It is a good choice if you are looking for a more lightweight option.
- MonoDevelop: MonoDevelop is a free and open-source IDE that can be used to create .NET applications on Linux and macOS.
Fundamental Tutorials of .NET: Getting Started Step by Step
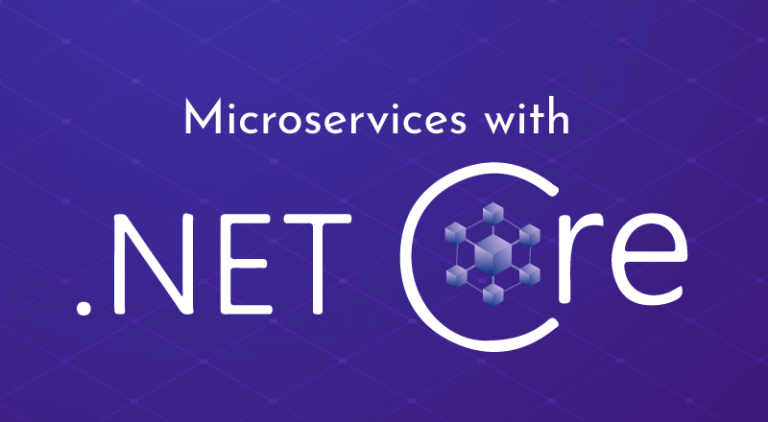
The following steps are the fundamental tutorials of .NET:
- Create a simple .NET application
To create a simple .NET application, you can follow these steps:
1. Open a text editor and create a new file with the .cs extension.
2. In the file, add the below code:
using System;
public class HelloWorld
{
public static void Main(string[] args)
{
Console.WriteLine("Hello, world!");
}
}
- Save the file as
HelloWorld.cs
. - Open Visual Studio or another .NET IDE.
- Generate a new project and choose the “Console App” template.
- In the project, add the
HelloWorld.cs
file that you created. - Run the project.
This will create a simple console application that prints the text “Hello, world!” to the console.
- Write code in .NET
.NET applications are written in a variety of languages, including C#, Visual Basic, and F#. The syntax of these languages is similar to the syntax of Java and C++.
Example of a simple C# program:
using System;
public class HelloWorld
{
public static void Main(string[] args)
{
Console.WriteLine("Hello, world!");
}
}
This program prints the text “Hello, world!” to the console.
- Running a .NET application
To run a .NET application, you can use the .NET command-line tools or a development environment like Visual Studio.
To run a .NET application from the command line, you can use the following command:
dotnet run
This will run the current project.
To run a .NET application from Visual Studio, you can press F5.
- Deploying a .NET application
To deploy a .NET application, you can use a variety of methods, including:
* Distributing the application as an executable file.
* Deploying the application to a web server.
* Deploying the application to a cloud service.
- Troubleshooting .NET applications
If you have problems with your .NET application, you can use the .NET debugger to step through the code and identify the problem.
To use the .NET debugger, you can press F11 to step into the next line of code, F5 to run the application, and F10 to step over the next line of code.
- Why Can’t I Make Create A New Folder on External Drive on Mac – Solved - April 28, 2024
- Tips on How to Become a DevOps Engineer - April 28, 2024
- Computer Programming Education Requirements – What You Need to Know - April 28, 2024