What is Node.js?
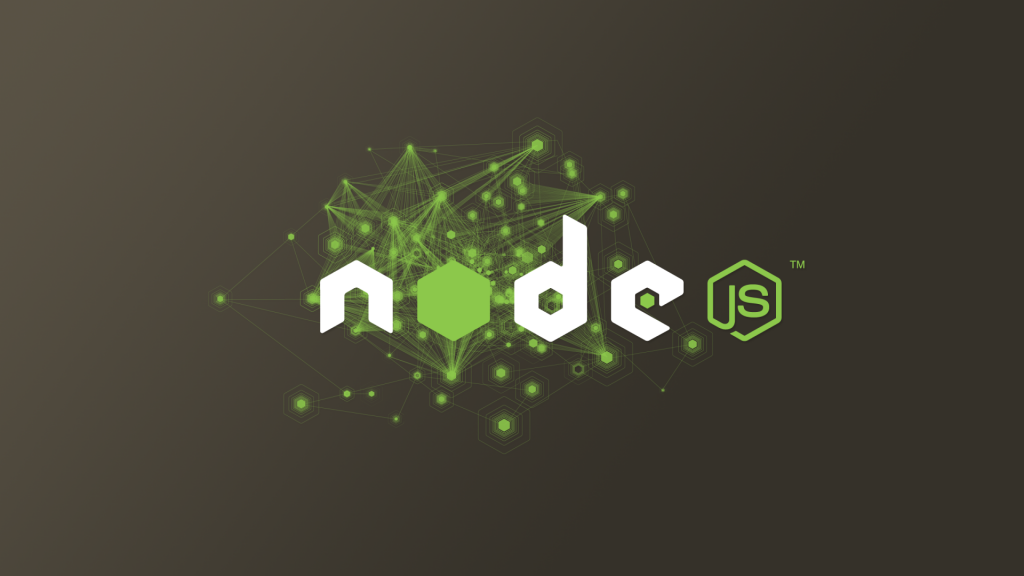
Node.js is an open-source, cross-platform, JavaScript runtime environment that executes JavaScript code outside a web browser. It allows developers to execute JavaScript code on the server, outside of the browser context. This enables building scalable and high-performance network applications, as Node.js is designed to handle asynchronous I/O operations efficiently.
Top Use Cases of Node.js:
- Web Applications: Node.js is commonly used to build real-time web applications, including social networking sites, online gaming platforms, and collaborative tools.
- API Development: Node.js is suitable for creating APIs that handle large numbers of concurrent connections efficiently.
- Single-Page Applications (SPAs): Node.js can serve as the backend for SPAs, managing data and handling server-side rendering.
- Streaming Services: Node.js is excellent for building streaming platforms that deliver audio, video, or live events.
- Microservices: Its lightweight and modular nature makes Node.js a good choice for creating microservices architecture.
- Real-Time Chat Applications: Node.js’s event-driven model makes it ideal for building real-time chat applications.
- IoT Applications: Node.js is used for building server-side applications that interact with Internet of Things (IoT) devices.
- Content Management Systems (CMS): Node.js can power the backend of content management systems and blogging platforms.
- Collaborative Tools: Applications like collaborative document editing or project management tools can benefit from Node.js’s real-time capabilities.
- E-commerce Platforms: Node.js can handle concurrent user interactions on e-commerce sites efficiently.
What are the features of Node.js?
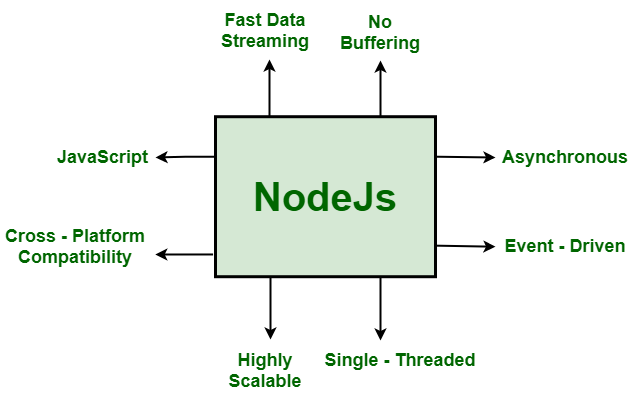
- Non-Blocking I/O: Node.js uses an event-driven, non-blocking I/O model, making it highly efficient for handling asynchronous operations.
- Scalability: Node.js applications can handle a large number of concurrent connections with minimal resource consumption.
- Fast Execution: Node.js’s use of the V8 engine results in high-performance execution of JavaScript code.
- NPM (Node Package Manager): NPM provides a vast ecosystem of open-source libraries and packages that can be easily integrated into Node.js applications.
- Cross-Platform: Node.js is compatible with multiple operating systems, allowing developers to build applications that work seamlessly on various platforms.
- Community and Modules: Node.js has a large and active community that contributes to its growth, providing a wide range of modules and libraries.
- Real-Time Capabilities: Node.js is well-suited for building real-time applications thanks to its event-driven architecture.
- Lightweight and Fast: Node.js is lightweight, enabling quick startup times and efficient memory usage.
- Open Source: Node.js is open source and free to use, making it accessible to developers and organizations.
What is the workflow of Node.js?
- Setup and Configuration: Install Node.js and necessary modules using NPM.
- Code Development: Write JavaScript code for your application.
- Module Management: Utilize external modules and libraries using NPM.
- Application Logic: Build the core logic of your application, handling data, interactions, and business rules.
- Routing and Middleware: Define routes and use middleware for handling requests and responses.
- Data Handling: Manage data storage, retrieval, and manipulation using databases or other data sources.
- Testing: Write and run tests to ensure the correctness of your application’s functionality.
- Deployment: Deploy your application to a server or cloud environment for public access.
- Monitoring and Optimization: Monitor application performance, analyze bottlenecks, and optimize for better efficiency.
- Maintenance: Regularly update dependencies, handle bug fixes, and introduce new features as needed.
How Node.js Works & Architecture?
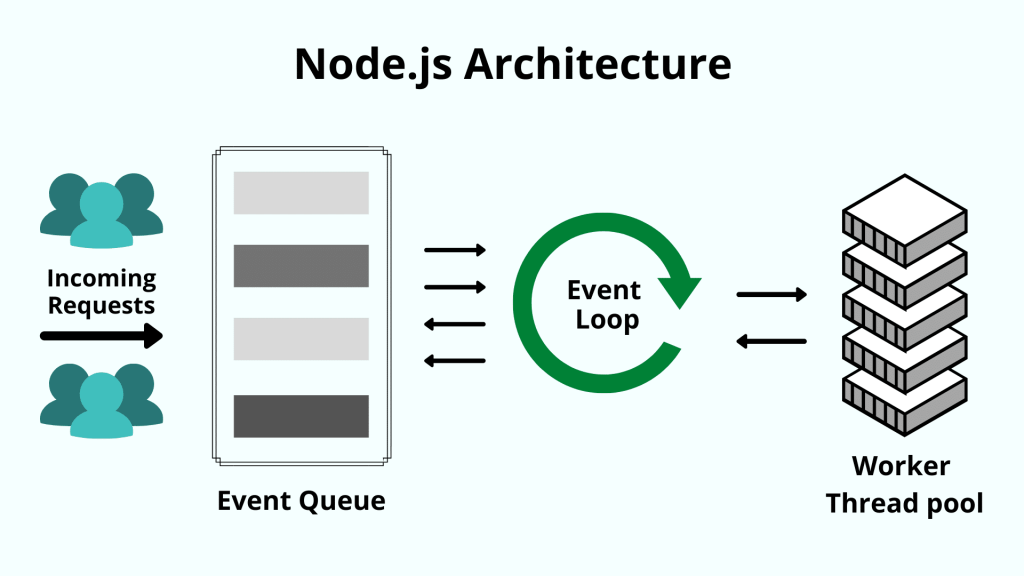
Node.js uses an event-driven, non-blocking architecture that allows it to handle multiple concurrent connections without blocking the execution of other tasks. When an asynchronous operation (e.g., reading from a file or making a network request) is initiated, Node.js continues executing other code while waiting for the operation to complete. Once the operation is finished, a callback function is executed.
Event Loop: The event loop is the heart of Node.js. It continuously checks for pending events, such as I/O operations or timers, and processes them sequentially. This approach ensures efficient use of system resources and enables asynchronous behavior.
How to Install and Configure Node.js?
- Download Node.js: Go to the official Node.js website (https://nodejs.org/) and download the appropriate installer for your operating system.
- Install Node.js: Run the installer and follow the installation instructions. Make sure to install the recommended version of Node.js.
- Verify Installation: Open a terminal and run the following commands to verify that Node.js and NPM (Node Package Manager) are installed:
node -v # Check Node.js version
npm -v # Check NPM version
- Configuration (Optional): Node.js does not require extensive configuration. However, you might configure environment variables, package.json for project dependencies, and other settings specific to your project.
- Create a Simple Application:
- Create a new directory for your project.
- Open a terminal, navigate to the project directory, and run
npm init
to create apackage.json
file. - Install dependencies using NPM, e.g.,
npm install express
. - Create an entry point file (e.g.,
app.js
) and start writing your Node.js application code.
Remember that the installation process may slightly vary depending on your operating system. For more advanced setups and configurations, refer to the official Node.js documentation.
Fundamental Tutorials of Node.js: Getting Started Step by Step
Sure, here are some step-by-step fundamental tutorials of Node.js:
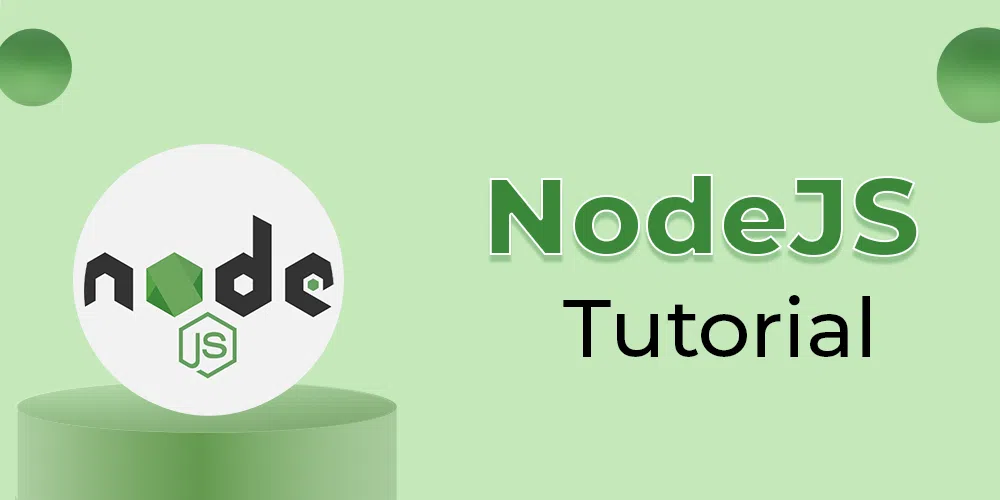
- Installing Node.js
To install Node.js, you can visit the Node.js website: https://nodejs.org/en/ and download the installer for your operating system. Once the installer is downloaded, run it and follow the instructions to install Node.js.
- Creating a simple web server
To create a simple web server with Node.js, you can use the following code:
JavaScript
const http = require('http');
const server = http.createServer((req, res) => {
res.writeHead(200, {'Content-Type': 'text/plain'});
res.end('Hello, world!');
});
server.listen(8080);
console.log('Server is listening on port 8080');
This code will generate a web server that reacts on port 8080. When a client makes a request to the server, the server will respond with the message “Hello, world!”.
- Using Node.js modules
Node.js has a large library of modules that can be used to extend its functionality. To use a module, you can use the require()
function. For example, the following code uses the http
module to create a web server:
JavaScript
const http = require('http');
const server = http.createServer((req, res) => {
res.writeHead(200, {'Content-Type': 'text/plain'});
res.end('Hello, world!');
});
server.listen(8080);
console.log('Server is listening on port 8080');
The require()
function will load the http
module and make it available to the code. The http
module then provides a function called createServer()
that can be used to create a web server.
- Debugging Node.js applications
Node.js applications can be debugged using the Node Inspector: https://nodejs.org/en/docs/inspector/. To use the Node Inspector, you can start the application in debug mode by passing the -inspect
flag to the Node.js command. For example, the following command will start the application in debug mode:
node -inspect myapp.js
Once the application is started in debug mode, you can connect to it using the Node Inspector. The Node Inspector will open a web page that allows you to inspect the application’s state and debug the code.
These are just a few of the many step-by-step fundamental tutorials that are available for Node.js. With a little practice, you will be able to use Node.js to build powerful and scalable applications.
- Why Can’t I Make Create A New Folder on External Drive on Mac – Solved - April 28, 2024
- Tips on How to Become a DevOps Engineer - April 28, 2024
- Computer Programming Education Requirements – What You Need to Know - April 28, 2024