What is Numpy?
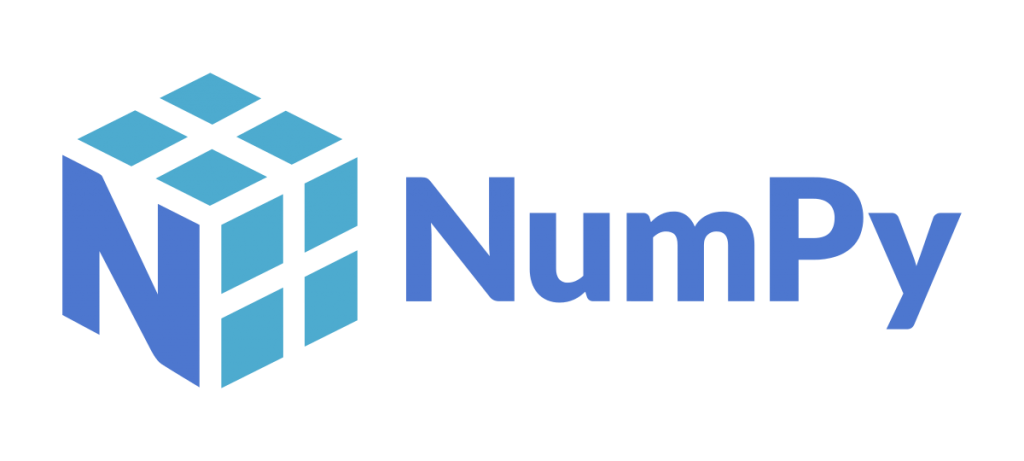
NumPy, short for Numerical Python, is a powerful open-source library for numerical computing in Python. It gives support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to handle on these arrays. NumPy is a fundamental library for scientific computing in Python and serves as the foundation for many other libraries, such as SciPy, pandas, and scikit-learn.
Key Features of NumPy:
- Multidimensional Arrays: NumPy provides a powerful
array
object that allows the creation of multi-dimensional arrays (vectors, matrices, and higher-dimensional arrays) to represent numerical data efficiently. - Element-wise Operations: NumPy supports element-wise operations on arrays, allowing for efficient and concise mathematical expressions.
- Broadcasting: NumPy allows operations between arrays of different shapes and sizes through broadcasting, which simplifies code and eliminates the need for explicit loops.
- Mathematical Functions: NumPy includes a vast collection of mathematical functions for operations such as trigonometry, logarithms, exponentials, statistical analysis, linear algebra, and more.
- Random Number Generation: NumPy provides functions for generating random numbers and random samples, essential for simulations and statistical applications.
- Indexing and Slicing: NumPy arrays support advanced indexing and slicing operations, providing flexibility in accessing and manipulating data.
- Linear Algebra Operations: NumPy includes a comprehensive set of linear algebra operations, including matrix multiplication, eigenvalue decomposition, and singular value decomposition.
- Memory Efficiency: NumPy arrays are memory-efficient and allow the manipulation of large datasets without a significant performance overhead.
- Integration with Other Libraries: NumPy seamlessly integrates with other scientific computing libraries in Python, forming the foundation for projects such as SciPy (scientific computing), pandas (data manipulation), and scikit-learn (machine learning).
What is top use cases of Numpy?
Top Use Cases of NumPy:
- Numerical Computing and Data Analysis:
- NumPy is widely used for numerical computing and data analysis in scientific research, engineering, and various domains where efficient manipulation of numerical data is required.
- Machine Learning and Data Science:
- NumPy is a fundamental library for machine learning and data science tasks. It is used for handling and preprocessing data, as well as for implementing algorithms and models.
- Signal Processing:
- NumPy is used in signal processing applications for tasks such as filtering, Fourier analysis, and time-domain analysis.
- Image Processing:
- NumPy is employed in image processing tasks for operations such as filtering, transformation, and manipulation of image data.
- Simulation and Modeling:
- NumPy is used in simulations and modeling to handle large datasets and perform numerical computations efficiently.
- Financial and Economic Modeling:
- NumPy is utilized in finance and economics for tasks such as risk analysis, option pricing, and economic modeling.
- Optimization and Numerical Analysis:
- NumPy is employed in optimization problems and numerical analysis for solving mathematical and engineering problems efficiently.
- Physics and Engineering Simulations:
- NumPy is used in simulations and computational tasks in physics and engineering, providing a fast and efficient platform for numerical calculations.
- Bioinformatics:
- NumPy is applied in bioinformatics for tasks such as analyzing genetic data, protein structure prediction, and computational biology.
- Game Development:
- NumPy is used in game development for tasks like physics simulations, collision detection, and handling large datasets representing game assets.
- Education and Research:
- NumPy is widely used in educational settings and research environments for teaching and conducting experiments in scientific computing and numerical analysis.
NumPy’s efficient array operations and mathematical functions make it a cornerstone of the Python scientific computing ecosystem, enabling a wide range of applications across various disciplines.
What are feature of Numpy?
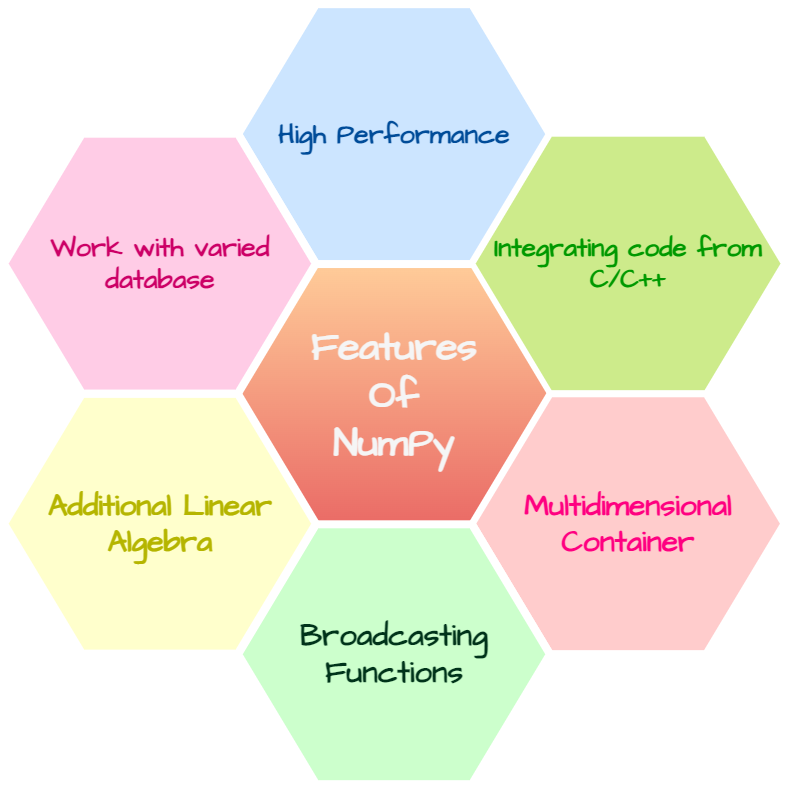
NumPy is a powerful library for numerical computing in Python, offering a variety of features that make it essential for scientific and mathematical applications:
- Multidimensional Arrays:
- NumPy provides the
numpy.array
object, which allows the creation of multi-dimensional arrays (vectors, matrices, and higher-dimensional arrays) for efficient storage and manipulation of numerical data.
- NumPy provides the
- Element-wise Operations:
- NumPy supports element-wise operations, enabling users to perform mathematical operations on entire arrays without the need for explicit looping.
- Broadcasting:
- NumPy supports broadcasting, a powerful feature that allows operations between arrays of different shapes and sizes. This simplifies code and enhances computational efficiency.
- Mathematical Functions:
- NumPy includes a comprehensive set of mathematical functions for basic arithmetic, trigonometry, logarithms, exponentials, statistics, linear algebra, and more.
- Random Number Generation:
- NumPy provides functions for generating random numbers and random samples, essential for simulations and statistical applications.
- Indexing and Slicing:
- NumPy arrays support advanced indexing and slicing operations, providing flexibility in accessing and manipulating data elements.
- Linear Algebra Operations:
- NumPy includes a rich set of linear algebra functions, including matrix multiplication (
numpy.dot
), eigenvalue decomposition (numpy.linalg.eig
), singular value decomposition (numpy.linalg.svd
), and more.
- NumPy includes a rich set of linear algebra functions, including matrix multiplication (
- Memory Efficiency:
- NumPy arrays are memory-efficient and offer performance benefits for handling large datasets. They provide a contiguous block of memory for efficient storage and retrieval of elements.
- Integration with Other Libraries:
- NumPy seamlessly integrates with other scientific computing libraries in Python, serving as the foundation for projects such as SciPy, pandas, and scikit-learn.
- Data Type Support:
- NumPy supports a variety of data types, allowing users to choose the appropriate type for their numerical data, whether it be integers, floating-point numbers, or complex numbers.
- Efficient Array Operations:
- NumPy implements highly optimized array operations in C and Fortran, providing performance benefits over traditional Python lists.
- File I/O:
- NumPy allows users to read and write array data to and from files, facilitating data storage and retrieval.
What is the workflow of Numpy?
The workflow of using NumPy typically involves the following steps:
- Import NumPy:
- Start by importing the NumPy library into your Python script or Jupyter notebook using the
import numpy as np
convention.
import numpy as np
- Create Arrays:
- Create NumPy arrays to represent numerical data. Arrays can be created from lists, tuples, or using functions like
numpy.array
.
# Create a 1D array
arr_1d = np.array([1, 2, 3])
# Create a 2D array
arr_2d = np.array([[1, 2, 3], [4, 5, 6]])
- Perform Operations:
- Utilize NumPy’s array operations for mathematical computations. NumPy supports element-wise operations and broadcasting.
# Element-wise addition
result = arr_1d + 10
# Broadcasting in a 2D array
result_2d = arr_2d * 2
- Indexing and Slicing:
- Access and manipulate elements within arrays using indexing and slicing operations.
# Accessing elements
element = arr_1d[0]
# Slicing a 2D array
sliced_array = arr_2d[:, 1:]
- Use Mathematical Functions:
- Leverage NumPy’s extensive collection of mathematical functions for various numerical computations.
# Calculate mean and standard deviation
mean_value = np.mean(arr_1d)
std_deviation = np.std(arr_1d)
- Linear Algebra Operations:
- Apply linear algebra operations for tasks such as matrix multiplication, eigenvalue decomposition, and singular value decomposition.
# Matrix multiplication
mat_product = np.dot(arr_2d, arr_2d.T)
# Eigenvalue decomposition
eigenvalues, eigenvectors = np.linalg.eig(mat_product)
- Random Number Generation:
- Generate random numbers and random samples using NumPy’s random module.
# Generate random array
random_array = np.random.rand(3, 3)
- File I/O:
- Read and write array data to and from files using NumPy’s file I/O functions.
# Save array to a file
np.save('my_array.npy', arr_2d)
# Load array from a file
loaded_array = np.load('my_array.npy')
- Integration with Other Libraries:
- Integrate NumPy with other scientific computing libraries like SciPy, pandas, and scikit-learn for advanced data analysis, statistics, and machine learning tasks.
This workflow can be adapted based on the specific requirements of the numerical computing or data analysis task at hand. NumPy’s efficiency and versatility make it a foundational tool for a wide range of applications in scientific computing and data science.
How Numpy Works & Architecture?
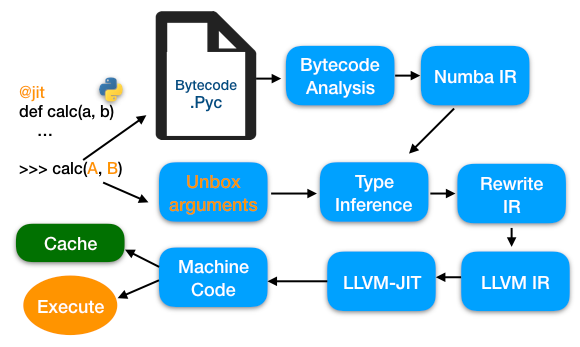
NumPy (Numerical Python) is a powerful library in Python for scientific computing. It offers a multidimensional array object called ndarray
and a collection of high-level mathematical functions that operate on these arrays efficiently. Understanding NumPy’s internal workings and architecture can enhance your programming skills and make you a more efficient user of this powerful library.
1. Core Functionality:
- Multidimensional Arrays:
ndarray
stores data in a multidimensional grid, allowing efficient manipulation of large datasets. - Data Types: Supports various data types like integers, floats, strings, complex numbers, and custom data types.
- Vectorized Operations: Operations like addition, subtraction, multiplication, and element-wise comparisons are applied to entire arrays, not individual elements, leading to significant performance gains.
- Broadcasting: Automatically adjusts dimensions of arrays for element-wise operations, simplifying calculations.
- Linear Algebra: Provides functions for matrix operations, eigenvalues/eigenvectors, and other linear algebra computations.
- Random Number Generation: Generates random numbers from various distributions for simulations and statistical analysis.
2. Architecture:
- C-based Core: NumPy’s core functionalities are written in C, enabling efficient memory management and high-performance calculations.
- Python Interface: Python bindings provide a user-friendly interface to access and manipulate NumPy objects from within Python code.
- NumPy Arrays: Arrays are stored in contiguous memory blocks, allowing for fast access and efficient operations.
- Memory Management: NumPy manages memory allocation and deallocation internally, reducing the risk of memory leaks and crashes.
- Lazy Evaluation: Certain operations are evaluated only when needed, optimizing performance and memory usage.
3. Advantages of NumPy’s Architecture:
- Performance: C-based core and vectorized operations lead to significant speedups compared to traditional Python loops.
- Memory Efficiency: Contiguous memory allocation and lazy evaluation optimize memory usage.
- Ease of Use: Python interface makes NumPy accessible for users with basic Python knowledge.
- Flexibility: Supports various data types, array dimensions, and operations, catering to diverse scientific computing needs.
- Extensible: NumPy integrates seamlessly with other scientific libraries like SciPy and Matplotlib for advanced scientific computing tasks.
By understanding how NumPy works under the hood, you can leverage its strengths and unlock its full potential for efficient and powerful scientific computing in Python.
How to Install and Configure Numpy?
Installing and Configuring NumPy in Python:
Installing NumPy:
- Package manager: The most common way is through your Python package manager.
- pip:
pip install numpy
- conda:
conda install numpy
- pip:
- Virtual environment: It’s recommended to install NumPy in a virtual environment for project isolation.
- Create a virtual environment:
python -m venv venv
- Activate it:
source venv/bin/activate
- Install NumPy:
pip install numpy
- Create a virtual environment:
- Binary installers: Download pre-built installers from the NumPy website
- This is useful if package managers are unavailable or internet access is limited.
Configuring NumPy:
- Import: Typically, you’ll import NumPy using
import numpy as np
. - Environment variables: You can configure NumPy’s behavior through environment variables.
OMP_NUM_THREADS
: Sets the number of threads used for parallel operations.MKL_THREADING
: Controls multithreading for Intel Math Kernel Library (MKL).
- NumPy settings: Access and modify NumPy settings through attributes and methods.
np.set_printoptions
: Customize printing of NumPy arrays.np.seterr
: Adjust error handling behavior.
- Additional packages: Consider installing additional packages like SciPy and Matplotlib for advanced scientific computing and data visualization.
By following these steps and utilizing available resources, you can successfully install and configure NumPy for your Python projects and start exploring its powerful capabilities for scientific computing.
Fundamental Tutorials of Numpy: Getting started Step by Step
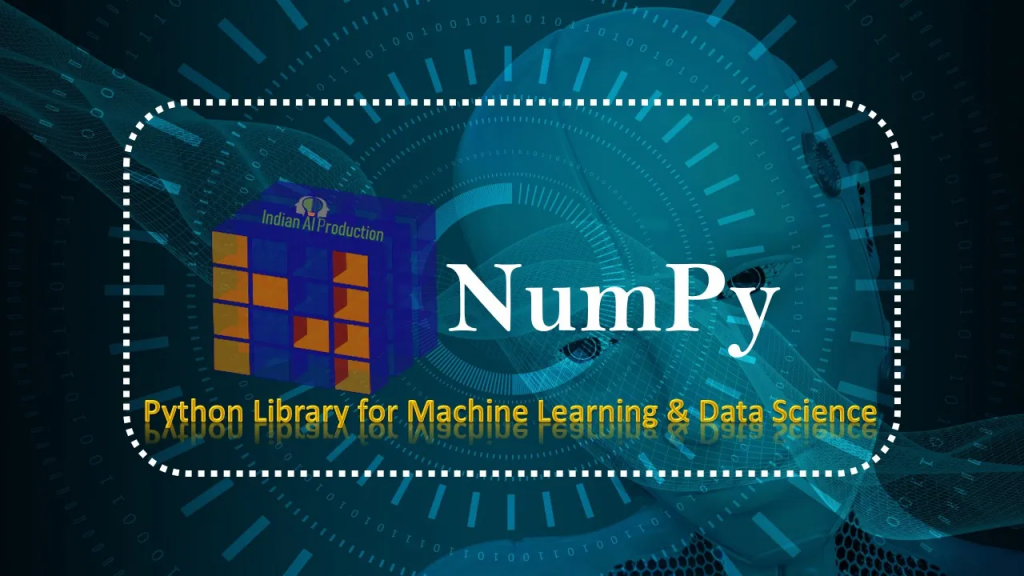
Following is a Step-by-Step Basic Tutorials of NumPy:
1. Introduction and Setup:
- What is NumPy? Understand its purpose and functionalities for scientific computing in Python.
- Benefits of NumPy: Explore advantages like vectorized operations, multidimensional arrays, and performance gains.
- Setting Up: Install NumPy using your preferred method (pip, conda, etc.) and import it as
np
.
2. Creating NumPy Arrays:
- Basic Syntax: Learn how to create arrays using:
- Literal values:
np.array([1, 2, 3])
- Existing sequences:
np.array([x, y, z])
- Functions like
np.zeros
,np.ones
, andnp.random.rand
.
- Literal values:
- Data Types: Specify data types like integers, floats, strings, and booleans for elements.
- Shape and Dimensions: Understand the concept of array dimensions and shapes.
3. Array Indexing and Slicing:
- Accessing Elements: Use indexes to access individual elements (e.g.,
arr[0]
). - Slicing Arrays: Extract sub-arrays using slices (e.g.,
arr[1:3]
). - Boolean Indexing: Select elements based on conditions using boolean masks.
4. Basic Array Operations:
- Arithmetic Operations: Learn vectorized operations like addition, subtraction, multiplication, and division.
- Comparison Operators: Compare elements using operators like
==
,<
,>
, etc. - Element-wise Functions: Apply functions like
np.sin
,np.exp
, andnp.log
to each element.
5. Broadcasting and Universal Functions:
- Broadcasting: Understand how NumPy automatically adjusts dimensions for operations.
- Universal Functions (ufuncs): Utilize powerful functions like
np.sum
,np.mean
, andnp.std
for efficient calculations.
6. Data Manipulation and Reshaping:
- Stacking and Concatenation: Combine arrays vertically (stack) or horizontally (concatenate).
- Splitting and Transposing: Split arrays and change their dimensions (transpose).
- Reshaping: Modify the shape of an array while preserving data.
7. Advanced Topics (Optional):
- Linear Algebra: Explore functions for matrix operations, eigenvalues/eigenvectors, and other linear algebra calculations.
- Random Number Generation: Generate random numbers from various distributions for statistical analysis.
- NumPy with Other Libraries: Combine NumPy with SciPy and Matplotlib for advanced scientific computing and data visualization.
Practice each step with small code examples and experiment with different arrays and operations. Don’t hesitate to utilize resources and ask the community for help. By mastering these basic tutorials, you’ll be well on your way to harnessing the power of NumPy for efficient and powerful scientific computing in Python.
- Mutual of Omaha: Selection and Interview process, Questions/Answers - April 15, 2024
- AES: Selection and Interview process, Questions/Answers - April 15, 2024
- Amphenol: Selection and Interview process, Questions/Answers - April 15, 2024