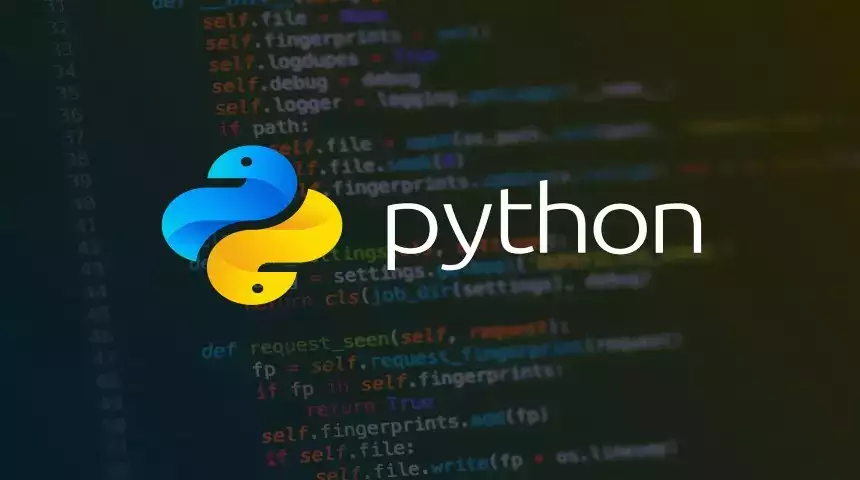
Python 3.x is a major version of the Python programming language, succeeding Python 2.x. Python 3 introduced several significant changes and improvements over Python 2, with a focus on cleaning up and modernizing the language. Python 3 is the current and actively maintained version of Python, and it includes various enhancements to make the language more consistent, efficient, and robust.
What is top use cases of Python-3.x?
- Web Development: Python 3 is widely used for building web applications and websites. Frameworks like Django and Flask are popular choices for creating web applications, RESTful APIs, and dynamic websites.
- Data Science and Machine Learning: Python 3 is the dominant language in the field of data science and machine learning. Libraries such as NumPy, pandas, scikit-learn, TensorFlow, and PyTorch are extensively used for data analysis, machine learning, and artificial intelligence.
- Scientific Computing: Python 3 is used in scientific research and engineering for tasks like numerical simulations, data analysis, and visualization. Libraries like SciPy and Matplotlib are commonly employed in this domain.
- Automation and Scripting: Python 3 is an excellent choice for writing scripts and automating tasks. It is used for tasks like file manipulation, data extraction, system administration, and batch processing.
- Web Scraping: Python 3 is often used for web scraping and data extraction from websites. Libraries like BeautifulSoup and Scrapy simplify the process of parsing web pages and extracting information.
- Databases: Python 3 has libraries for working with various databases, both relational (e.g., SQLite, PostgreSQL) and NoSQL (e.g., MongoDB, Redis). It is used to build database-driven applications and data analysis tools.
- Game Development: Python 3 is used in game development, often with libraries like Pygame. While not as common as other languages for game development, Python’s simplicity makes it accessible for creating 2D games.
- Desktop Applications: Python 3 can be used to develop cross-platform desktop applications using libraries like PyQt or Tkinter. These applications can have graphical user interfaces (GUIs) and are suitable for various purposes.
- Networking and Network Automation: Python 3 is used for networking tasks, including network device configuration, monitoring, and automation. Libraries like Paramiko and Netmiko are used for interacting with network devices.
- Education and Learning: Python 3 is a popular language for teaching programming and computer science concepts due to its readability and simplicity. Many educational institutions use Python as a first programming language.
- Server-Side Scripting: Python 3 is used for server-side scripting, powering web servers and web applications. Frameworks like FastAPI are gaining popularity for building RESTful APIs.
- Natural Language Processing (NLP): Python 3 is frequently used for NLP tasks, including text analysis, sentiment analysis, chatbots, and language translation.
Python 3.x’s versatility, extensive library ecosystem, and ease of use have contributed to its widespread adoption across various domains, making it one of the most popular programming languages in the world.
What are the features of Python-3.x?
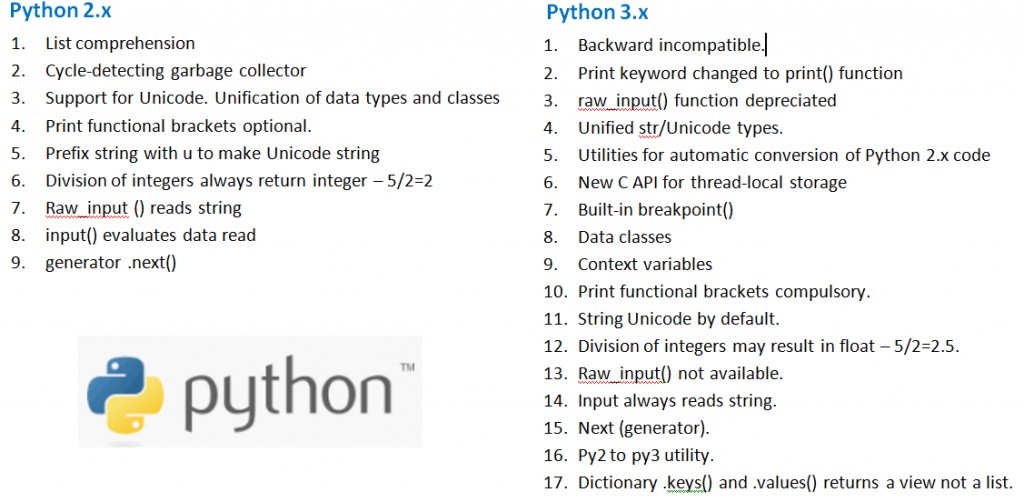
Python 3.x, the third major version of the Python programming language, introduced several key features and improvements over Python 2.x. Here are some of the notable features of Python 3.x:
- Print Function: Python 3.x replaces the print statement with the
print()
function, making it more versatile and consistent with other functions in the language. This change allows for better formatting and handling of multiple arguments. - Unicode Support: Python 3.x has native support for Unicode strings, making it the default string type. This enhances text processing capabilities, especially for internationalization and character encoding.
- Division Behavior: In Python 3.x, division between integers results in true division, where the result is a float. To perform integer division (floor division), the
//
operator is used. This change helps avoid common sources of bugs in Python 2.x. - Bytes and Strings Separation: Python 3.x enforces a clear distinction between bytes and text (Unicode) strings. This separation helps prevent issues related to character encoding and decoding.
- Enhanced Iterators and Generators: Python 3.x improves support for iterators and generators with features like the
yield from
statement, which simplifies generator delegation. - Syntax Enhancements: Python 3.x includes several syntax enhancements and simplifications, such as function annotations, extended unpacking (e.g.,
*args
and**kwargs
in function calls), and keyword-only arguments. - Advanced Data Structures: Python 3.x introduces advanced data structures like sets and dictionary comprehensions, making it more expressive and efficient for tasks that involve sets and dictionaries.
- Type Hints: Python 3.x supports optional type hints using the
typing
module. While Python remains dynamically typed, type hints provide valuable information for code analysis and static type checking tools like MyPy. - Improved Standard Library: Python 3.x includes a more robust and feature-rich standard library. Notable additions include the
asyncio
module for asynchronous programming and theenum
module for creating enumerations. - Performance Improvements: Python 3.x includes various performance enhancements and optimizations, making the language faster and more efficient in many scenarios compared to Python 2.x.
- Functionality Additions: Python 3.x introduces new functions and methods, such as
bytes()
andbytearray()
, to improve the handling of binary data. Additionally, thenonlocal
keyword allows nested functions to access and modify variables in their containing scope. - Improved Exception Handling: Python 3.x enhances exception handling with features like exception chaining, which makes it easier to trace the origin of exceptions.
What is the workflow of Python-3.x?
The workflow of Python 3.x is similar to that of Python 2.x, as Python 3 builds upon the same programming concepts. Here’s a general workflow when working with Python 3.x:
- Code Creation: Python 3.x code is created using a text editor or integrated development environment (IDE). Python source code files typically have the “.py” extension.
- Code Execution: Python 3.x code is executed by running it through a Python interpreter. The interpreter reads and interprets the code, executing it line by line.
- Syntax Validation: During execution, Python checks the syntax of the code for correctness. Syntax errors, if present, are reported as exceptions, and the program halts.
- Program Logic: Python 3.x code defines the logic and behavior of a program. It may include variable assignments, function definitions, conditionals (if statements), loops (for and while loops), and more.
- Data Manipulation: Python 3.x offers a wide range of data structures and functions for working with data, including lists, dictionaries, strings, and numeric types. Data manipulation and transformation are common tasks.
- Modules and Libraries: Python 3.x encourages code modularity and reuse. External libraries and modules can be imported and used in Python programs to extend functionality.
- Testing and Debugging: Python 3.x supports testing and debugging through various tools and frameworks. Testing helps ensure code correctness and reliability.
- Documentation: Good code is well-documented. Python 3.x allows developers to include comments and docstrings to explain code functionality and usage.
- Packaging and Distribution: Python 3.x code can be packaged and distributed as standalone applications or libraries using tools like setuptools or pip. This facilitates sharing and deployment.
- Deployment: Python 3.x applications can be deployed on various platforms, including servers, desktops, cloud services, and embedded systems.
- Maintenance and Updates: As with any software, Python 3.x code may require maintenance, updates, and bug fixes over time. Developers can use version control systems like Git to manage code changes.
- Optimization and Performance Tuning: Profiling and optimization techniques can be applied to improve the performance of Python 3.x applications when necessary.
- Monitoring and Scaling: In production environments, Python 3.x applications may be monitored for performance and reliability. Scalability considerations may also be addressed to handle increased loads.
Python 3.x’s versatility, readability, and extensive library ecosystem make it a powerful and popular choice for a wide range of applications, from web development to data analysis and scientific computing. Its workflow encompasses various stages of code development, testing, deployment, and maintenance.
How Python-3.x Works & Architecture?
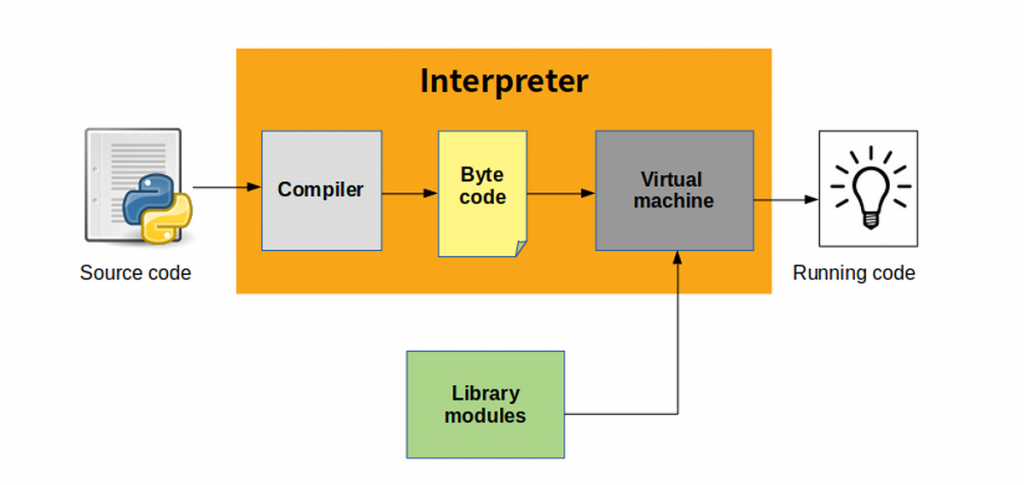
Python 3 is the latest major version of the Python programming language. It was released in 2008 and is a significant departure from previous versions of Python. Python 3 is not backward compatible with Python 2, so if you are writing code for Python 3, you cannot run it on Python 2.
Python 3 is a general-purpose programming language that can be used for a variety of tasks, including:
- Web development
- Data science
- Machine learning
- Artificial intelligence
- Game development
- Scripting
Python 3 is a interpreted language, which means that it is not compiled into machine code before it is executed. This makes Python 3 slower than compiled languages, but it also makes it more portable. Python 3 code can run on any platform that has a Python interpreter installed.
Python 3 is a dynamically typed language, which means that the types of variables are not declared explicitly. This makes Python 3 code more concise, but it can also lead to errors if the types of variables are not used correctly.
Python 3 is a garbage-collected language, which means that the programmer does not need to worry about deallocating memory that is no longer being used. This makes Python 3 code easier to write and maintain.
The architecture of Python 3 is object-oriented. This means that everything in Python 3 is an object, including numbers, strings, and functions. Object-oriented programming makes it easier to write modular code and to reuse code.
How to Install and Configure Python-3.x?
To install Python 3, you can follow these steps:
- Go to the Python website and download the installer for your operating system.
- Run the installer and apply the instructions.
- Once the installer is finished, you will have Python 3 installed on your computer.
To configure Python 3, you can follow these steps:
- Open a text editor and create a file called
.pythonrc.py
in your home directory. - In the file, add the below lines:
import sys
sys.path.append('/path/to/your/python/modules')
Replace /path/to/your/python/modules
with the path to the directory where your Python modules are located.
- Save the file and shutdown the text editor.
Once you have configured Python 3, you can start using it to write Python code.
Fundamental Tutorials of Python-3.x: Getting started Step by Step
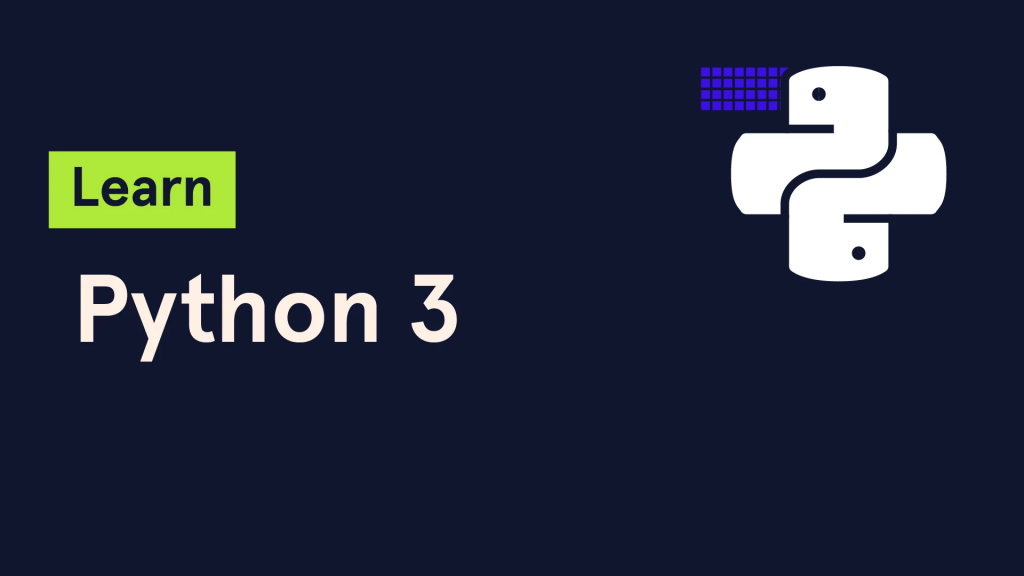
The following are the fundamental tutorials of Python 3.x:
- Create a simple Python program
A Python program is a sequence of instructions that are executed by the Python interpreter. To create a simple Python program, you can use a text editor to create a file with the .py extension. In the file, you can write the following code:
print("Hello, world!")
This code prints the text “Hello, world!” to the console.
- Variables
Variables are used to store data in Python. To generate a variable, you assign a value to it.
name = "John Doe"
age = 30
The variable name
is assigned the value “John Doe” and the variable age
is assigned the value 30.
- Data types
Python has a variety of data types, including numbers, strings, lists, dictionaries, and tuples.
- Numbers: Integers, floats, and complex numbers.
- Strings: A sequence of characters.
- Lists: A collection of ordered elements.
- Dictionaries: A collection of key-value pairs.
- Tuples: A collection of immutable elements.
4. Operators
Python has a variety of operators, which are used to perform operations on data.
- Arithmetic operators: +, -, *, /, //, %.
- Comparison operators: ==, !=, <, >, <=, >=.
- Logical operators: and, or, not.
- Assignment operators: =, +=, -=, *=, /=, //=, %=.
5. Control flow statements
Python has a variety of control flow statements, which are used to control the flow of execution of a program.
- If statement: Used to execute a block of code if a condition is met.
- For loop: Applied to iterate over a series of elements.
- While loop: Used to iterate over a block of code while a condition is met.
- Break statement: Used to break out of a loop.
- Continue statement: Used to skip the current iteration of a loop.
6. Functions
Functions are used to group together related code. They can be used to make code more modular and reusable.
def factorial(n):
"""Calculates the factorial of a number."""
if n == 0:
return 1
else:
return n * factorial(n - 1)
- Modules
Modules are a way to organize Python code. They can be used to import code from other files.
import math
print(math.pi)
- Object-oriented programming
Object-oriented programming is a programming paradigm that applies objects to represent data and behavior.
class Dog:
"""A class representing a dog."""
def __init__(self, name, breed):
self.name = name
self.breed = breed
def bark(self):
print("Woof!")
my_dog = Dog("Spot", "Labrador")
my_dog.bark()
These are just some of the fundamental concepts of Python 3.x.
- Why Can’t I Make Create A New Folder on External Drive on Mac – Solved - April 28, 2024
- Tips on How to Become a DevOps Engineer - April 28, 2024
- Computer Programming Education Requirements – What You Need to Know - April 28, 2024