What is Python?
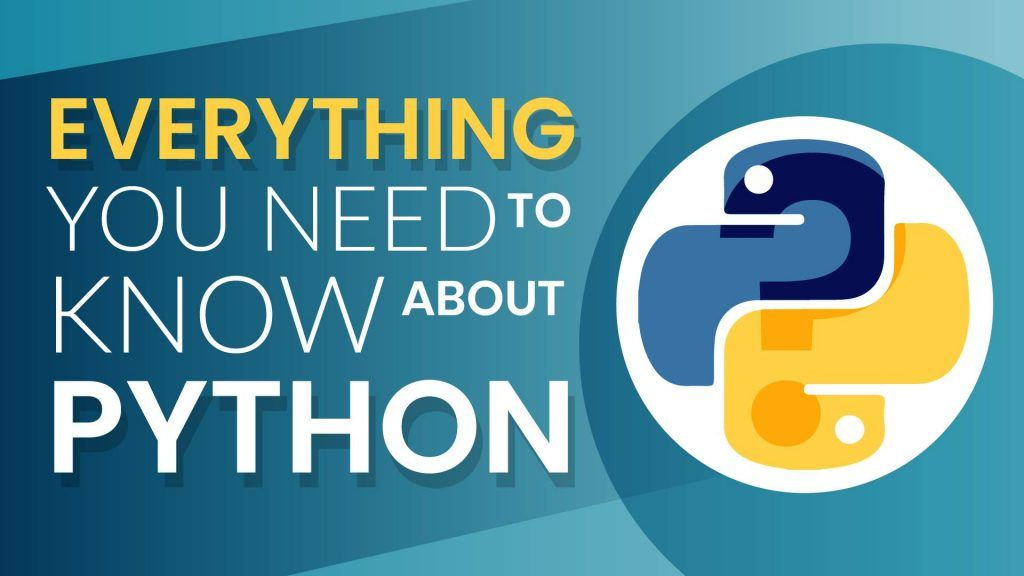
Python is a popular high-level programming language that is widely used for general-purpose programming. It was created by Guido van Rossum and first released in 1991, and has since become one of the most popular programming languages in the world. In this article, we will explore the top use cases of Python, its features, workflow, architecture, installation and configuration, and fundamental tutorials for getting started step by step.
What is the top use cases of Python?
Python has a wide range of use cases, from web development to machine learning. Some of the top use cases of Python include:
- Web development: Python is used to build web applications and websites, with popular web frameworks such as Django and Flask.
- Data analysis and visualization: Python is used for data analysis and visualization with libraries such as NumPy, Pandas, and Matplotlib.
- Machine learning and artificial intelligence: Python is a popular language for machine learning and AI development with libraries such as TensorFlow and Keras.
- Scientific computing: Python is used for scientific computing with libraries such as SciPy and SymPy.
- Game development: Python is used for game development with libraries such as Pygame.
What are the features of Python?
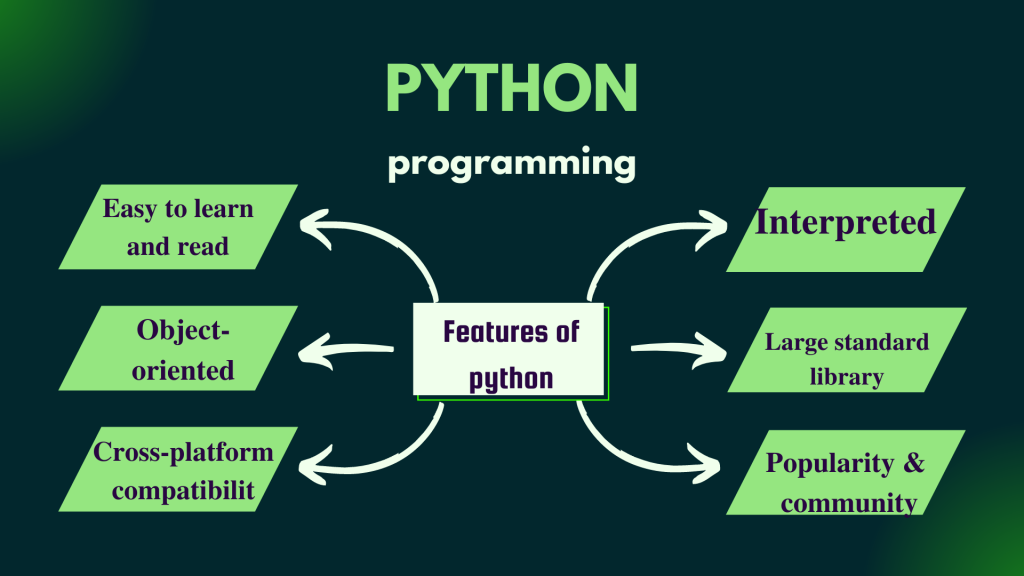
Python has many features that make it a popular programming language, including:
- Easy to learn and read: Python has a simple syntax and is easy to learn and read, making it a good language for beginners.
- Interpreted language: Python is an interpreted language, which means that it does not need to be compiled before it can be run.
- Object-oriented: Python is an object-oriented language, which means that it supports object-oriented programming concepts such as inheritance and encapsulation.
- Dynamic typing: Python is dynamically typed, which means that the data type of a variable is determined at runtime.
- Large standard library: Python has a large standard library that provides many useful modules and functions.
What is the workflow of Python?
The workflow of Python typically involves the following steps:
- Write code: The first step is to write Python code using a text editor or an integrated development environment (IDE).
- Run code: The next step is to run the Python code using a Python interpreter, which will execute the code and produce output.
- Debug code: If there are errors in the code, the next step is to debug the code using a debugger or by adding print statements.
- Test code: Once the code is working correctly, the next step is to test the code to ensure that it works as expected.
- Deploy code: Finally, the code can be deployed to a production environment where it can be used by users.
How Python Works & Architecture?
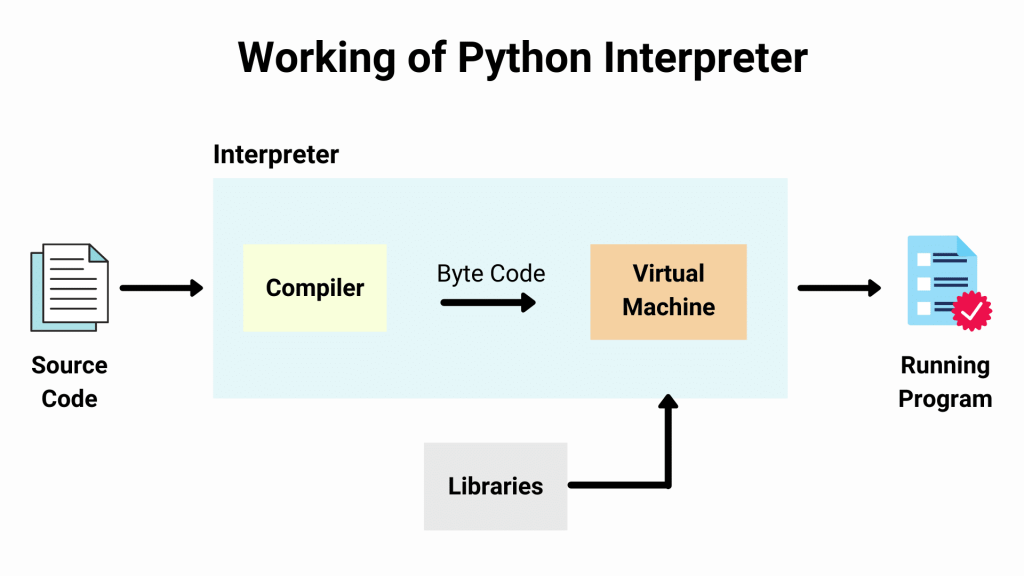
Python is an interpreted language, which means that it does not need to be compiled before it can be run. When Python code is executed, it is first compiled into bytecode, which is then interpreted by the Python interpreter. The Python interpreter is responsible for executing the bytecode and producing output.
Python has a modular architecture, with a large standard library that provides many useful modules and functions. Python also supports third-party modules, which can be installed using package managers such as pip.
How to Install and Configure Python?
Python can be installed on a variety of platforms, including Windows, macOS, and Linux. To install Python, you can download the installer from the official Python website and follow the installation instructions.
Once Python is installed, you can configure it by setting environment variables and installing third-party modules using package managers such as pip.
Fundamental Tutorials of Python: Getting Started Step by Step
Getting Started with Python
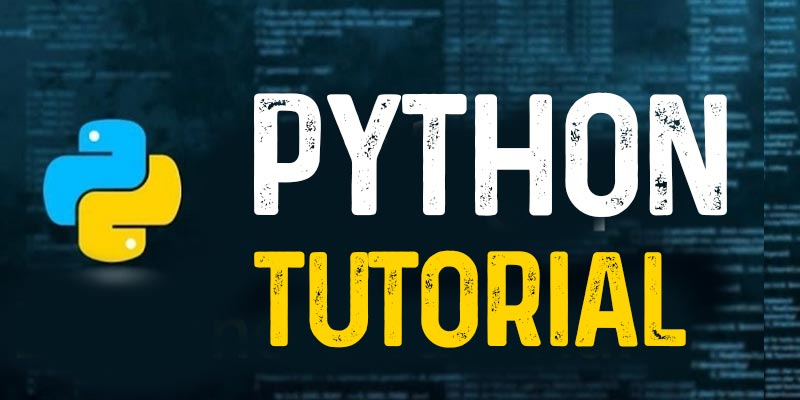
Installing Python
Before we can start coding in Python, we need to install the Python interpreter on our computer. There are several ways to do this, depending on your operating system and preferences. In this tutorial, we will use the Anaconda distribution, which is a popular and user-friendly platform for data science and scientific computing.
To install Anaconda, follow these steps:
- Go to the Anaconda website at https://www.anaconda.com/products/individual.
- Click on the Download button for your operating system (Windows, macOS, or Linux).
- Follow the instructions to download and install the Anaconda installer.
- Open the Anaconda Navigator, which is a graphical user interface for managing your Python environments and packages.
Basic Python Syntax
Python code is written in plain text files, with the extension .py. To write and run Python code, we can use a text editor or an integrated development environment (IDE). In this tutorial, we will use the Jupyter Notebook, which is a web-based IDE that allows us to write and run Python code in interactive cells.
To open a new Jupyter Notebook, follow these steps:
- Open the Anaconda Navigator.
- Click on the Launch button for Jupyter Notebook.
- In the Jupyter Notebook interface, click on the New button and select Python 3 to create a new notebook.
In Python, we use indentation to indicate the structure of our code. Blocks of code are indented with four spaces, and statements within a block are aligned vertically. For example:
if x > 0:
print("x is positive")
else:
print("x is non-positive")
Python has many built-in data types, such as integers, floats, strings, lists, tuples, and dictionaries. We can use operators and functions to manipulate and combine these data types. For example:
x = 2 + 3 * 4 / 2
y = "Hello, " + "world!"
z = [1, 2, 3]
w = {"a": 1, "b": 2, "c": 3}
Control Flow and Functions
In Python, we can use control flow statements to execute code based on conditions or loops. For example:
if x > 0:
print("x is positive")
elif x < 0:
print("x is negative")
else:
print("x is zero")
for i in range(10):
print(i)
while x < 10:
x = x + 1
def my_function(x, y=1):
z = x + y
return z
result = my_function(2, 3)
Functions are blocks of code that perform a specific task and can be reused in different parts of a program. We can define functions with parameters and return values, and call them with arguments. For example:
def greet(name):
print("Hello, " + name + "!")
greet("Alice")
greet("Bob")
File I/O and Modules
Python can read and write files using the built-in functions open() and close(), as well as the methods read(), readline(), write(), and writelines(). For example:
file = open("example.txt", "w")
file.write("Hello, world!\n")
file.close()
file = open("example.txt", "r")
content = file.read()
print(content)
file.close()
Python also has a large and diverse ecosystem of external modules and libraries, which provide additional functionality and tools for specific tasks. We can import modules using the import statement, and use their functions and classes in our code. For example:
import math
x = math.sin(0.5)
y = math.sqrt(2)
Conclusion: Keep Learning Python
Congratulations! You have completed the fundamental tutorials of Python step by step. You now have a solid foundation in Python syntax, control flow, functions, file I/O, and modules. However, this is just the beginning of your journey as a Python programmer. There is much more to learn and explore, such as object-oriented programming, web frameworks, data visualization, machine learning, and more. Keep practicing, experimenting, and asking questions, and you will become a proficient and creative Python developer. Happy coding!
- Why Can’t I Make Create A New Folder on External Drive on Mac – Solved - April 28, 2024
- Tips on How to Become a DevOps Engineer - April 28, 2024
- Computer Programming Education Requirements – What You Need to Know - April 28, 2024