What is Qt?
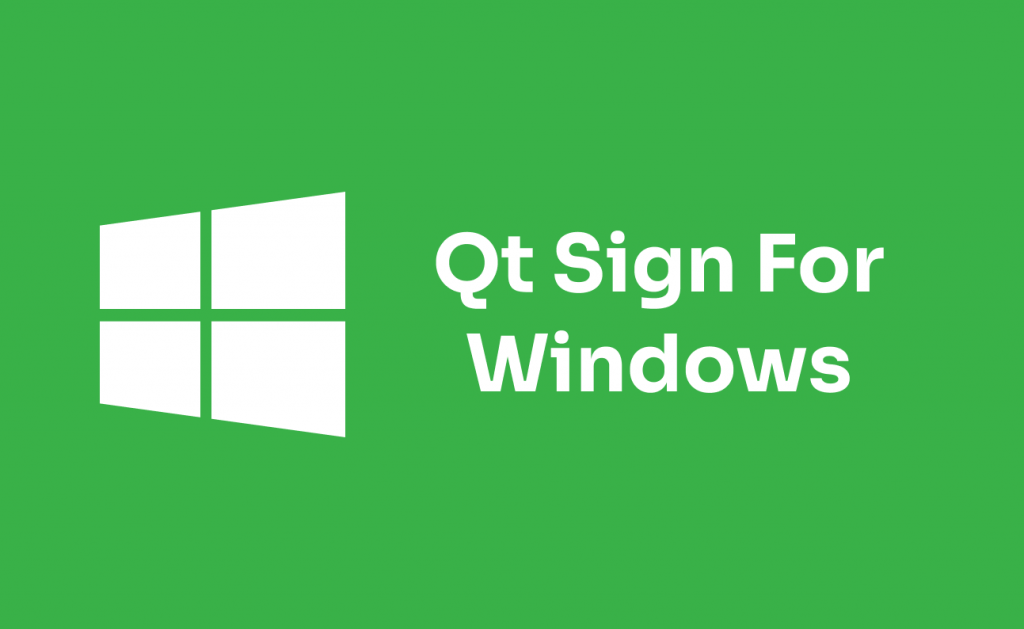
Qt is a cross-platform C++ framework that facilitates the development of applications with graphical user interfaces (GUIs) and provides a wide range of tools and libraries for software development. Originally developed by Trolltech (now part of The Qt Company), Qt has become one of the most popular frameworks in the software development industry. It is known for its flexibility, ease of use, and support for building applications that can run on multiple platforms without significant modifications.
Key Features of Qt:
- Cross-Platform Development:
- Qt enables the development of applications that can run on various operating systems, including Windows, Linux, macOS, Android, iOS, and more.
- Widgets and Layouts:
- Qt provides a comprehensive set of customizable UI components (widgets) and layout managers for creating modern and responsive user interfaces.
- Signal and Slot Mechanism:
- Qt uses a powerful signal and slot mechanism for communication between objects, promoting a flexible and decoupled design.
- Object-Oriented Framework:
- Qt is designed with object-oriented principles, allowing developers to create modular and reusable components using encapsulation, inheritance, and polymorphism.
- Internationalization and Localization:
- Qt supports internationalization (i18n) and localization (l10n), making it easy to create applications that can be adapted to different languages and regions.
- OpenGL Integration:
- Qt seamlessly integrates with OpenGL, enabling the development of graphics-intensive applications and 3D visualization.
- Database Integration:
- Qt includes modules for working with databases, allowing developers to integrate database functionality into their applications.
- Networking:
- Qt provides networking libraries for tasks such as making HTTP requests, handling sockets, and implementing network protocols.
- Multithreading:
- Qt supports multithreading, allowing developers to create applications that can efficiently perform multiple tasks concurrently.
What is top use cases of Qt?
Top Use Cases of Qt:
- Desktop Application Development:
- Qt is widely used for creating desktop applications, including utilities, productivity tools, media players, and more.
- Cross-Platform Mobile App Development:
- Qt facilitates the development of mobile applications that can run on both Android and iOS platforms, enabling code sharing between different mobile operating systems.
- Embedded Systems:
- Qt is popular for developing embedded systems and devices, such as digital signage, set-top boxes, and home automation systems.
- Automotive Infotainment Systems:
- Many automotive manufacturers use Qt for building infotainment systems, dashboards, and in-car displays due to its cross-platform capabilities and rich UI components.
- Industrial Control Systems (ICS):
- Qt is employed in the development of Human-Machine Interface (HMI) applications for industrial control systems and automation.
- Medical Devices and Imaging Software:
- Qt is used in the development of medical devices and imaging software, providing a framework for creating complex and interactive user interfaces.
- Scientific and Engineering Software:
- Qt is chosen for developing scientific and engineering applications, providing tools for data visualization, simulations, and analysis.
- Game Development:
- Qt can be utilized in game development, particularly for creating game interfaces, tools, and certain game components.
- Educational Software:
- Qt is employed in the development of educational software, providing a framework for creating interactive and visually appealing applications for learning.
- Cross-Platform GUI Tools and IDEs:
- Qt itself is used for developing graphical tools and integrated development environments (IDEs) due to its capabilities in creating sophisticated user interfaces.
Qt’s versatility, robustness, and cross-platform capabilities make it a popular choice for a wide range of applications, from small utility programs to complex and industry-specific solutions.
What are feature of Qt?
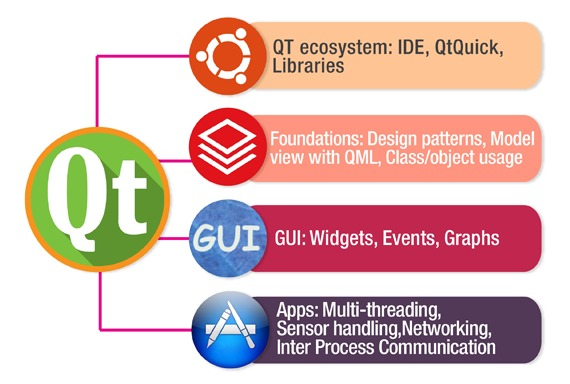
Features of Qt:
- Cross-Platform Development:
- Qt enables the development of cross-platform applications, allowing code reuse across different operating systems without major modifications.
- Widgets and UI Components:
- Qt provides a rich set of customizable UI components (widgets) and layout managers for creating modern and responsive user interfaces.
- Signals and Slots Mechanism:
- Qt uses a powerful signal and slot mechanism for communication between objects, allowing developers to create flexible and modular code.
- Object-Oriented Framework:
- Qt is designed with object-oriented principles, supporting features like encapsulation, inheritance, and polymorphism.
- Internationalization (i18n) and Localization (l10n):
- Qt supports internationalization and localization, allowing developers to create applications that can be easily adapted to different languages and regions.
- Graphics and OpenGL Integration:
- Qt seamlessly integrates with OpenGL, providing capabilities for graphics-intensive applications, 2D and 3D graphics rendering, and image manipulation.
- Database Integration:
- Qt includes modules for working with databases, making it convenient to integrate database functionality into applications.
- Networking Libraries:
- Qt provides networking libraries for tasks such as making HTTP requests, handling sockets, and implementing network protocols.
- Multithreading Support:
- Qt supports multithreading, allowing developers to create applications that efficiently perform multiple tasks concurrently.
- File System Abstraction:
- Qt provides a cross-platform file system abstraction, simplifying file and directory operations.
- Qt Quick and QML:
- Qt Quick is a declarative language for designing UIs, and QML (Qt Meta-Object Language) is a JavaScript-based language for defining user interface-centric applications.
- Styles and Themes:
- Qt allows developers to customize the appearance of applications through styles and themes.
- Integration with IDEs:
- Qt integrates well with popular integrated development environments (IDEs) like Qt Creator, making the development process smoother.
- Plugin System:
- Qt supports a plugin system that allows developers to extend and customize the functionality of their applications.
- Test-Driven Development (TDD) Support:
- Qt provides tools and libraries for supporting test-driven development, including the Qt Test module for unit testing.
What is the workflow of Qt?
The workflow of developing with Qt generally involves the following steps:
- Installation:
- Install the Qt framework and development tools on your machine.
- Project Creation:
- Create a new Qt project using Qt Creator or another preferred IDE. Specify project details, such as project type, template, and target platforms.
- UI Design:
- Design the user interface using Qt Designer or by manually editing UI files. Qt supports both visual design and programmatic UI creation.
- Code Implementation:
- Write the application logic and functionality in C++ or other supported languages. Implement the application’s features, business logic, and interactions.
- Signals and Slots:
- Use the signals and slots mechanism to handle events and communicate between different components of the application.
- Debugging:
- Debug the application using built-in debugging tools or external debuggers. Identify and fix issues in the code.
- Testing:
- Write unit tests using the Qt Test module or other testing frameworks. Perform testing to ensure the correctness of the application.
- Building:
- Build the application using the Qt build system or the build tools provided by your chosen IDE. Generate executable files for the target platform.
- Deployment:
- Deploy the application on the target platforms. This may involve packaging the application, handling dependencies, and preparing for distribution.
- Localization:
- If necessary, perform localization by translating strings and adapting the application for different languages and regions.
- Version Control:
- Use version control systems (e.g., Git) to handle the source code and collaborate with other developers.
- Documentation:
- Create documentation for the application, including user manuals, API documentation, and any other relevant documentation.
- Maintenance and Updates:
- Monitor and maintain the application, addressing bug fixes, adding new features, and releasing updates as needed.
The workflow may vary depending on the type of application, the chosen development environment, and the specific requirements of the project. Qt’s modular and flexible design allows developers to tailor their workflow based on the needs of their application.
How Qt Works & Architecture?
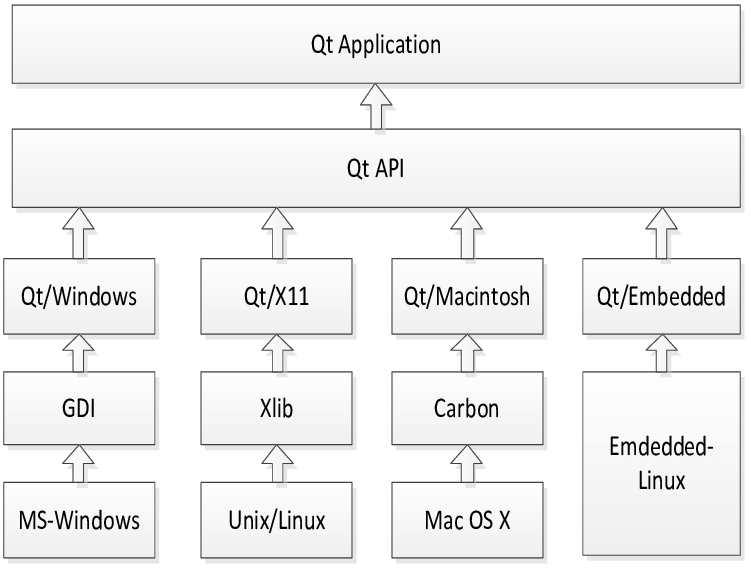
Qt is a powerful C++ framework for developing cross-platform applications with rich graphics and user interfaces. Its architecture is layered and modular, providing flexibility and control for developers. Here’s a breakdown of how Qt works:
1. Core Layers:
- Core: This foundational layer provides essential functionalities like memory management, threading, and event handling. It’s the lowest-level layer and interacts directly with the operating system.
- GUI Framework: This layer builds upon the core to provide widgets, layout managers, and graphics APIs for building user interfaces. It offers a wide range of pre-built widgets like buttons, lists, and text editors, and allows for custom widget creation.
- QML: This declarative language allows for UI development using a syntax similar to JavaScript. It offers a more concise and visual approach to building UIs, often used in combination with C++ code.
2. Plugins and Modules:
- Plugins: These extend Qt’s functionalities by offering additional features like networking, multimedia, and database access. They can be dynamically loaded at runtime, making Qt highly customizable.
- Modules: These are pre-built libraries for specific tasks, such as Qt Charts for data visualization or Qt WebEngine for web browsing capabilities. They offer ready-to-use functionality without requiring custom development.
3. Application Development:
- Development Process: Qt provides tools and libraries for all stages of application development, from designing UI mockups to writing C++ code and deploying the application.
- Signal-Slot Mechanism: This communication system allows different parts of the application to interact by sending and receiving signals and slots. It promotes decoupling and simplifies communication between components.
- Cross-Platform Development: Qt applications are written once in C++ and can be compiled for multiple platforms like Windows, macOS, Linux, Android, and iOS, with minimal platform-specific adjustments.
Benefits of Qt Architecture:
- Modular and Flexible: The layered architecture allows for selective use of features and customization to fit specific needs.
- Cross-Platform Development: Build once, deploy everywhere with minimal platform-specific code.
- Rich Functionality: Extensive pre-built widgets, plugins, and modules cover diverse functionalities.
- Efficient Performance: Native C++ code ensures efficient resource usage and application performance.
- Large Community and Resources: A vast community and extensive documentation provide ample support and learning materials.
By understanding how Qt works and its architecture, you can leverage its strengths to build powerful and user-friendly cross-platform applications for various purposes.
How to Install and Configure Qt?
Here are the general steps on how to install and configure Qt:
1. Download the Installer:
- Visit the official Qt website and download the appropriate installer for your operating system.
- Choose between the open-source version (Qt Creator for Open-Source) or the commercial version (Qt Creator).
2. Run the Installer:
- Launch the installer and follow the on-screen instructions.
- Select the desired Qt version, modules, and components you want to install.
- Specify the installation location.
- Important: Make sure to check the box to add Qt to your PATH environment variable during installation.
3. Set Up Qt Creator (Optional):
- If you’re using Qt Creator, launch it and configure the following:
- Kits: Define the Qt version and compiler to use for building your projects.
- Build & Run: Set up deployment settings for different platforms if needed.
4. Verify Installation:
- Open a command prompt or terminal and type
qmake -v
orqtcreator -v
. If Qt is installed correctly, you’ll see the version information.
5. Additional Configuration (If Needed):
- Environment Variables: If you didn’t add Qt to your PATH during installation, manually set the
QTDIR
environment variable to the Qt installation directory. - Compiler Configuration: Ensure your compiler is properly configured to work with Qt.
- Third-Party Libraries: If you need third-party libraries, configure Qt to link against them.
Specific Guides:
- Windows: Download Qt for windows version from their official site.
- macOS: Download Qt for macOS version from their official site
- Linux: Download Qt for Linux version from their official site
Troubleshooting:
- Consult the Qt documentation and forums for troubleshooting tips if you encounter issues.
- Search for solutions online in the vast Qt community.
Important Notes:
- Consider using Qt Maintenance Tool for updates and managing Qt installations.
- Explore Qt Creator for a comprehensive development environment with integrated tools.
- Keep your Qt installation up-to-date to benefit from bug fixes and new features.
Fundamental Tutorials of Qt: Getting started Step by Step
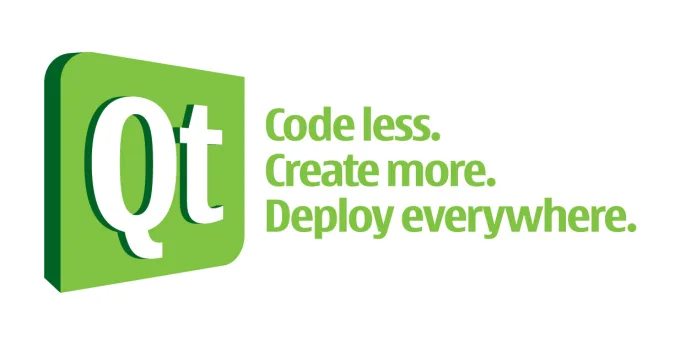
Here’s a guide to get you started with basic Qt development:
1. Setting Up:
- Install Qt: Download and install Qt Creator for your platform (Windows, macOS, Linux) from their official website.
- Open Qt Creator: Launch the application.
- Create a New Project: Choose “Qt Widgets Application” and select a name and location.
- Explore the Interface: Familiarize yourself with the main window layout, including the code editor, project explorer, and property editor.
2. Building Your First App:
- Adding Widgets: Drag and drop widgets like buttons, labels, and text edits from the “Widget Box” to the design area.
- Connecting Signals and Slots: Right-click a widget and choose “Go to Slot…” to create a slot function in your code. Connect signals (e.g., button click) to slots to handle user interaction.
- Writing C++ Code: Implement the slot functions and any other logic required for your app in the C++ files provided.
- Building and Running: Click the “Run” button to compile and launch your app.
3. Basic Tutorials:
- HelloWorld: This classic example demonstrates displaying a simple message. Follow the steps above to create a label with the text “Hello, world!” and display it.
- Button Click Counter: Create a button and a label. Connect the button’s click signal to a slot that increments a counter variable. Update the label’s text with the current count in the slot function.
- Simple Calculator: Build a basic calculator with buttons for numbers, operators, and an output label. Use signals and slots to handle user input and perform calculations, updating the label with the result.
4. Important Tips:
- Begin with simple projects and gradually grow to more complex ones.
- Use the provided templates and examples as a starting point.
- Don’t hesitate to experiment and explore different functionalities.
- Refer to the documentation and online resources when you need help.
- Practice regularly to hone your Qt development skills.
Remember, learning Qt takes time and dedication. Be patient, persistent, and utilize the available resources to build your knowledge and skills. Have fun exploring the world of Qt!
- Mutual of Omaha: Selection and Interview process, Questions/Answers - April 15, 2024
- AES: Selection and Interview process, Questions/Answers - April 15, 2024
- Amphenol: Selection and Interview process, Questions/Answers - April 15, 2024