What is ReactJS?
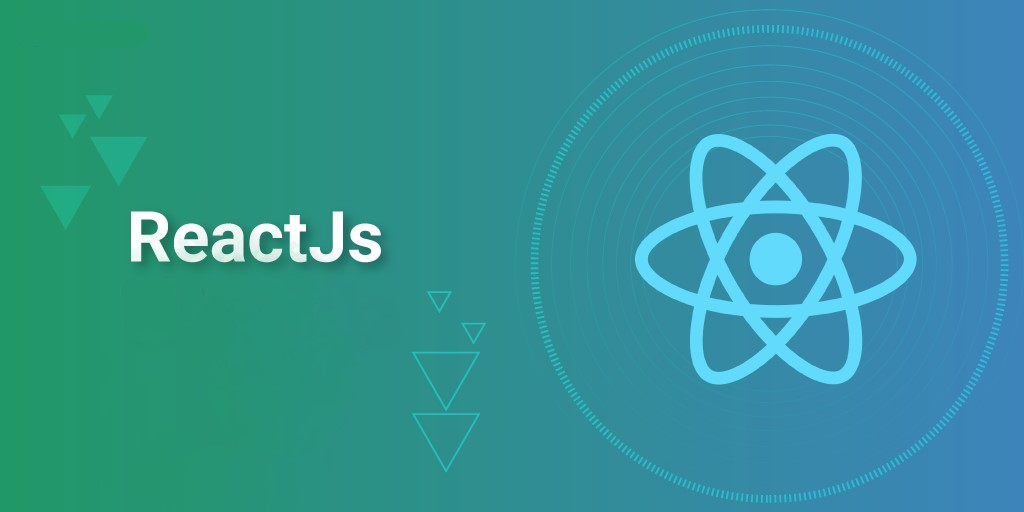
ReactJS, commonly referred to as React, is an open-source JavaScript library used for building user interfaces (UIs) and user interface components for web applications. It was developed by Facebook and later maintained by both Facebook and a community of developers. React focuses on creating reusable UI components and managing the dynamic rendering of those components in response to changes in application state.
Top Use Cases of ReactJS:
- Single Page Applications (SPAs): React is often used to build single-page applications where most of the UI interaction occurs within a single web page, without the need to reload the entire page.
- Component-Based Architecture: React’s component-based architecture makes it ideal for applications with complex UIs that can be broken down into reusable and maintainable components.
- Dynamic User Interfaces: React excels at creating dynamic UIs where different components can update independently based on changes in the application state.
- Mobile App Development: With tools like React Native, you can use React to build native mobile apps for iOS and Android platforms.
- Real-Time Applications: React’s efficient rendering process makes it suitable for real-time applications like chat applications or dashboards that require frequent updates.
- Interactive Web Applications: React’s virtual DOM and efficient rendering help create interactive and responsive web applications.
What are the features of ReactJs?
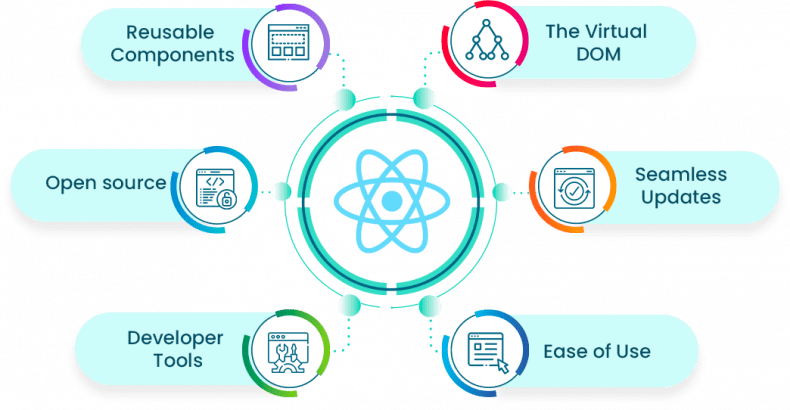
- Virtual DOM: React uses a virtual representation of the actual DOM, which allows for efficient updates and reduces the need to directly manipulate the real DOM.
- Component-Based Architecture: React applications are built as a composition of reusable and independent components.
- JSX: React uses JSX (JavaScript XML) syntax, which allows developers to write HTML-like code within JavaScript, making it easier to define UI components.
- Unidirectional Data Flow: React enforces a one-way data flow, which makes it easier to manage application state and predict how changes will affect the UI.
- Reconciliation Algorithm: React’s reconciliation algorithm efficiently updates the virtual DOM and minimizes the number of actual DOM updates needed.
- Declarative Syntax: React focuses on declaring what the UI should look like in different states rather than how to achieve those states.
What is the workflow of ReactJs?
- Component Design: Design and break down the UI into components, considering reusability and maintainability.
- Component Implementation: Write React components using JSX and JavaScript to define the structure and behavior of UI elements.
- State Management: Manage application state using React’s built-in state management or external libraries like Redux.
- Event Handling: Implement event handlers to respond to user interactions and update the application state accordingly.
- Rendering: React automatically updates the virtual DOM and efficiently re-renders components based on changes in state.
- Virtual DOM Diffing: React performs a diffing process to identify changes in the virtual DOM and applies the minimum necessary updates to the actual DOM.
How ReactJs Works & Architecture?
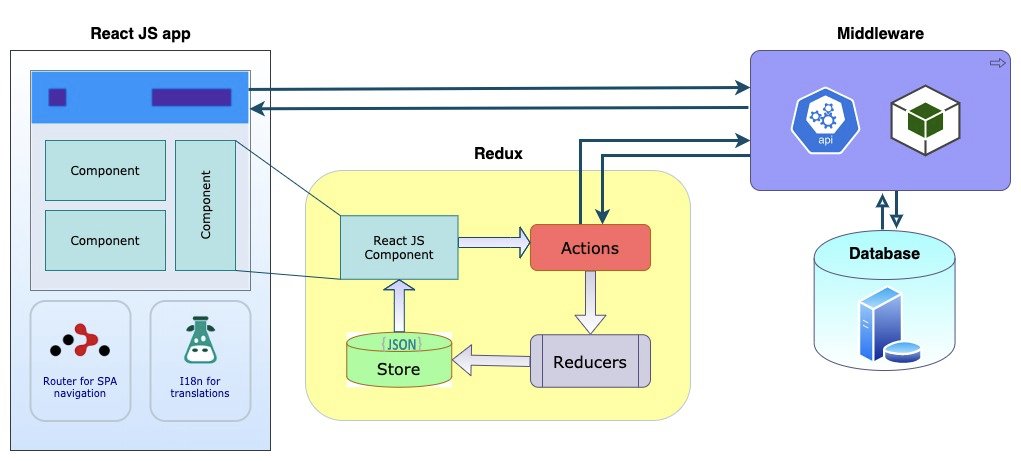
ReactJS works by utilizing a virtual representation of the DOM (Document Object Model) and efficiently updating the actual DOM in response to changes in application state. Its architecture is centered around the concept of building reusable components and managing their rendering and interaction efficiently. Here’s an overview of how ReactJS works and its architecture:
1. Virtual DOM:
The Virtual DOM is a lightweight copy of the actual DOM kept in memory. It’s a representation of how the UI should look. React creates and maintains this virtual representation to optimize the process of updating the UI.
2. Component-Based Architecture:
React applications are built using components. Components are self-contained, reusable pieces of the UI. A component can be a simple UI element (like a button) or a more complex part of the application (like a user profile).
3. Rendering:
When the application’s state changes, React re-renders the components. It compares the previous virtual DOM with the updated one (this process is called “reconciliation” or “diffing”). It identifies the differences and calculates the minimum number of changes required to update the actual DOM.
4. Reconciliation Algorithm:
React’s reconciliation algorithm efficiently identifies changes in the virtual DOM and optimizes updates to the actual DOM. It minimizes the number of expensive operations, like direct manipulation of the DOM.
5. JSX:
React uses JSX (JavaScript XML) to define components and their structure. JSX allows developers to write HTML-like code within JavaScript, making it easier to express UI components.
6. Component Lifecycle:
React components go through a lifecycle that includes different phases like creation, updating, and destruction. You can tap into these phases using lifecycle methods or React’s newer Hooks API, which provides a more flexible way to manage component lifecycle.
7. State and Props:
React components can have both state and props. State is mutable and represents the internal data of a component. Props are immutable and are passed from parent components to child components.
8. One-Way Data Flow:
React imposes a one-way data flow, meaning that data flows in a mono direction—from parent to child components. Changes to the parent’s state will propagate to its child components, triggering re-rendering.
9. Component Reusability:
Components can be reused throughout the application, leading to a modular and maintainable codebase. This reusability encourages the development of a library of UI components.
10. Declarative Syntax:
React uses a declarative syntax, where you define what the UI should look like based on the current state, rather than detailing the steps to update it.
11. Virtual DOM Diffing:
When changes occur in the application, React compares the previous virtual DOM with the updated virtual DOM. It identifies which elements have changed and calculates the most efficient way to apply those changes to the actual DOM.
12. JSX Transformation:
During development, JSX code is transformed into JavaScript using tools like Babel. This transformed code includes calls to React functions that create and manage the virtual DOM.
React’s architecture revolves around its virtual DOM and component-based structure. It efficiently updates the UI by minimizing direct manipulation of the actual DOM and calculating the most efficient way to reflect changes. This approach contributes to React’s performance, reusability, and the ability to build complex user interfaces.
How to Install and Configure ReactJs?
To install and configure ReactJS, follow these steps:
- Install Node.js: React requires Node.js and npm (Node Package Manager) to manage dependencies. Install them from the official Node.js website: https://nodejs.org/
- Create a React App: Open your terminal and run the following command to create a new React app:
npx create-react-app my-demo
This will generate a new directory called my-
demo with a basic React project module.
- Navigate to the App Directory: Move to the newly created app directory:
cd my-demo
- Start the Development Server: Run the following command to start the development server and see your app in a web browser:
npm start
- Edit and Customize: You can now start editing the source files in the
src
directory to build your React app. The changes you make will automatically update in the browser.
Remember that this is a basic setup. As your project grows, you might want to configure additional tools and libraries for state management, routing, and more.
Fundamental Tutorials of ReactJs: Getting Started Step by Step
Certainly, here’s a step-by-step guide with more detailed explanations for fundamental concepts of ReactJS:
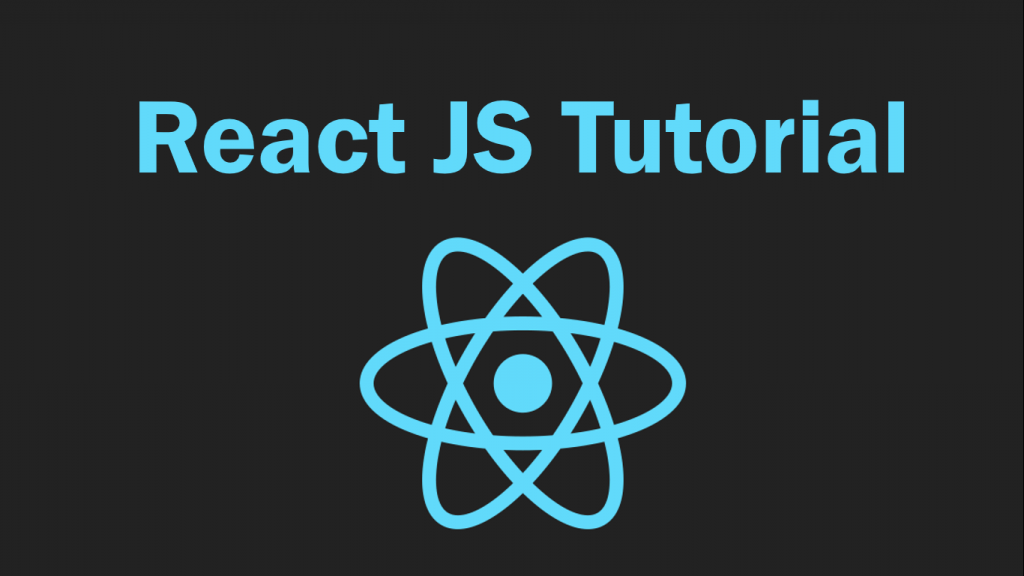
Step 1: Setup and Installation
- Install Node.js and npm: If you haven’t already, download and install Node.js from the official website: https://nodejs.org/
- Create a React App: Open your terminal and run the following command to create a new React app:
npx create-react-app my-react-app
This command sets up a new React project in a directory named my-react-app
.
- Navigate to the App Directory: Move to the newly created app directory:
cd my-react-app
- Start the Development Server: Run the following command to start the development server:
npm start
This command will start the development server and open your app in a web browser at http://localhost:3000
.
Step 2: Understanding Components
- Component Structure: In React, UIs are broken down into components. Open
src/App.js
to see the main component structure created bycreate-react-app
. This component is the root of your application. - Creating a Component: Create a new file named
MyWorld.js
in thesrc
folder. In this file, define a functional component:
import React from 'react';
function MyWorld() {
return <h1>Hello from MyWorld!</h1>;
}
export default MyWorld;
- Using Components: Import and use your custom component in
src/App.js
:
import React from 'react';
import MyWorld from './MyWorld';
function App() {
return (
<div>
<h1>Hello React App</h1>
<MyWorld />
</div>
);
}
export default App;
Step 3: JSX and Rendering
- JSX Syntax: JSX allows you to write HTML-like code within your JavaScript. This makes it easy to describe your UI elements:
const element = <h1>Hello, JSX!</h1>;
- Rendering JSX: Use curly braces
{}
to embed JavaScript expressions within JSX:
function Greeting(props) {
return <h1>Hello, {props.name}</h1>;
}
- Rendering Components: Components can be rendered just like HTML elements:
function App() {
return (
<div>
<Greeting name="Alice" />
<Greeting name="Bob" />
</div>
);
}
Step 4: State and Props
- State with
useState
: Use theuseState
hook to manage component state:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
- Props: Props allow passing data from a parent component to a child component:
function Welcome(props) {
return <h1>Hello, {props.name}</h1>;
}
function App() {
return <Welcome name="Alice" />;
}
Step 5: Handling Events
- Event Handling: Add event handlers like
onClick
,onChange
, etc., to elements:
function Button() {
const handleClick = () => {
alert('Button clicked!');
};
return <button onClick={handleClick}>Click Me</button>;
}
Step 6: Conditional Rendering
- Conditional Rendering: Use conditional statements to render content based on conditions:
function Greeting(props) {
if (props.isLoggedIn) {
return <h1>Welcome back!</h1>;
} else {
return <h1>Please log in.</h1>;
}
}
Step 7: Lists and Keys
- Lists and Keys: Render arrays of elements using
map
and provide a uniquekey
for each element:
function ListItems(props) {
const items = props.items.map(item => <li key={item.id}>{item.name}</li>);
return <ul>{items}</ul>;
}
Step 8: Styling Components
- Styling Components: You can apply styles using inline styles or CSS classes:
function StyledComponent() {
const style = {
backgroundColor: 'blue',
color: 'white',
padding: '10px 20px',
border: 'none',
cursor: 'pointer'
};
return <button style={style}>Styled Button</button>;
}
This comprehensive guide covers the fundamental concepts of ReactJS in a step-by-step manner. As you progress, explore more advanced topics like component lifecycle, hooks (e.g., useEffect
), context API, and state management libraries. Don’t forget to consult the official React documentation for in-depth information: https://reactjs.org/docs/getting-started.html
- Why Can’t I Make Create A New Folder on External Drive on Mac – Solved - April 28, 2024
- Tips on How to Become a DevOps Engineer - April 28, 2024
- Computer Programming Education Requirements – What You Need to Know - April 28, 2024