What is Regex?
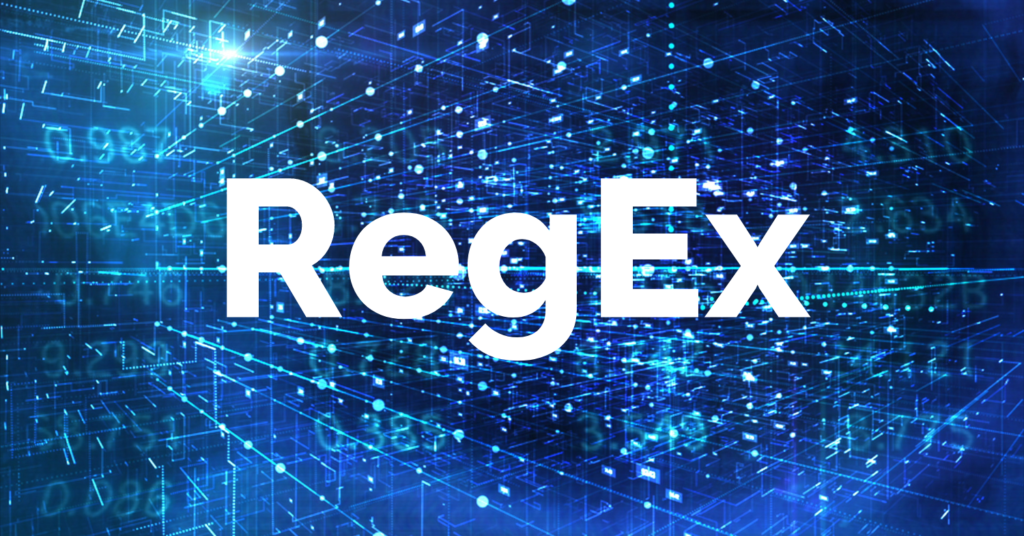
Regex, short for Regular Expression, is a sequence of characters that forms a search pattern. It is a powerful tool used for pattern matching within strings. Regular expressions deliver a flexible and concise way to search, match, and manipulate text.
Key Concepts in Regular Expressions:
- Metacharacters: Characters with special meanings in regex, such as
.
(any character),*
(zero or more occurrences),+
(one or more occurrences),[]
(character class), and()
(grouping). - Quantifiers: Specify the number of occurrences of a character or a group. Examples include
*
(zero or more),+
(one or more),?
(zero or one),{n}
(exactly n occurrences),{n,}
(n or more occurrences), and{n,m}
(between n and m occurrences). - Anchors: Identify the position in the string where a match must happen. Examples include
^
(start of a line) and$
(end of a line). - Character Classes: Define a set of characters. For example,
[aeiou]
matches any vowel, and[^0-9]
matches any non-digit. - Escape Characters: Use a backslash (
\
) to escape special characters and treat them as literal characters. - Groups and Capturing: Parentheses
()
are used to create groups, and the content of a group can be captured for later use.
What is top use cases of Regex ?
- Text Search and Validation:
- Search for specific patterns or words in a document or text file.
- Validate user input, such as email addresses, phone numbers, or passwords.
- Data Extraction:
- Extract information from strings or documents, such as extracting email addresses, URLs, or dates.
- String Manipulation:
- Replace or remove specific substrings from a text.
- Format and clean up text data.
- Data Validation in Forms:
- Validate user input in forms to ensure it adheres to a specific format (e.g., phone numbers, ZIP codes).
- Log File Analysis:
- Parse and analyze log files to extract relevant information.
- Search for specific error patterns or events.
- Web Scraping:
- Extract data from web pages by matching patterns in the HTML source code.
- Filter and process content retrieved through web scraping.
- Programming Code Analysis:
- Search for specific patterns in source code files.
- Replace or refactor code using regular expressions.
- Data Cleaning in Databases:
- Clean and standardize data in databases by applying regular expressions to text fields.
- Extract information from unstructured data in databases.
- URL Matching and Routing:
- Define URL patterns for routing in web applications.
- Extract parameters from URLs.
- Validation in Programming Languages:
- Use regular expressions in programming languages for string matching and validation tasks.
- Check if a string matches a specific pattern before further processing.
- Network Protocol Analysis:
- Analyze network traffic and filter packets based on specific patterns.
- Extract information from network protocol data.
- Natural Language Processing (NLP):
- Tokenize and process text in natural language processing applications.
- Identify patterns and entities in text data.
Regular expressions are a powerful tool for manipulation and text processing. While they can be very effective, they can also be complex, and creating them requires a good understanding of the syntax and patterns involved. There are online tools and resources to help users build and test regular expressions for specific use cases.
What are feature of Regex?
Features of Regular Expressions (Regex):
- Pattern Matching:
- Regex allows you to define patterns to match specific sequences of characters in a text.
- String Searching:
- It provides a powerful tool for searching for substrings or patterns within a larger text.
- Text Validation:
- Regex is commonly used for validating input strings against predefined patterns, such as email addresses, phone numbers, or passwords.
- Text Extraction:
- You can use regex to extract specific information from a text by capturing parts of the text that match certain patterns.
- Text Manipulation:
- Regex enables you to perform various text manipulations, such as find and replace, using patterns.
- Quantifiers:
- Quantifiers like
*
(zero or more occurrences),+
(one or more occurrences),?
(zero or one occurrence),{n}
(exactly n occurrences), and others allow for flexibility in matching.
- Quantifiers like
- Character Classes:
- Character classes, such as
[a-zA-Z]
or\d
(digits), allow you to specify groups of characters to match.
- Character classes, such as
- Anchors:
- Anchors like
^
(start of a line) and$
(end of a line) help define the position in the text where a match should occur.
- Anchors like
- Groups and Capturing:
- Parentheses
()
are used to create groups, and the content of a group can be captured for later use.
- Parentheses
- Escape Characters:
- Special characters can be escaped using the backslash (
\
) to treat them as literal characters.
- Special characters can be escaped using the backslash (
- Alternation:
- Alternation using the pipe (
|
) allows you to match one of several patterns.
- Alternation using the pipe (
- Assertions:
- Assertions like
(?=...)
(positive lookahead) and(?!...)
(negative lookahead) allow for more advanced matching conditions.
- Assertions like
- Greedy and Lazy Matching:
- Quantifiers can be greedy (matching as much as possible) or lazy (matching as little as possible) using
*?
,+?
,??
, etc.
- Quantifiers can be greedy (matching as much as possible) or lazy (matching as little as possible) using
What is the workflow of Regex?
Following is a workflow of Regular Expressions (Regex):
- Define the Objective:
- Clearly define the pattern or set of patterns you want to match or extract from the text.
- Construct the Regex Pattern:
- Create a regular expression pattern based on the defined objective. This involves using the appropriate regex syntax to specify the desired sequence of characters.
- Test the Regex:
- Use regex testing tools or platforms to test the regex pattern against sample texts. This helps ensure that the pattern matches the desired strings.
- Implement in Code or Tools:
- Once the regex pattern is validated, implement it in your code or use it in tools that support regular expressions. Popular programming languages, text editors, and command-line tools often support regex.
- Match or Extract:
- Apply the regex pattern to the target text. Depending on the context, you may want to check for matches, extract specific information, or validate the entire text.
- Iterate and Refine:
- If necessary, iterate and refine the regex pattern based on additional testing or changes in requirements. Regex patterns can be complex, and refinement may be needed to handle edge cases.
- Handle Edge Cases:
- Consider edge cases and potential variations in the text that the regex pattern should handle. Update the pattern accordingly.
- Optimize for Performance:
- Depending on the size of the text and the frequency of regex operations, consider optimizing the regex pattern for performance. This may involve fine-tuning quantifiers or using more efficient constructs.
- Document the Regex Pattern:
- Document the regex pattern in your code or project documentation. This is important for future maintenance and collaboration.
- Test in Different Environments:
- Test the regex pattern in different environments and with various datasets to ensure its robustness and compatibility.
- Monitor and Update:
- Regularly monitor the performance of regex patterns in production. If needed, update the patterns to accommodate changes in data or requirements.
Regular expressions are a powerful tool, but they can be intricate and may require careful testing and validation. Developing a solid understanding of regex syntax and its applications is key to effectively using it in various contexts.
How Regex Works & Architecture?
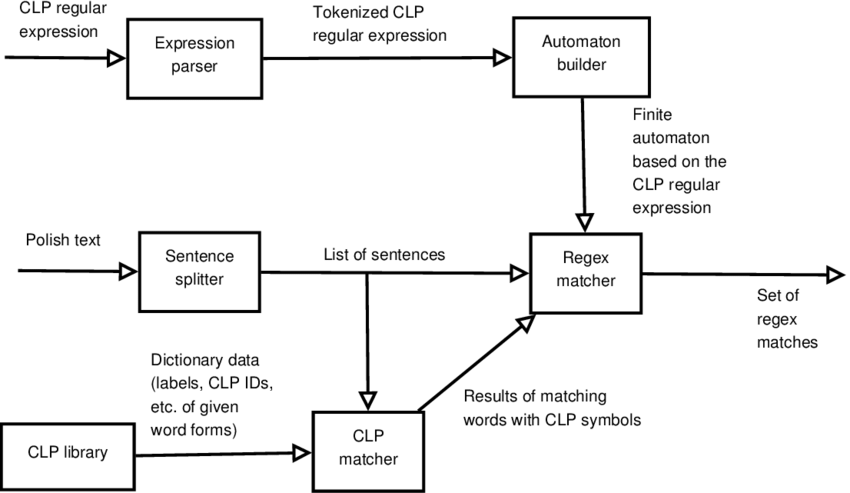
Regular expressions (regex) are a powerful tool for pattern matching and text processing. They are used in various applications, including text editors, programming languages, and search engines. Regex provides a concise and flexible way to search for, identify, and manipulate text based on specific patterns.
Regex Syntax and Components:
Regex syntax consists of a set of metacharacters, quantifiers, and grouping constructs that define the patterns to match.
- Metacharacters: Metacharacters have special meanings in regex, such as
.
,*
,+
,?
,^
, and$
. They represent specific patterns or control the matching behavior. - Quantifiers: Quantifiers specify the number of occurrences of a pattern element. Common quantifiers include
*
(zero or more times),+
(one or more times),?
(zero or one time), and{n}
(exactly n times). - Grouping Constructs: Grouping constructs allow for grouping pattern elements and applying quantifiers to the group. Parentheses
()
are used for grouping, and|
(or operator) allows for alternative matching within a group.
Regex Matching Process:
- Pattern Compilation: The regex pattern is compiled into an internal representation that the regex engine can efficiently process.
- Text Scanning: The regex engine scans the input text, comparing each character or sequence of characters against the compiled pattern.
- Pattern Matching: If the text matches the pattern, the regex engine identifies the matched portion of the text.
- Backtracking: In certain cases, the regex engine may backtrack to previous positions in the text to find alternative matches.
Regex Architecture:
The regex engine, the core component of regex functionality, implements the matching process. It typically consists of:
- Pattern Parser: Parses the regex pattern into an internal representation.
- NFA (Nondeterministic Finite Automaton): An NFA represents the regex pattern as a state machine that transitions based on input characters.
- DFA (Deterministic Finite Automaton): A DFA is a deterministic version of the NFA, used for efficient matching.
- Backtracking Mechanism: Handles backtracking during matching, allowing for alternative paths within the pattern.
- Capture Groups: Tracks and captures matching portions of the text, allowing for extraction and manipulation.
Applications of Regex:
- Text Editors: Regex is used for search and replace operations, syntax highlighting, and text validation.
- Programming Languages: Regex is embedded in programming languages for text processing, data validation, and input parsing.
- Search Engines: Regex is used for pattern-based indexing, search query matching, and relevance ranking.
- Data Validation: Regex is used to validate user input, ensure data integrity, and enforce formatting rules.
- Text Analysis: Regex is used for identifying patterns, extracting information, and analyzing text structures.
How to Install and Configure Regex?
Installing and configuring regular expressions (regex) depends on the specific programming language or environment you’re using. Here’s a general guide for installing and configuring regex in Python:
Installing Regex in Python:
Regular expressions are built into the Python standard library, so you don’t need to install any additional packages. The re
module provides the core regex functionality.
Configuring Regex in Python:
- Import the re module: To use regex functionality, import the
re
module in your Python script:
import re
- Define the regex pattern: Create a regex pattern string using the desired metacharacters, quantifiers, and grouping constructs.
- Compile the regex pattern: Compile the regex pattern into a regular expression object using the
re.compile()
function:
pattern = re.compile(r"[a-z]+")
- Apply the regex pattern: Use the
search()
,findall()
, orsub()
methods of the compiled regular expression object to perform pattern matching, searching, or text manipulation.
match = pattern.search("Hello, world!")
if match:
print("Found match:", match.group())
Example:
import re
# Define the pattern
pattern = r"\d+"
# Compile the pattern
regex = re.compile(pattern)
# Search for matches in a text string
text = "The quick brown fox jumps over the lazy dog."
matches = regex.findall(text)
# Print the matched numbers
print(matches)
This code will print the following output:
['1', '2', '3']
Fundamental Tutorials of Regex: Getting started Step by Step
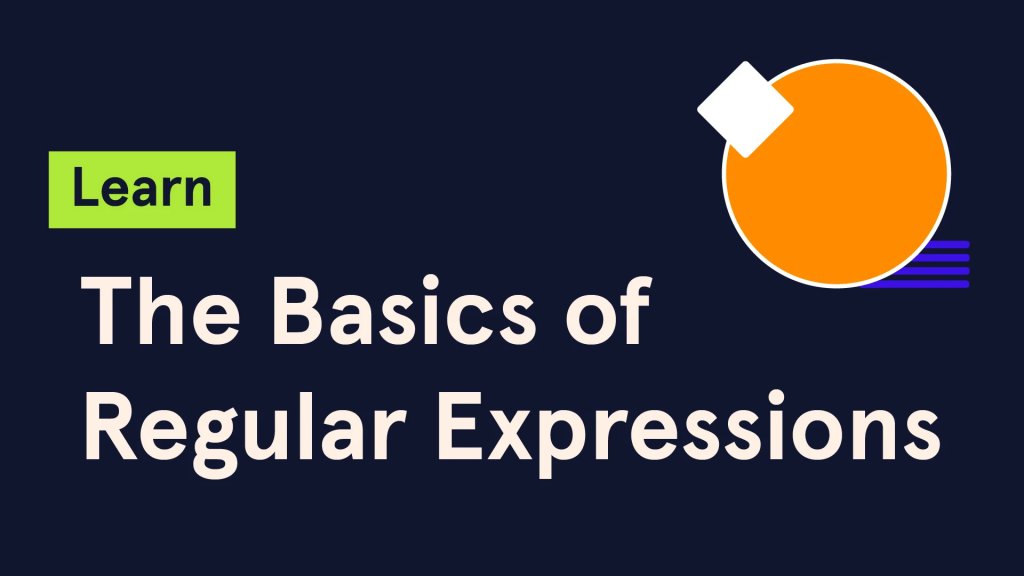
Following is a step-by-step fundamental tutorial of Regex (Regular Expressions), covering essential concepts and operations to get you started with this powerful pattern matching tool:
Step 1: Introduction to Regex
- What is Regex?: Regex, short for Regular Expressions, is a powerful tool for matching patterns in text. It’s used in various applications, including text processing, programming, and data validation.
- Why Use Regex?: Regex provides efficient and flexible pattern matching capabilities, allowing you to extract, search, and manipulate text based on specific patterns.
- Regex Flavors: Different programming languages and tools have their own Regex flavors, but the syntax and concepts are generally consistent.
Step 2: Basic Regex Metacharacters
- Character Literals: Match individual characters directly, such as ‘a’, ‘b’, or ‘1’.
- Dot (‘.’): Tests any single character except a newline character.
- Caret (‘^’) and Dollar Sign (‘$’): Text the beginning and end of the string, respectively.
- Square Brackets (‘[]’): Match a single character from a specified set of characters.
- Parentheses (‘()’): Group characters for grouping and capturing.
Step 3: Quantifiers
- Question Mark (‘?’): Makes the preceding character optional (matches zero or one occurrence).
- Asterisk (‘*’): Makes the preceding character zero or more times.
- Plus Sign (‘+’): Makes the preceding character one or more times.
- Curly Braces (‘{}’): Specify the exact number of repetitions of the preceding character or group.
Step 4: Character Classes
- Predefined Character Classes: Shorthand notations for common character sets, such as ‘\d’ (digits), ‘\w’ (word characters), ‘\s’ (whitespace).
- Negation in Character Classes: Use the caret (‘^’) within square brackets to negate the character set, matching characters not in the set.
- Character Ranges: Use hyphen (-) to define a range of characters within square brackets.
Step 5: Grouping and Capturing
- Parentheses (‘()’): Group characters for grouping and capturing, allowing selective matching and extracting substrings.
- Capturing Groups: Use parentheses to create capturing groups, which capture matched substrings for later use.
- Backreferences: Use ‘\1’, ‘\2’, etc., to refer to captured groups within the pattern.
Step 6: Practical Regex Applications
- Email Validation: Use Regex to validate email addresses, ensuring they follow the correct format.
- Phone Number Extraction: Extract phone numbers from text using Regex patterns specific to different phone number formats.
- Data Cleaning and Validation: Use Regex to clean and validate user input, ensuring it matches the desired format and constraints.
- Text Search and Replace: Perform efficient pattern-based search and replace operations on text using Regex.
- Password Strength Validation: Use Regex to enforce password strength requirements, such as minimum length, character variety, and special characters.
Remember, Regex is a vast and powerful tool, and these fundamental steps provide a starting point. By following these steps and dedicating time to practice, you’ll be well on your way to mastering regex and unlocking its vast potential for efficient text manipulation!
- PPG Industries: Selection and Interview process, Questions/Answers - April 3, 2024
- Fiserv: Selection and Interview process, Questions/Answers - April 3, 2024
- Estee Lauder: Selection and Interview process, Questions/Answers - April 3, 2024