What is Ruby?
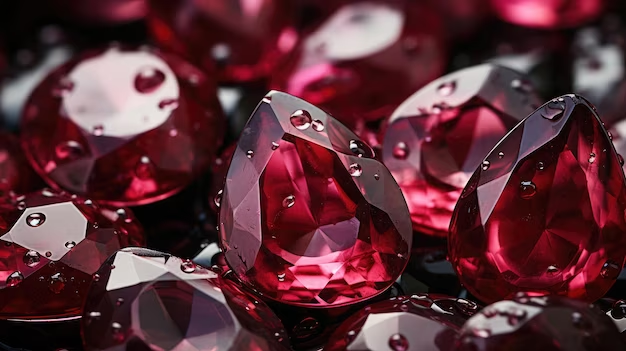
Ruby is a dynamic, object-oriented programming language that emphasizes productivity and simplicity. It was planned and developed by Yukihiro “Matz” Matsumoto in the mid-1990s. Ruby is known for its elegant syntax, readability, and a philosophy often summarized as “optimization for developer happiness.” It is often used for web development, scripting, and general-purpose programming.
Key Features of Ruby:
- Object-Oriented: Everything in Ruby is an object, including numbers and functions. It follows a pure object-oriented approach.
- Dynamic Typing: Ruby is dynamically typed, meaning that variable types are determined at runtime.
- Garbage Collection: Ruby features automatic memory management with a garbage collector, freeing developers from manual memory allocation and deallocation.
- Blocks and Closures: Ruby supports blocks, which are chunks of code passed to methods, and closures, which are blocks of code that can be passed around like objects.
- Metaprogramming: Ruby allows for a high degree of metaprogramming, enabling developers to write code that modifies itself or other code at runtime.
- Mixins: Ruby supports mixins, allowing developers to reuse code in a modular and flexible way.
- Readable and Elegant Syntax: Ruby’s syntax is designed to be easy to read and write, which contributes to its developer-friendly nature.
What is top use cases of Ruby?
Following is a top use cases of Ruby:
- Web Development (Ruby on Rails):
- Ruby on Rails (Rails): A popular web application framework built in Ruby. It follows the convention over configuration (CoC) and don’t repeat yourself (DRY) principles, making it efficient for building scalable and maintainable web applications.
- Scripting and Automation:
- Ruby is commonly used as a scripting language for automating repetitive tasks and system administration.
- Prototyping:
- Due to its expressiveness and ease of use, Ruby is often used for rapid prototyping of software projects.
- Testing:
- Ruby is widely used for writing tests, especially with testing frameworks like RSpec and Minitest.
- DevOps Tools:
- Many DevOps tools, such as Puppet and Chef, are written in Ruby. It is used for configuring and managing servers.
- Command-Line Utilities:
- Ruby is suitable for writing command-line utilities and small tools to streamline development workflows.
- Libraries and Gems:
- Ruby’s package manager, RubyGems, hosts a vast ecosystem of libraries and tools (gems) that can be easily integrated into Ruby projects.
- Data Analysis and Visualization:
- Ruby can be used for data analysis and visualization tasks, leveraging libraries such as Numo for numerical computing and visualization libraries.
- Game Development:
- While not as common as in some other languages, Ruby can be used for simple game development, especially with the Rubygame library.
- Educational Purposes:
- Ruby’s readability and simplicity make it a good choice for teaching programming concepts to beginners.
- Content Management Systems (CMS):
- Some content management systems, like Radiant CMS, are built using Ruby.
- Desktop Applications:
- Ruby can be used for developing desktop applications using frameworks like Shoes.
Ruby’s versatility, combined with its developer-friendly syntax, has contributed to its popularity in various domains. Its active and supportive community also plays a crucial role in the ongoing development and adoption of the language.
What are feature of Ruby?
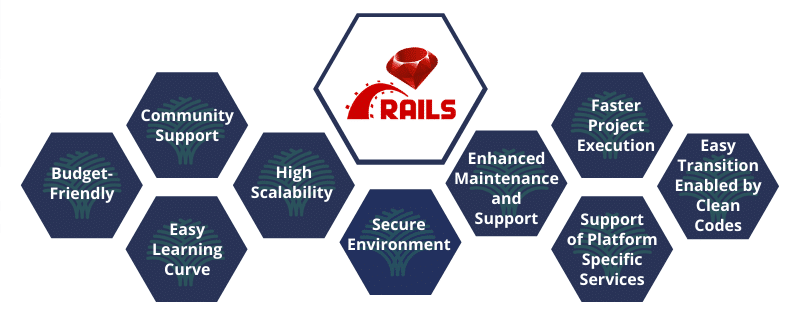
Features of Ruby:
- Object-Oriented:
- Ruby is a fully object-oriented language where everything is an object, and classes and objects are fundamental to its structure.
- Dynamic Typing:
- Ruby is dynamically typed, allowing for flexible and dynamic programming without explicit type declarations.
- Simple Syntax:
- Ruby’s syntax is designed to be clear and readable, emphasizing simplicity and developer-friendly code.
- Garbage Collection:
- Ruby includes automatic garbage collection, managing memory and freeing objects that are no longer in use.
- Mixins and Modules:
- Ruby supports mixins, allowing the inclusion of modules in classes for code reuse without multiple inheritance issues.
- Blocks and Procs:
- Blocks and Procs provide a way to create anonymous functions and pass them as arguments to methods.
- Closures:
- Ruby supports closures, allowing blocks of code to capture and remember the surrounding scope’s variables.
- Metaprogramming:
- Ruby allows dynamic modification and extension of classes and objects at runtime, enabling metaprogramming techniques.
- Community and Gems:
- Ruby has a vibrant and active community. The RubyGems package manager provides a vast ecosystem of libraries and tools.
- Rails Framework:
- Ruby on Rails (Rails) is a powerful web application framework built in Ruby, emphasizing convention over configuration (CoC) and don’t repeat yourself (DRY) principles.
- Duck Typing:
- Ruby follows the principle of duck typing, focusing on an object’s behavior rather than its type.
- Readable and Elegant Code:
- Ruby emphasizes writing code that is elegant, concise, and readable, contributing to the developer’s happiness.
- Open Classes:
- Ruby allows modification of existing classes during runtime, providing flexibility but requiring caution.
- Symbol and String Interchangeability:
- Ruby allows symbols and strings to be used interchangeably in many cases, providing flexibility in coding.
What is the workflow of Ruby?
Following is a workflow of Ruby:
- Install Ruby:
- Begin by installing Ruby on your system. You can use a version manager like RVM or rbenv for managing Ruby versions.
2. Text Editor/IDE:
- Choose a text editor or integrated development environment (IDE) for writing Ruby code. Popular choices include Visual Studio Code, Atom, or RubyMine.
3. Write Ruby Code:
- Create Ruby scripts or programs by writing code in your chosen editor. Ruby scripts typically have a
.rb
extension.
puts "Hello, Ruby!"
- Run Ruby Code:
- Execute Ruby scripts using the Ruby interpreter. Open a terminal, navigate to the script’s directory, and run:
ruby your_script.rb
- Explore Interactive Ruby (IRB):
- Utilize Interactive Ruby (IRB), an interactive Ruby shell, for experimenting with Ruby code snippets and exploring language features.
irb
- Use Gems:
- Leverage RubyGems to install and manage third-party libraries (gems) that extend Ruby’s functionality.
gem install gem_name
- Learn Ruby on Rails (Optional):
- If web development is of interest, explore Ruby on Rails. Install Rails and generate a new project.
gem install rails
rails new your_project
- Create and Run Rails Applications:
- Build web applications using Ruby on Rails. Follow the Rails conventions for structuring and organizing code.
rails server
- Test and Debug:
- Write tests for your Ruby code using testing frameworks like Minitest or RSpec. Use debugging tools and techniques when needed.
- Version Control:
- Use version control systems like Git to track changes in your code and collaborate with others.
- Documentation:
- Document your Ruby code using comments and follow best practices for code documentation.
- Community Engagement:
- Engage with the Ruby community by participating in forums, attending meetups, and contributing to open-source projects.
- Continuous Learning:
- Stay updated with the Ruby language and ecosystem. Explore new gems, tools, and practices to enhance your skills.
- Deployment:
- Deploy Ruby applications to servers or cloud platforms when ready for production use.
Ruby’s workflow is flexible and adaptable to various types of projects, from scripting and automation to web development. It encourages a clean and expressive coding style, and its rich ecosystem provides tools and libraries for diverse application domains.
How Ruby Works & Architecture?
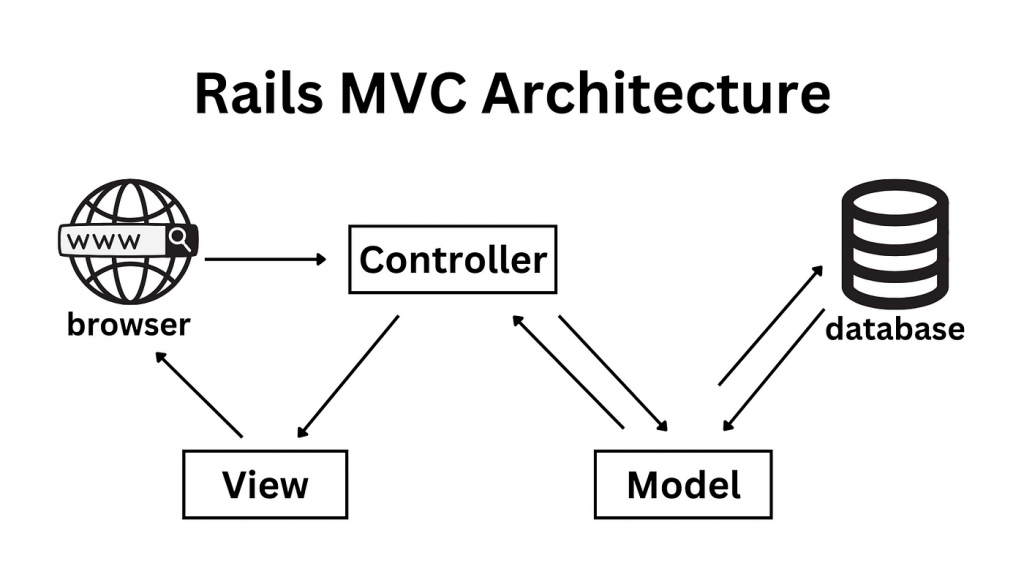
It is known for its elegant syntax, powerful features, and extensive standard library. Ruby is widely used for web development, scripting, and general-purpose programming.
Ruby’s Architecture
Ruby’s architecture is designed to be flexible, dynamic, and extensible. It consists of several key components:
- MRI (Matz’s Ruby Interpreter): The core interpreter that executes Ruby code.
- RubyVM (Ruby Virtual Machine): The runtime environment that manages memory allocation, garbage collection, and other runtime tasks.
- C Ruby Kernel: The foundation of Ruby’s functionality, providing essential classes and methods like Object, Array, Hash, and String.
- Standard Library: A vast collection of modules and classes that provide a wide range of functionalities, including networking, file I/O, and data structures.
- Gems: The package management system for Ruby, allowing the installation and management of third-party libraries and extensions.
How Ruby Works
Ruby’s execution process can be summarized as follows:
- Source Code Compilation: The MRI parses the Ruby source code and converts it into an intermediate bytecode representation.
- Bytecode Execution: The RubyVM executes the bytecode, performing operations like method calls, variable assignments, and control flow statements.
- Dynamic Dispatch: Ruby’s dynamic dispatch mechanism allows for late binding of methods, enabling flexible and adaptable code.
- Garbage Collection: Ruby’s garbage collection mechanism automatically manages memory allocation and deallocation, ensuring efficient memory usage.
- Exception Handling: Ruby provides a robust exception handling mechanism to manage errors and unexpected program behavior.
Ruby’s Object-Oriented Programming (OOP) Features
Ruby is a pure object-oriented language, meaning that everything in Ruby is an object. This OOP approach provides several benefits:
- Encapsulation: Objects encapsulate data and behavior, promoting modularity and code organization.
- Inheritance: Objects can inherit properties and methods from other objects, enabling code reusability and extensibility.
- Polymorphism: Objects of different classes can respond to the same method calls with different behaviors, promoting flexibility and adaptability.
Ruby’s Applications
Ruby is a versatile language used in various domains, including:
- Web Development: Ruby on Rails is a popular framework for building web applications, known for its rapid development capabilities and MVC (Model-View-Controller) architecture.
- Scripting: Ruby is widely used for scripting tasks due to its concise syntax and powerful tools like Rake and RSpec.
- General-Purpose Programming: Ruby is also used for general-purpose programming tasks, such as data analysis, scientific computing, and network programming.
Ruby’s simplicity, flexibility, and powerful features have made it a popular choice for programmers of all levels. Its extensive standard library and vast ecosystem of third-party libraries further expand its capabilities and make it a versatile tool for a wide range of applications.
How to Install and Configure Ruby?
Installing and configuring Ruby is a straightforward process. Here’s a step-by-step guide for both Windows and macOS systems:
Installing Ruby on Windows:
Prerequisites:
- Administrator Privileges: You’ll need administrator privileges to install Ruby.
Installation Steps:
- Download Ruby Installer: Download the Ruby installer for Windows from the official RubyInstaller website
- Run Ruby Installer: Run the downloaded Ruby installer executable file.
- Follow Installation Wizard: Follow the on-screen instructions in the installation wizard.
- Select Installation Options: Choose the desired installation options, such as installation location, Ruby version, and additional components.
- Complete Installation: Press “Install” to begin the installation process. Once completed, Ruby will be installed on your system.
Configuring Ruby on Windows:
- Add Ruby to Path: To use Ruby commands from any directory, add the Ruby installation directory to the system PATH environment variable.
- Set RubyGems Source: Set the RubyGems source to a reliable repository for RubyGems packages. You can use the following command:
gem sources -a https://rubygems.org
- Update RubyGems: Update the RubyGems package manager to ensure you have the latest versions of gems:
gem update --system
Installing Ruby on macOS:
Prerequisites:
- Homebrew: Homebrew is a package manager for macOS. If you haven’t installed Homebrew yet, you can install it by following the instructions on the official website.
Installation Steps:
- Install Ruby: Open Terminal and run the following command to install Ruby with Homebrew:
brew install ruby
- Set Ruby as Default: Set Ruby as the default Ruby interpreter to use:
ruby -e "$(curl -fsSL [https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh])"
Configuring Ruby on macOS:
- Set RubyGems Source: Set the RubyGems source to a reliable repository for RubyGems packages. You can apply the following command:
gem sources -a https://rubygems.org
- Update RubyGems: Update the RubyGems package manager to ensure you have the latest versions of gems:
gem update --system
Fundamental Tutorials of Ruby: Getting started Step by Step
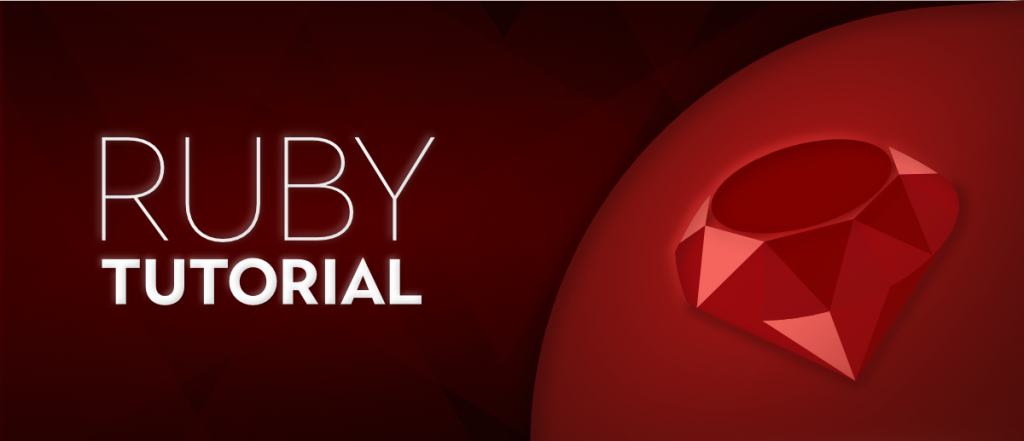
Let’s have a look at a step-by-step fundamental tutorial of Ruby, covering essential concepts and operations to get you started with this dynamic programming language:
Step 1: Setting Up the Ruby Environment
Before you begin your Ruby journey, ensure you have the Ruby programming language installed on your system. The installation process may vary depending on your operating system. For Windows users, RubyInstaller is a recommended tool. For macOS users, you can use Homebrew.
Once Ruby is installed, verify its installation by opening a command prompt or terminal and typing ruby -v
. This should display the installed Ruby version.
Step 2: Introduction to Basic Syntax
Ruby’s syntax is known for its simplicity and elegance. Let’s explore some basic syntax elements:
- Comments: Comments are used to explain code or provide non-executable notes. Single-line comments start with the ‘#’ character. Multi-line comments use the ‘begin’ and ‘end’ keywords.
- Variables: Variables are containers for storing data. Declare variables using assignment statements. For example,
name = "Alice"
assigns the string “Alice” to the variablename
. - Data Types: Ruby supports various data types, including Integer, Float, String, Boolean, and Arrays. Each data type represents a different kind of information.
- Operators: Ruby provides arithmetic operators (+, -, *, /), comparison operators (==, !=, <, >, <=, >=), and logical operators (&&, ||, !).
Step 3: Control Flow Statements
Control flow statements determine the order in which code is executed. Let’s explore some common control flow statements:
- Conditional Statements: Use ‘if’ and ‘else’ statements to decide which code to execute based on a condition. For example,
if age >= 18
checks ifage
is greater than or equal to 18. - Loops: Loops allow repetitive execution of code blocks. Use ‘while’ and ‘until’ loops for conditional iteration, and ‘for’ loops for iterating over collections.
- Blocks: Blocks are closures that encapsulate code and can be passed as arguments or stored in variables. They provide flexibility in code organization.
Step 4: Objects and Classes
Object-oriented programming (OOP) is a fundamental concept in Ruby. Let’s delve into objects and classes:
- Objects: Objects are instances of classes. They encapsulate data (attributes) and behavior (methods).
- Classes: Classes serve as designs for creating objects. They define the attributes and methods that objects of that class will possess.
- Creating Objects: Use the ‘new’ method of a class to create an instance of that class. For example,
person = Person.new("Bob", 30)
creates an object of the classPerson
with attributesname
andage
. - Accessing Object Attributes and Methods: Use the dot operator (‘.’) to access object attributes and methods. For instance,
person.name
retrieves thename
attribute of theperson
object.
Step 5: Modules and Methods
Modules and methods are essential components of Ruby’s OOP structure:
- Modules: Modules provide a way to organize and group related methods and constants. They promote code reusability and modularity.
- Including Modules: Use the ‘include’ keyword to mix the methods of a module into a class. This allows the class to utilize the module’s functionalities.
- Method Definitions: Define methods using the ‘def’ keyword, followed by the method name, parameters, and code block. Methods encapsulate specific actions or behaviors.
- Method Invocation: Call methods using the dot operator (‘.’) on an object or using the class name and method name directly. This invokes the method’s defined behavior.
Step 6: RubyGems and Libraries
RubyGems is Ruby’s package manager for installing and managing third-party libraries:
- Installing Gems: Use the
gem install
command followed by the gem name to install gems from the RubyGems repository. - Requiring Gems: Use the
require
statement to load the required gems in your code. This makes the gem’s features accessible. - Using Gem Libraries: Access and utilize the features provided by the installed gems. Explore the documentation of each gem to understand its functionalities.
Remember, this is a fundamental introduction to Ruby. Continue learning and exploring more advanced concepts, such as object-oriented programming principles, data structures, file I/O, networking, and web development frameworks like Ruby on Rails.
- Mutual of Omaha: Selection and Interview process, Questions/Answers - April 15, 2024
- AES: Selection and Interview process, Questions/Answers - April 15, 2024
- Amphenol: Selection and Interview process, Questions/Answers - April 15, 2024